Arduino Nano - Measure Voltage
In this guide, we will learn how to measure voltage between 0V and 25V using a voltage sensor with an Arduino Nano. We will discuss:
- How to attach a voltage sensor to Arduino Nano
- How to set up Arduino Nano to measure voltage from the sensor
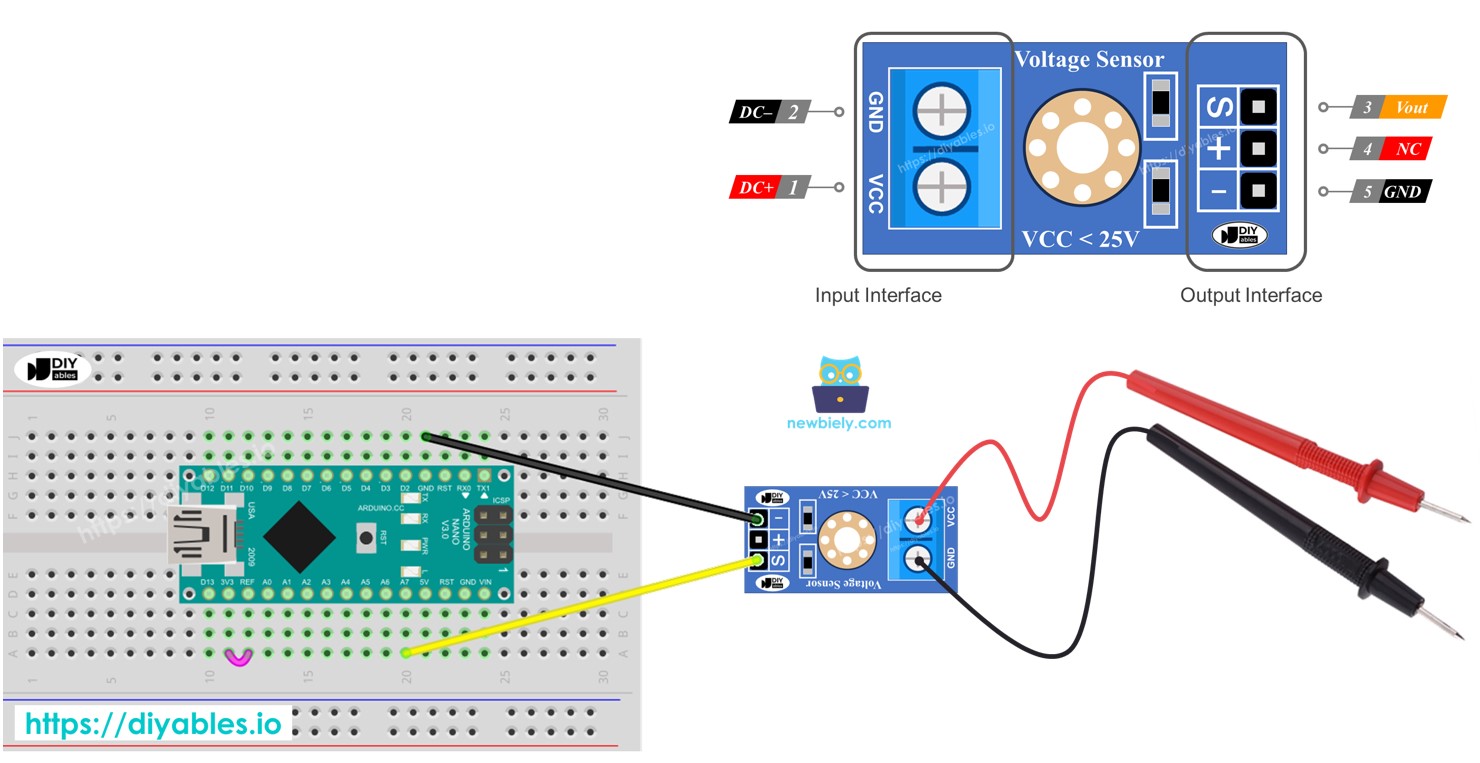
Hardware Preparation
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables .
Overview of Voltage Sensor
A Voltage Sensor is a ready-to-use circuit that divides voltage for easy measuring. It has two resistors: one 30 KΩ and one 7.5 KΩ. With a reference voltage of 5V for the ADC, the sensor can measure voltages from 0 to 25V DC. If the reference voltage of the ADC is 3.3V, the sensor can measure from 0 to 16.5V DC.
Pinout
A voltage sensor includes two groups of pins.
- Input Interface (connect where you need to measure voltage):
- VCC pin: This is the positive pin. Connect it to the point with higher voltage.
- GND pin: This is the negative pin. Connect it to the point with lower cost.
- Output Interface (connect to the Arduino Nano):
- Vout pin (S): This is the signal pin. Connect it to an analog input on the Arduino Nano.
- NC pin (+): Do not connect this.
- GND pin (-): This is the ground pin. Connect it to the GND (0V) on the Arduino Nano.
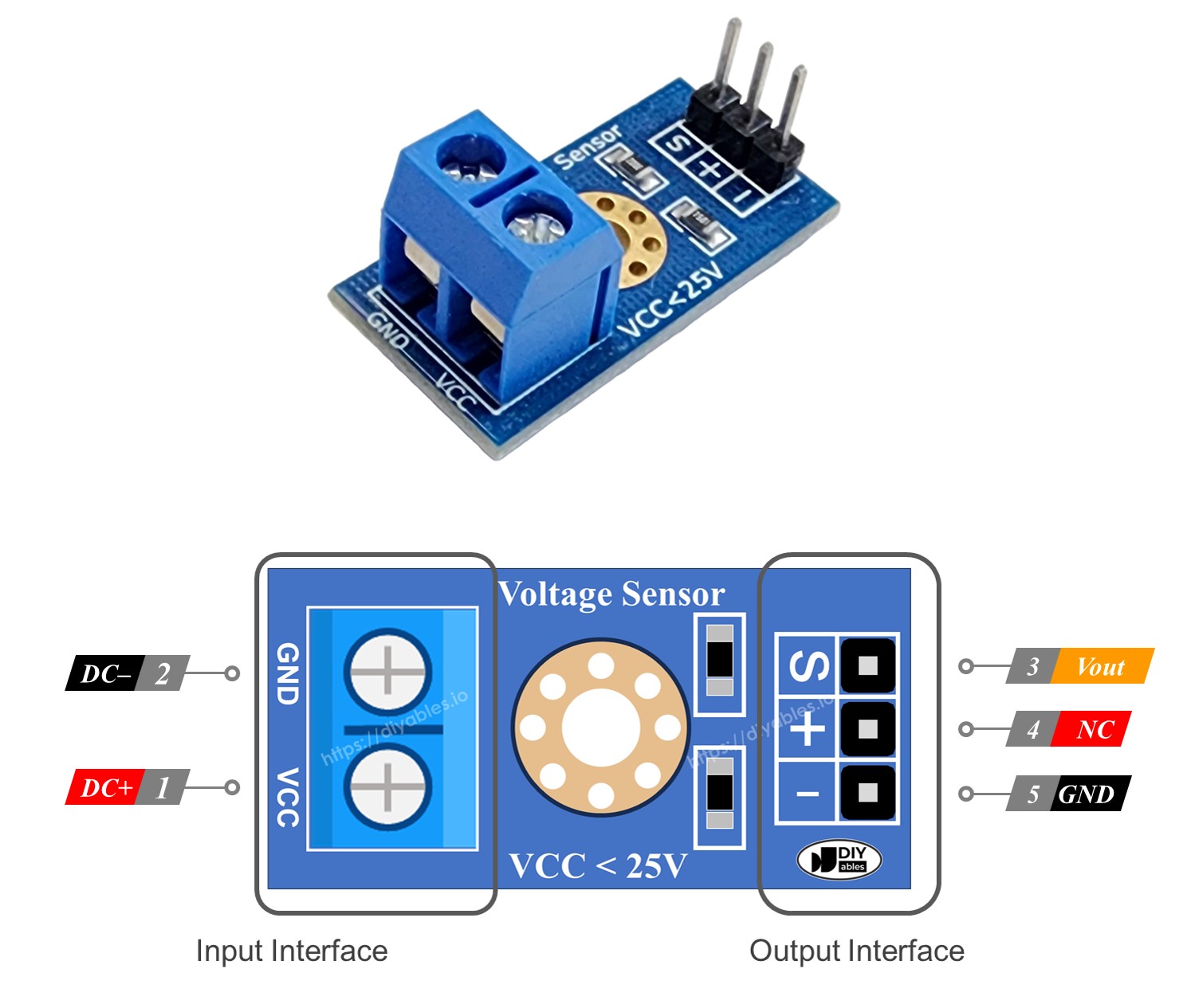
Wiring Diagram
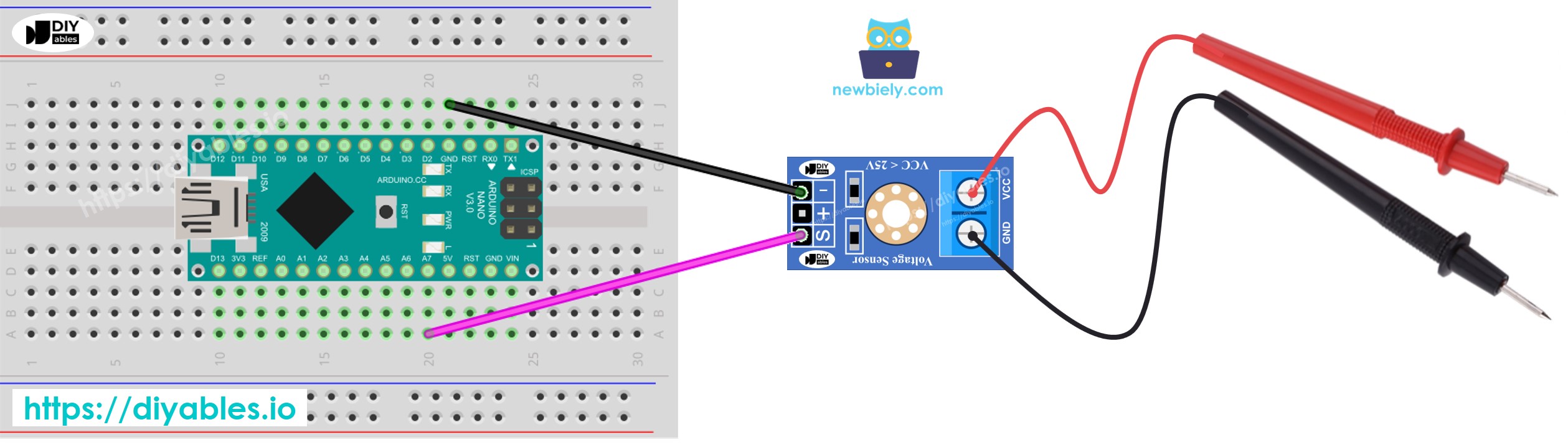
This image is created using Fritzing. Click to enlarge image
Arduino Nano Code
Detailed Instructions
- Connect the Arduino Nano to the voltage sensor.
- Connect the Arduino Nano to a computer using a USB cable.
- Open the Arduino IDE and select the correct board and port.
- Copy the code and open it in the Arduino IDE.
- Click the Upload button in the Arduino IDE to transfer the code to the Arduino Nano.
- Test by measuring 5 volts and 3.3 volts on the Arduino Nano.
- Check the results on the Serial Monitor.
You might observe that the measurement result is incorrect or significantly different from the actual value. This isn't the fault of the voltage sensor module. The measured value may drift because the default voltage reference is 5V, which can be unstable and dependent on the power supply. Here are some solutions to address this issue:
- Ensure the power supply provides sufficient voltage for the Arduino. You can verify this by using a voltmeter to check if the 5V pin on the Arduino outputs 5V.
- Use an external voltage reference of 3.3V. However, with this method, you can only measure voltages from 0 to 16.5V DC.
Measuring Voltage with a 3.3V Reference
To use this method, you need to set up both the hardware and the code. For the hardware, connect the AREF pin of the Arduino to 3.3V as shown in the diagram below.
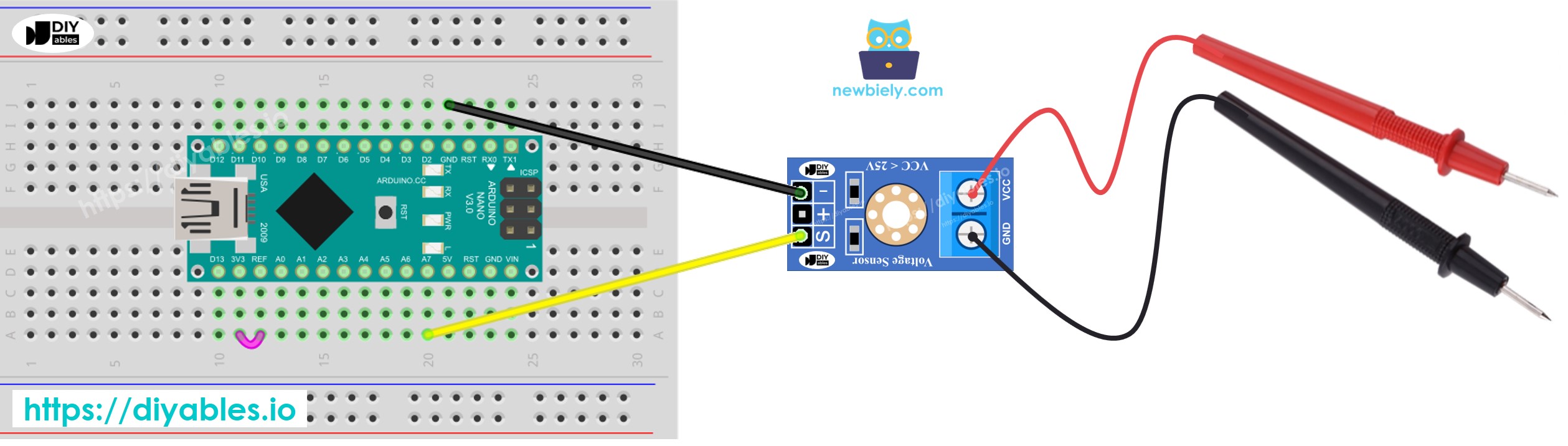
This image is created using Fritzing. Click to enlarge image
See The best way to supply power to the Arduino Nano and other components.
Then, use the following code: