Arduino Nano - Joystick
This tutorial instructs you how to use Joystick with Arduino Nano. In detail, we will learn:
- How a 2-axis joystick works
- How to connect joystick to Arduino Nano and programming for it
- How to convert the values from joystick into controllable values such as XY coordinates, the motor's up/down/left/right direction...
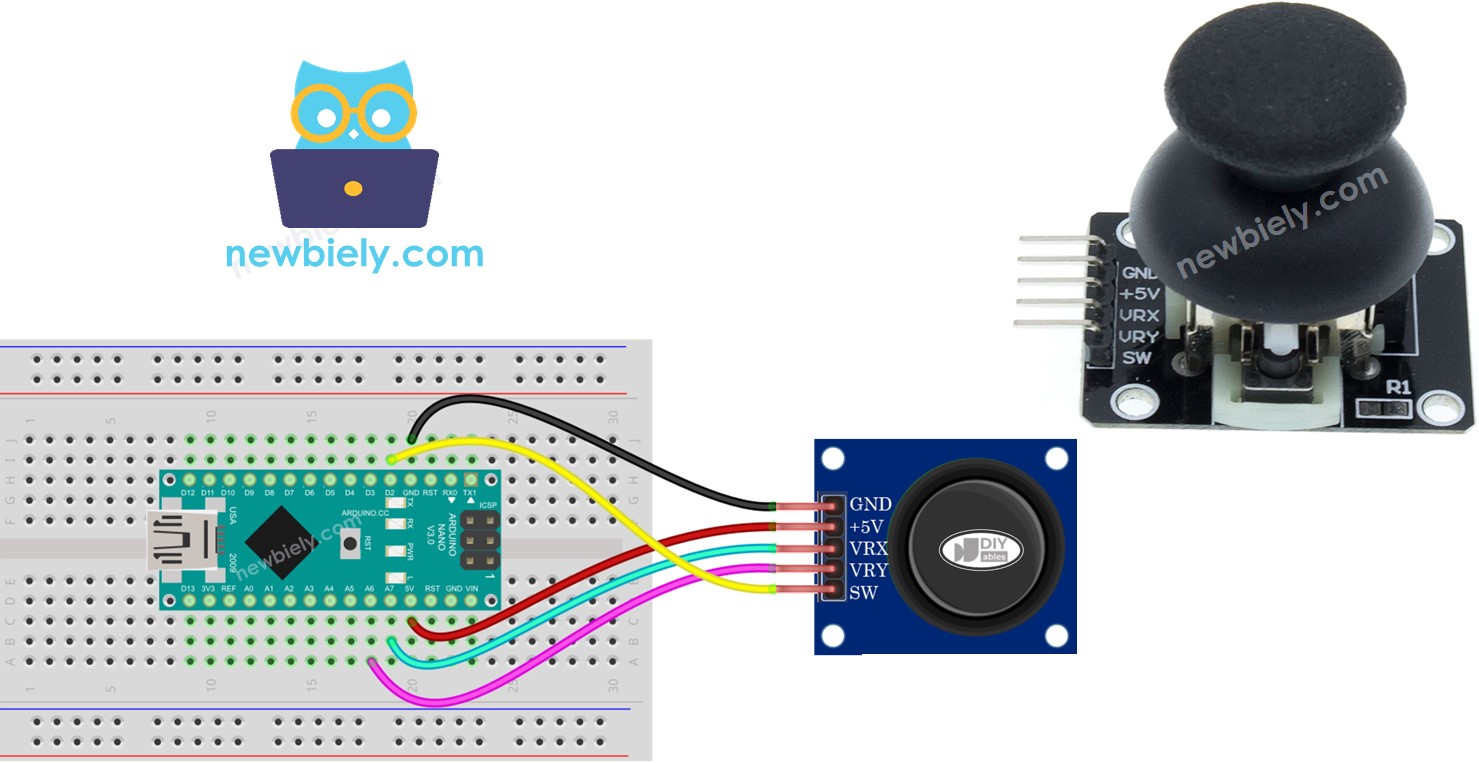
Hardware Preparation
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables.
Overview of 2-axis Joystick
You may have encountered a joystick in various places, like a game controller, a toy controller, or even a large machine such as an excavator's control panel.
The joystick is constructed of two potentiometers that are perpendicular to each other and a push button. As such, it produces the following outputs:
- An analog value ranging from 0 to 1023 (read by Arduino) that corresponds to the horizontal position (known as the X-coordinate)
- An analog value ranging from 0 to 1023 (read by Arduino) that corresponds to the vertical position (known as the Y-coordinate)
- A digital value of the push button (either HIGH or LOW)
Therefore:
- Two analog values can be merged to create 2-D coordinates.
- The center of the 2-D coordinate system is the resting position of the joystick.
- To determine the direction of the joystick, execute a test code which will present this information in the next section.
Some applications may use all three outputs, while others may utilize only some of them.
The Joystick Pinout
A Joystick has 5 pins:
- GND pin: This needs to be connected to GND (0V).
- VCC pin: This needs to be connected to VCC (5V).
- VRX pin: This outputs an analog value that corresponds to the horizontal position (known as the X-coordinate).
- VRY pin: This outputs an analog value that corresponds to the vertical position (known as the Y-coordinate).
- SW pin: This is the output from the pushbutton inside the joystick. It’s normally open. If a pull-up resistor is used in this pin, the SW pin will be HIGH when it is not pressed, and LOW when it is pressed.
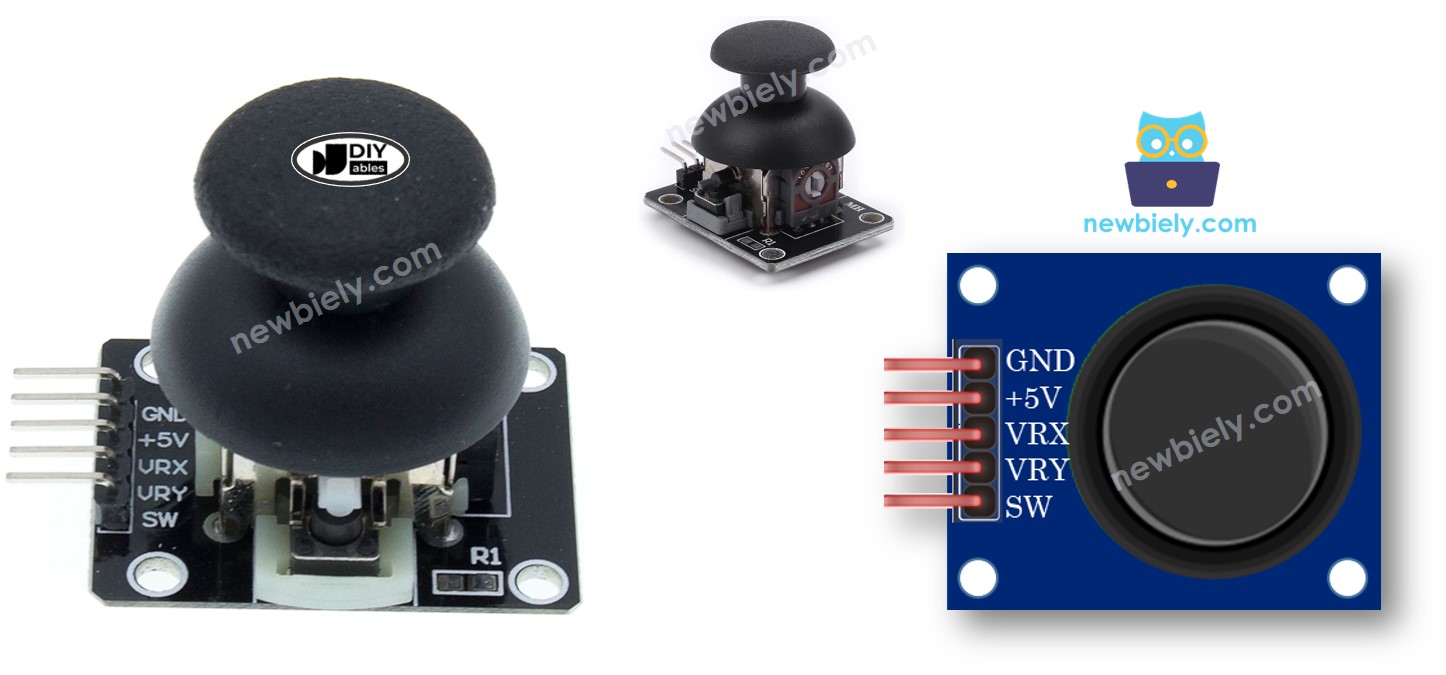
How It Works
- When you move the joystick's thump to the left or right, the voltage in the VRX pin varies from 0 to 5V, with 0 being at the left and 5V at the right, resulting in a reading value on Arduino's analog pin from 0 to 1023.
- When you move the joystick's thump up or down, the voltage in the VRY pin varies is from 0 to 5V, with 0 being at the top and 5V at the bottom, resulting in a reading value on Arduino's analog pin from 0 to 1023.
- When you move the joystick's thump in any direction, the voltage in both VRX and VRY pins is changed in proportion to the projection of position on each axis.
- When you push the joystick's thump from top to bottom, the pushbutton inside the joystick is closed. If we use a pull-up resistor in the SW pin, the output from SW pin will change from 5V to 0V, resulting in a reading value on Arduino's digital pin changed from HIGH to LOW.
Wiring Diagram
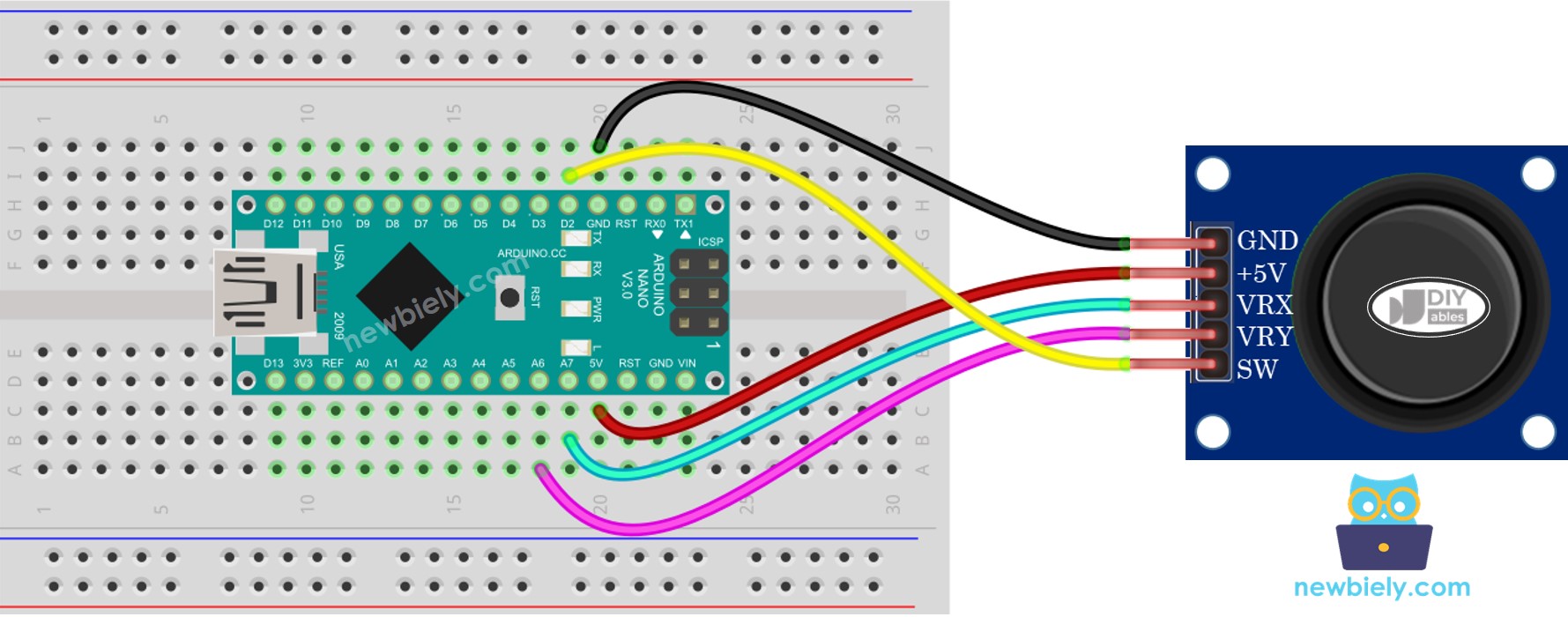
This image is created using Fritzing. Click to enlarge image
How To Program For Joystick
The joystick is composed of two components: analog (X, Y axis) and digital (pushbutton):
- For the analog parts (X, Y axis), simply read the value from the analog input pin using the analogRead() function.
- For the digital part (pushbutton): it is a button. The simplest and most convenient way to use it is with the ezButton library. This library has debounce support for buttons, as well as an internal pull-up resistor. To learn more about buttons, you can check out the Arduino Nano - Button tutorial. The code for this will be presented in the following session of this tutorial.
After obtaining the values from analog pins, we may need to convert them into controllable values. The following section will provide example codes for this.
Arduino Nano Code
This section will give Arduino Nano example codes as follows:
- Example code: read analog values from joystick
- Example code: read analog values and button state from joystick
- Example code: convert analog value to MOVE_LEFT, MOVE_RIGHT, MOVE_UP, MOVE_DOWN commands
- Example code: convert analog values to angles to manage two servo motors (e.g. in pan-tilt camera)
Reads analog values from joystick
Detailed Instructions
- Copy the code and open it in the Arduino IDE.
- Click the Upload button to transfer the code to the Arduino Nano.
- Push the joystick's thump all the way and rotate it in a circular motion (clockwise or counterclockwise).
- Check out the result on the Serial Monitor.
While turning the joystick's thumb, keep an eye on the Serial Monitor.
If the X value is 0, note or remember the current position as left, with the opposite being right.
If the Y value is 0, note or remember the current position as up, with the opposite being down.
Reads analog values and reads the button state from a joystick
Detailed Instructions
- Click to the Libraries icon on the left bar of the Arduino IDE.
- Search for “ezButton” and locate the button library from ArduinoGetStarted.com.
- Then, click the Install button to install the ezButton library.
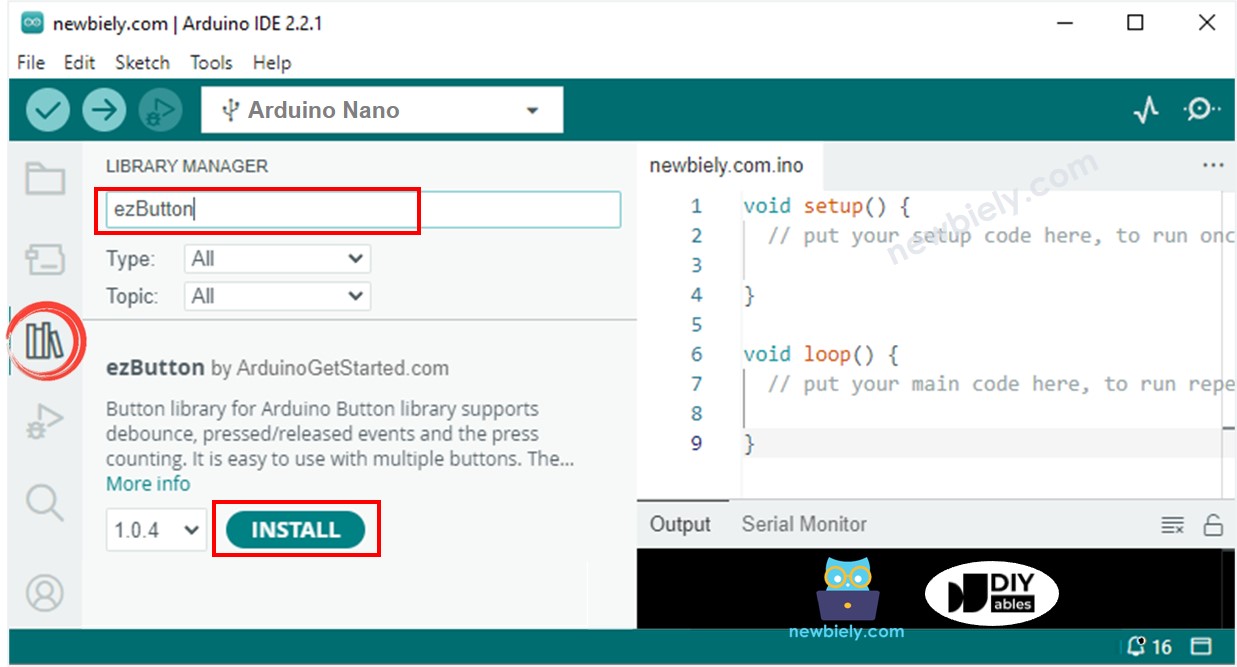
- Copy the code and open it with the Arduino IDE.
- Click the Upload button to send it to the Arduino Nano.
- Move the joystick's thumb in the left, right, up, and down directions.
- Push the joystick's thumb from the top.
- Check out the result on the Serial Monitor.
Converts analog value to MOVE LEFT/RIGHT/UP/DOWN commands
Detailed Instructions
- Copy the code and open it with the Arduino IDE.
- Click the Upload button in the IDE to send the code to the Arduino Nano.
- Move the joystick in any direction with your thumb.
- Check the results in the Serial Monitor.
※ NOTE THAT:
At any given moment, there could be no command, one command, or two commands (e.g. UP and LEFT simultaneously).
Converts analog values to angles to control two servo motors
The specifics can be found in the tutorial titled Arduino Nano - Joystick controls Servo Motor.