Arduino Nano - RS422
In this tutorial, we'll explore the process of establishing RS422 communication with an Arduino Nano. We'll cover the following steps in detail:
- Establishing the connection between the Arduino Nano and the TTL to RS422 module.
- Programming the Arduino Nano to receive data from the TTL to RS422 module.
- Programming the Arduino Nano to transmit data to the TTL to RS422 module.
- Performing bidirectional data transmission between your PC and the Arduino Nano via RS422.
The tutorial also provides the instruction for both Hardware Serial and SoftwareSerial.
Hardware Preparation
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables .
Overview of TTL to RS422 Module
When employing serial communication on the Arduino Nano using functions such as Serial.print(), Serial.read(), and Serial.write(), data transmission occurs via the TX pin while data reception takes place through the RX pin. These pins operate at TTL level, meaning they handle signals with limited range. Consequently, for serial communication over extended distances, it becomes necessary to convert the TTL signal to standards such as RS232, RS422, or RS485.
In this tutorial, we'll explore the integration of RS422 (also referred to as RS-422) with the Arduino Nano, achieved through the utilization of a TTL to RS422 module. This module facilitates the conversion of TTL signals to RS422 signals and vice versa.
Pinout
The RS422 to TTL module has two interfaces:
- The TTL interface (connnected to Arduino Nano) includes 4 pins
- VCC pin: power pin, needs to be connected to VCC (5V, or 3.3V)
- GND pin: power pin, needs to be connected to GND (0V)
- RXD pin: data pin, needs to be connected a TX pin of Arduino Nano
- TXD pin: data pin, needs to be connected a RX pin of Arduino Nano
- The RS422 interface comprises the following pins:
- A (R+) pin: RX+ pin of the module, connect this pin to TX+ pin (T+ or Y pin) of the other RS422 device.
- B (R-) pin: RX- pin of the module, connect this pin to TX- pin (T- or Z pin) of the other RS422 device.
- Y (T+) pin: TX+ pin of the module, connect this pin to RX+ pin (R+ or A pin) of the other RS422 device.
- Z (T-) pin: TX- pin of the module, connect this pin to RX- pin (R- or B pin) of the other RS422 device.
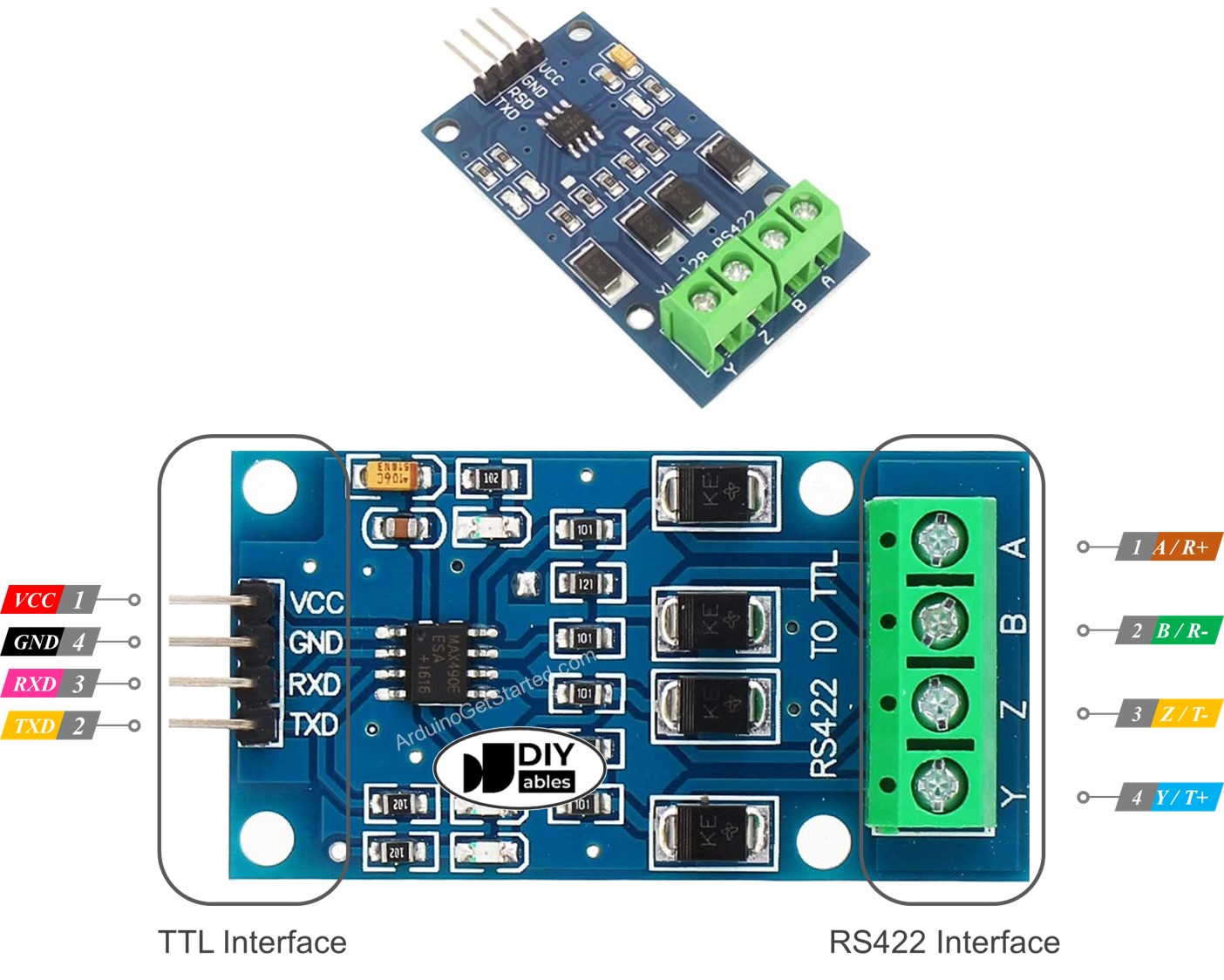
Wiring Diagram
- Wiring diagram if using hardware serial
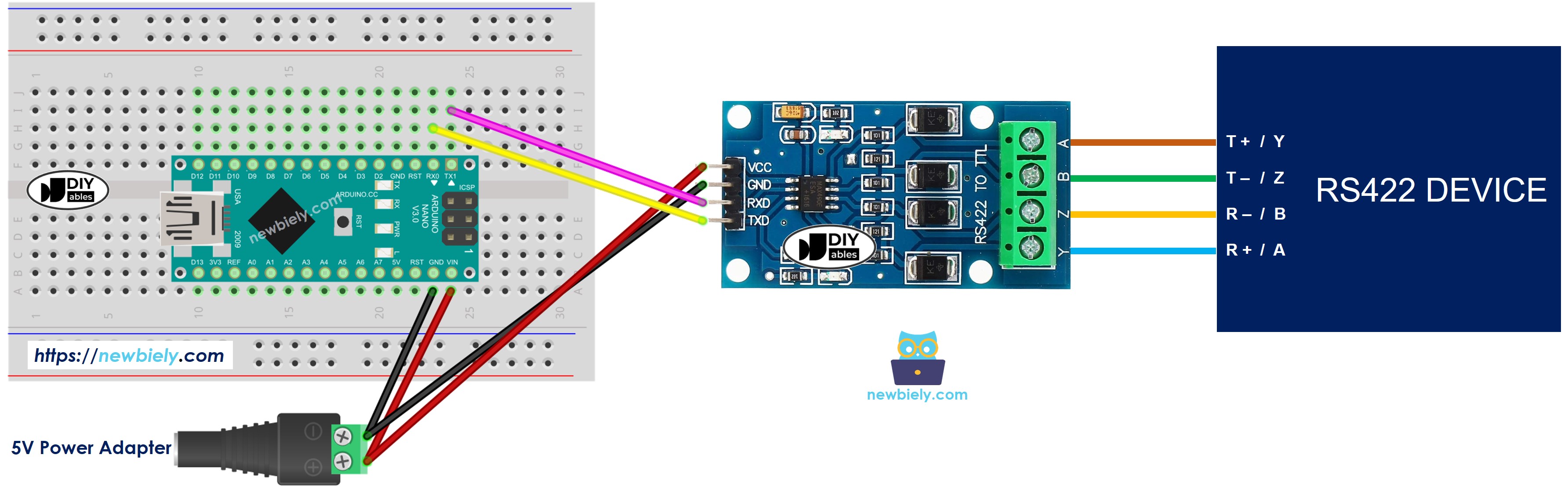
This image is created using Fritzing. Click to enlarge image
- Wiring diagram if using software serial
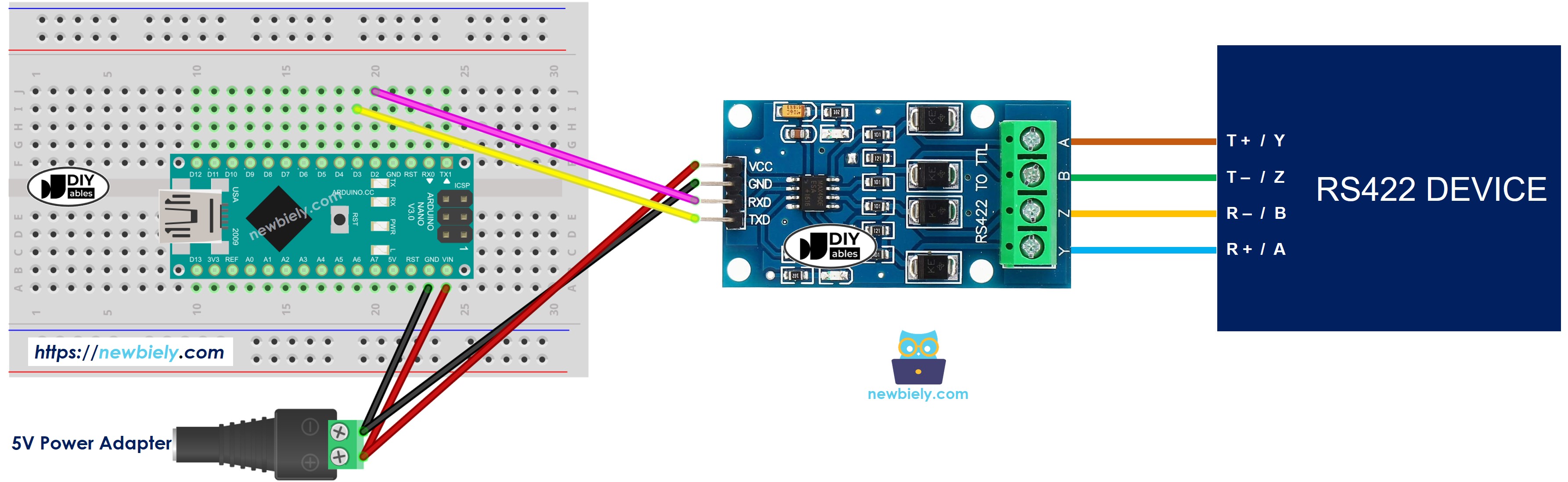
This image is created using Fritzing. Click to enlarge image
See The best way to supply power to the Arduino Nano and other components.
How To Program Arduino Nano to use the RS422 module
- Initializes the Serial interface:
- If you use SoftwareSerial, you need to include the library and declare a SoftwareSerial object:
- To read data come from RS422, you can use the following functions:
- To write data to RS422, you can use the following functions:
- And more functions to use with RS422 in Serial reference
Arduino Nano Code for Hardware Serial
Arduino Nano Code for Software Serial
Testing
You can do a test by sending data from your PC to Arduino Nano via RS-422 and vice versa. To do it, follow the below steps:
- Connect Arduino Nano to your PC via RS422-to-USB cable as below:
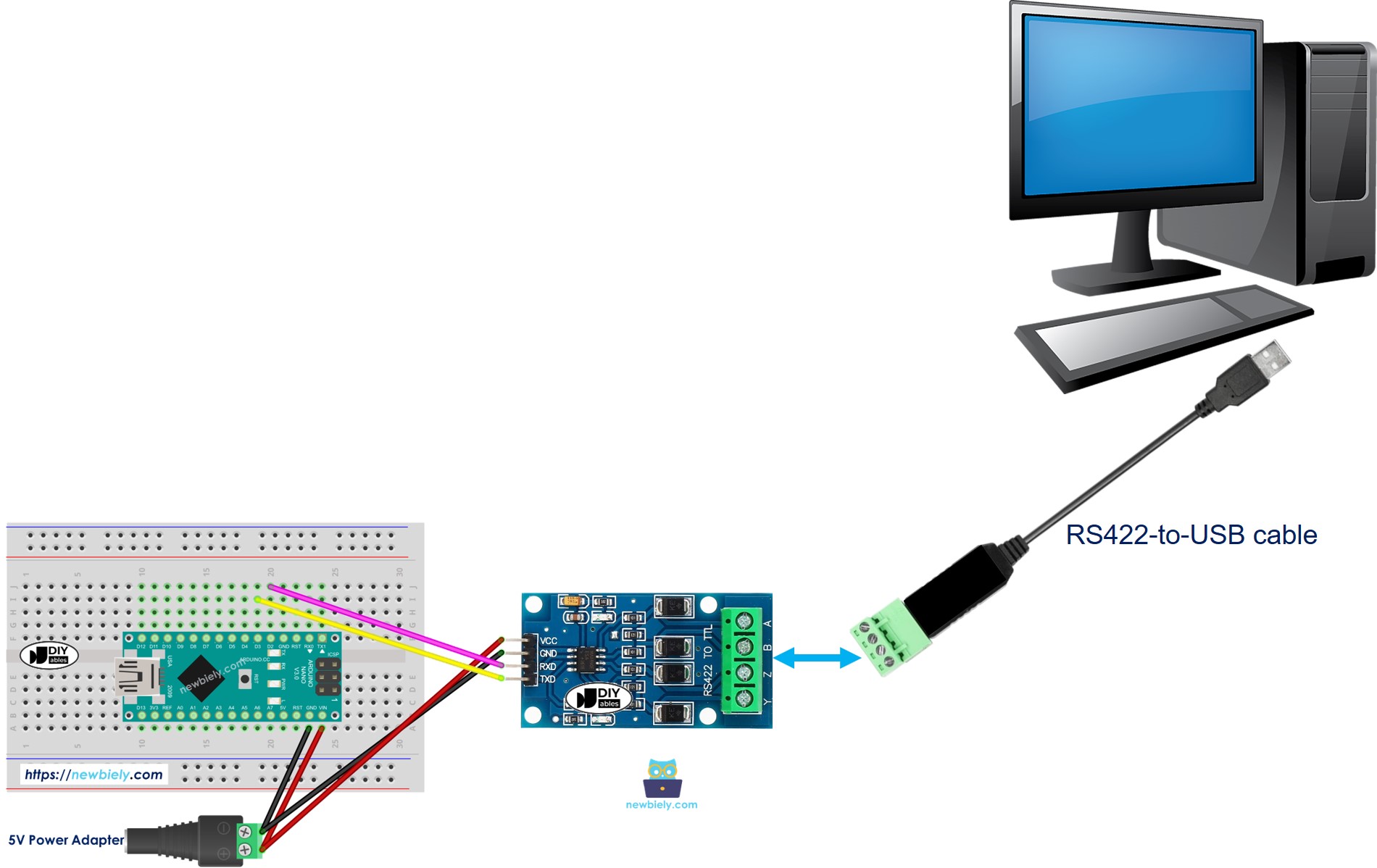
- Open the Serial Terminal Program and configure the Serial parameters (COM port, baurate...)
- Type some data from the Serial Termial to send it to Arduino Nano.
- If successful, you will see the echo data on the Serial Terminal.