Arduino Nano - Ethernet
This guide shows you how to connect the Arduino Nano to the internet or your local network using the W5500 Ethernet module. We will learn the following details:
- Connecting Arduino Nano to W5500 Ethernet Module
- Writing Code for Arduino Nano to Make HTTP Requests via Ethernet
- Creating a simple Web Server on Arduino Nano with Ethernet
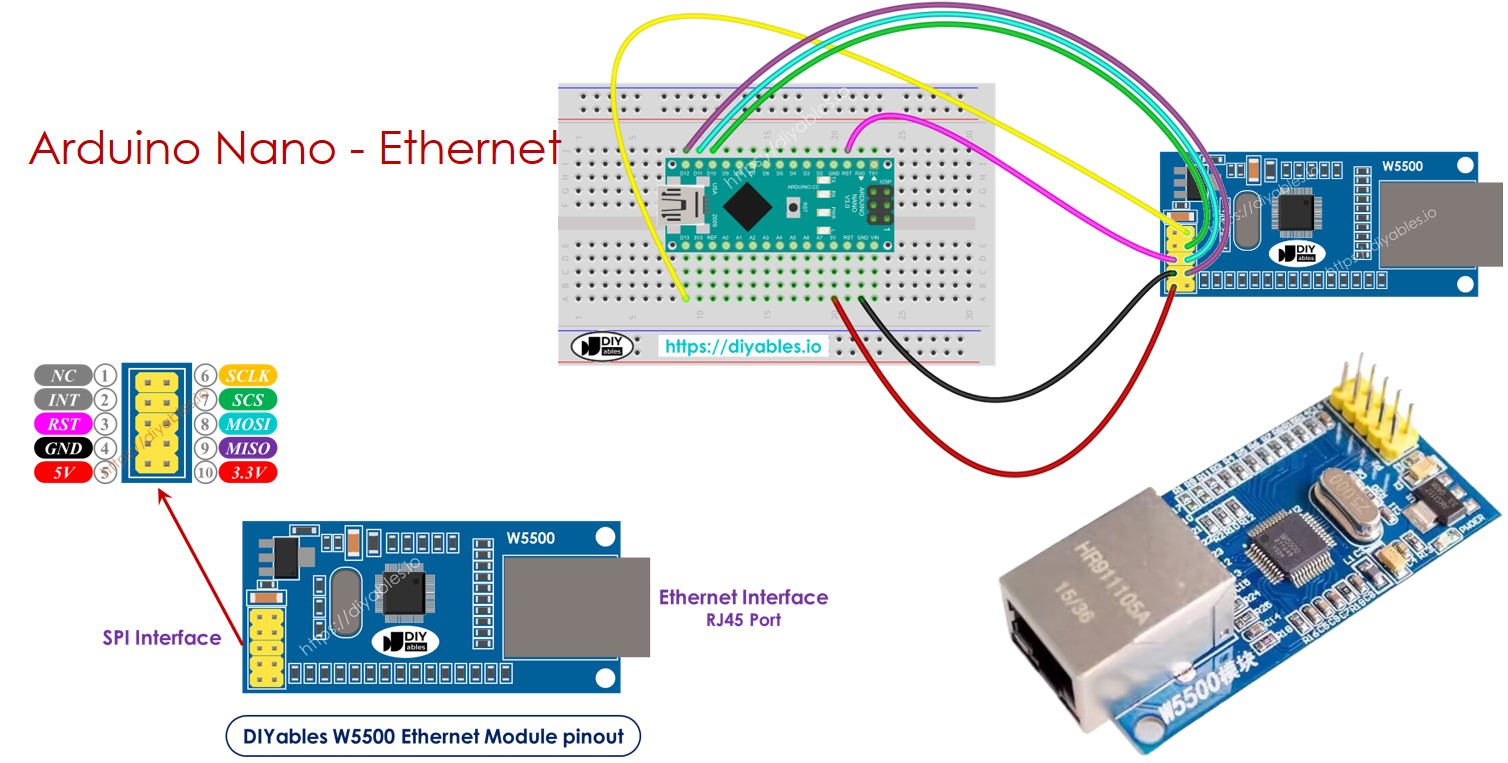
Hardware Preparation
1 | × | Arduino Nano | |
1 | × | USB A to Mini-B USB cable | |
1 | × | W5500 Ethernet Module | |
1 | × | Ethernet Cable | |
1 | × | Jumper Wires | |
1 | × | Breadboard | |
1 | × | (Recommended) Screw Terminal Expansion Board for Arduino Nano |
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Disclosure: Some of the links provided in this section are Amazon affiliate links. We may receive a commission for any purchases made through these links at no additional cost to you. We appreciate your support.
Overview of W5500 Ethernet module
The W5500 Ethernet module offers two kinds of connections:
- RJ45 interface: Use this to connect to a router or switch with an Ethernet cable.
- SPI interface: Use this for connecting to an Arduino Nano board. It includes 10 pins:
- NC pin: Leave this pin unconnected.
- INT pin: Leave this pin unconnected.
- RST pin: This is the reset pin. Connect it to the Arduino Nano's EN pin.
- GND pin: Connect this pin to the Arduino Nano's GND pin.
- 5V pin: Connect this pin to the Arduino Nano's 5V pin.
- 3.3V pin: Leave this pin unconnected.
- MISO pin: Connect this pin to the Arduino Nano's SPI MISO pin.
- MOSI pin: Connect this pin to the Arduino Nano's SPI MOSI pin.
- SCS pin: Connect this pin to the Arduino Nano's SPI CS pin.
- SCLK pin: Connect this pin to the Arduino Nano's SPI SCK pin.
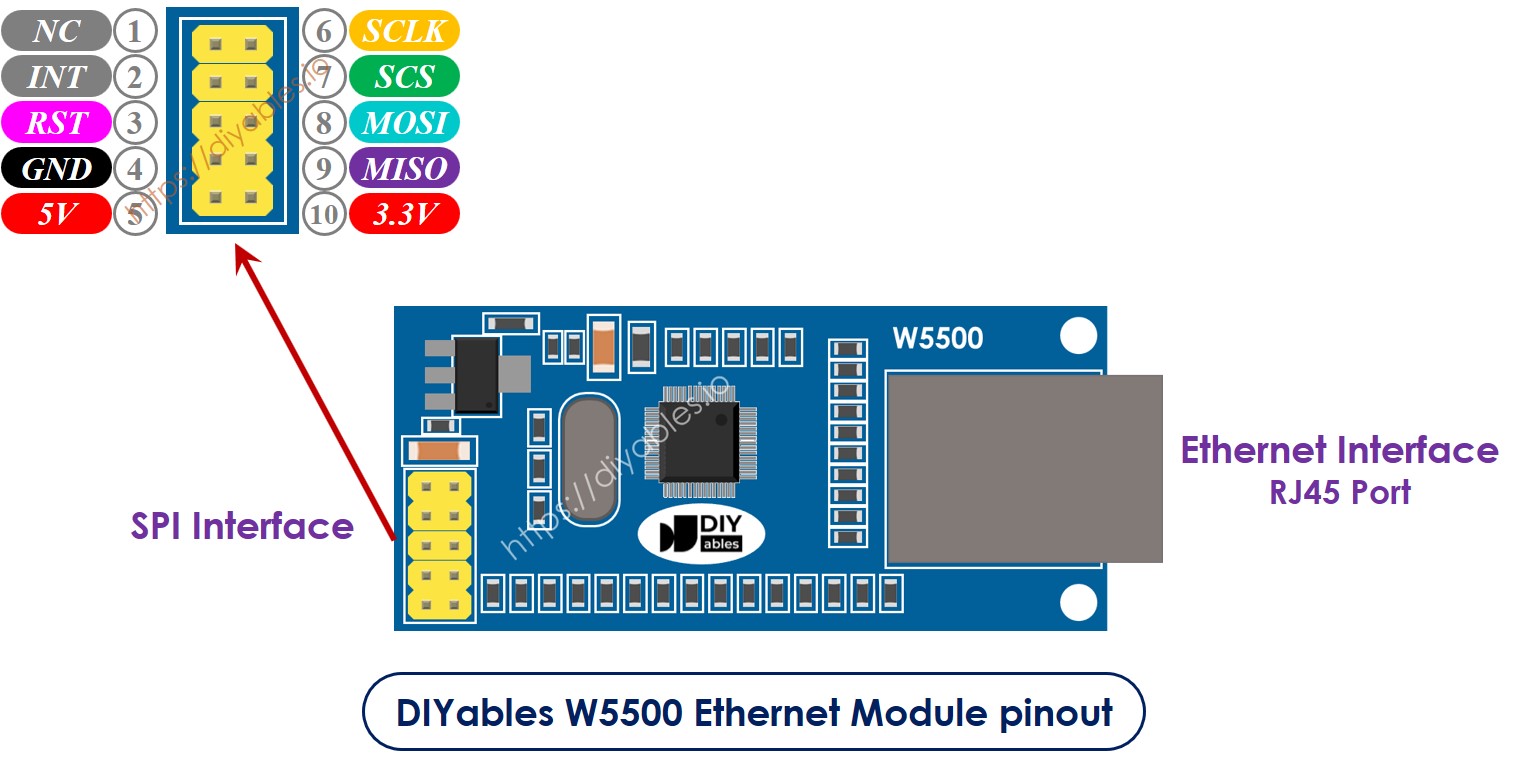
image source: diyables.io
Wiring Diagram between Arduino Nano and W5500 Ethernet module
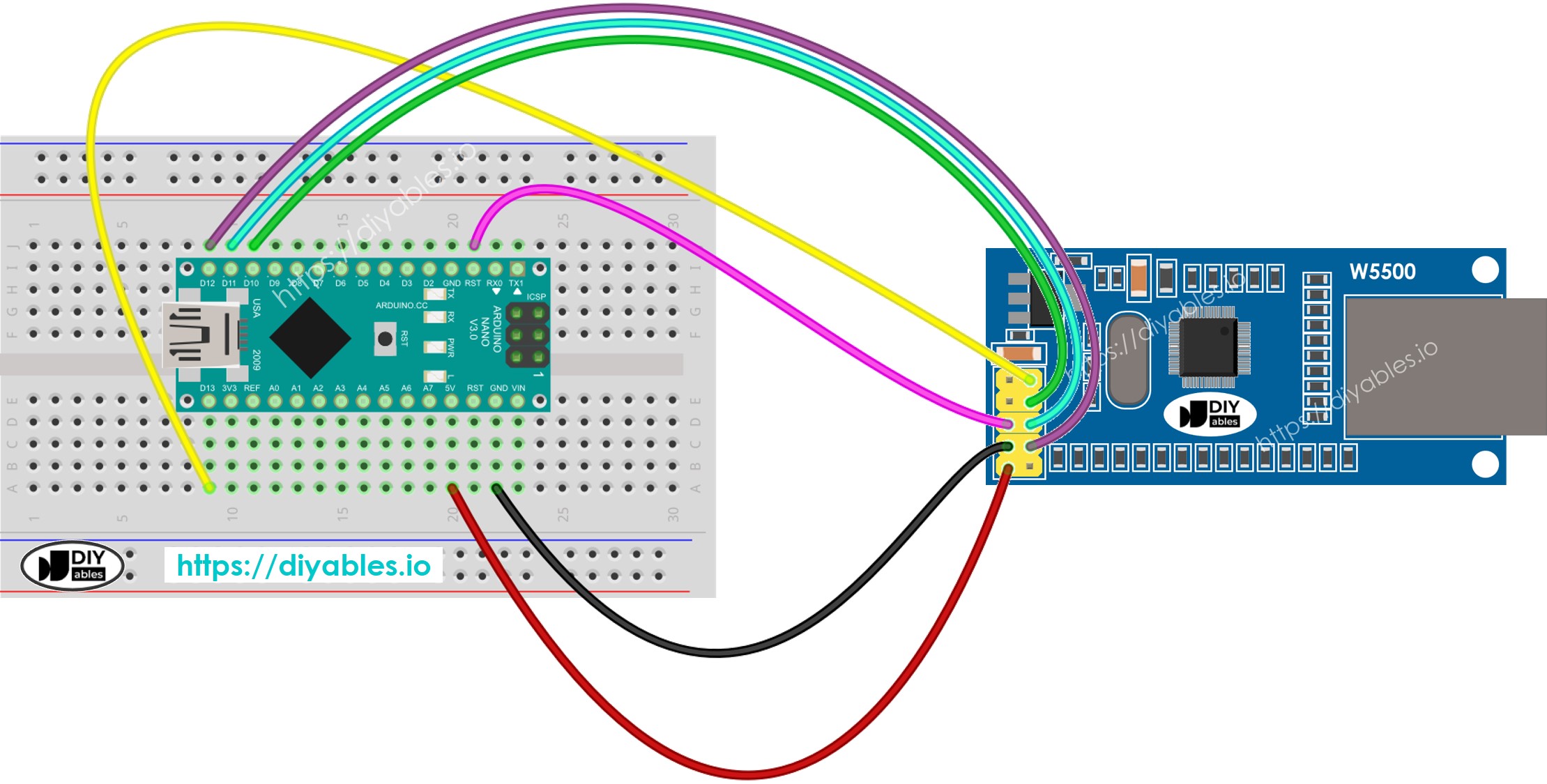
This image is created using Fritzing. Click to enlarge image
image source: diyables.io
Arduino Nano code for Ethernet Module - Making HTTP request via Ethernet
This code works as a web client. It sends HTTP requests to the web server found at http://example.com/.
/*
* This Arduino Nano code was developed by newbiely.com
*
* This Arduino Nano code is made available for public use without any restriction
*
* For comprehensive instructions and wiring diagrams, please visit:
* https://newbiely.com/tutorials/arduino-nano/arduino-nano-ethernet
*/
#include <SPI.h>
#include <Ethernet.h>
// replace the MAC address below by the MAC address printed on a sticker on the Arduino Shield 2
byte mac[] = { 0xDE, 0xAD, 0xBE, 0xEF, 0xFE, 0xEF };
EthernetClient client;
int HTTP_PORT = 80;
String HTTP_METHOD = "GET"; // or POST
char HOST_NAME[] = "example.com";
String PATH_NAME = "/";
void setup() {
Serial.begin(9600);
delay(1000);
Serial.println("Arduino Nano - Ethernet Tutorial");
// initialize the Ethernet shield using DHCP:
if (Ethernet.begin(mac) == 0) {
Serial.println("Failed to obtaining an IP address");
// check for Ethernet hardware present
if (Ethernet.hardwareStatus() == EthernetNoHardware)
Serial.println("Ethernet shield was not found");
// check for Ethernet cable
if (Ethernet.linkStatus() == LinkOFF)
Serial.println("Ethernet cable is not connected.");
while (true)
;
}
// connect to web server on port 80:
if (client.connect(HOST_NAME, HTTP_PORT)) {
// if connected:
Serial.println("Connected to server");
// make a HTTP request:
// send HTTP header
client.println(HTTP_METHOD + " " + PATH_NAME + " HTTP/1.1");
client.println("Host: " + String(HOST_NAME));
client.println("Connection: close");
client.println(); // end HTTP header
while (client.connected()) {
if (client.available()) {
// read an incoming byte from the server and print it to serial monitor:
char c = client.read();
Serial.print(c);
}
}
// the server's disconnected, stop the client:
client.stop();
Serial.println();
Serial.println("disconnected");
} else { // if not connected:
Serial.println("connection failed");
}
}
void loop() {
}
Detailed Instructions
- Connect the Ethernet module to the Arduino Nano as shown in the wiring diagram.
- Connect the Arduino Nano to your computer with a USB cable.
- Use an Ethernet cable to connect the Ethernet module to your router or switch.
- Open the Arduino IDE on your computer.
- Select the right Arduino Nano board and the COM port.
- Click to the Libraries icon on the left bar of the Arduino IDE.
- Search “Ethernet”, then find the Ethernet library by Various
- Click Install button to install Ethernet library.
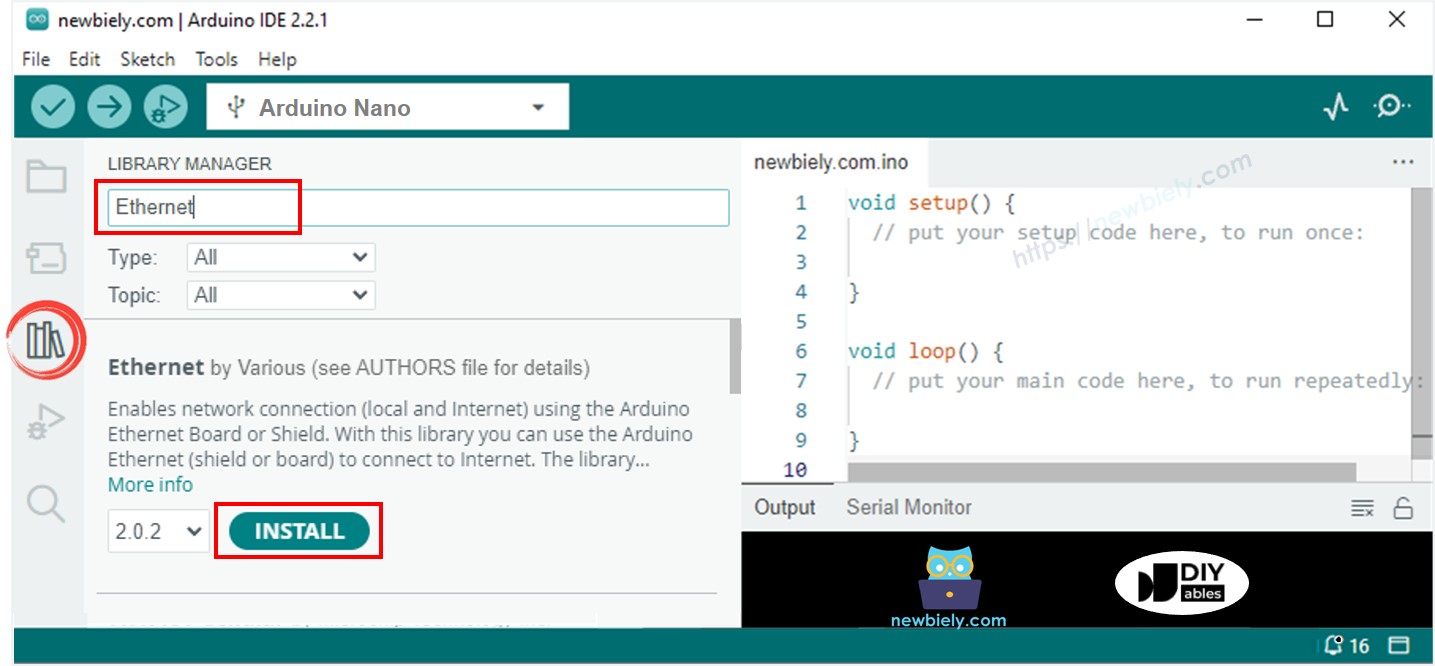
- Open the Serial Monitor in the Arduino IDE.
- Copy the code given and paste it into the Arduino IDE.
- Press the Upload button in the Arduino IDE to send the code to the ESP25.
- Look at the Serial Monitor to see the output, which should show the result as indicated.
COM6
Arduino Nano - Ethernet Tutorial
Connected to server
HTTP/1.1 200 OK
Accept-Ranges: bytes
Age: 208425
Cache-Control: max-age=604800
Content-Type: text/html; charset=UTF-8
Date: Fri, 12 Jul 2024 07:08:42 GMT
Etag: "3147526947"
Expires: Fri, 19 Jul 2024 07:08:42 GMT
Last-Modified: Thu, 17 Oct 2019 07:18:26 GMT
Server: ECAcc (lac/55B8)
Vary: Accept-Encoding
X-Cache: HIT
Content-Length: 1256
Connection: close
<!doctype html>
<html>
<head>
<title>Example Domain</title>
<meta charset="utf-8" />
<meta http-equiv="Content-type" content="text/html; charset=utf-8" />
<meta name="viewport" content="width=device-width, initial-scale=1" />
</head>
<body>
<div>
<h1>Example Domain</h1>
<p>This domain is for use in illustrative examples in documents. You may use this
domain in literature without prior coordination or asking for permission.</p>
<p><a href="https://www.iana.org/domains/example">More information...</a></p>
</div>
</body>
</html>
disconnected
Autoscroll
Clear output
9600 baud
Newline
※ NOTE THAT:
If another device on the same network uses the same MAC address, it may not function properly.
Arduino Nano code for Ethernet Module - Web Server
The code below turns the Arduino Nano into a web server. This server delivers a simple webpage to internet browsers.
/*
* This Arduino Nano code was developed by newbiely.com
*
* This Arduino Nano code is made available for public use without any restriction
*
* For comprehensive instructions and wiring diagrams, please visit:
* https://newbiely.com/tutorials/arduino-nano/arduino-nano-ethernet
*/
#include <SPI.h>
#include <Ethernet.h>
// replace the MAC address below by the MAC address printed on a sticker on the Arduino Shield 2
byte mac[] = { 0xDE, 0xAD, 0xBE, 0xEF, 0xFE, 0xEF };
EthernetServer server(80);
void setup() {
Serial.begin(9600);
delay(1000);
Serial.println("Arduino Nano - Ethernet Tutorial");
// initialize the Ethernet shield using DHCP:
if (Ethernet.begin(mac) == 0) {
Serial.println("Failed to obtaining an IP address");
// check for Ethernet hardware present
if (Ethernet.hardwareStatus() == EthernetNoHardware)
Serial.println("Ethernet shield was not found");
// check for Ethernet cable
if (Ethernet.linkStatus() == LinkOFF)
Serial.println("Ethernet cable is not connected.");
while (true)
;
}
server.begin();
Serial.print("Arduino Nano - Web Server IP Address: ");
Serial.println(Ethernet.localIP());
}
void loop() {
// listen for incoming clients
EthernetClient client = server.available();
if (client) {
Serial.println("new client");
// an HTTP request ends with a blank line
bool currentLineIsBlank = true;
while (client.connected()) {
if (client.available()) {
char c = client.read();
Serial.write(c);
// if you've gotten to the end of the line (received a newline
// character) and the line is blank, the HTTP request has ended,
// so you can send a reply
if (c == '\n' && currentLineIsBlank) {
// send a standard HTTP response header
client.println("HTTP/1.1 200 OK");
client.println("Content-Type: text/html");
client.println("Connection: close"); // the connection will be closed after completion of the response
client.println();
client.println("<!DOCTYPE HTML>");
client.println("<html>");
client.println("<body>");
client.println("<h1>Arduino Nano - Web Server with Ethernet</h1>");
client.println("</body>");
client.println("</html>");
break;
}
if (c == '\n') {
// you're starting a new line
currentLineIsBlank = true;
} else if (c != '\r') {
// you've gotten a character on the current line
currentLineIsBlank = false;
}
}
}
// give the web browser time to receive the data
delay(1);
// close the connection:
client.stop();
Serial.println("client disconnected");
}
}
Detailed Instructions
- Copy the above code and paste it into the Arduino IDE
- Click the Upload button in the Arduino IDE to send the code to your Arduino Nano
- Check the results on the Serial Monitor, it will display as follows:
COM6
Arduino Nano - Ethernet Tutorial
Arduino Nano - Web Server IP Address: 192.168.0.2
Autoscroll
Clear output
9600 baud
Newline
- Copy the IP address from above and paste it into your web browser's address bar. You will see a simple webpage displayed by the Arduino Nano.
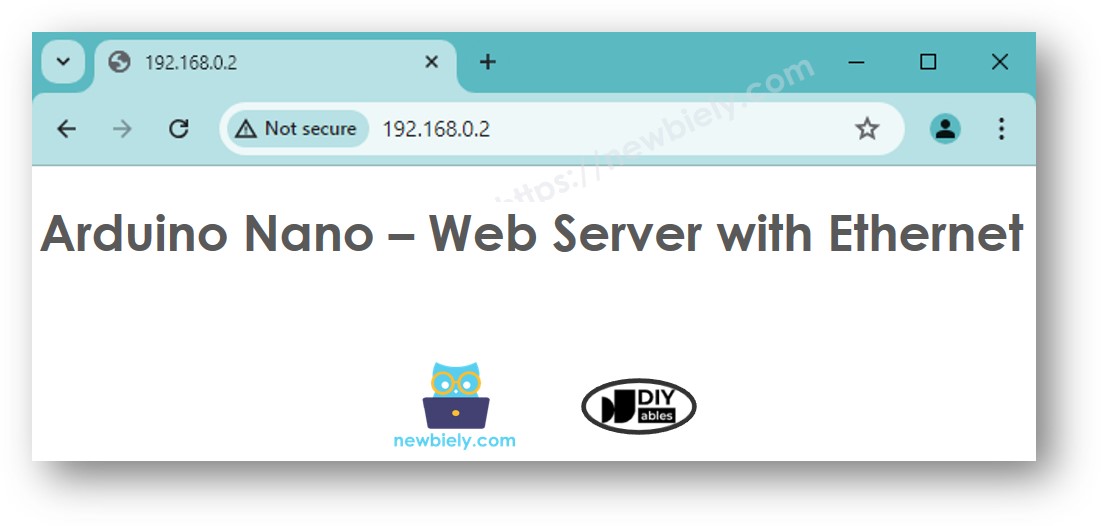