Arduino Nano - Water Leak Detector
In this guide, we will learn how to use the Arduino Nano and a water leak sensor to detect water leaks.
Hardware Preparation
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables.
Overview of Water Leak Detector
The water leak detector or sensor can find unwanted water early to prevent damage.
Water Leak Detector Pinout
The water leak detector includes two wires:
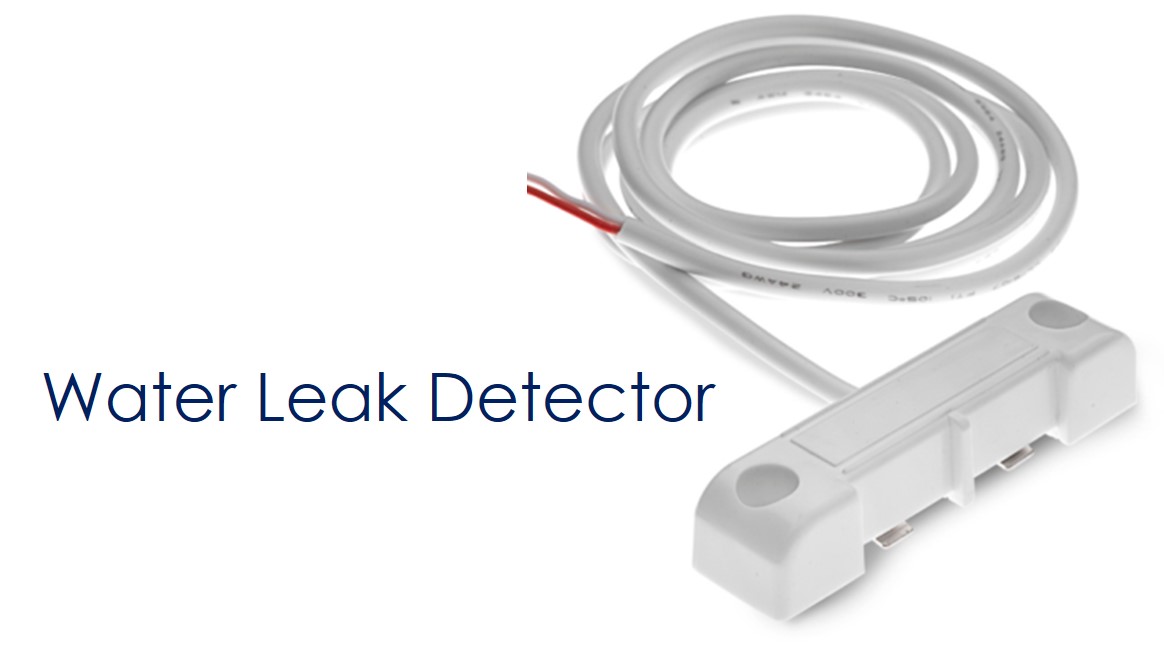
Just like a switch or button, we don’t have to identify the difference between the two wires in the water leak detector.
How the Water Leak Detector Works
When water is present, the circuit becomes complete. When there is no water, the circuit remains open.
To connect a water leak detector to an Arduino Nano, attach one wire to the GND and the other wire to a digital input pin set as a pull-up. When water is present, this pin on the Arduino Nano will read as LOW. If there is no water, it will read as HIGH.
※ NOTE THAT:
The water leak detector cannot sense pure water because it does not conduct electricity. To solve this, sprinkle some salt close to the sensor. The salt will mix with the water and allow the detector to sense it.
Wiring Diagram between Water Leak Detector and Arduino Nano
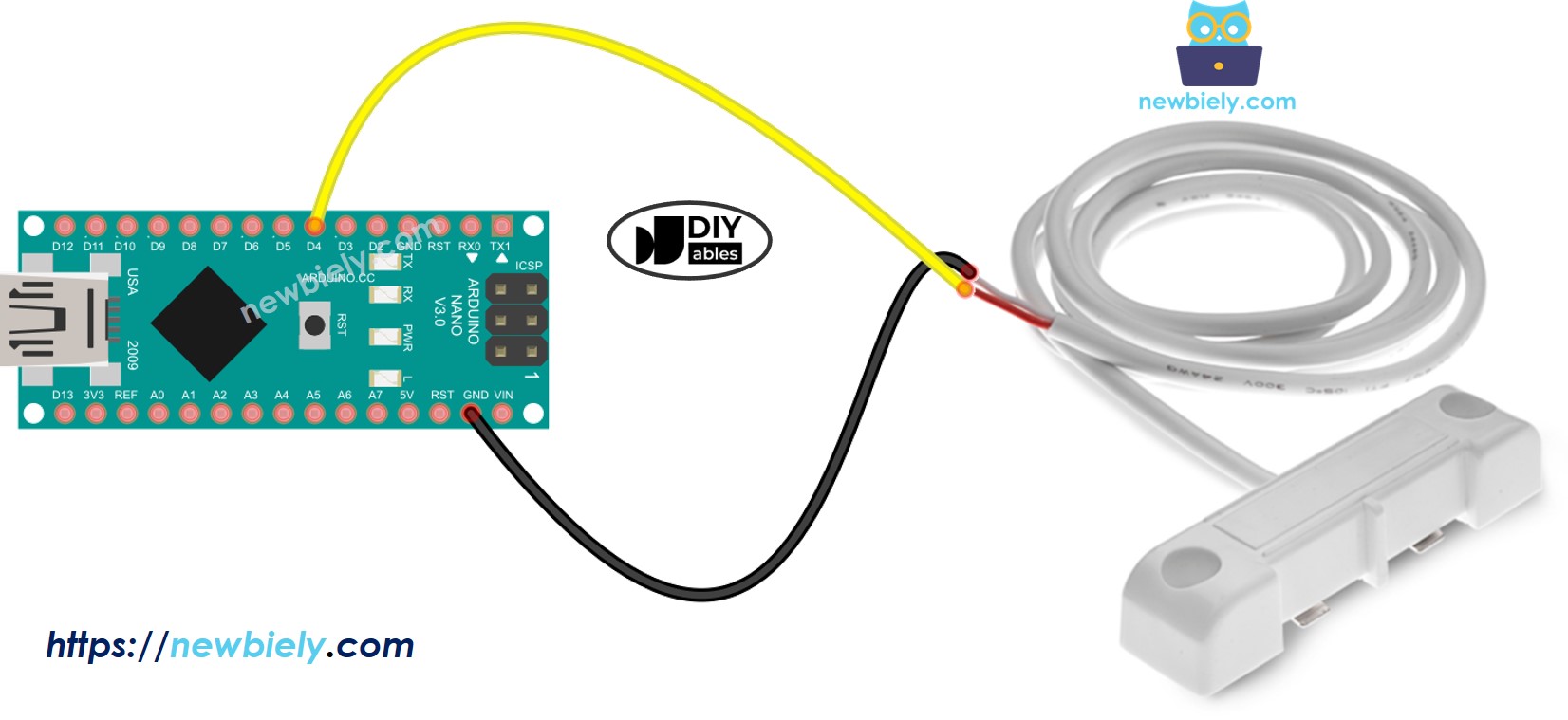
This image is created using Fritzing. Click to enlarge image
How To Program Arduino Nano to read value from Water Leak Detector
- Use the pinMode() function to set the Arduino Nano pin as a digital input. For example, use it for pin D4.
- Uses the digitalRead() function to examine the status of the Arduino Nano pin.
Arduino Nano Code - Detecting Water Leakage
Detailed Instructions
- Copy the code above and paste it into the Arduino IDE.
- To uoload the code to your Arduino Nano board, click the Upload button in the Arduino IDE.
- Pour water close to the water leak detector.
- Look at the results on the Serial Monitor. It will show like this:.