Arduino Nano - Temperature Sensor
This tutorial instructs you how to use Arduino Nano and the waterproof DS18B20 1-wire temperature sensor to measure the temperature. This sensor is cost-effective, simple to use, and has a neat appearance among other alternatives.
Hardware Preparation
Or you can buy the following kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables .
Buy Note: Many DS18B20 sensors available in the market are unreliable. We strongly recommend buying the sensor from the DIYables brand using the link provided above. We tested it, and it worked reliably.
Overview of 1-Wire DS18B20 Temperature Sensor
The Temperature Sensor Pinout
The DS18B20 temperature sensor has three pins that need to be connected:
- GND pin: needs to be linked to GND (0V)
- VCC pin: needs to be linked to VCC (5V or 3.3V)
- DATA pin: is the 1-wire data bus and should be connected to a digital pin on Arduino Nano.
The sensor typically comes in two varieties: TO-92 package (which resembles a transistor) and a waterproof probe. We will be utilizing the waterproof probe in this tutorial.
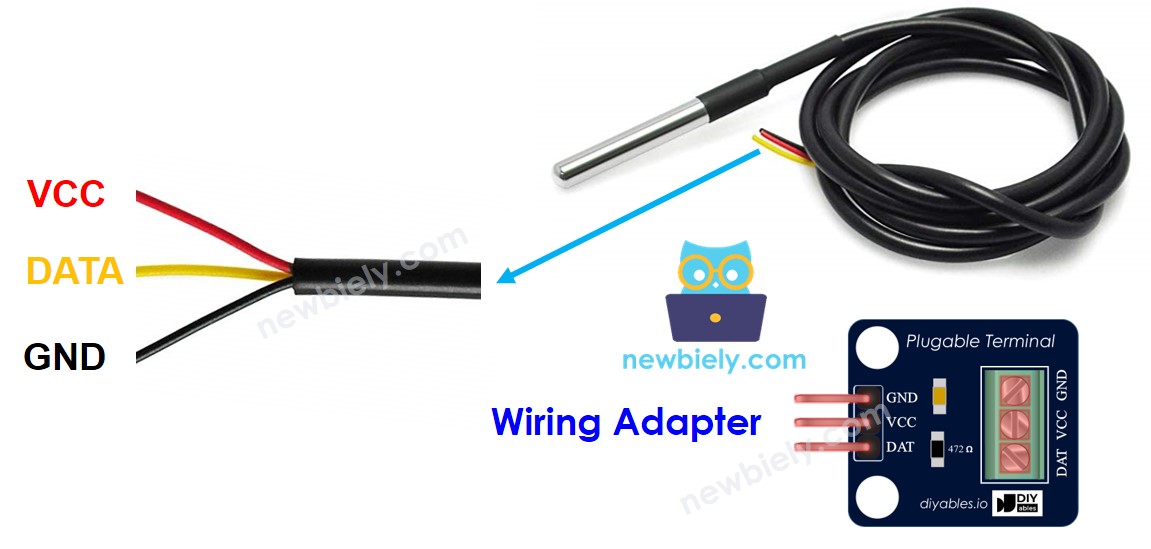
Connecting the DS18B20 temperature sensor with Arduino requires a pull-up resistor, making it a bit of a hassle. However, some manufacturers make it easier by offering a wiring adapter that comes equipped with a built-in pull-up resistor and a screw terminal block, reducing the hassle and making it more convenient.
Wiring Diagram
- Wiring diagram using a breadboard.
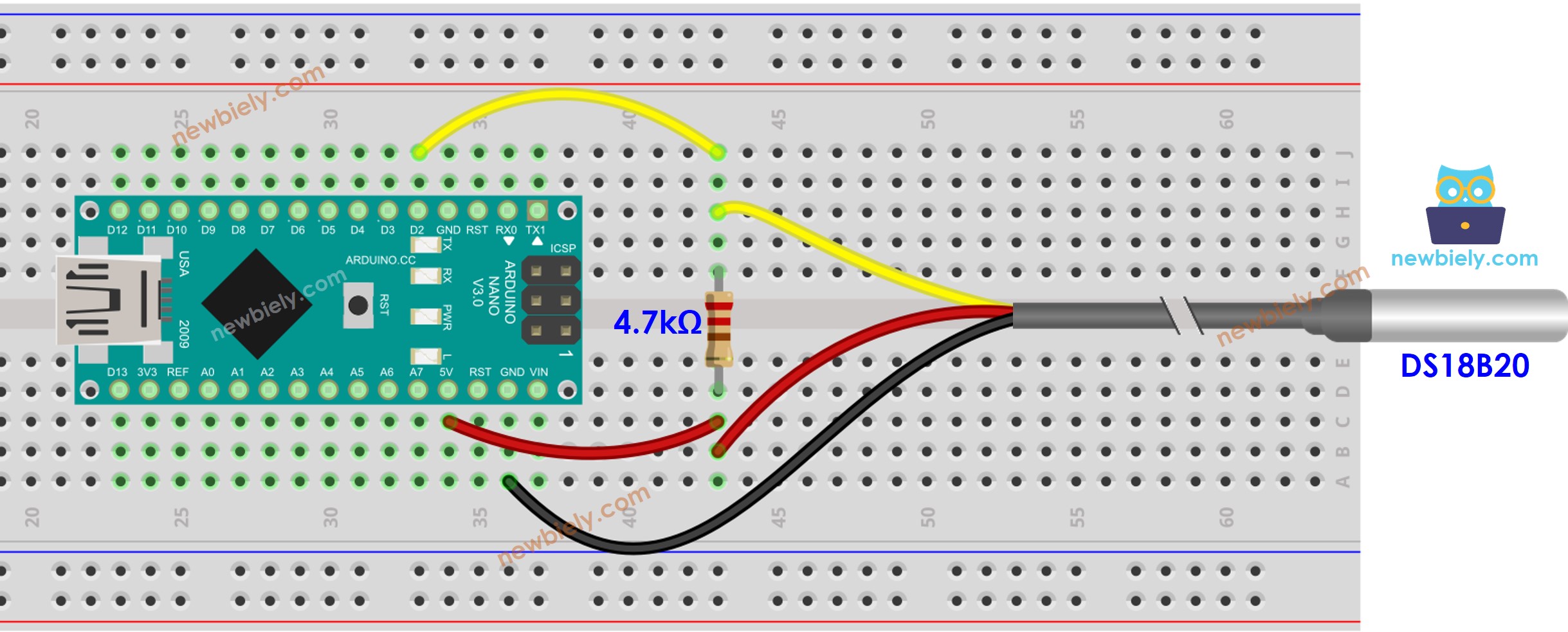
This image is created using Fritzing. Click to enlarge image
- Wiring diagram using a the wiring adapter (recommended).
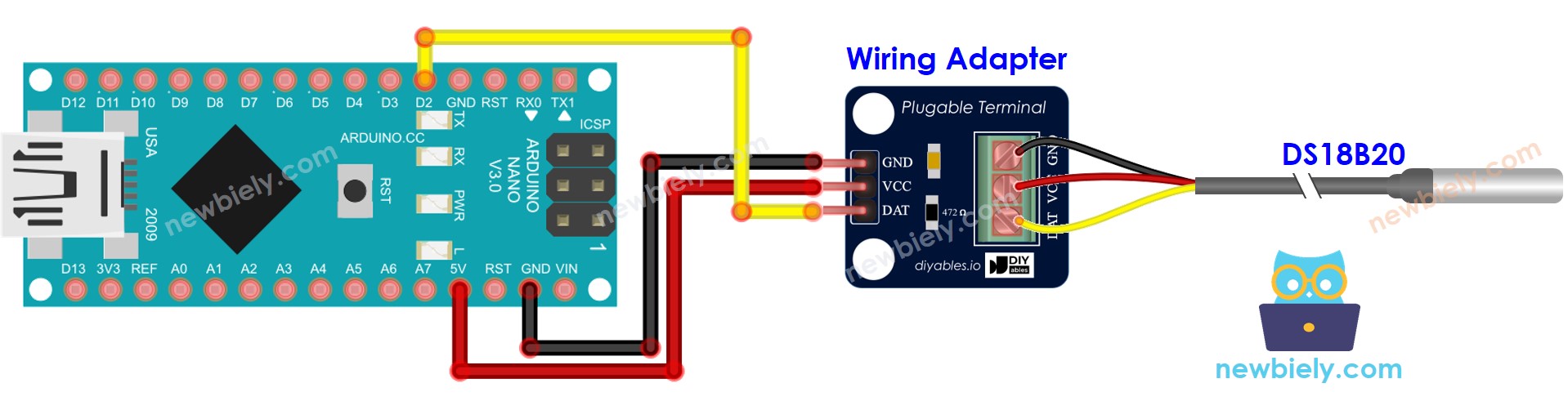
This image is created using Fritzing. Click to enlarge image
See The best way to supply power to the Arduino Nano and other components.
We recommend buying a DS18B20 sensor along with its accompanying wiring adapter for a seamless setup. This adapter includes an integrated resistor, removing the need for an additional resistor in the wiring. We also tested it and worked well.
How To Program For DS18B20 Temperature Sensor
- Include the following library:
- Declare an object for OneWire, corresponding to the pin connected to the sensor's DATA pin. Also declare an object for DallasTemperature.
- Initialize the sensor.
- Issue the command to obtain temperatures.
- Check the temperature in Celsius.
- Calculate Fahrenheit from Celsius:
Arduino Nano Code
Detailed Instructions
- Connect an USB cable to the Arduino Nano and the PC.
- Open the Arduino IDE and select the appropriate board and port.
- Click to the Libraries icon on the left bar of the Arduino IDE.
- Search for “Dallas”, then locate the DallasTemperature library created by Miles Burton.
- Press the Install button to install the DallasTemperature library.
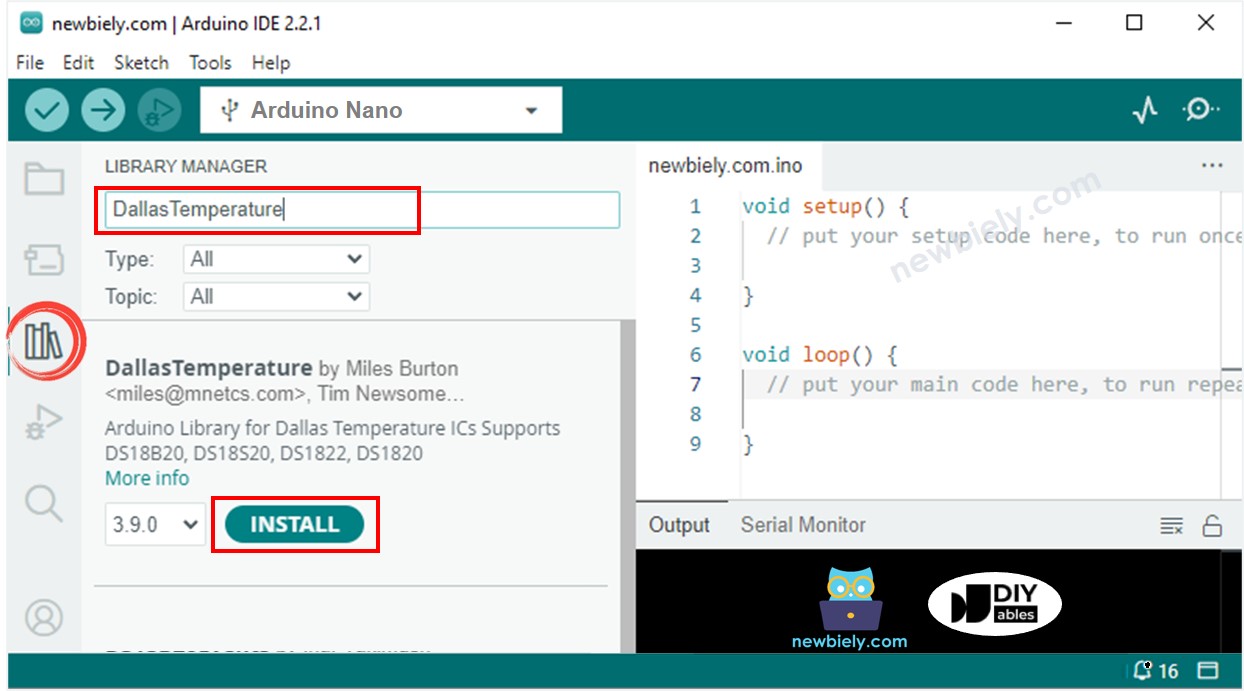
- You will be asked to install the dependency. Click Install All button to install OneWire library.
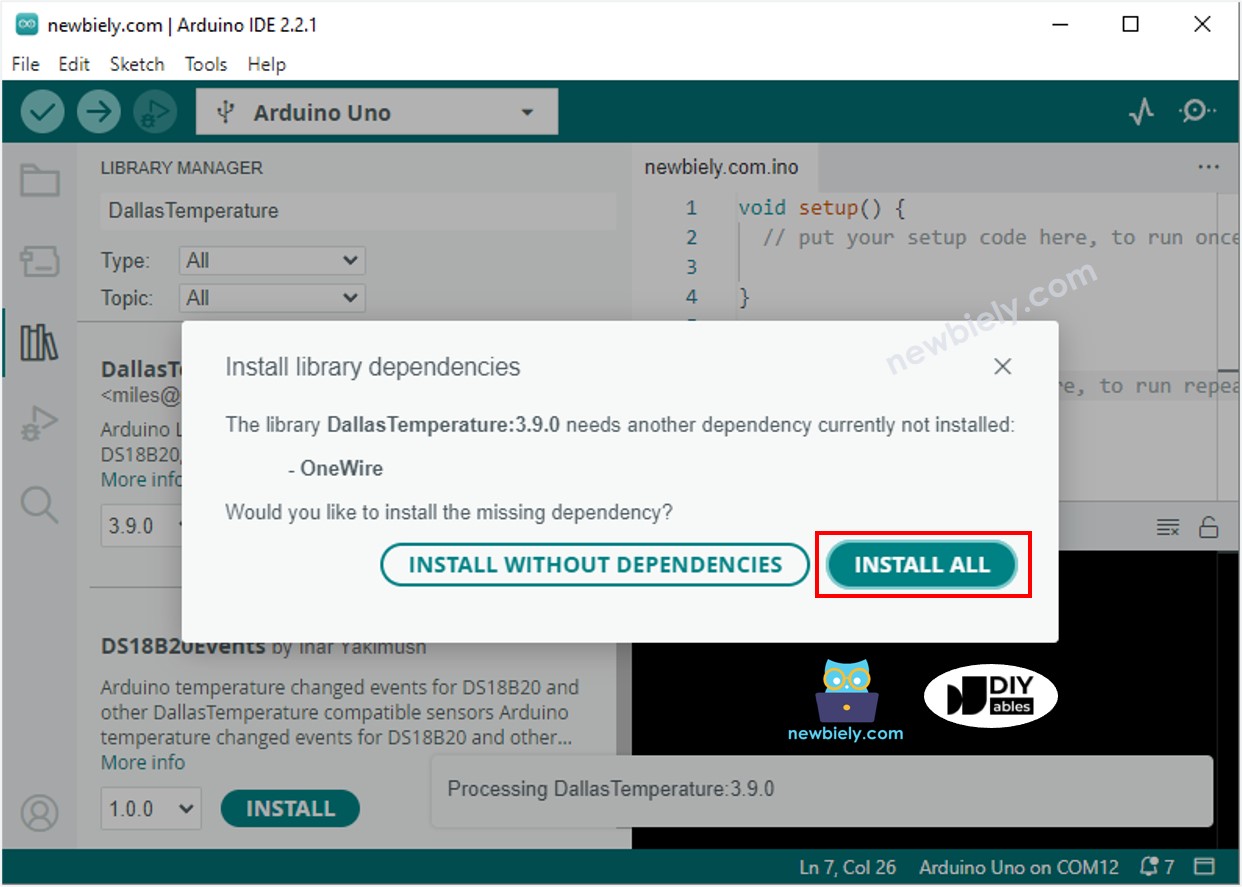
- Copy the code and open it in Arduino IDE.
- Click the Upload button on Arduino IDE to send the code to Arduino Nano.
- Place the sensor in hot and cold water, or hold it in your hand.
- Check out the result on the Serial Monitor.