Arduino Nano - Button - Long Press Short Press
This tutorial instructs you how to use Arduino Nano to delect the button's long press and short press. In detail, we will go through the following examples:
- Arduino Nano detects a short press of the button
- Arduino Nano detects a long press of the button
- Arduino Nano detects both a long press and a short press of the button
- Arduino Nano debounces for long press and short press
In the last section, we will learn how to use debounce to detect button presses in practical applications. To learn more about why debouncing is necessary for buttons, please refer to this article. Without it, we may mistakenly detect a short press of the button.
Hardware Preparation
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables .
Wiring Diagram
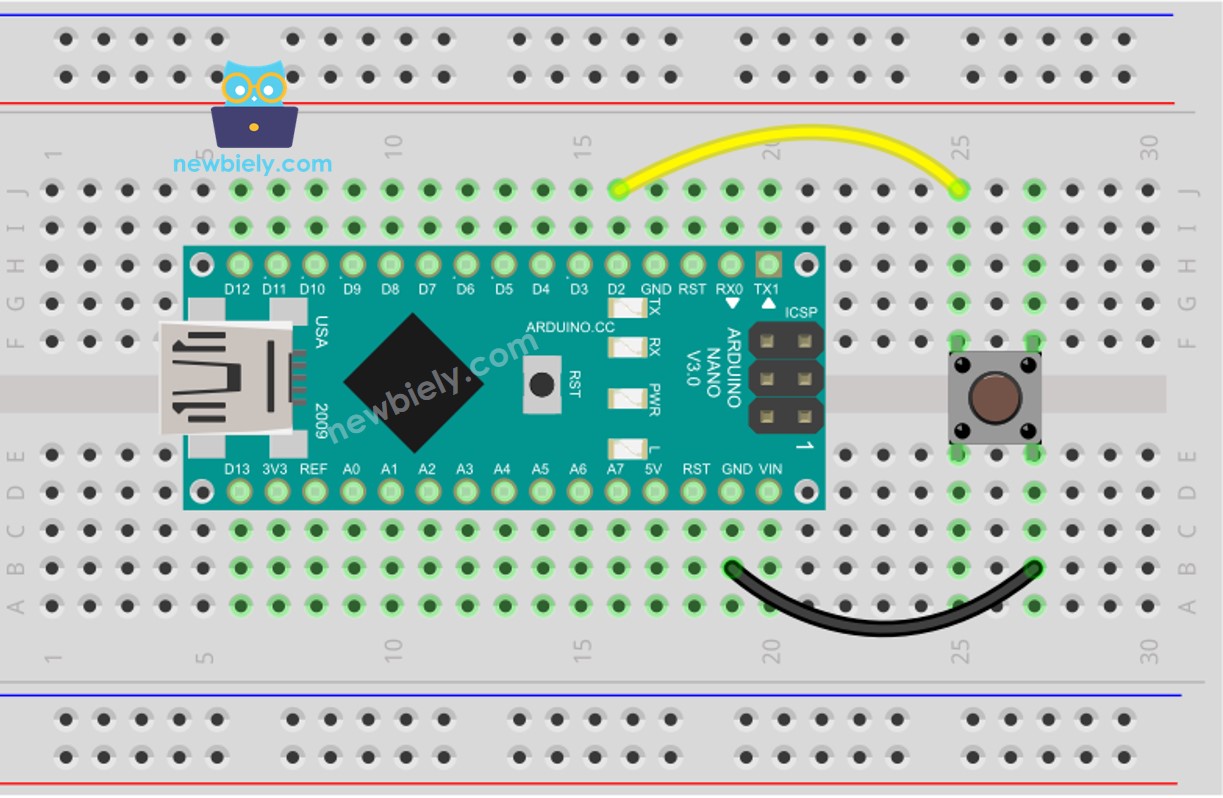
This image is created using Fritzing. Click to enlarge image
See The best way to supply power to the Arduino Nano and other components.
In this tutorial, we will be utilizing the internal pull-up resistor. As a result, the state of the button is HIGH in its normal condition and LOW when it is pressed.
How To Detect Short Press
We calculate the interval between the pressed and released events. If the duration is less than a pre-defined time, then the short press event is identified.
Specify the duration of a short press.
- Identify when the button has been pressed and record the time it was pushed.
- Detect when the button is released and record the time of release.
- Pressure
- Calculate the length of time and the amount of pressure applied.
- Compare the press duration to the specified short press time to identify a short press.
Arduino Nano Code for detecting the short press
Detailed Instructions
- Upload the code to Arduino Nano using the Arduino IDE.
- Press the button briefly multiple times.
- Check the output on the Serial Monitor.
※ NOTE THAT:
The Serial Monitor may display multiple short press detections for a single press. This is the expected behavior of the button, known as the “chattering phenomenon”. We will address this issue in the concluding part of this tutorial.
How To Detect Long Press
There are two scenarios for detecting a long press:
- The long-press event is identified once the button has been released
- The long-press event is identified while the button is being held down, even before it has been released.
- For the initial application, we will calculate the time period between the pressed and released events. 2. If the duration is more than a predetermined amount of time, then the long-press event is identified.
In the second use case, when the button is pressed, the pressing time is measured continuously and the long-press event is checked until the button is released. While the button is being pressed, if the duration exceeds a predefined time, the long-press event is detected.
Arduino Nano Code for detecting long press when released
Detailed Instructions
- Upload the code to Arduino Nano using the Arduino IDE.
- Wait one second and then press and release the button.
- Check out the result on the Serial Monitor.
The event of long-pressing is only identified when the button is let go.
Arduino Nano Code for detecting long press during pressing
Detailed Instructions
- Upload the code to Arduino Nano using the Arduino IDE.
- Press and hold the button for a few seconds.
- Check the output on the Serial Monitor.
The event of long-pressing is only registered when the button has not been released.
How To Detect Both Long Press and Short Press
Short Press and Long Press after released
Detailed Instructions
- Upload the code to the Arduino Nano using the Arduino IDE.
- Press the button for a short or a long time.
- Check the output on the Serial Monitor.
※ NOTE THAT:
The Serial Monitor may display multiple short press detections when a long press is made. This is the expected behavior of the button and is referred to as the "chattering phenomenon". A solution to this issue will be provided in the concluding section of this tutorial.
Short Press and Long Press During pressing
Detailed Instructions
- Upload the code to the Arduino Nano using the Arduino IDE.
- Press the button for a long time and then a short time.
- Check out the result on the Serial Monitor.
※ NOTE THAT:
The Serial Monitor may display multiple short presses being detected when a long press is made. This is the expected behavior of the button and is referred to as the “chattering phenomenon”. This issue will be addressed in the concluding section of this tutorial.
Long Press and Short Press with Debouncing
It is essential to implement debouncing on the button in numerous applications.
Debouncing can be complicated, particularly when multiple buttons are involved. To make it simpler for novices, we have developed a library, known as ezButton.
We will use this library in the codes below.
Short Press and Long Press with debouncing after released
Detailed Instructions
- Install the ezButton library.
- Refer to How To for instructions.
- Upload the code to Arduino Nano using the Arduino IDE.
- Press and hold the button for a short or long period of time.
- Check out the results on the Serial Monitor.
Short Press and Long Press with debouncing During Pressing
Detailed Instructions
- Include the ezButton library in your project. Refer to How To for instructions.
- Compile and upload the code to your Arduino Nano board using the Arduino IDE.
- Press and hold the button for a short or long period of time.
- Check out the output on the Serial Monitor.
Video Tutorial
Why Needs Long Press and Short Press
- To minimize the number of buttons, a single button can have multiple functions. For instance, a short press can be used to switch the operation mode, while a long press can be used to turn off the device.
- Long pressing is used to prevent accidental activation. For example, some devices use a button for factory reset. If it is pressed unintentionally, it could be hazardous. To prevent this, the device is designed so that factory reset is only triggered when the button is held down for a certain amount of time (e.g. 5 seconds).