Arduino Nano - Keypad 1x4
In this tutorial, we will learn how to use a 1x4 keypad with an Arduino Nano. We will cover:
- How to link a 1x4 keypad with an Arduino Nano.
- How to write code for Arduino Nano to detect which keys are pressed on a 1x4 keypad.
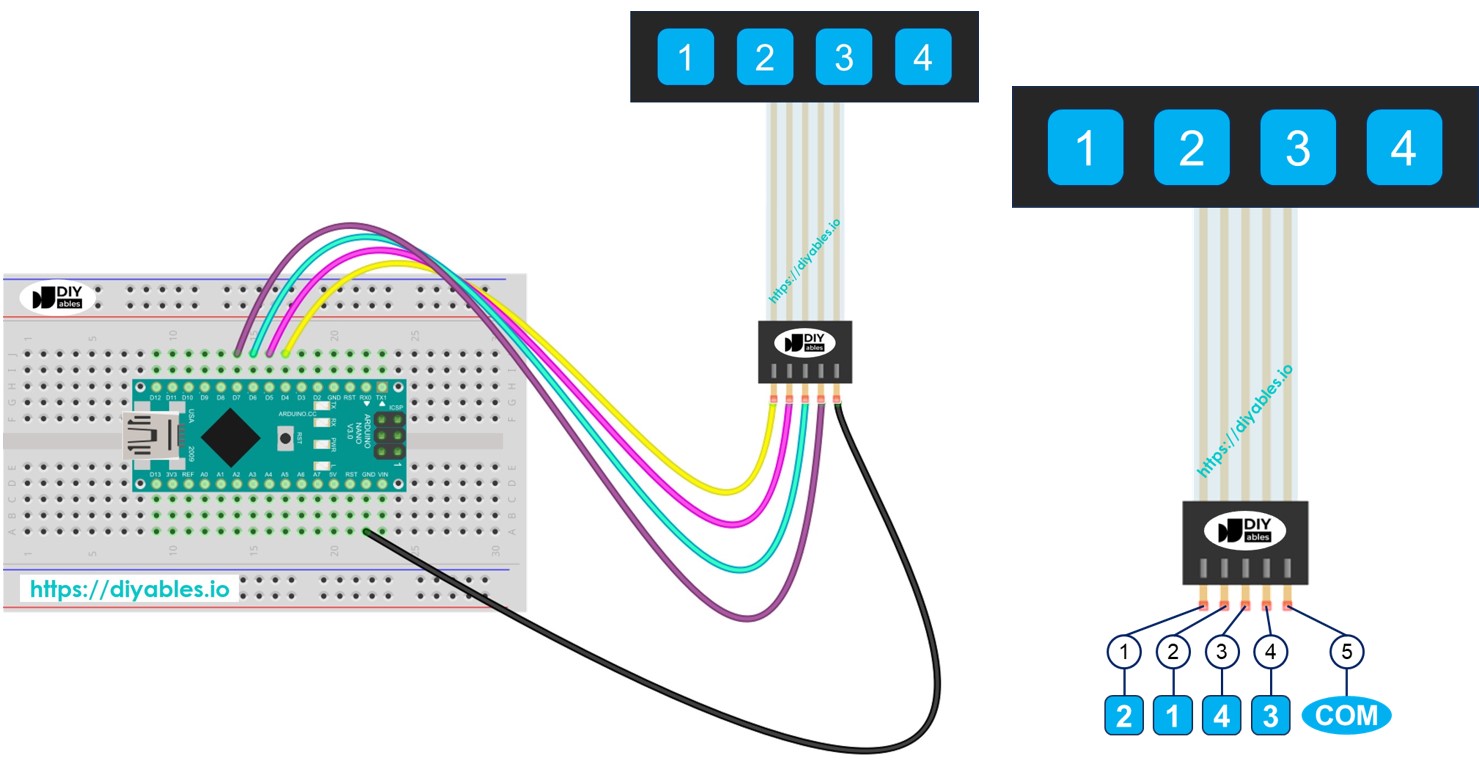
Hardware Preparation
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables .
Overview of Keypad 1x4
A 1x4 keypad has four buttons lined up in a row. It is often used to enter passwords, move through menus, or control devices.
Pinout
The 1x4 keypad has five pins. These pins are not arranged in the same order as the keys on the keypad.
- Pin 1: connects to key 2
- Pin 2: connects to key 1
- Pin 3: connects to key 4
- Pin 4: connects to key 3
- Pin 5: is a common pin connected to all keys
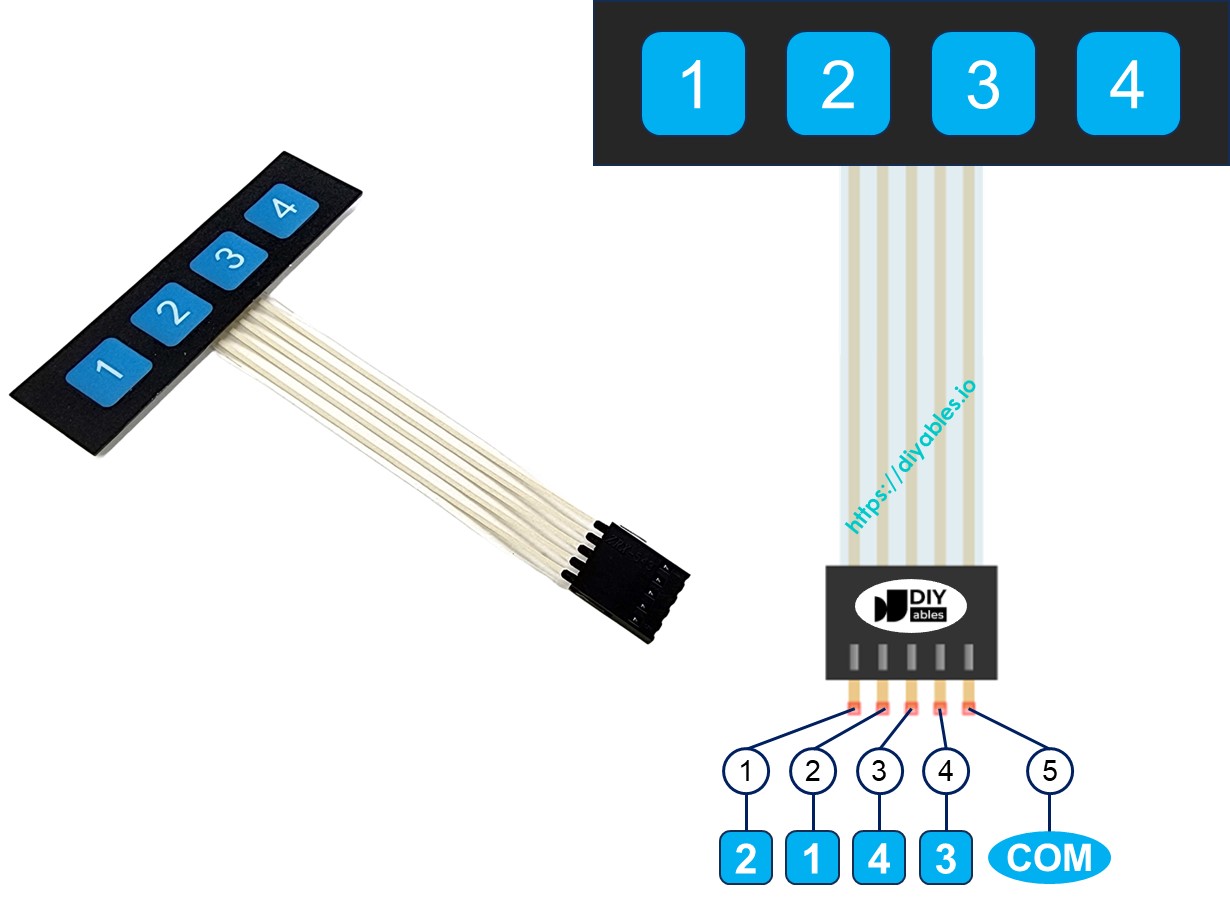
Wiring Diagram
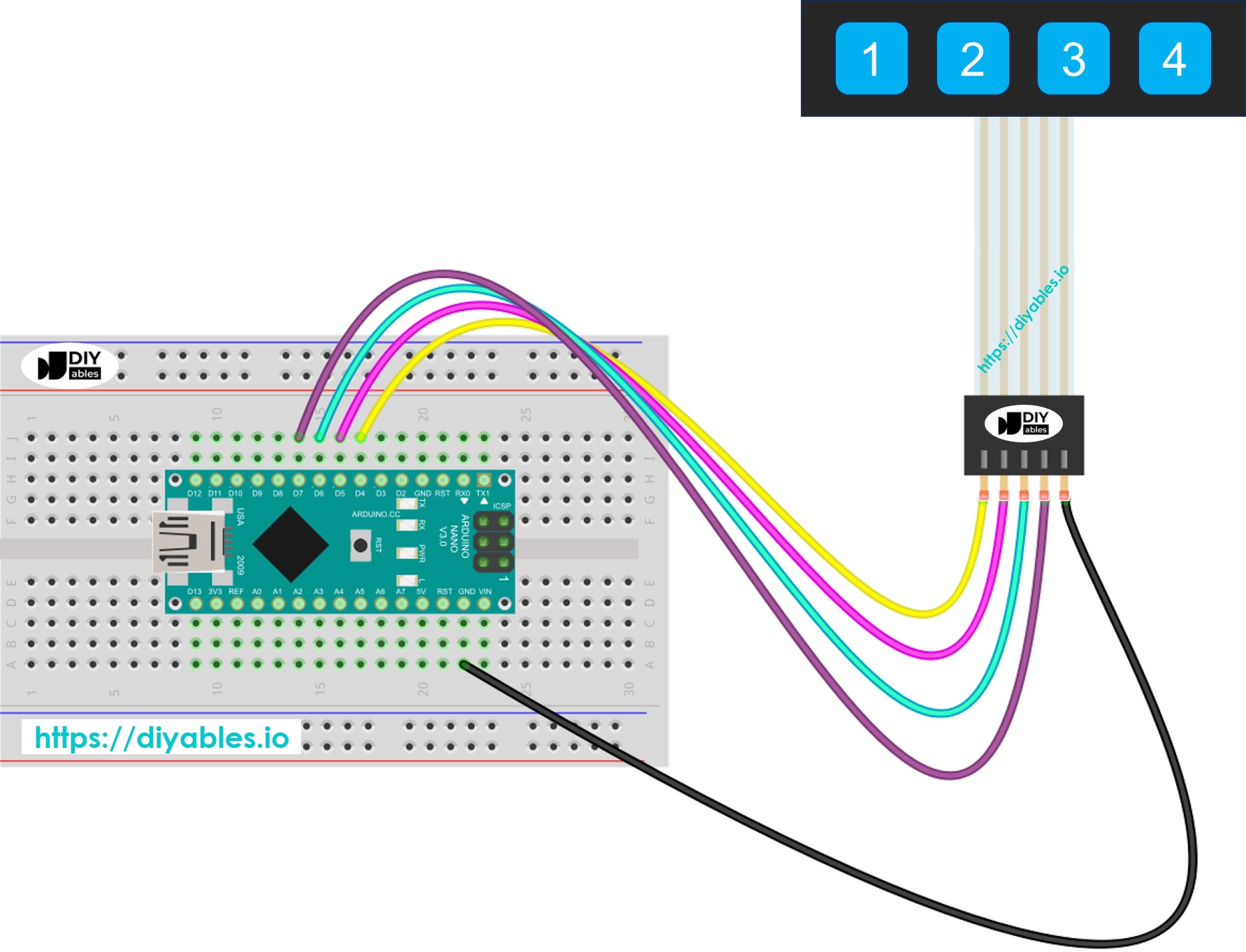
This image is created using Fritzing. Click to enlarge image
See The best way to supply power to the Arduino Nano and other components.
Arduino Nano Code
Each key on the 1x4 keypad works as a button. This lets us use the digitalRead() function to check if a key is pressed. However, keys can sometimes bounce, meaning a single press appears as several presses. To fix this, we have to debounce each key. Debouncing four keys at once can be tough without stopping other code from running. Luckily, the ezButton library helps make this easier.
Detailed Instructions
- Connect the Arduino Nano to the 1x4 keypad.
- Connect the Arduino Nano to the computer using a USB cable.
- Open the Arduino IDE and choose the correct board and port.
- Click on the Libraries icon on the left side of the Arduino IDE.
- Type ezButton in the search box and locate the button library from Arduino NanoGetStarted.com.
- Press the Install button to add the ezButton library.
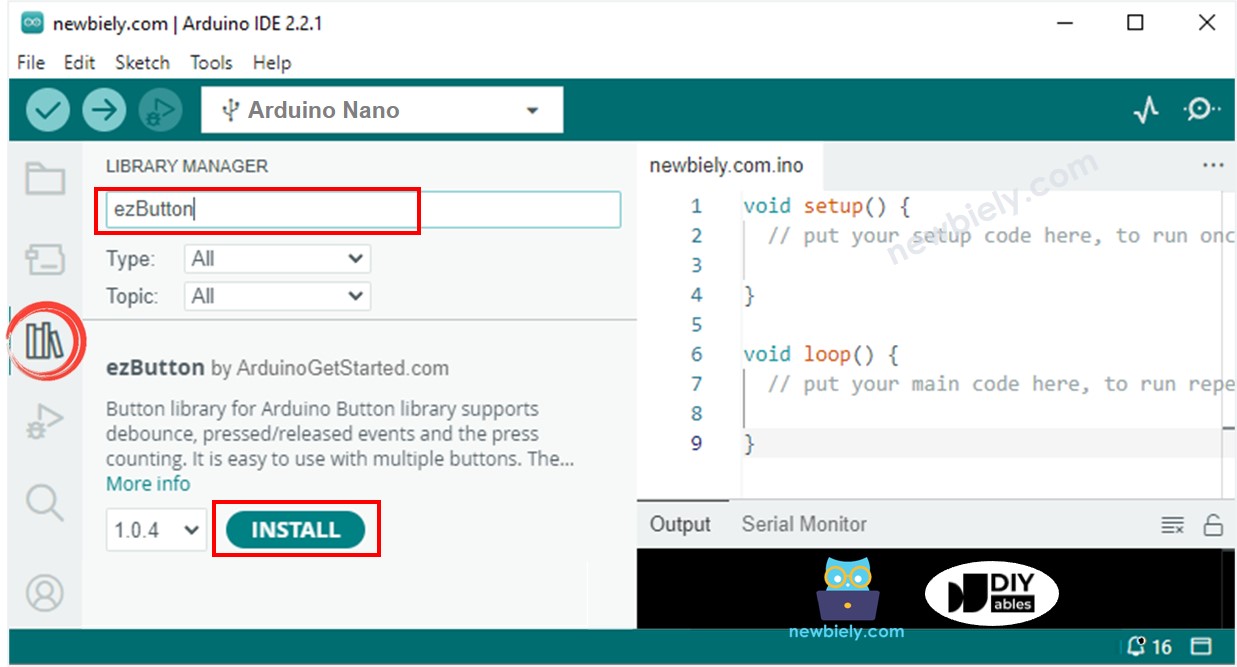
- Copy the code and open it in Arduino IDE
- Click the Upload button in Arduino IDE to send the code to Arduino Nano
- Open the Serial Monitor
- Press each key on the 1x4 keypad individually
- Check the results in the Serial Monitor