Arduino Nano - Keypad
This tutorial instructs you how to connect Arduino Nano to keypad 3x4 and 4x4 and how to program for it. The tutorials also provide the method and Arduino code to verify the the password inputted from keypad by users.
Hardware Preparation
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables .
Overview of Keypad
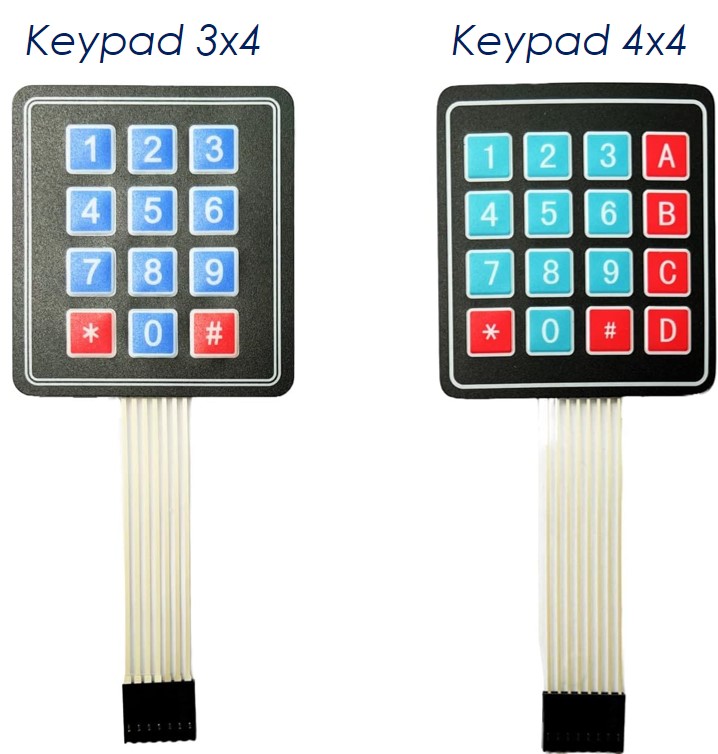
A keypad is composed of keys arranged in a matrix. Each individual button is referred to as a key. Keypads come in various styles. Two of the most commonly used for DIY projects are the 3x4 keypad (12 keys) and the 4x4 keypad (16 keys).
The Keypad Pinout
The pins are split into two categories: rows and columns.
- A 3x4 keypad has seven pins: Four of them are row-pins, labeled R1, R2, R3, and R4. The other three are column-pins, labeled C1, C2, and C3.
- A 4x4 keypad has 8 pins: Four of them are row-pins, labeled R1, R2, R3, and R4. The other four are column-pins, labeled C1, C2, C3, and C4.
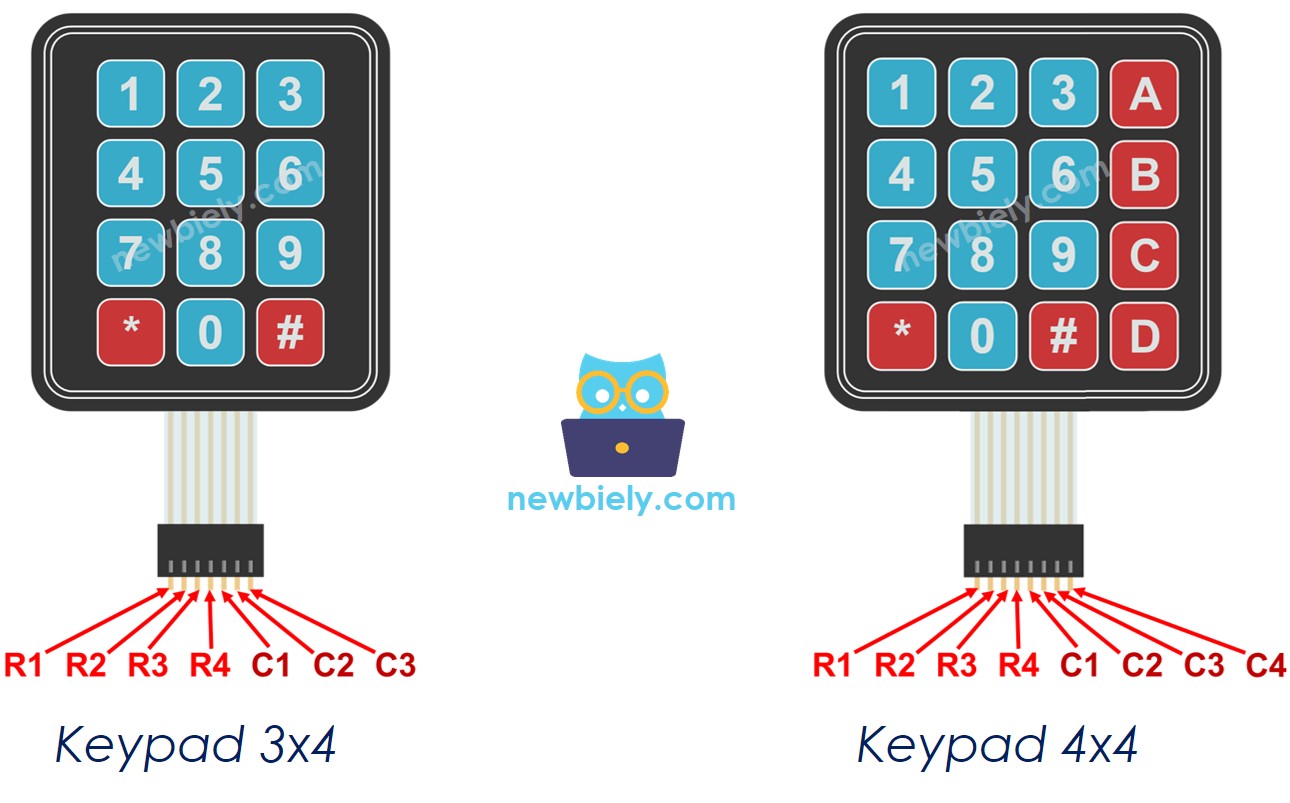
Wiring Diagram
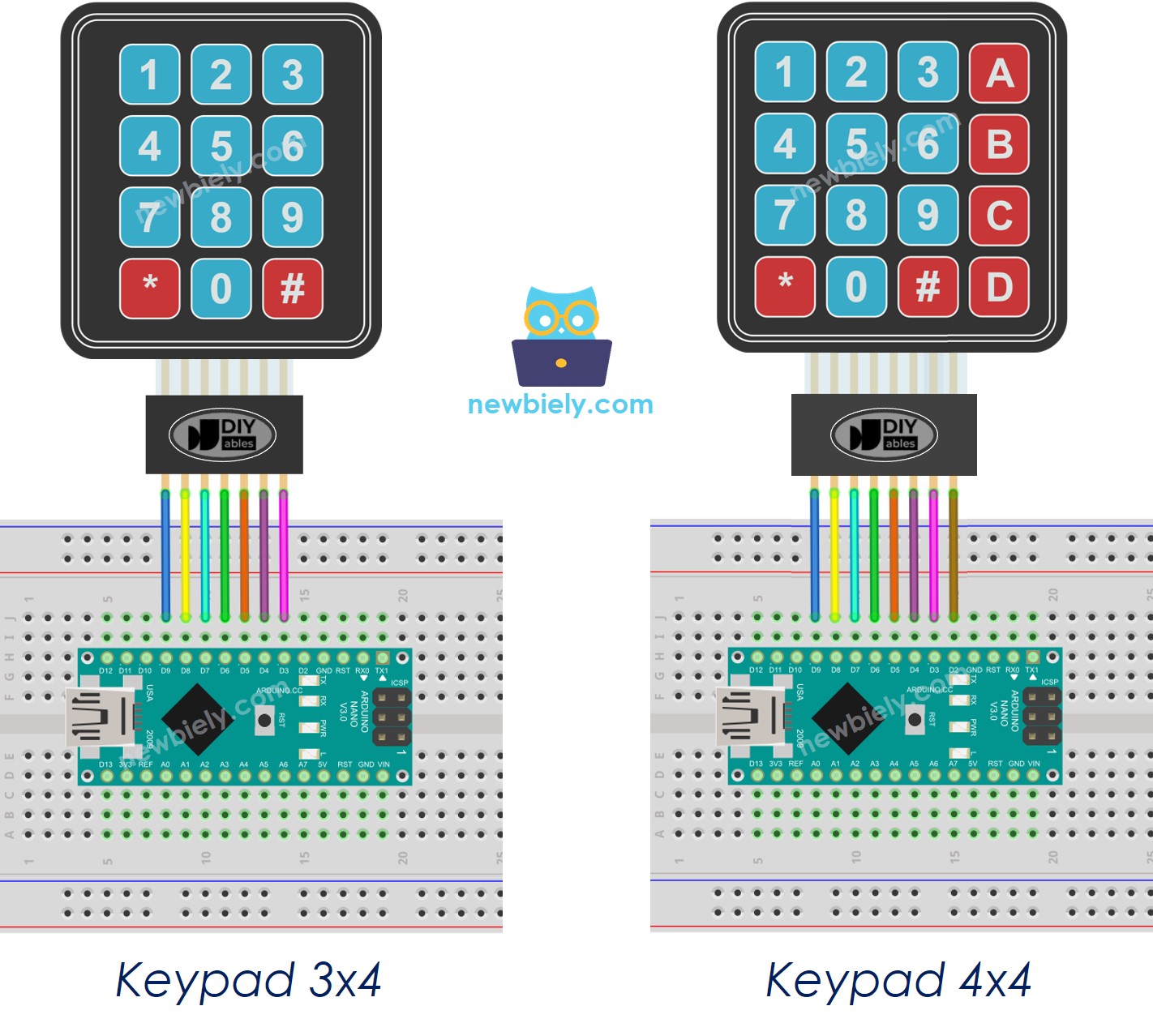
This image is created using Fritzing. Click to enlarge image
See The best way to supply power to the Arduino Nano and other components.
Arduino Nano Code
Arduino Nano Code for Keypad 3x4
Arduino Nano Code for Keypad 4x4
Detailed Instructions
- Click to the Libraries icon on the left bar of the Arduino IDE.
- Search for “keypad” and locate the keypad library created by Mark Stanley and Alexander Brevig.
- Then, press the Install button to complete the installation of the keypad library.
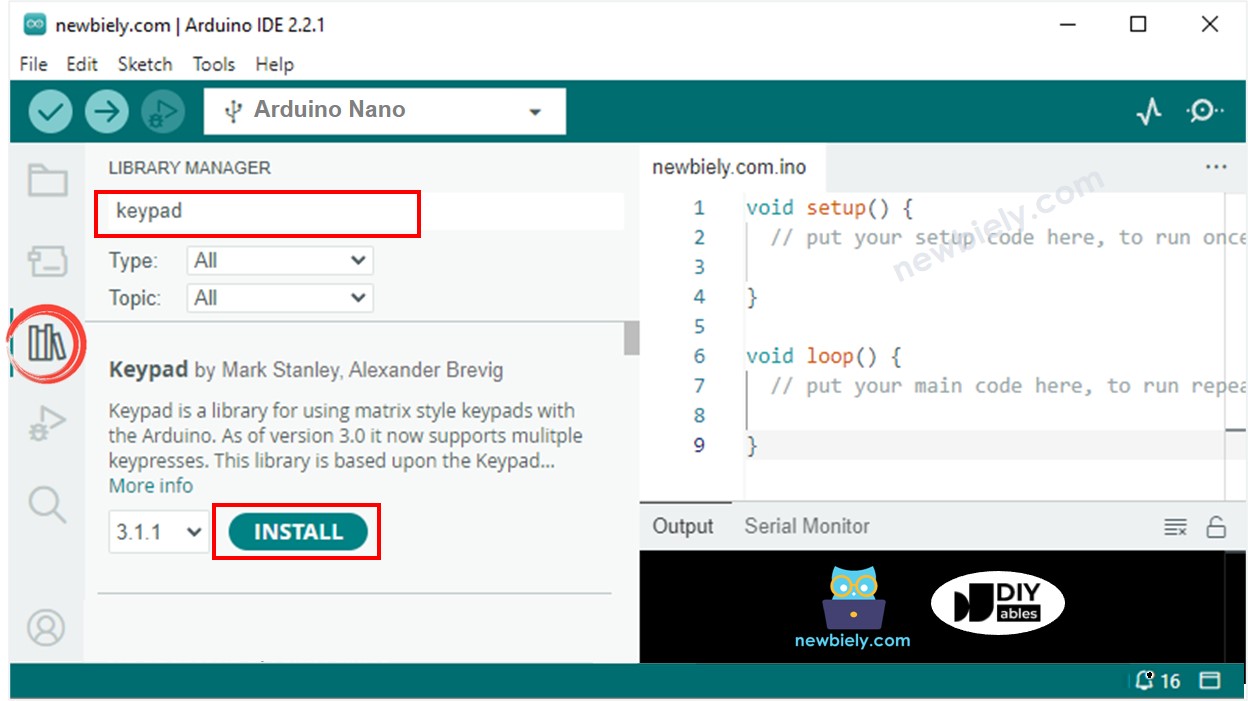
- Copy the code above and open it with the Arduino IDE.
- Click the Upload button on the Arduino IDE to compile and upload the code to the Arduino Nano.
- Open the Serial Monitor.
- Press some keys on the keypad.
- Check the result in the Serial Monitor.
Keypad and Password
A common use of the keypad is for password entry. Two special keys are used for this purpose:
- A key to initiate or restart the password input, such as the "*" key
- A key to end the password input, such as the "#" key
The password will be a string comprised of all the keys, except for two specific special keys.
When a key is pressed:
- If the key is not "*" or "#", add the key to the user's input password string.
- If the key is "#", compare the user's input string with the authorized passwords to determine if the input password is correct, then clear the user's input password string.
- If the key is "*", erase the user's input password string.
Keypad - Password Code
Detailed Instructions
- Run the code above.
- Open the Serial Monitor.
- Press the "123456" keys followed by the "#" key.
- Press the "1234" keys followed by the "#" key.
- Check out the result on the Serial Monitor.