Arduino Nano - LED Matrix
This tutorial instructs you how to use Arduino Nano with LED matrix display. In detail, we will learn:
- About LED matrix display
- How to connect Arduino Nano to 8x8 LED matrix
- How to connect Arduino Nano to 32x8 LED matrix
- How to program Arduino Nano to display text, numbers, and animated effects on the LED matrix.
Once that is done, you can quickly adjust the code to suit other LED matrices such as 16x8 LED matrix, 64x8 LED matrix ...
Hardware Preparation
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables .
Overview of LED Matrix
LED matrix displays are commonly referred to as LED displays or dot matrix displays.
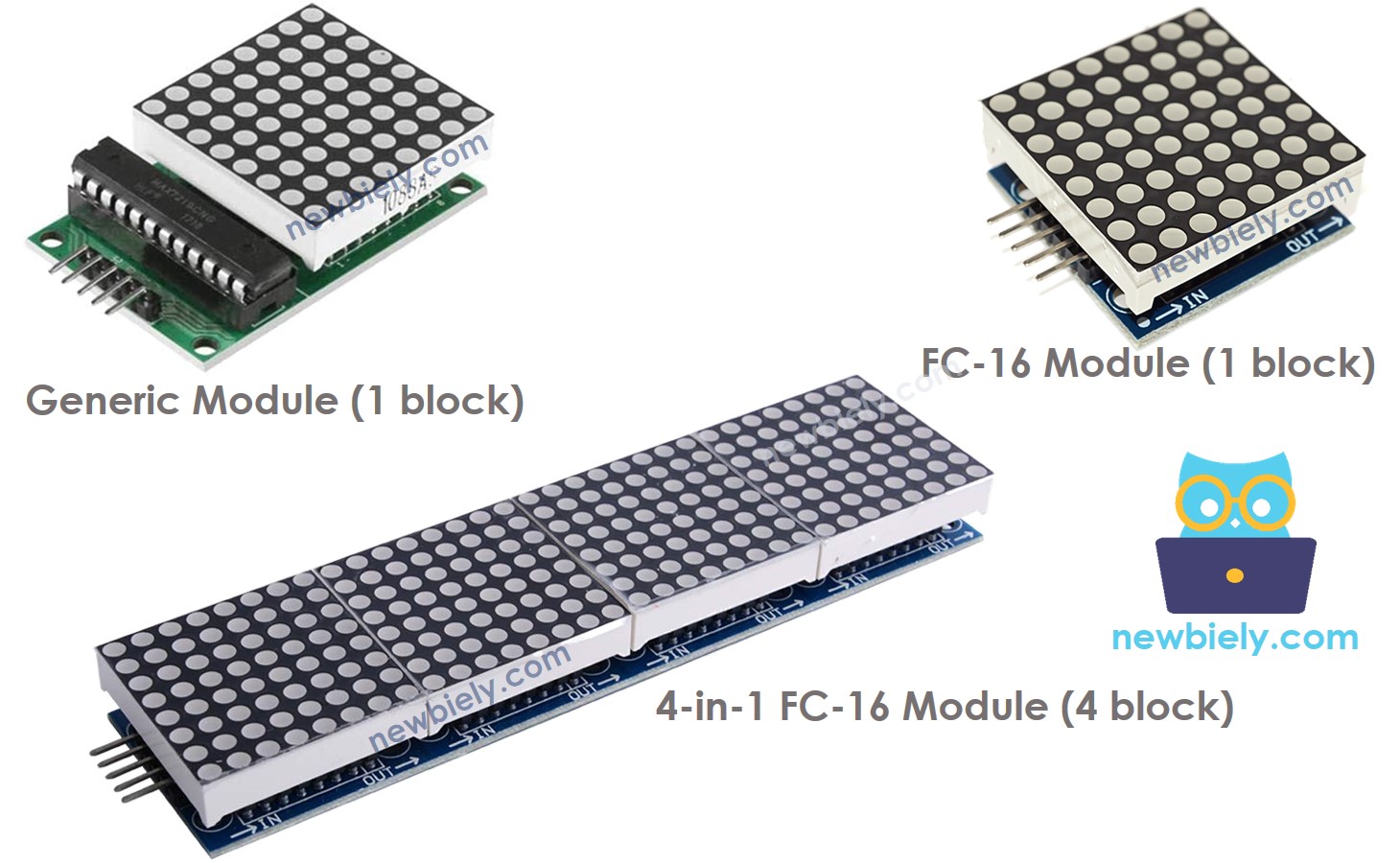
LED Matrix come in many varieties. The MAX7219-based LED matrix is a popular choice for use with Arduino Nano. It has the following features:
- Each block consists of an 8x8 LED matrix (64 LED) and a MAX7219 driver.
- Two common block forms are the generic module and the FC-16 module.
- A LED matrix can be made up of one or multiple blocks connected in a daisy-chain.
- You can purchase pre-assembled LED matrices with multiple blocks (e.g. 4-in-1, 8-in-1).
- Alternatively, you can buy individual blocks and wire them together to create a LED matrix of the desired size.
- The size of the LED matrix used must be declared in the Arduino Nano code.
The LED Matrix Pinout
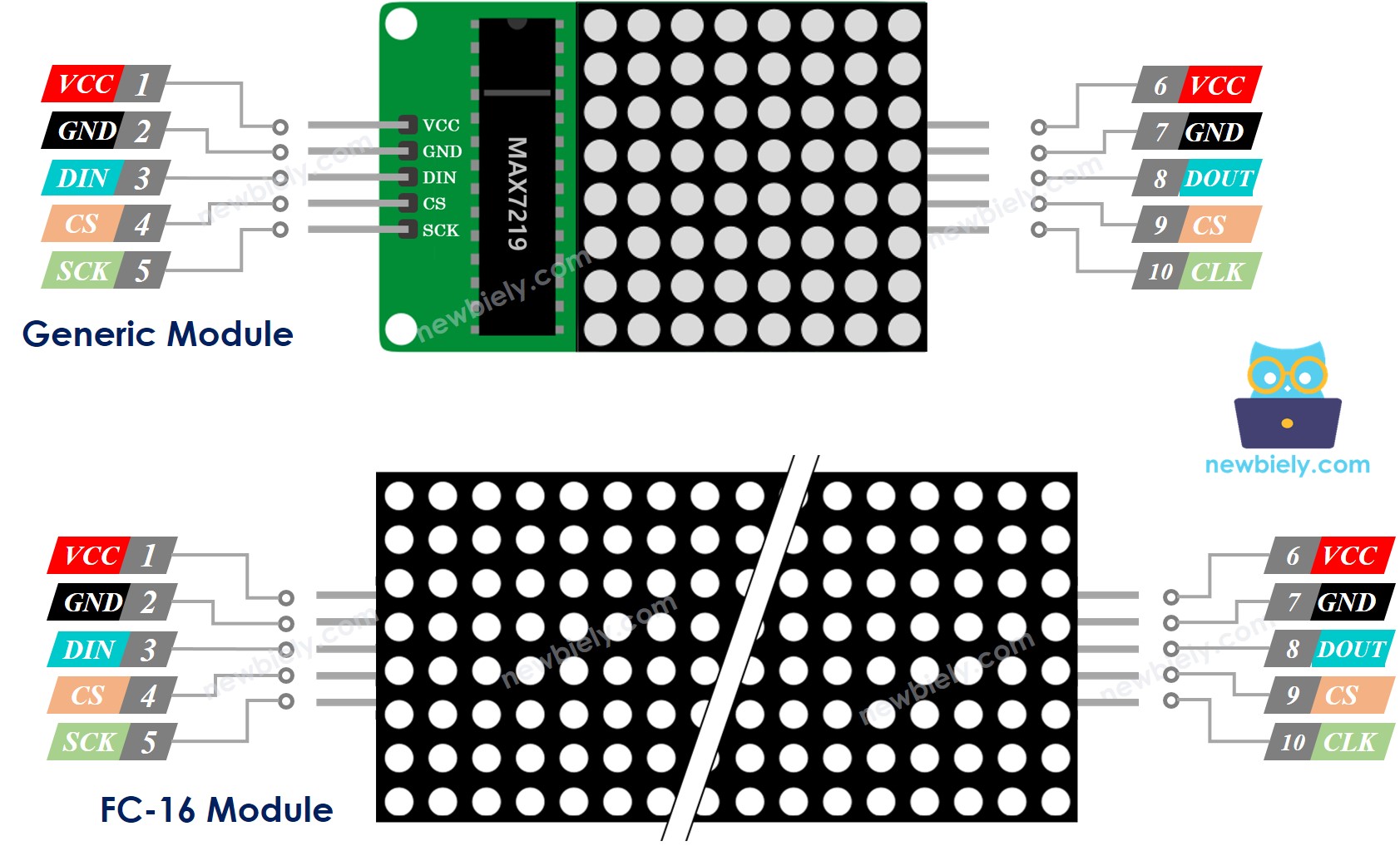
A LED Matrix is composed of one or more blocks. Each block has two sets of pins:
- Input pins group:
- VCC: connected to 5V power supply.
- GND: connected to ground.
- DIN: Data pin, connected to SPI MOSI pin of the Arduino Nano.
- CS: Chip Select, connected to any digital pin of the Arduino Nano.
- CLK: Clock pin, connected to SPI MOSI pin of the Arduino Nano.
- Output pins group:
- VCC: connected to VCC on the next block.
- GND: connected to GND on the next module.
- DOUT: Data Out, connected to the DIN pin of the next block.
- CS: connected to CS on the next block.
- CLK connected to CLK on the next block.
Wiring Diagram
If the LED matrix is made of a single block:
- Attach the input pins groups to Arduino Nano
- Leave the output pins group unconnected
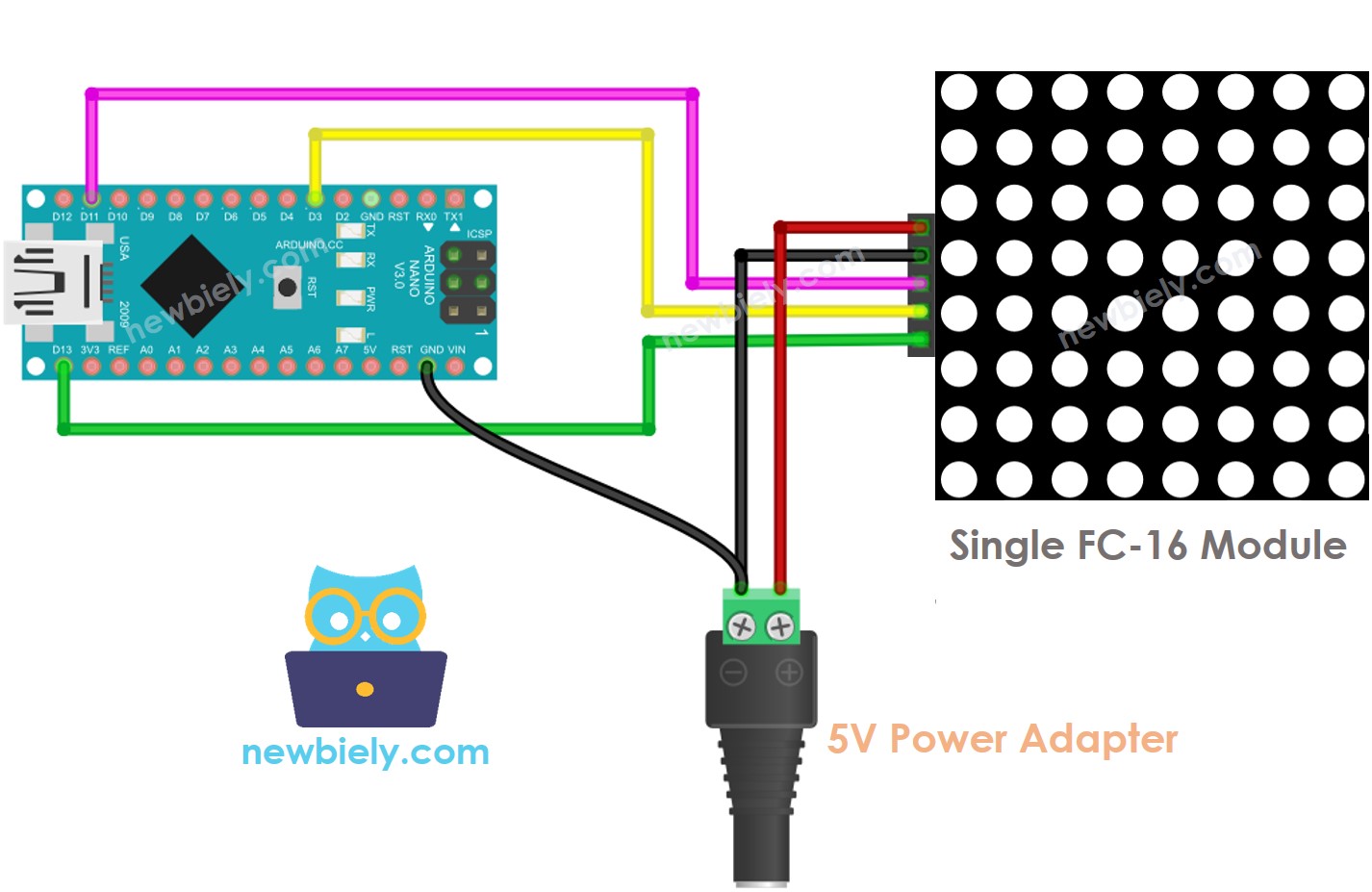
This image is created using Fritzing. Click to enlarge image
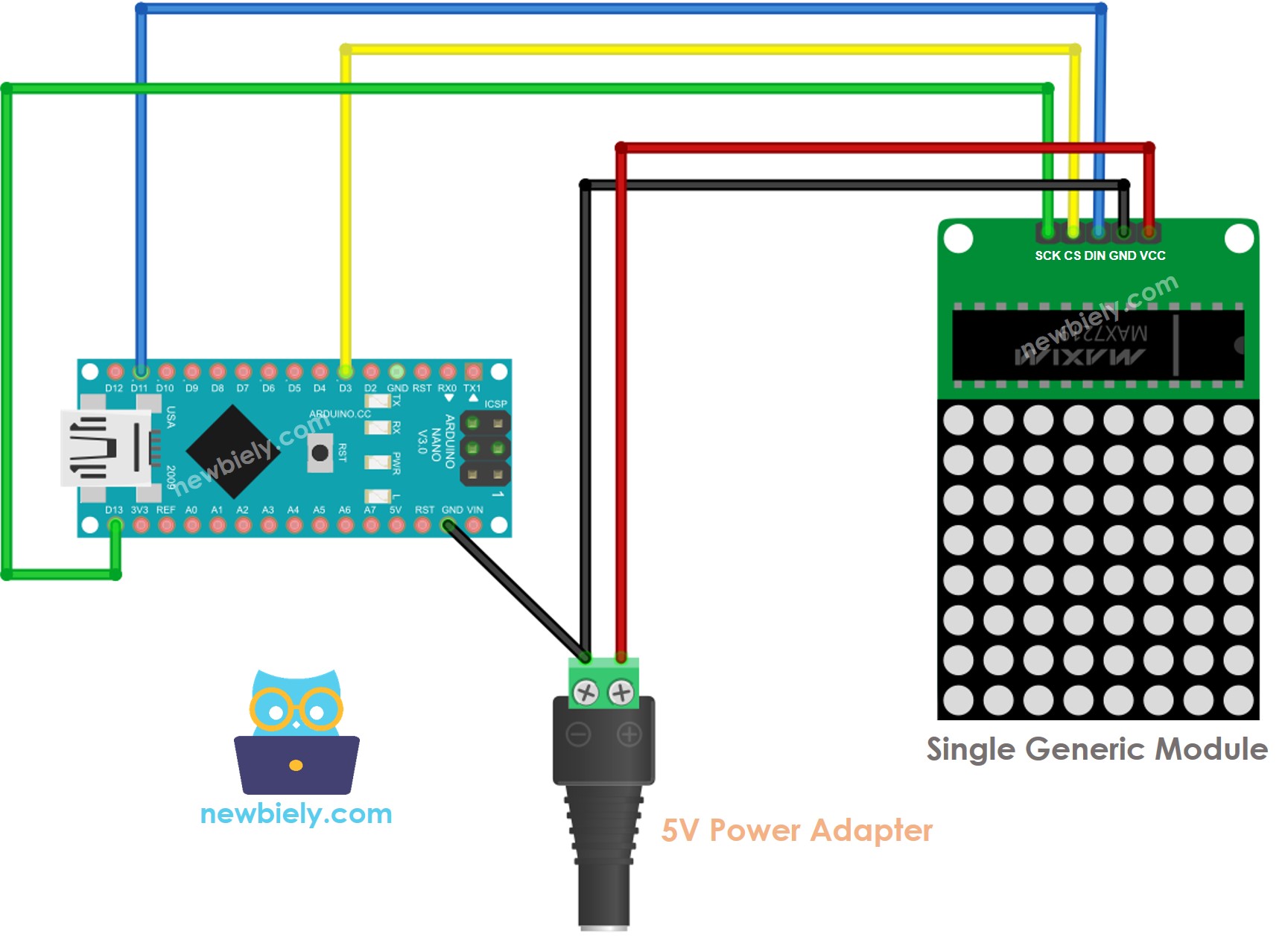
This image is created using Fritzing. Click to enlarge image
If the LED matrix is pre-built of multiple blocks:
- Connect the input pins group to Arduino Nano
- Leave the output pins group unconnected
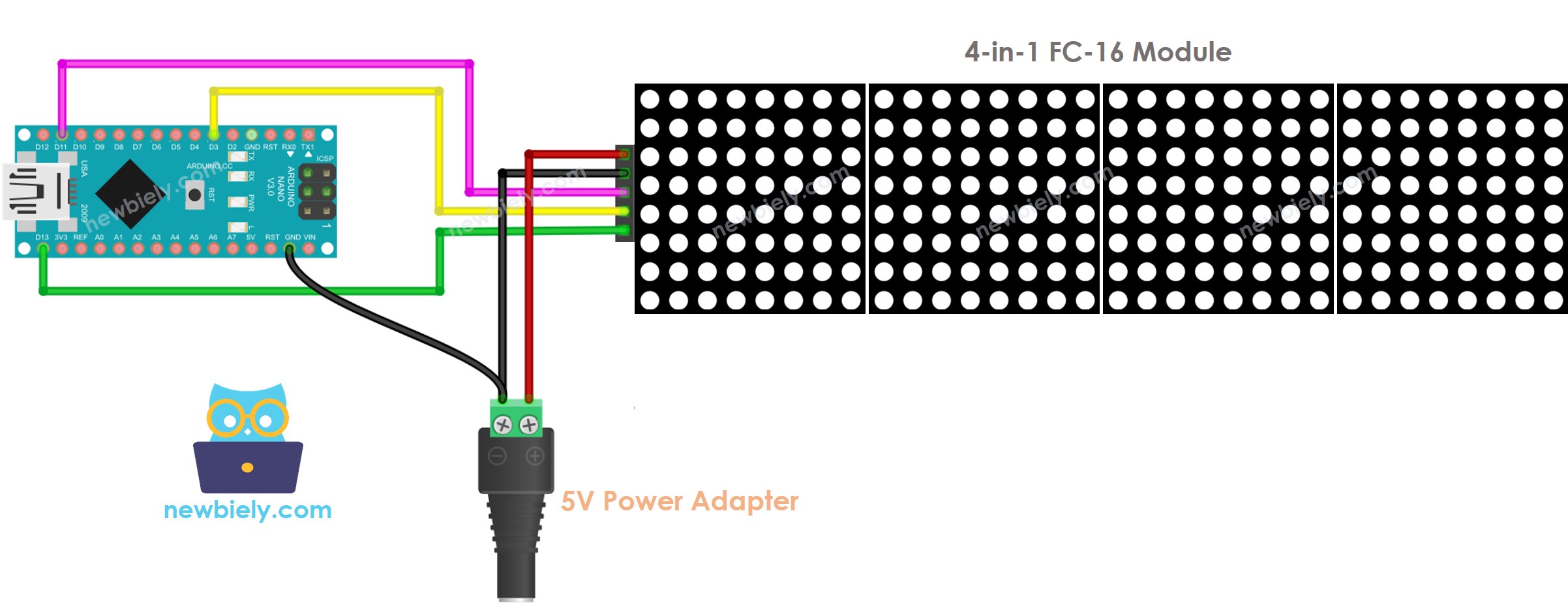
This image is created using Fritzing. Click to enlarge image
If the LED matrix is made of multiple blocks by yourself:
- Connect the input pins groups of the first block to Arduino Nano
- Link the output pins groups of each block to the input pins groups of the following block
- Leave the output pins group of the last block unconnected
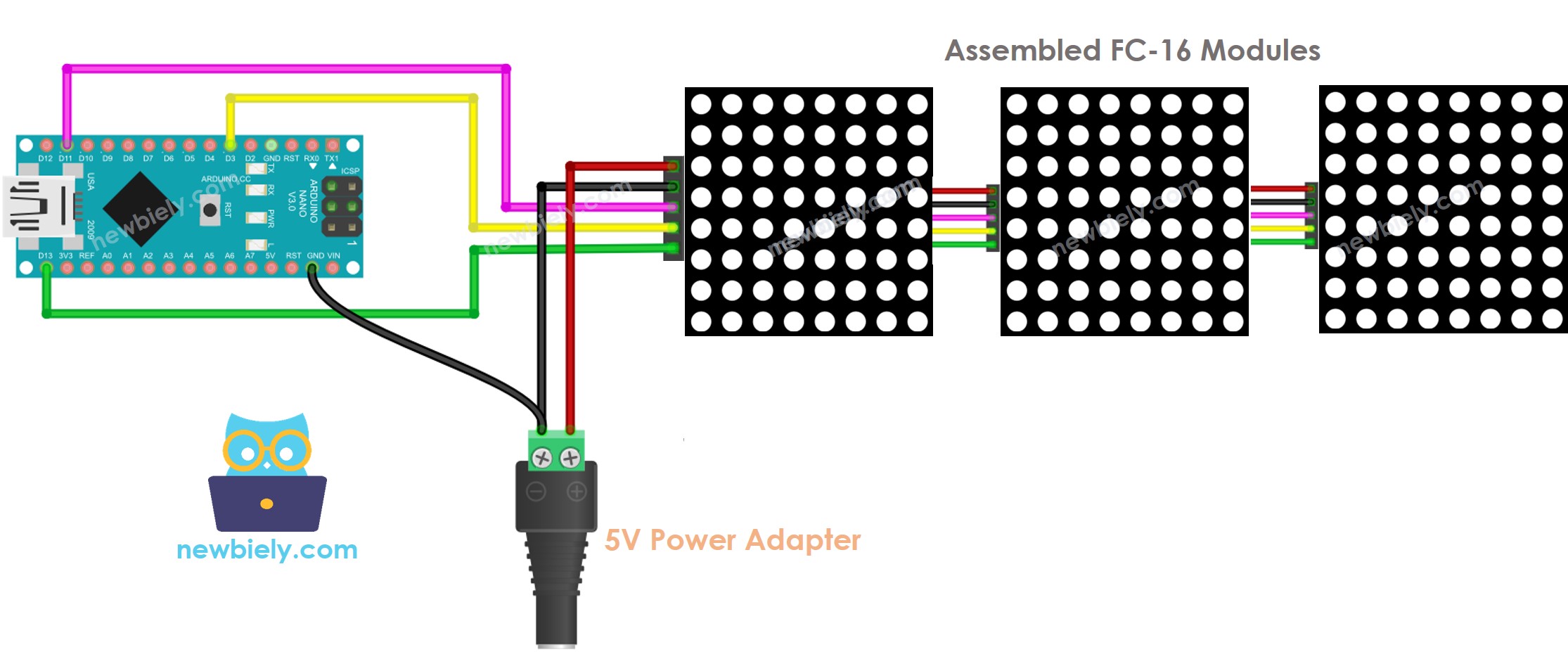
This image is created using Fritzing. Click to enlarge image
See The best way to supply power to the Arduino Nano and other components.
Due to the high current draw of the display (up to 1A at maximum brightness):
- Do not power it from the 5V pin of an Arduino Nano.
- Utilize an external 5V power supply instead. Both the Arduino Nano and LED matrix can be powered by a single 5V power adapter.
Since Arduino Nano is connected to the LED matrix through SPI pins:
- Pin 13 (SCK) and 11 (MOSI) on Arduino Nano should be utilized. If a different Arduino Nano board is being used, consult the official documentation for the equivalent SPI pins.
- Pin 3 (CS) can be altered to any pin on the Arduino Nano.
How To Program For LED Matrix
It is not a simple task to manage the LED matrix. Fortunately, libraries are available that make it much easier. Here is a step-by-step guide on how to write Arduino Nano code to control the LED matrix:
- Include libraries:
- Specify the type of hardware being used: GENERIC_HW or FC16_HW.
- Specify the number of LED blocks used. For instance, a 4-in-1 LED matrix contains four blocks.
- Specify the pin that is connected to the CS pin of the LED matrix. For example, pin D3.
- Create a MD_Parola object for the LED matrix display.
- Code in the setup() function:
- Show text, numerical values and animated effects: take a look at the following section
Arduino Nano - LED Matrix Code
This code is applicable for a 32x8 FC-16 LED matrix display consisting of four blocks. However, it can be adapted to 8x8, 16x8, 64x8 and other sizes with ease.
Detailed Instructions
- Connect Arduino Nano to the LED matrix as per the wiring diagram.
- Connect the Arduino Nano to a PC using a USB cable.
- Click to the Libraries icon on the left bar of the Arduino IDE.)
- Look for “MD_MAX72XX” and then locate the MD_MAX72XX library.
- Press the Install button.
- Search for “MD_Parola”, then locate the MD_Parola library.
- Press the Install button.
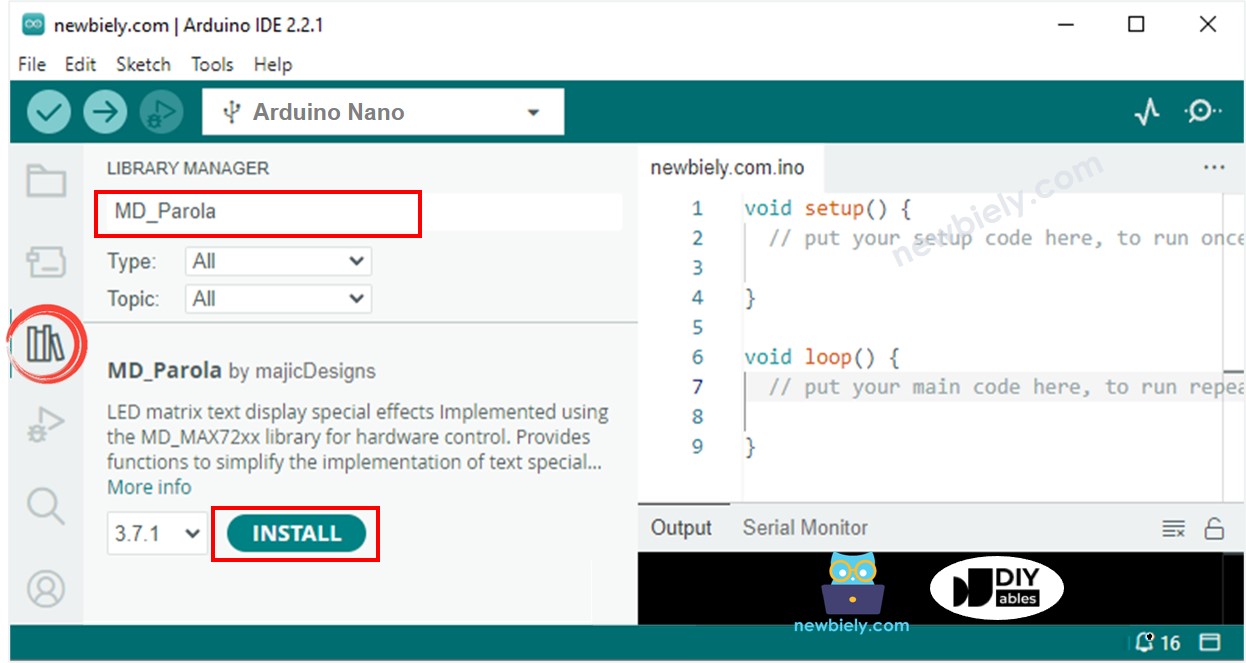
- You will be asked to install the “MD_MAX72XX” library
- Click Install All button to install the dependency.
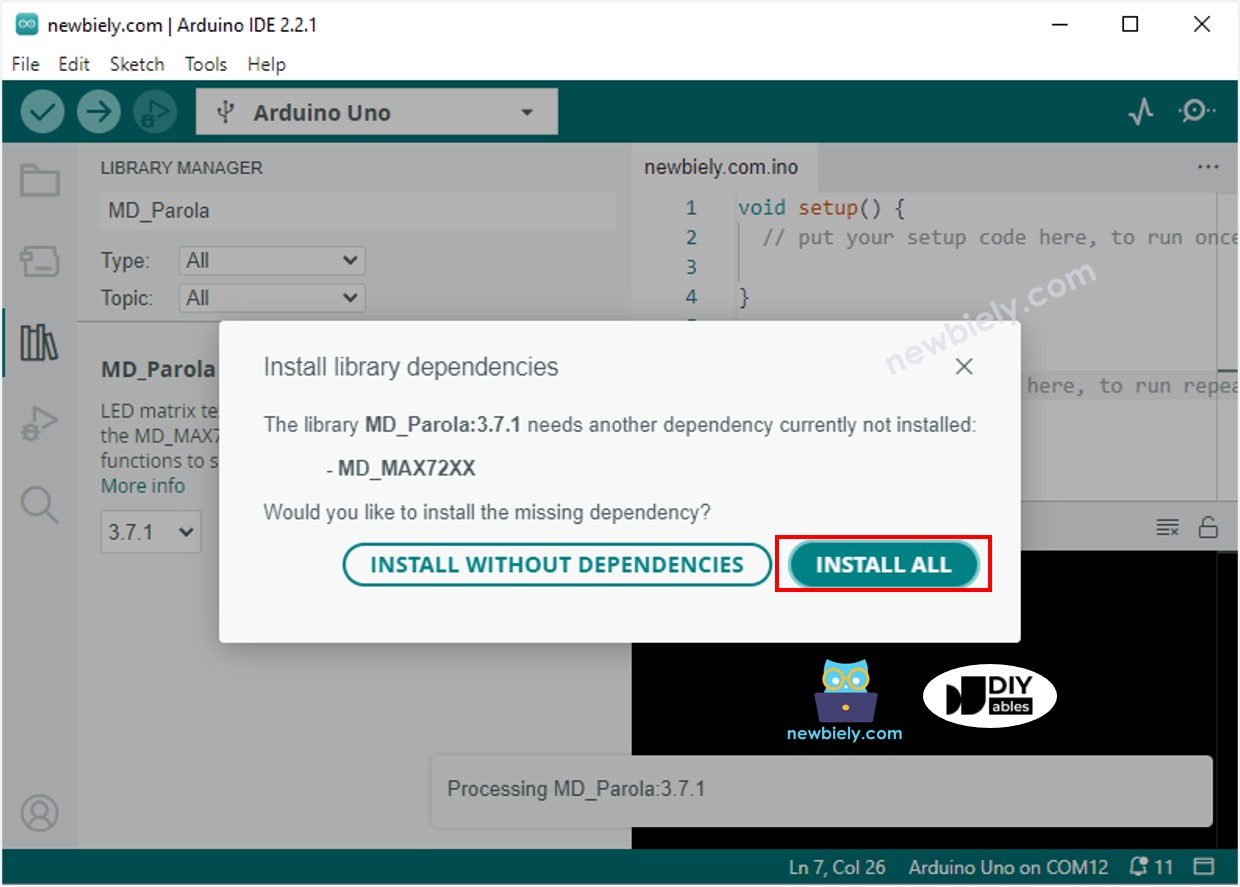
- Copy the code and open it with Arduino IDE.
- Click the Upload button on the IDE to send the code to the Arduino Nano.
- Check out the LED matrix display the message.
Arduino Nano LED Matrix Code – Scrolling Text
If a message is too lengthy to be displayed on a LED matrix, the scroll text effect can be used. This technique allows the text to be scrolled across the display. The scroll text effect enables the message to be moved across the LED matrix, even if it is too long to fit.
This Arduino Nano code illustrates how to move a message across the LED matrix display.
To explore additional text effects, please refer to the MD_Parola Library Reference.