Arduino Nano - Write Variable to SD Card
This tutorial instructs you how to write different types of variables to a Micro SD Card using Arduino Nano. Specifically, we will look at:
- Writing a string variable to a Micro SD Card with Arduino Nano
- Writing an int variable to a Micro SD Card with Arduino Nano
- Writing a float variable to a Micro SD Card with Arduino Nano
- Writing a char array variable to a Micro SD Card with Arduino Nano
- Writing a byte array variable to a Micro SD Card with Arduino Nano
- Writing a variable as a key-value pair to a Micro SD Card with Arduino Nano
To get the key-value from the Micro SD Card and change it to int, float, or string, refer to Arduino Nano - Read Config from SD Card. For instructions on how to read the key-value from the Micro SD Card and convert it to int, float, or string, have a look at Arduino Nano - Read Config from SD Card
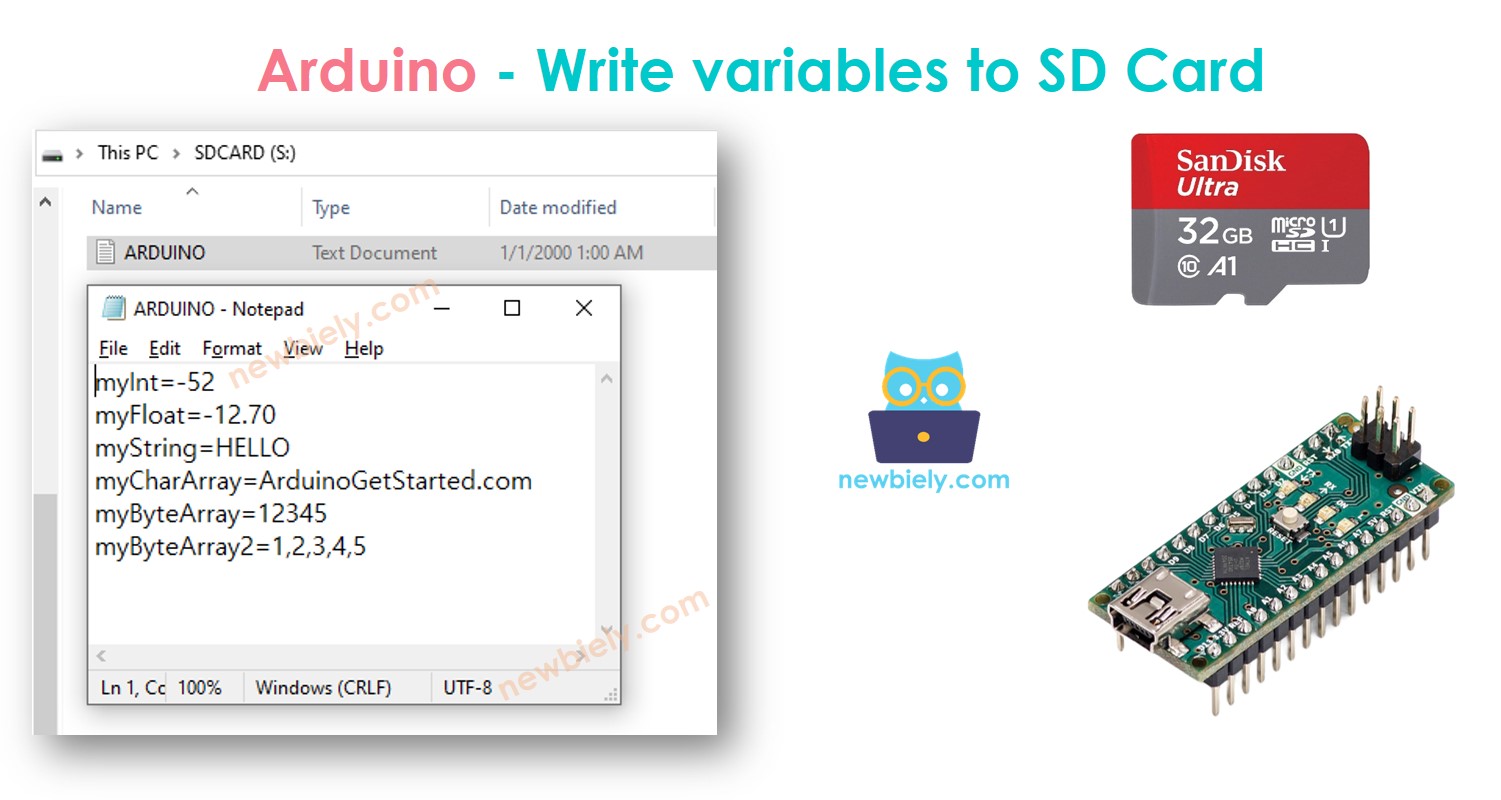
Hardware Preparation
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Overview of Micro SD Card Module
If you are unfamiliar with the Micro SD Card Module, including its pinout, how it works, and how to program it, you can find out more in the Arduino Nano - Micro SD Card tutorial.
Wiring Diagram
- You can use male-to-female jumper wires to connect the micro SD Card module to Arduino Nano
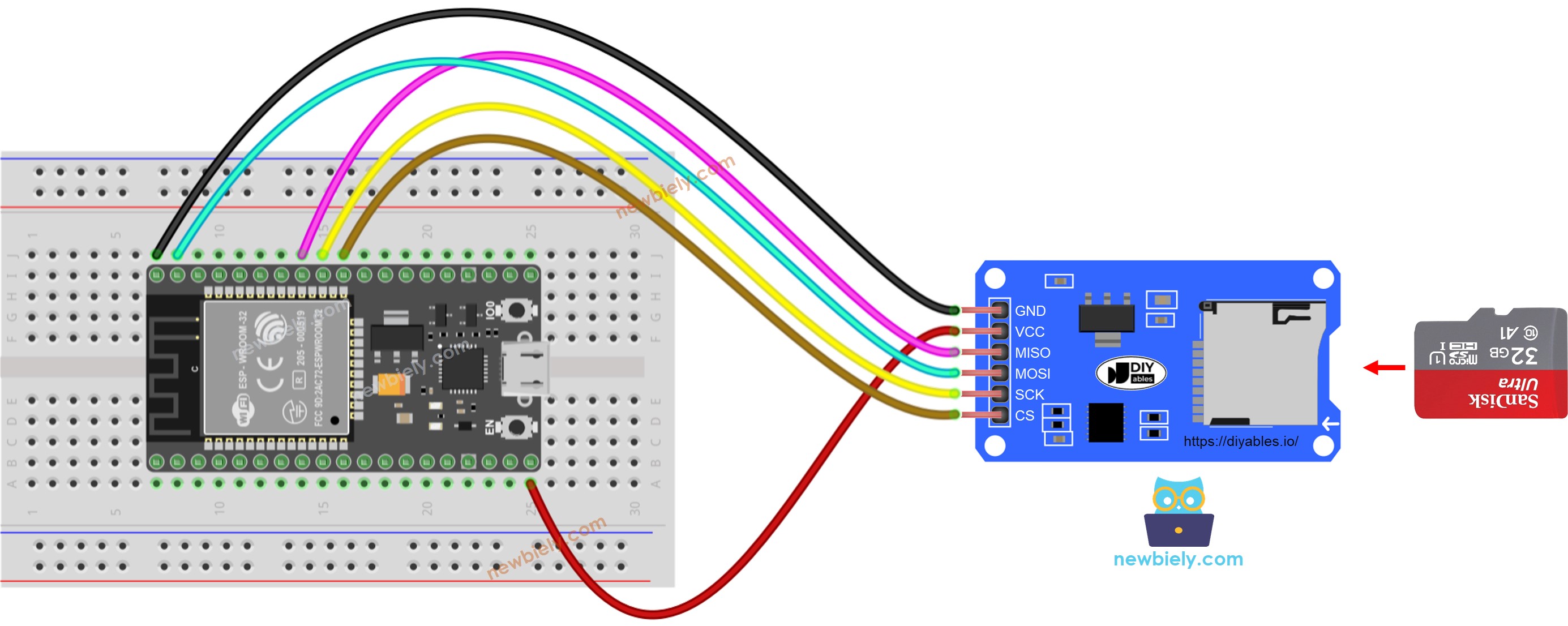
This image is created using Fritzing. Click to enlarge image
- Or you can plug the micro SD Card module to breadboard and then use the male-to-male jumper wires
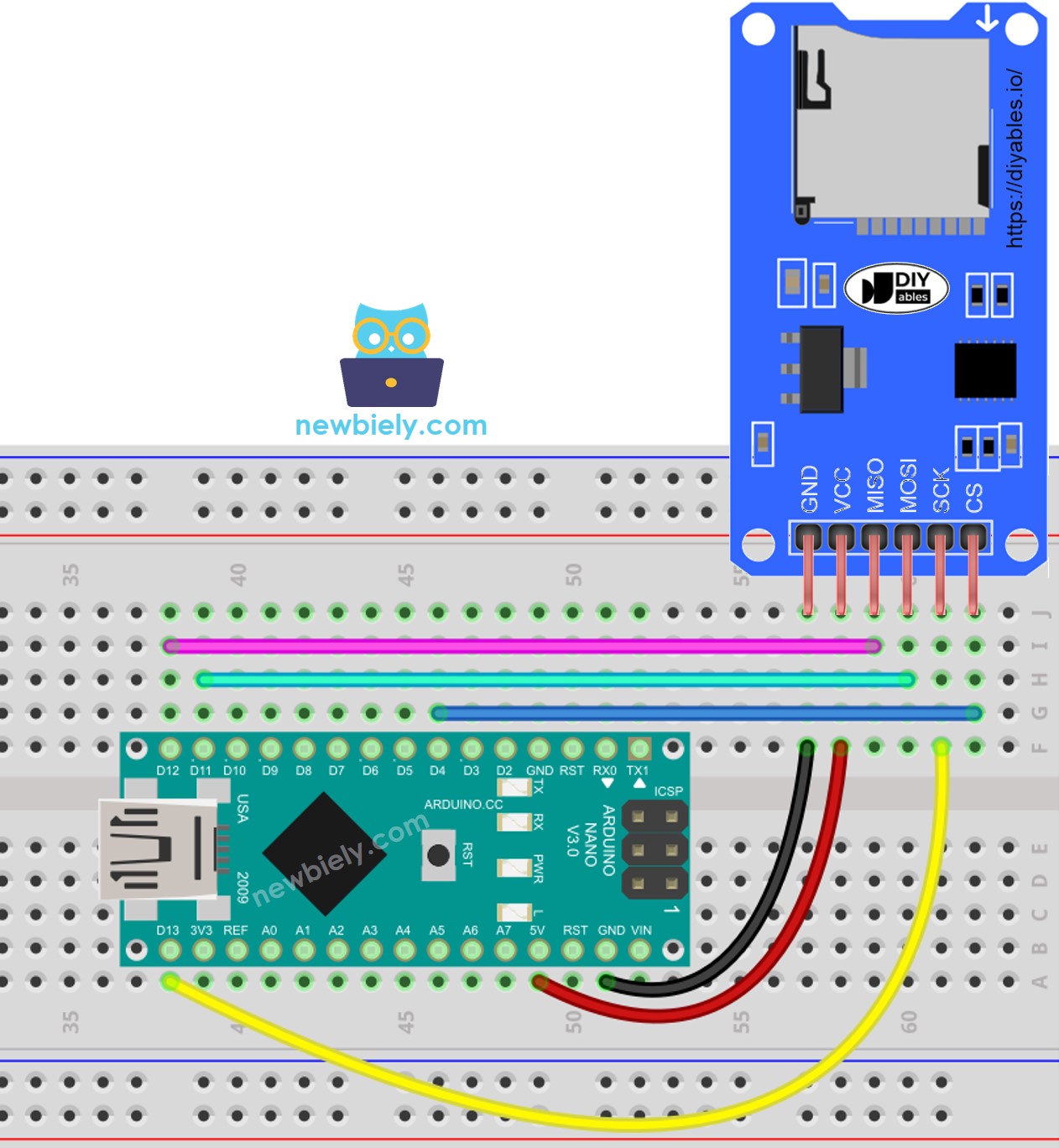
This image is created using Fritzing. Click to enlarge image
Arduino Nano - How to write a variable to a file on Micro SD Card
The following code:
- Stores an integer value on a Micro SD Card
- Stores a floating point value on a Micro SD Card
- Stores a string on a Micro SD Card
- Stores a character array on a Micro SD Card
- Stores a byte array on a Micro SD Card
Detailed Instructions
- Ensure that the Micro SD Card is formatted as either FAT16 or FAT32 (you can find instructions on how to do this by searching on Google).
- Copy the code and open it in the Arduino IDE.
- Then, press the Upload button in the Arduino IDE to upload the code to the Arduino Nano.
- Finally, view the result in the Serial Monitor.
- Remove the Micro SD Card from the Micro SD Card module.
- Insert the Micro SD Card into an USB SD Card reader.
- Connect the USB SD Card reader to the PC.
- Open the arduino-nano.txt file on your PC; it appears as follows.
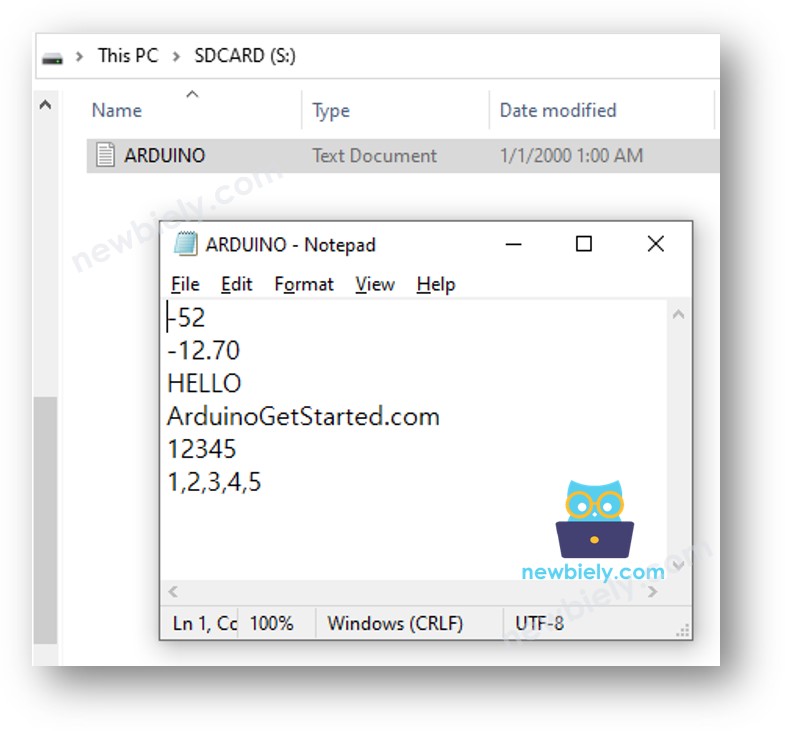
Arduino Nano - How to write a key-value to a file on Micro SD Card
Detailed Instructions
- Copy the code and open it using the Arduino IDE.
- Click the Upload button on the Arduino IDE to send the code to the Arduino Nano.
- Check out the result on the Serial Monitor.
- Remove the Micro SD Card from the Micro SD Card module.
- Insert the Micro SD Card into an USB SD Card reader.
- Plug the USB SD Card reader into the PC.
- Open the arduino-nano.txt file on your computer, it appears as follows:
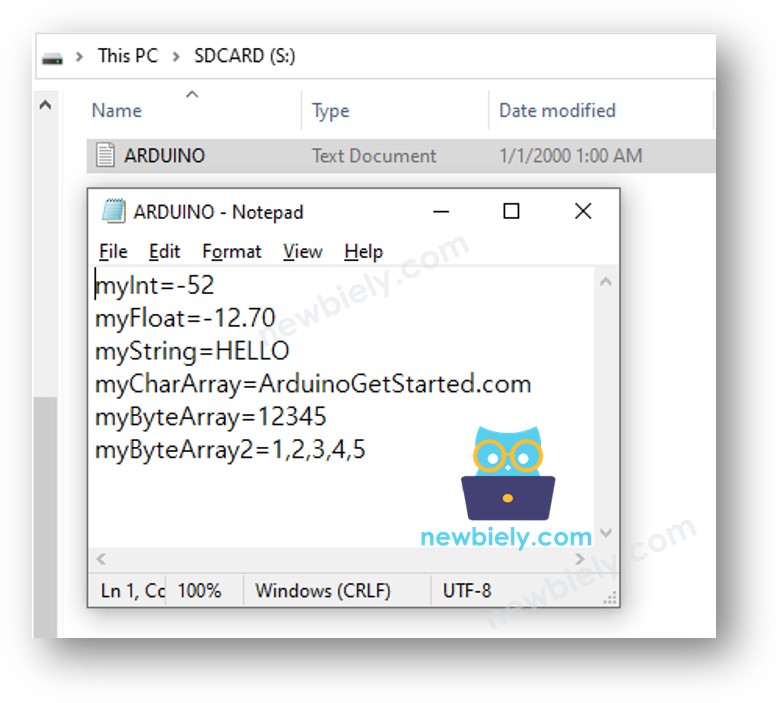