Arduino Nano - Relay
In a previous tutorial, we discovered how to switch on/off an LED. This tutorial instructs you how to turn on/off devices that use a high voltage power supply, like a light bulb, fan, electromagnetic lock, linear actuator, and so on by using Arduino Nano.
? What are the commons and differences between controlling an LED and controlling a light bulb by using Arduino?
The common: Similar to controlling an LED, we can utilize the Arduino's output pin to switch them on or off.
The difference:
- LEDs can be powered from the Arduino Nano board. This means they can be connected directly to an Arduino Nano pin.
- A light bulb requires a different power source (high voltage and/or high current), which could damage the Arduino Nano. Therefore, it cannot be connected directly to an Arduino Nano pin. A relay must be used between the Arduino Nano pin and the light bulb to protect the Arduino Nano from the high voltage/current.
Hardware Preparation
Or you can buy the following kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables .
Overview of Relay
A relay is an electrically programmable switch that can be managed by Arduino Nano or any micro-controller. It is used to control the on/off state of devices that use high voltage and/or high current in a programmatic manner.
It acts as a bridge between Arduino Nano and high voltage devices.
WARNING
When creating projects that involve mains voltage, it is essential to have a thorough understanding of the task at hand. Otherwise, you may be at risk of electric shock. This is a serious matter and we urge you to take all necessary precautions. If you are not completely confident in your abilities, please refrain from attempting the task and seek assistance from someone who is knowledgeable.
Although some relays are compatible with both DC and AC devices, we highly recommend using a DC device with a voltage of 24V or less for testing.
Relay Pinout
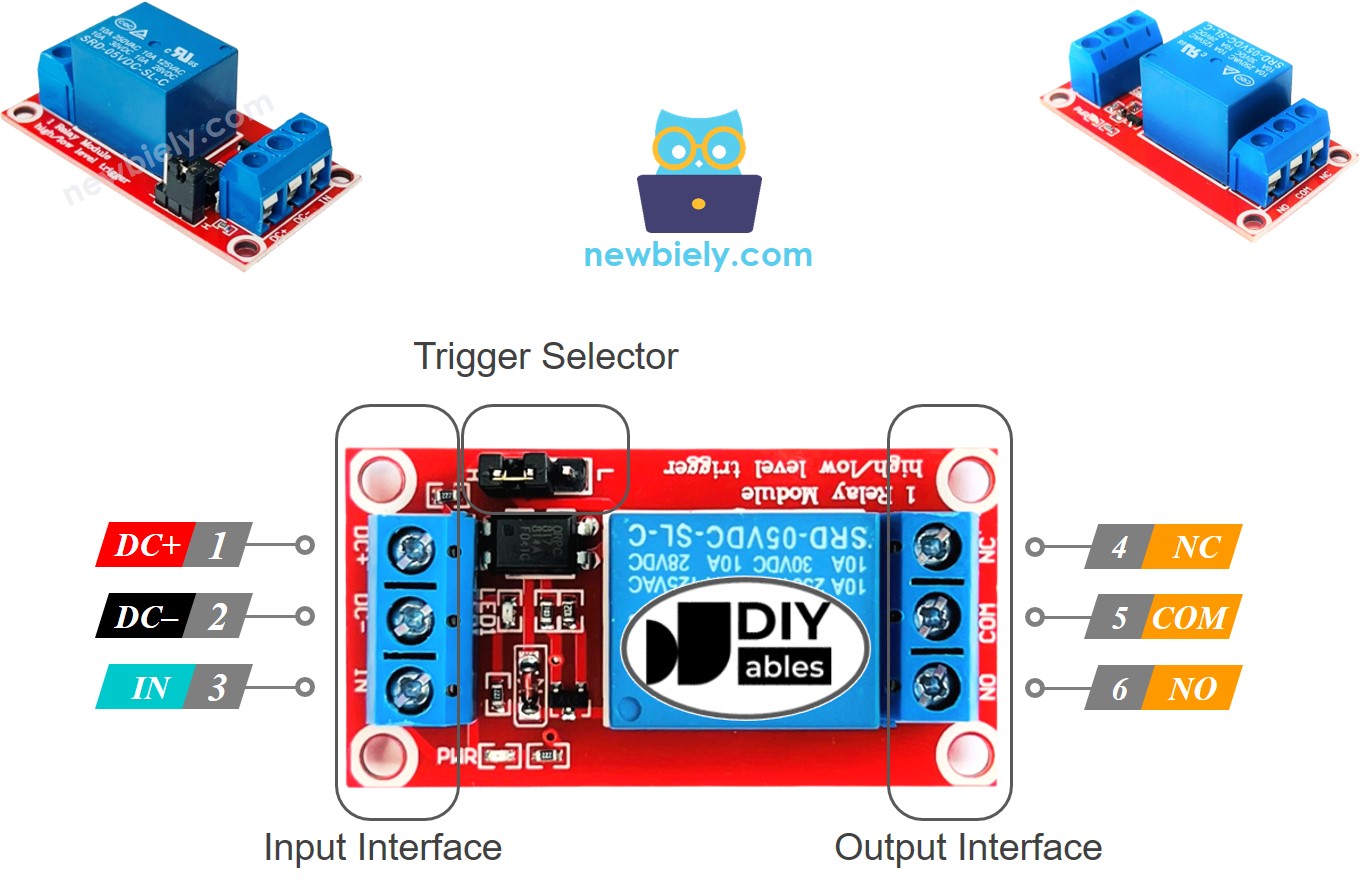
Relay has two sets of pins: one for input (low voltage) and the other for output (high voltage).
- The input group is connected to Arduino Nano, comprising three pins:
- DC- pin: must be linked to GND (0V)
- DC+ pin: must be linked to VCC (5V)
- IN pin: receives the control signal from Arduino Nano
- The output group is connected to the high voltage device, consisting of three pins (usually in screw terminal):
- NO pin: is normally open pin. It is used in the normally open mode
- NC pin: is normally closed pin. It is used in the normally closed mode
- COM pin: is the common pin. It is used in both normally open and normally closed modes
- If we use the normally open mode, we employ only the COM pin and the NO pin.
- If we use the normally closed mode, we use only the COM pin and the NC pin.
- LOW level trigger mode
- HIGH level trigger mode
- normally open mode
- normally closed mode. These modes are opposite to each other.
- The normally open and normally closed modes operate inversely
- Most relay modules support both normally open and normally closed modes
- The LOW level trigger and HIGH level trigger modes work inversely
- Not all relay modules support both LOW level trigger and HIGH level trigger modes
- At any given time, the relay module can only be in one of the two LOW level trigger and HIGH level trigger modes
- Linking an Arduino's pin to the IN pin of the relay
- Programming the pin to LOW or HIGH to control the relay
In practice, we do not usually utilize all of the pins in the high voltage group. We only use two of them:
Furthermore, if the relay has both LOW and HIGH level triggers, it typically has a jumper to pick either LOW level trigger or HIGH level trigger.
※ NOTE THAT:
Be sure to always refer to the labels printed on the relay module as the order of the pins can vary between manufacturers. Pay close attention!
How to Connect the High Voltage Device to Relay
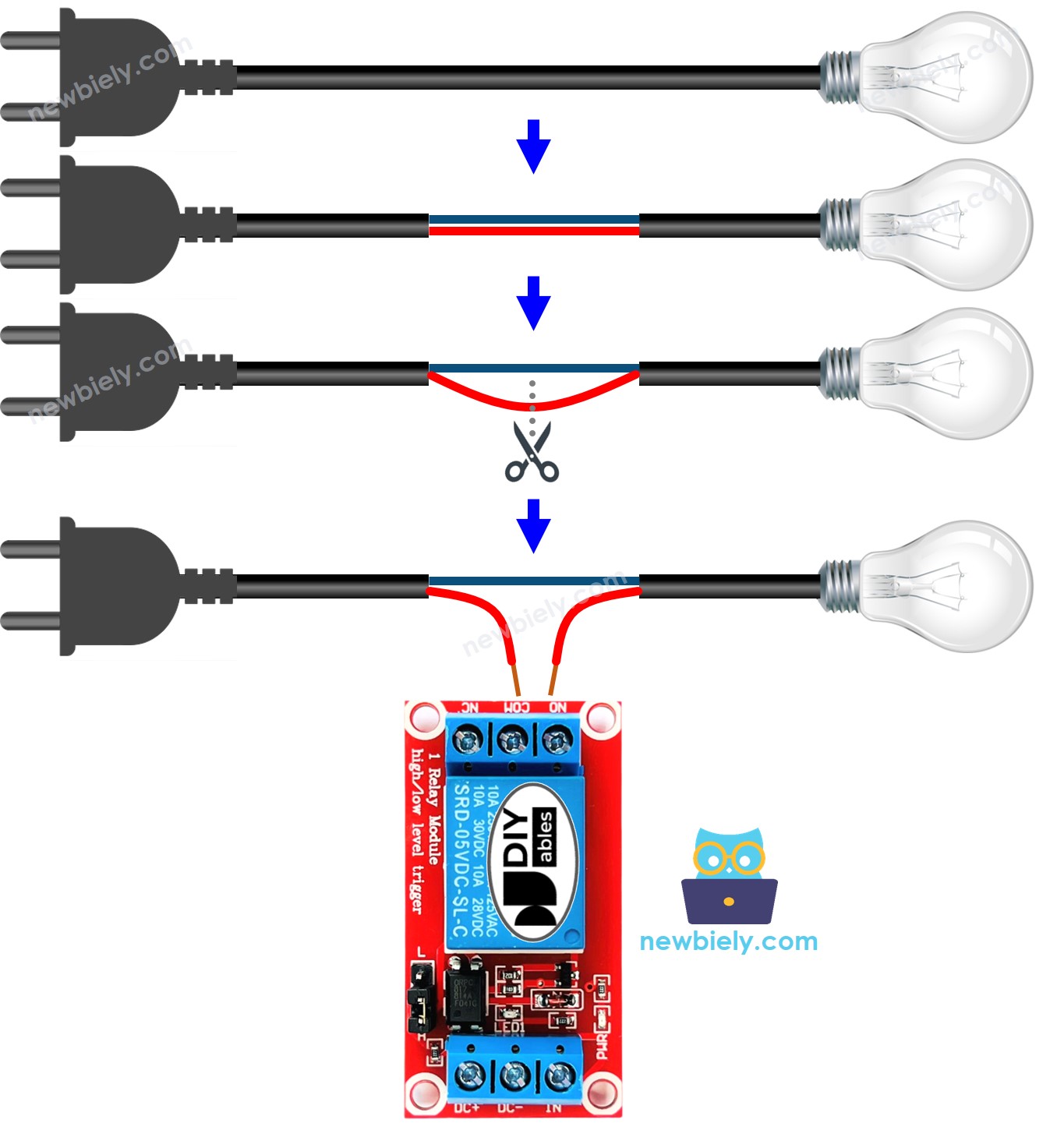
How It Works
The way a relay functions may vary, depending on the manufacturer and how it is installed by the user.
The input mode (for IN pin): There are two modes which cause the relay to operate in opposite ways:
The output mode (for output pins): There are two different modes that cause the relay to operate inversely:
The word "normally" implies that if the IN pin is connected to LOW (0V).
Before we go into detail, here are some quick facts:
The combination of input modes and output modes generates numerous applications. If you are a novice, we advise utilizing HIGH level trigger mode and normally open mode.
The HIGH level trigger mode will be explained in detail as it works in the opposite way to the LOW level trigger. The LOW level trigger operates differently.
HIGH Level Trigger - Normally Open Mode
To utilize this mode, we must attach the high voltage device to the COM pin and NO pin.
If the IN pin is linked to LOW (0V), the switch is in an open state. The device is OFF (or not functioning).
When the IN pin is connected to HIGH (5V), the switch is in a closed position. The device is ON (or working).
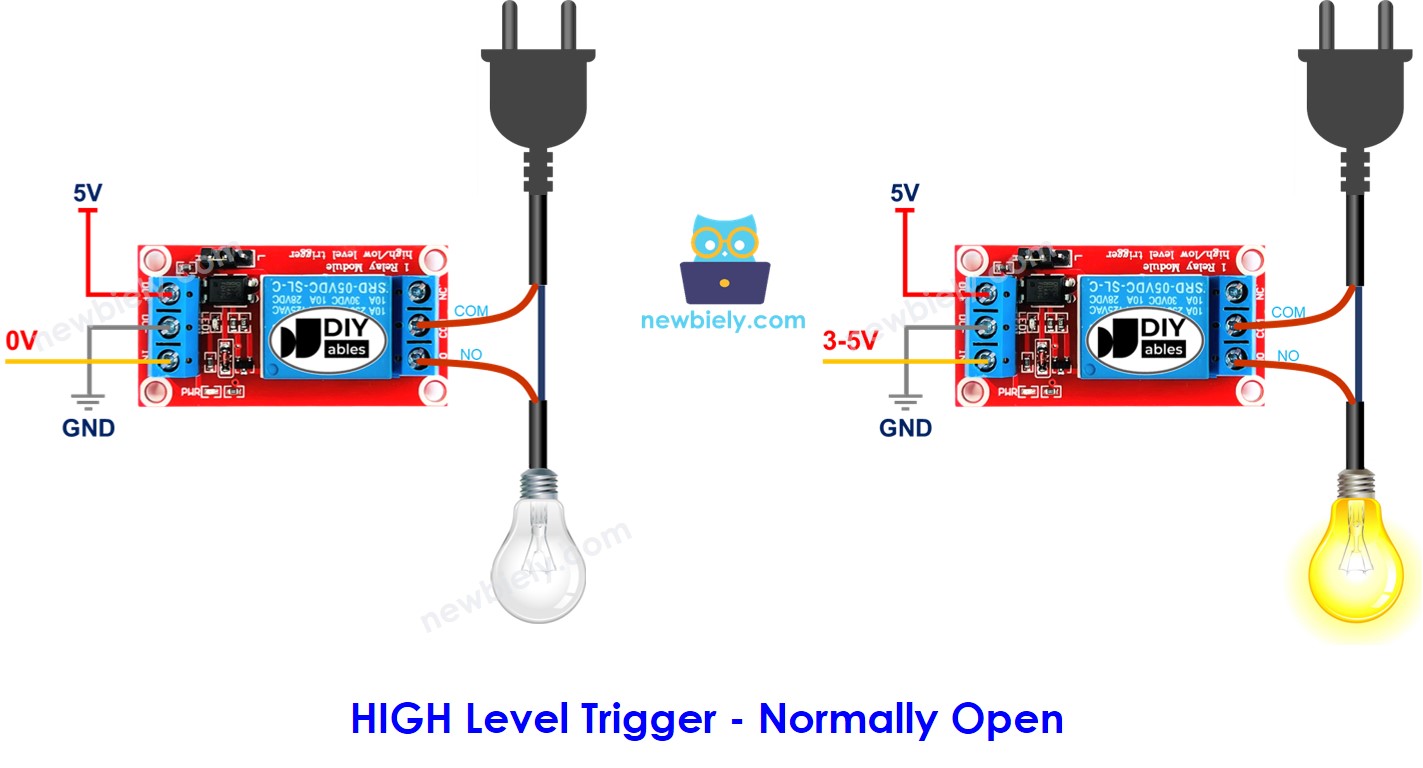
HIGH Level Trigger - Normally Closed Mode
In order to employ this mode, the high voltage device must be connected to the COM and NC pins.
When the IN pin is linked to LOW (0V), the switch is shut. This means the device is ON (or active).
On the other hand, if the IN pin is linked to HIGH (5V), the switch is open. This implies the device is OFF (or inactive).
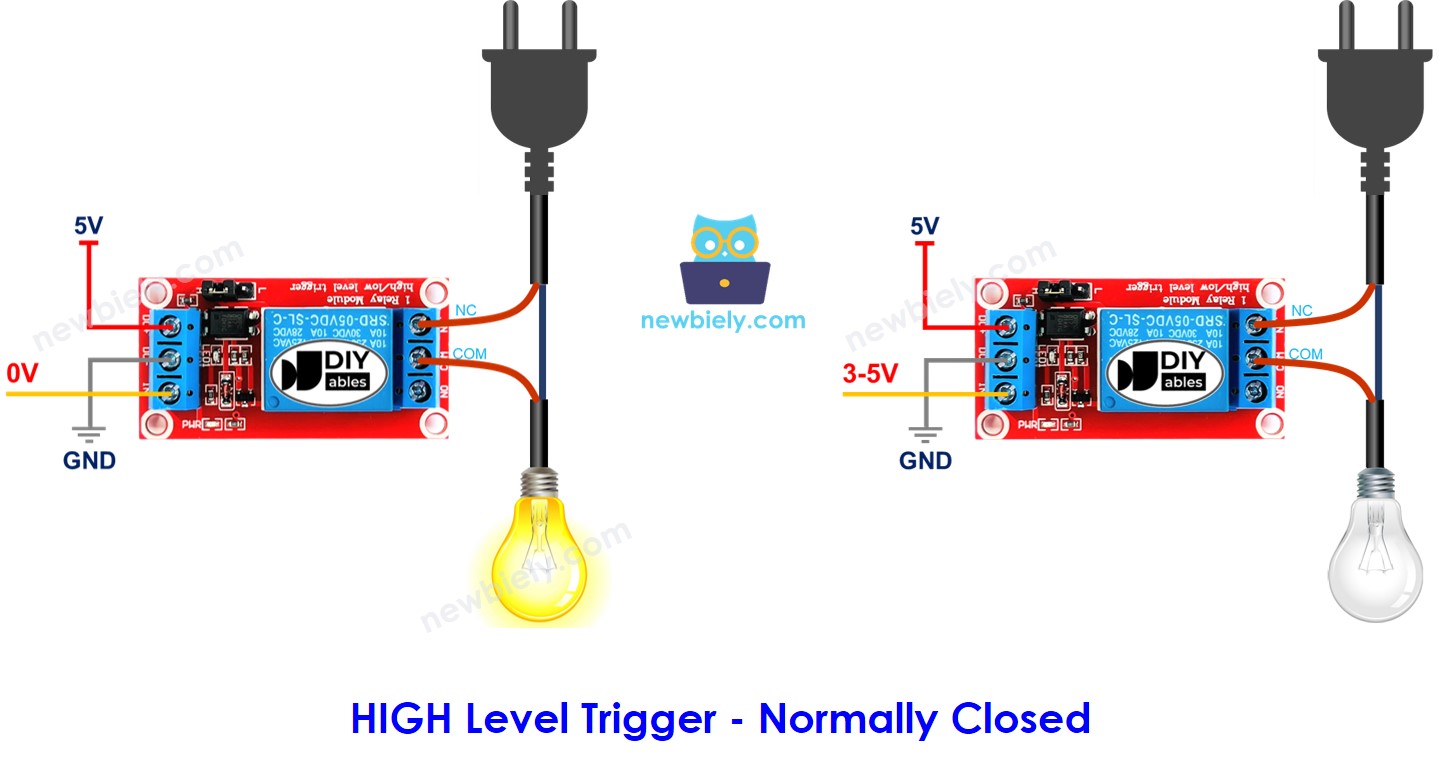
Summary
Input modes | Output Modes | IN pin (programmable) | Output pins | Relay state | Device state |
---|---|---|---|---|---|
HIGH Trigger | Normally Open | LOW | COM and NO pin | ⇒ open | ⇒ OFF |
HIGH Trigger | Normally Open | HIGH | COM and NO pin | ⇒ closed | ⇒ ON |
HIGH Trigger | Normally Closed | LOW | COM and NC pin | ⇒ closed | ⇒ ON |
HIGH Trigger | Normally Closed | HIGH | COM and NC pin | ⇒ open | ⇒ OFF |
LOW Trigger | Normally Open | LOW | COM and NO pin | ⇒ closed | ⇒ ON |
LOW Trigger | Normally Open | HIGH | COM and NO pin | ⇒ open | ⇒ OFF |
LOW Trigger | Normally Closed | LOW | COM and NC pin | ⇒ open | ⇒ OFF |
LOW Trigger | Normally Closed | HIGH | COM and NC pin | ⇒ closed | ⇒ ON |
There are a maximum of 8 use cases. It may be overwhelming. However, if you are a beginner, you only need to worry about the first two, which involve HIGH level trigger and normally open. The remainder of this tutorial will focus on those two use cases.
Arduino Nano - Relay
Arduino Nano manages a relay which in turn controls a high voltage device.
Managing a relay is straightforward. All that is required is:
Wiring Diagram
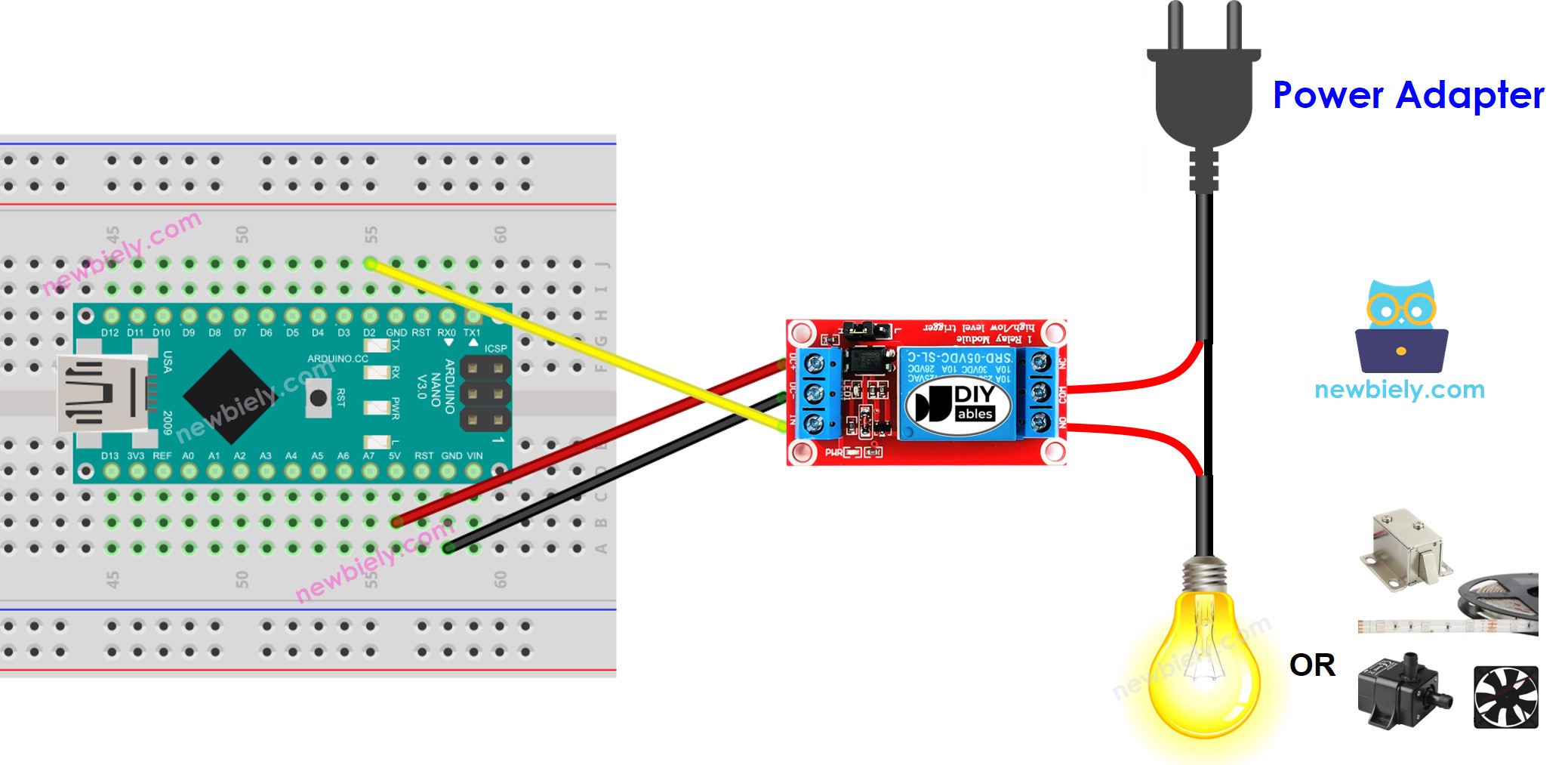
This image is created using Fritzing. Click to enlarge image
See The best way to supply power to the Arduino Nano and other components.
How To Program For Relay
- Set the Arduino's pin 2 to digital output mode by utilizing the pinMode() function. For example:
- Set the pin to 0V using the digitalWrite() function:
- Set the pin to 5V by utilizing the digitalWrite() function:
Arduino Nano Code
Detailed Instructions
- Copy the code and open it with the Arduino IDE.
- Click the Upload button on the Arduino IDE to compile and upload the code to the Arduino Nano.
- Check out the LED strip, it should be blinking.
Video Tutorial
Challenge Yourself
- When you enter your room, the light will be switched on automatically. After 30 seconds of your departure, the light will be turned off. For more information, please refer to Arduino Nano - Motion Sensor.