Arduino Nano - IR Remote Control
You've probably come across the infrared remote control, also called the IR remote control, when using devices like TVs and air conditioners at home... This tutorial instructs you how to use the infrared (IR) remote control and an infrared receiver to control the Arduino Nano. In detail, we will learn:
- How to connect an IR receiver to Arduino Nano board
- How to program Arduino Nano to read the command from IR remote controller via IR receiver
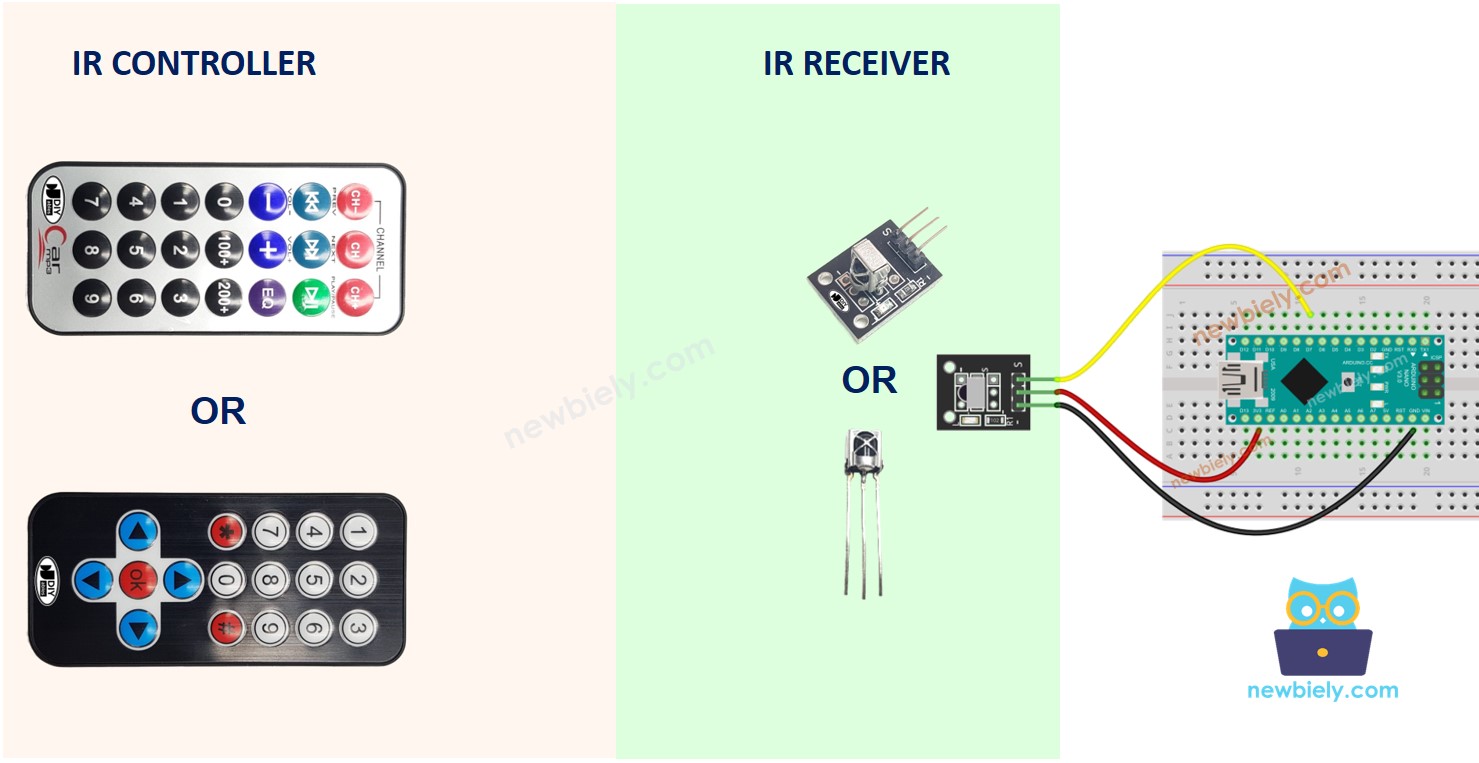
Hardware Preparation
Or you can buy the following kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables .
Overview of IR Remote Control
An IR control system has two components: an IR remote controller and an IR receiver.
- The IR remote controller is used to send commands using infrared signals.
- The IR receiver captures and interprets these signals to control the device.
An IR kit typically consists of the two components mentioned above: an IR remote controller and an IR receiver.
IR remote controller
The IR remote controller is a portable device that emits infrared signals. It is equipped with a keypad containing several buttons:
- Each button on the remote controller is assigned a specific function or command.
- When a button is pressed, the remote controller emits an invisible infrared signal. This signal carries a distinctive code or pattern linked to the pressed button.
- These infrared signals belong to the infrared spectrum and cannot be seen by the human eye.
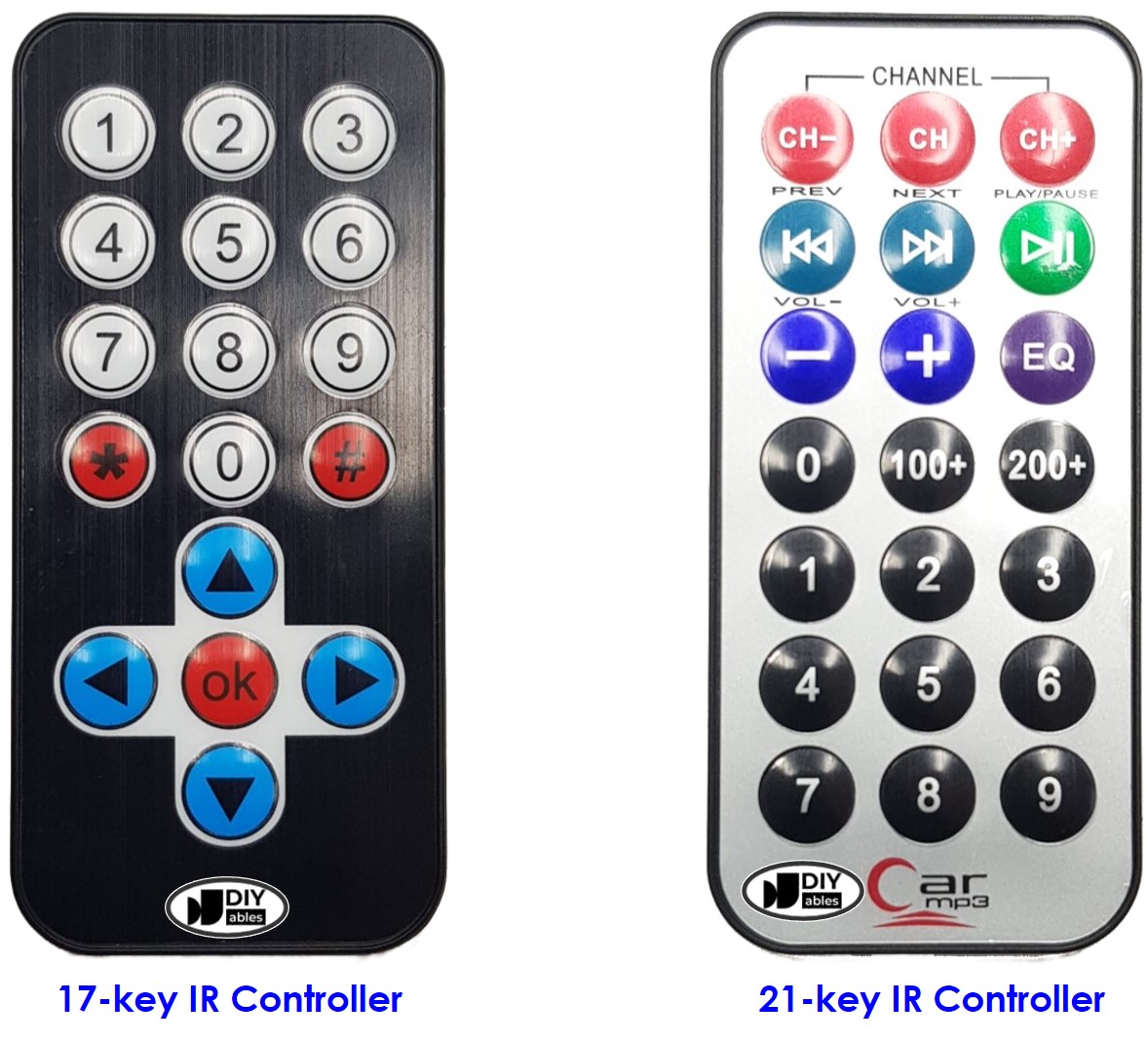
IR Receiver
The IR receiver module is a sensor that detects and receives the infrared signals emitted by the remote controller.
The infrared receiver detects the incoming infrared signals and converts them into the code (command) representing the button pressed on the remote controller.
The IR Receiver can be a sensor or a module. You can use the following choices:
- IR Receiver Module only
- IR Receiver Sensor only
- IR Receiver Sensor + Adapter
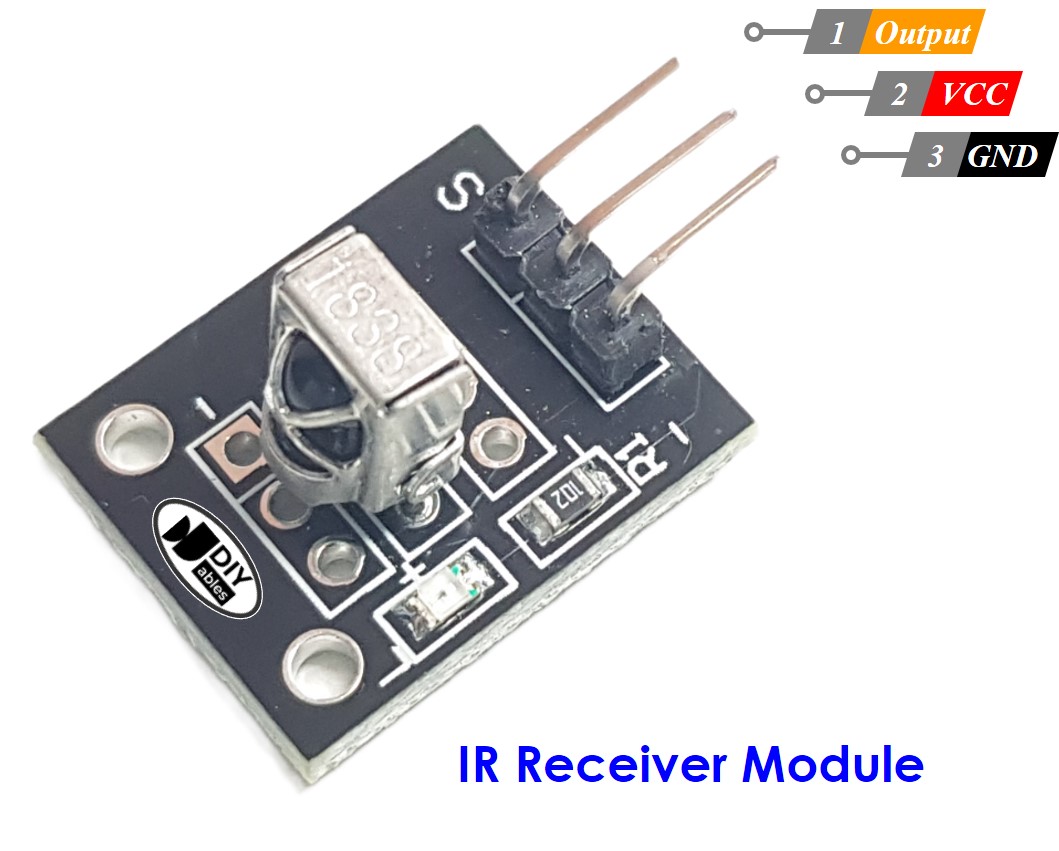
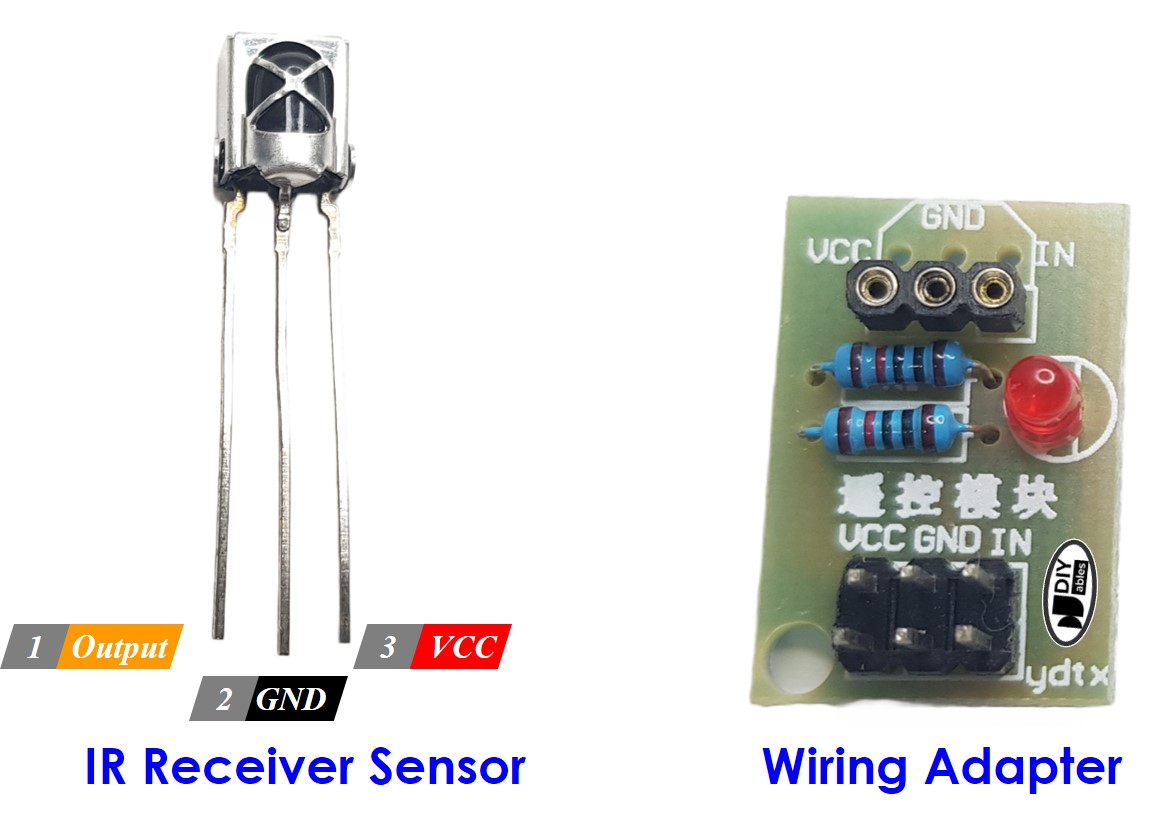
IR Receiver Pinout
IR receiver module or sensor has three pins:
- VCC pin: Connect this pin to the 3.3V or 5V pin of the Arduino Nano or external power source.
- GND pin: Connect this pin to GND pin of the Arduino Nano or external power source..
- OUT (Output) pin: This pin is the output pin of the IR receiver module. Connected to a digital input pin on the Arduino Nano.
How It Works
When a user presses a button on the IR remote controller, the following steps occur:
- The IR remote controller encodes the command associated with the button into an infrared signal using a specific protocol.
- The IR remote controller emits the encoded infrared signal.
- The IR receiver picks up the encoded infrared signal.
- The IR receiver decodes the encoded infrared signal, converting it back into the original command.
- The Arduino Nano reads the command received from the IR receiver.
- The Arduino Nano maps the received command to the corresponding key that was pressed on the IR remote controller.
These steps explain the process of how the IR remote controller's button press is translated into a recognizable command by the Arduino Nano.
It may seem complicated, but there's no need to worry. Thanks to the DIYables_IRcontroller library, it becomes incredibly easy.
Wiring Diagram
Wiring diagram between Arduino Nano and IR Receiver Module
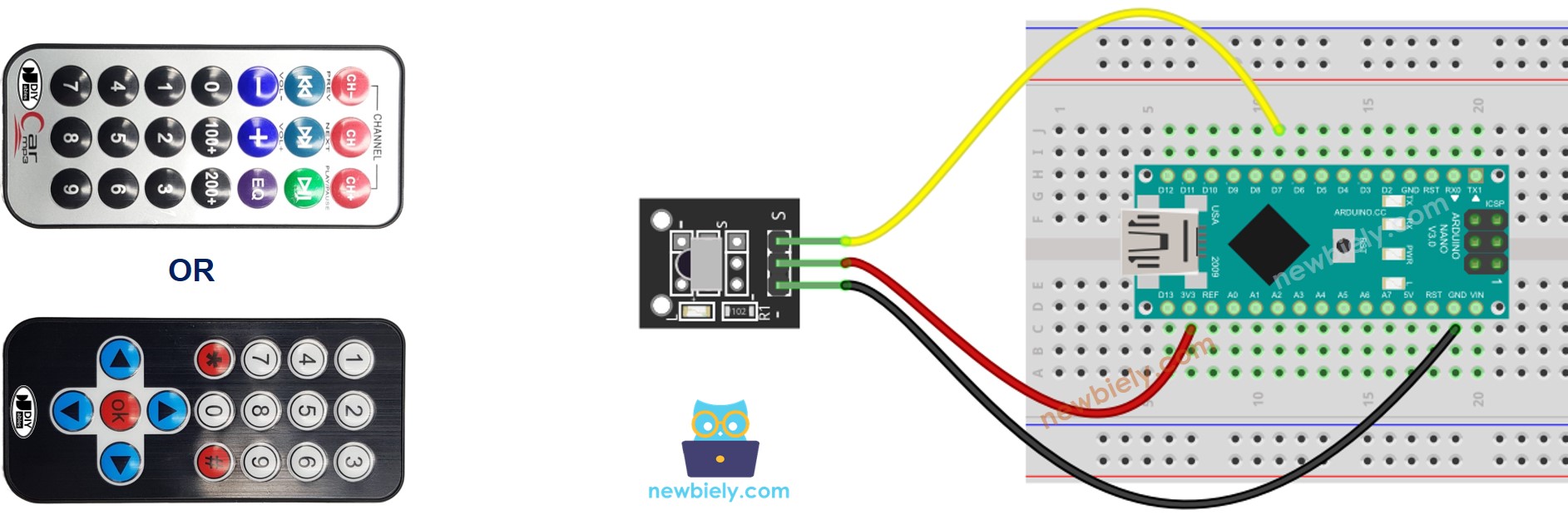
This image is created using Fritzing. Click to enlarge image
Wiring diagram between Arduino Nano and IR Receiver Sensor
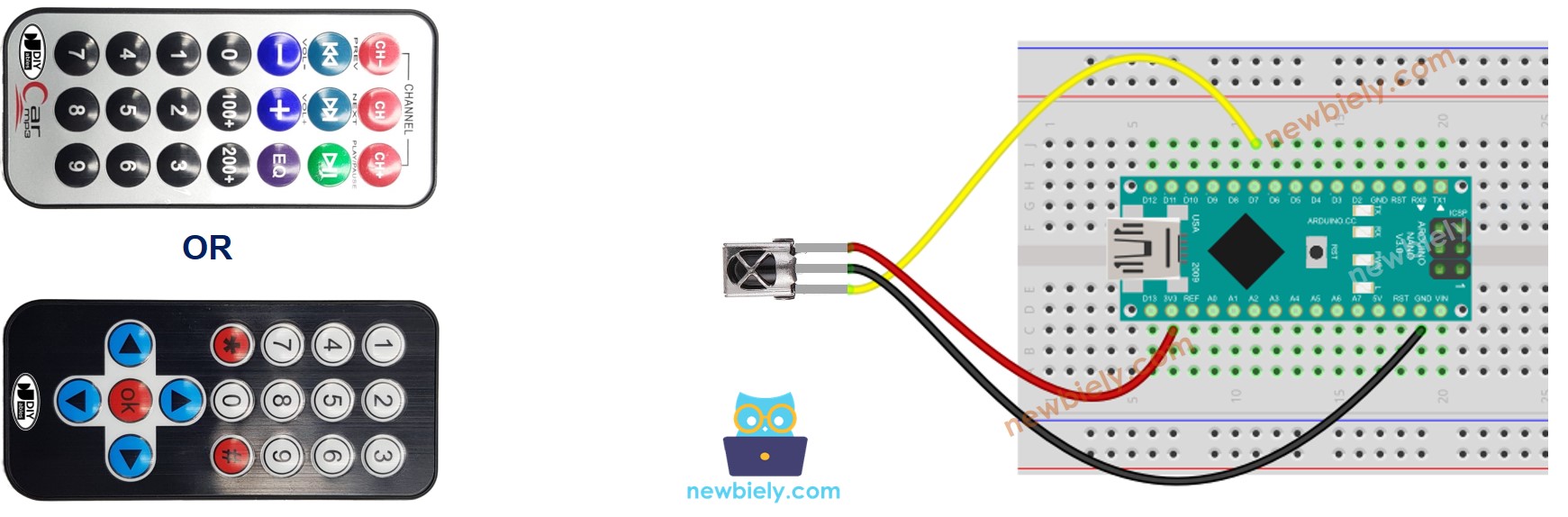
This image is created using Fritzing. Click to enlarge image
See The best way to supply power to the Arduino Nano and other components.
Wiring diagram between Arduino Nano and IR Receiver Sensor and Adapter
Before connecting the IR receiver sensor to the Arduino Nano, you have the option to connect it to the adapter. This allows for easier setup and ensures proper connection between the IR receiver sensor and the Arduino Nano.
You can also connect The IR receiver sensor to the adapter before connecting to the .
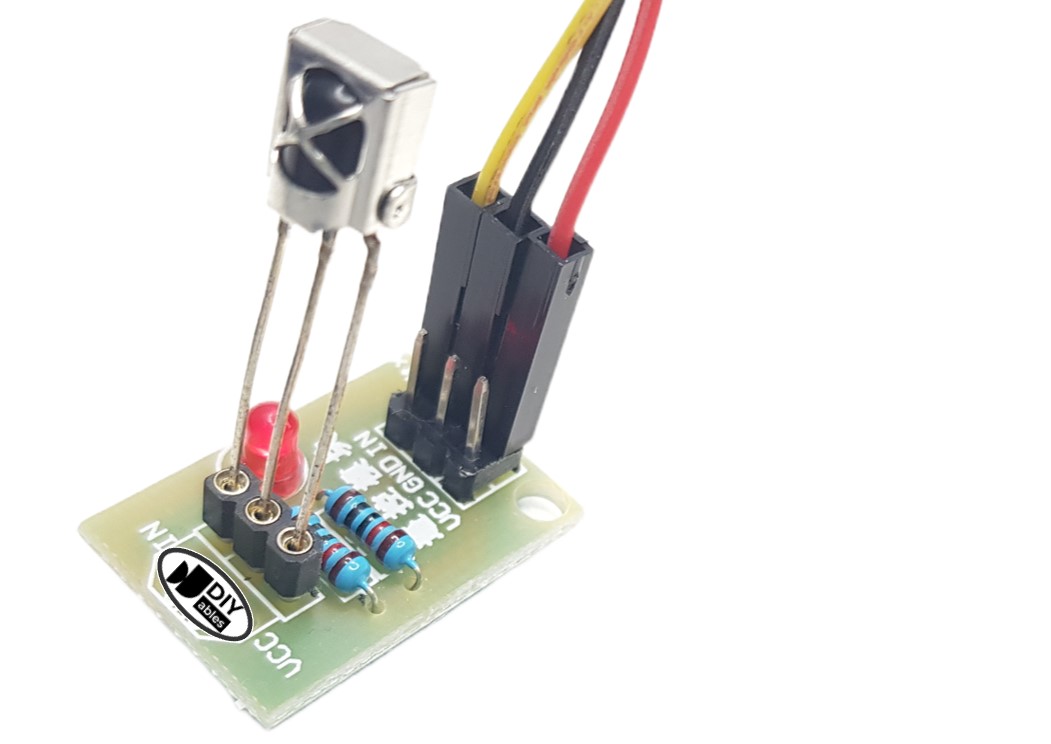
How To Program For IR Remote Controller
- Include the library:
- Declare a DIYables_IRcontroller_17 or DIYables_IRcontroller_21 object corresponds with 17-key or 21-key IR remote controllers:
- Initialize the IR Controller.
- In the loop, check if a key is pressed or not. If yes, get the key
- Upon detecting a key press, you can perform specific actions corresponding to each key.
Arduino Nano Code
- Arduino Nano code for DIYables 17-key IR remote controller
- Arduino Nano code for DIYables 21-key IR remote controller
Detailed Instructions
- Click to the Libraries icon on the left bar of the Arduino IDE.
- Search "DIYables_IRcontroller", then find the DIYables_IRcontroller library by DIYables
- Click Install button to install DIYables_IRcontroller library.
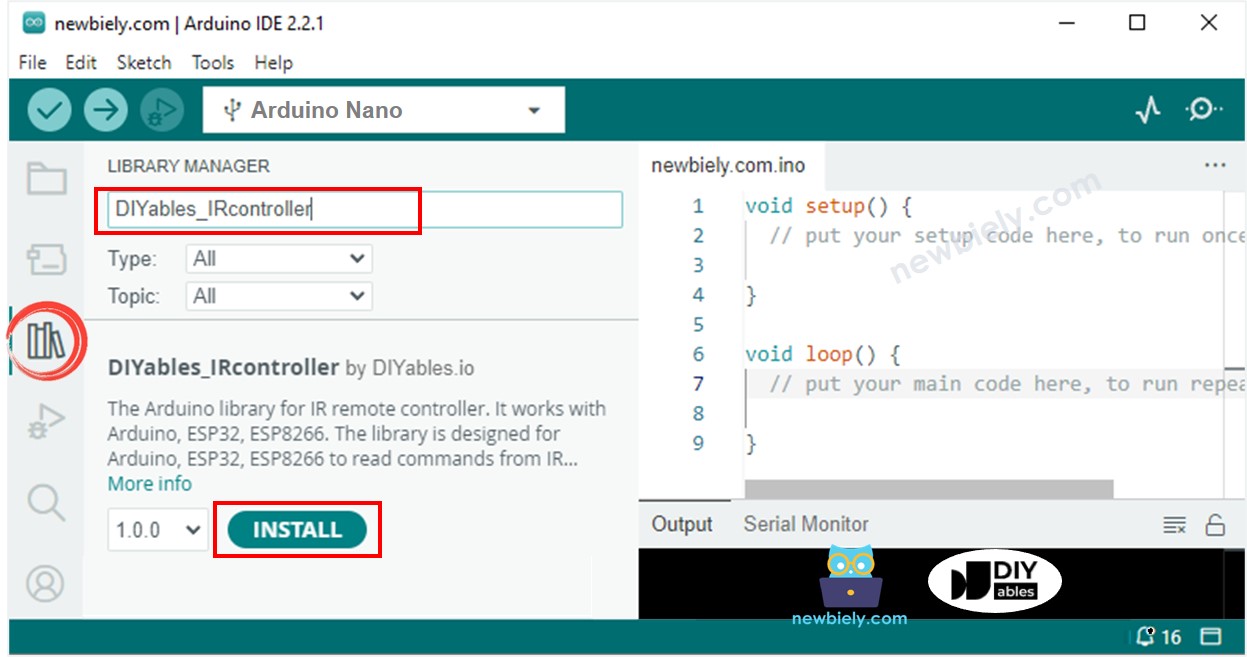
- You will be asked for installing the library dependency as below image:
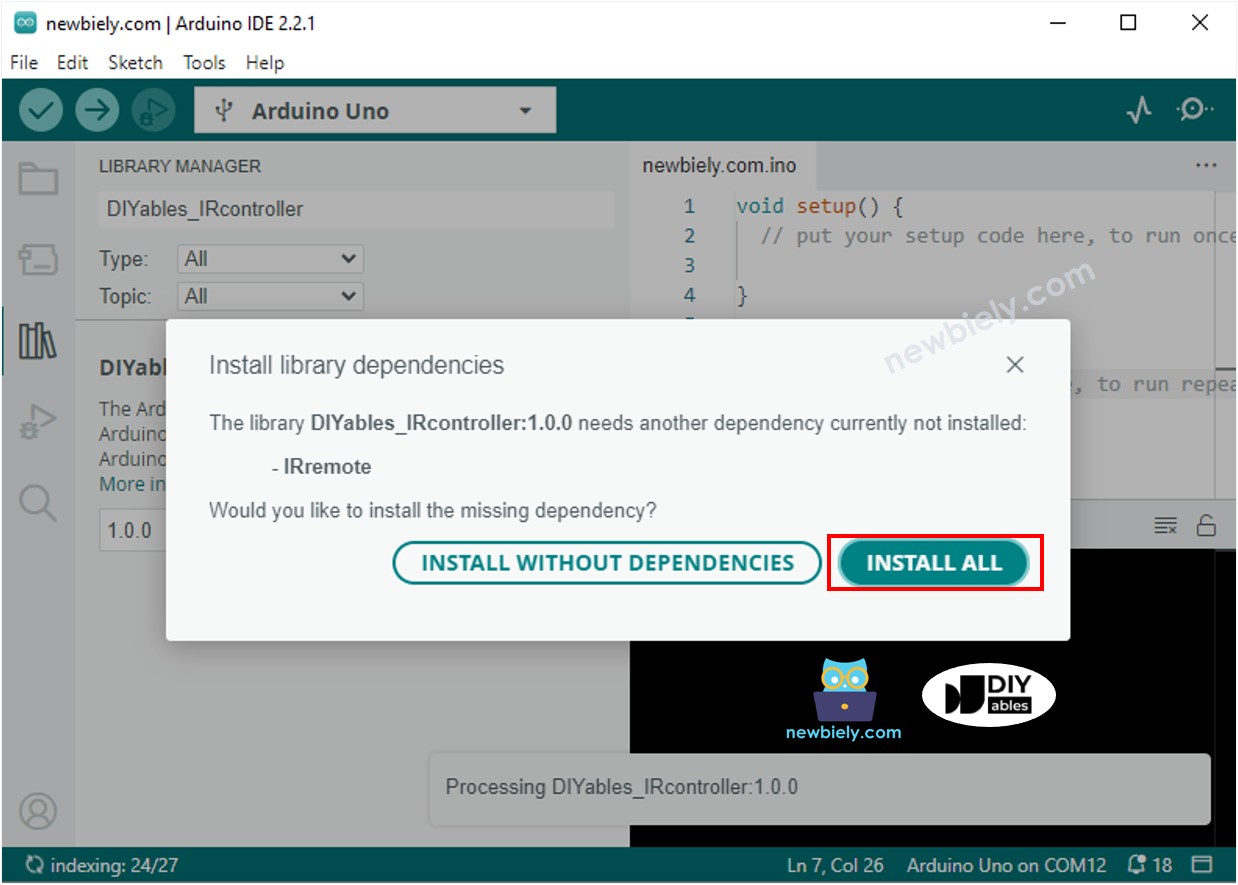
- Click Install all button to install the dependency
- Copy the above code and open with Arduino IDE
- Click Upload button on Arduino IDE to upload code to Arduino Nano
- Press keys on the remote controller one by one
- Check out the result on the Serial Monitor.
- Here's the result you can expect when you press the keys on a 17-key IR controller, one by one::
You can now make changes to the code in order to control devices like LEDs, fans, pumps, actuators, and more using IR remote controllers.