Arduino Nano - Cooling System using DHT Sensor
This tutorial instructs you how to use Arduino Nano to control temperature with a fan and either a DHT11 or DHT22 sensor.
- When the temperature is too high, the cooling fan should be activated.
- When the temperature is low, the cooling fan should be turned off.
If you would prefer to use a DS18B20 sensor instead of a DHT sensor, please refer to Arduino Nano - Cooling System using DS18B20 Sensor.
Hardware Preparation
You can use DHT22 sensor instead of DHT11 sensor.
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Overview of Cooling Fan and DHT Sensor
The fan for this tutorial requires a 12v power supply. If the power is supplied, the fan will turn on and if not, it will remain off. To control the fan with Arduino Nano, a relay must be used as an intermediary.
If you are not familiar with temperature sensor and fan (pinout, how it works, how to program ...), the following tutorials can help you understand them:
Wiring Diagram
- A schematic of the wiring for a system that includes a DHT11 module.
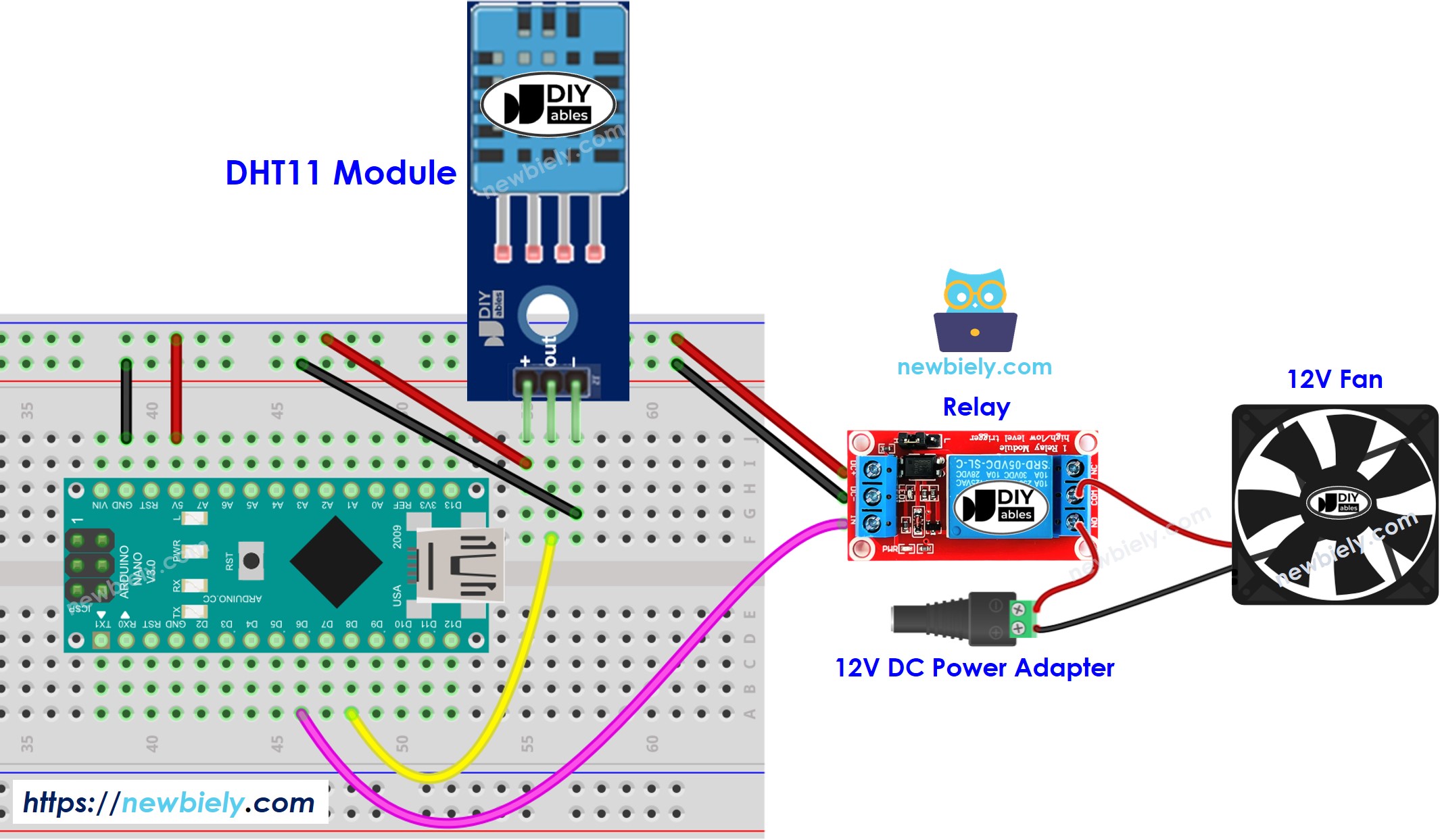
This image is created using Fritzing. Click to enlarge image
- A diagram showing the connections between the DHT22 module and other components.
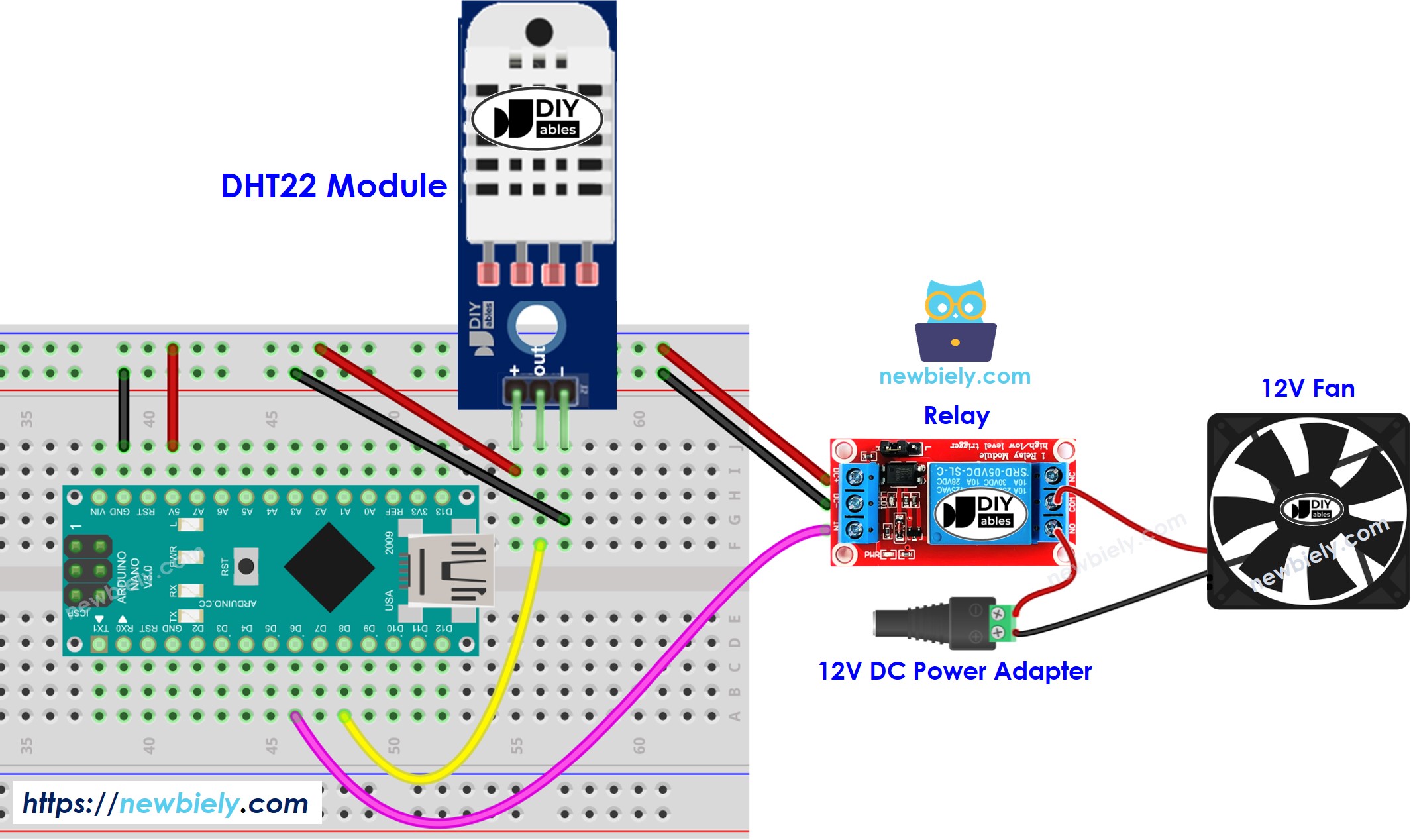
This image is created using Fritzing. Click to enlarge image
How System Works
- Arduino Nano obtains the temperature from the temperature sensor.
- If the temperature is higher than the upper threshold, Arduino Nano turns on the fan.
- If the temperature is lower than the lower threshold, Arduino Nano turns off the fan.
The loop is repeated continuously.
Arduino Nano Code
Arduino Nano Code for Cooling System with DHT11 sensor
Arduino Nano Code for Cooling System with DHT22 sensor
In the codes shown above, the Arduino Nano will activate the fan when the temperature is greater than 25°C and keep it running until the temperature drops below 20°C.
Detailed Instructions
- Connect Arduino Nano to a computer using a USB cable
- Launch the Arduino IDE, select the appropriate board and port
- Click to the Libraries icon on the left bar of the Arduino IDE.
- Search for “DHT” and locate the Adafruit DHT sensor library.
- Then, press the Install button to complete the installation.
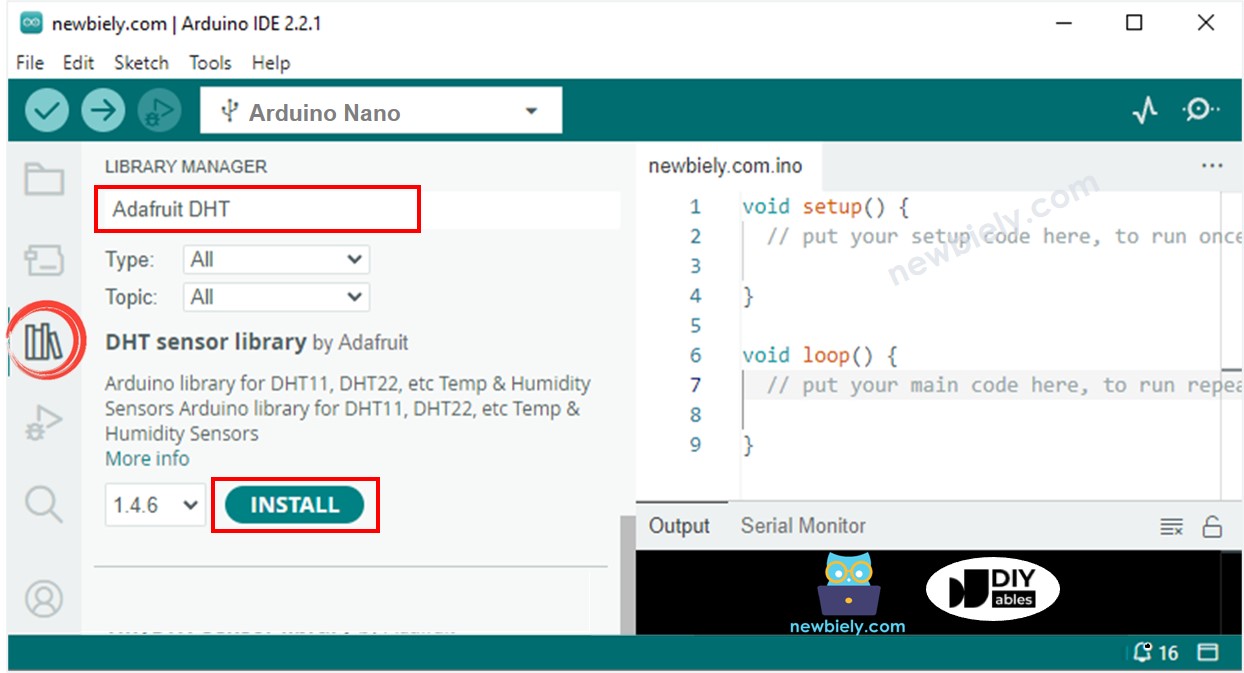
- You will be asked to install some other library dependencies.
- Click the Install All button to install all library dependencies.
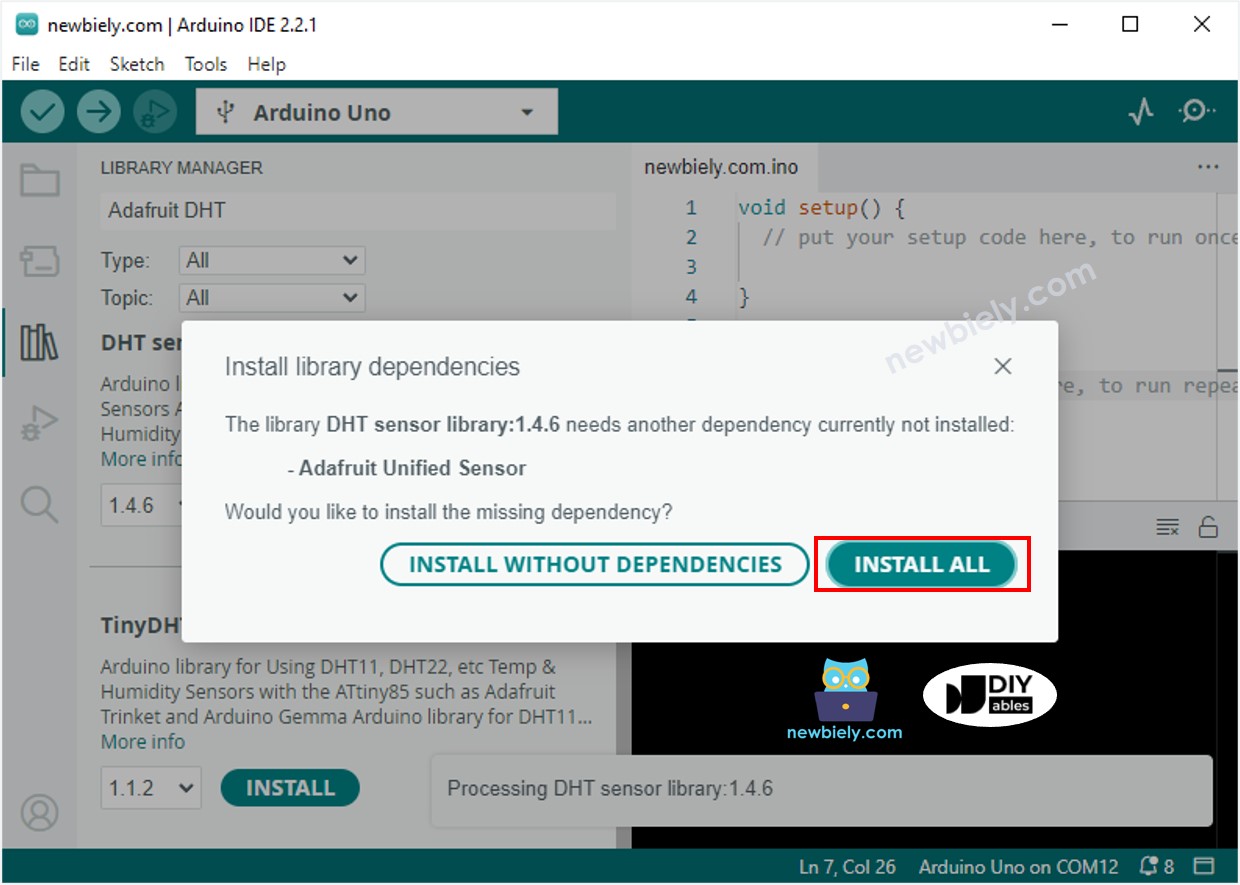
- On serial monitor
- Copy the code that corresponds to the sensor you have and open it with the Arduino IDE.
- Click the Upload button on the Arduino IDE to compile and upload the code to the Arduino Nano.
- Change the temperature of the environment around the sensor.
- Check the status of the fan on the serial monitor.
Advanced Knowledge
The above technique of regulation is the on-off controller, also referred to as a signaller or "bang-bang" controller. This approach is quite easy to execute.
An alternate approach known as the PID controller exists. This method is more effective in maintaining a steady temperature, however it is complex and challenging to comprehend and apply. As a result, the PID controller is not commonly used for temperature regulation.