Arduino Nano - Button Count - LCD
This tutorial instructs you how to use Arduino Nano to count the button's press and display the value on LCD display. It is possible to adapt this for alternative sensors, rather than just the button.
In this tutorial, we will be debouncing the button without using the delay() function. For further information on why debouncing is necessary, please refer to Why do we need debouncing?.
Hardware Preparation
Or you can buy the following kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Disclosure: Some of the links provided in this section are Amazon affiliate links. We may receive a commission for any purchases made through these links at no additional cost to you.
Additionally, some of these links are for products from our own brand, DIYables .
Additionally, some of these links are for products from our own brand, DIYables .
Wiring Diagram
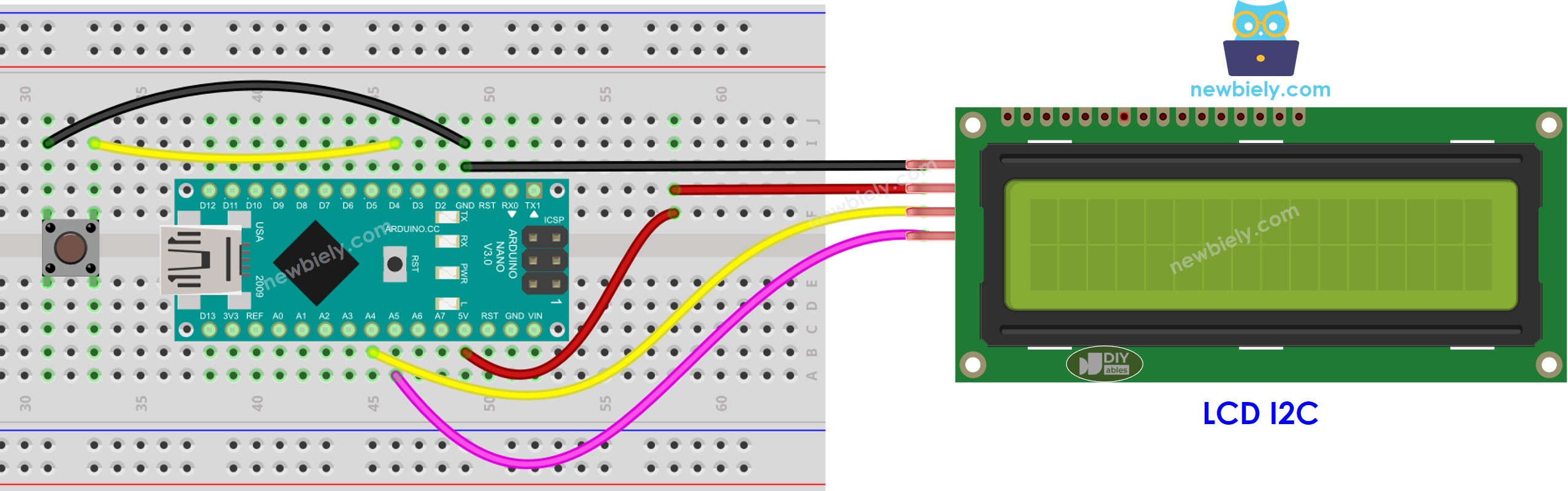
This image is created using Fritzing. Click to enlarge image
See The best way to supply power to the Arduino Nano and other components.