Arduino Nano - Web Server
In this guide, we’ll show you how to program an Arduino Nano board with an Ethernet module to act as a web server. You’ll be able to view data and control the Arduino Nano through web pages accessible from your computer or smartphone. We'll start with simple tasks and move to more advanced ones, as outlined below:
- Arduino Nano Web Server - Hello World!
- Arduino Nano Web Server - Monitoring sensor values via a webpage.
- Arduino Nano Web Server - Auto-Updating Values on the Webpage.
- Arduino Nano Web Server - Separating HTML, CSS, and JavaScript from Arduino code.
- Arduino Nano Web Server - Controlling the Arduino Nano through a webpage.
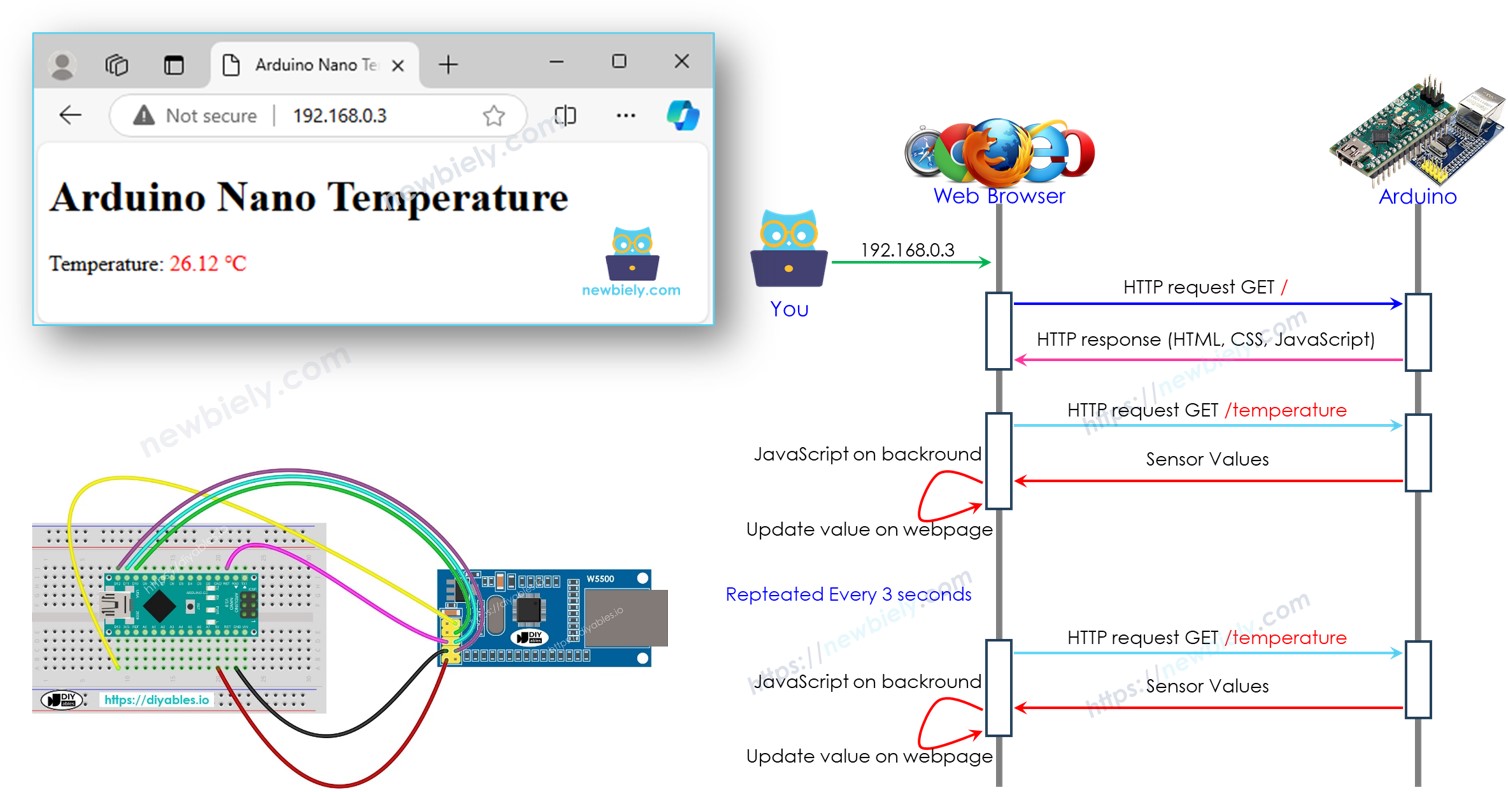
Hardware Preparation
Or you can buy the following kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables .
Wiring Diagram between Arduino Nano and W5500 Ethernet module
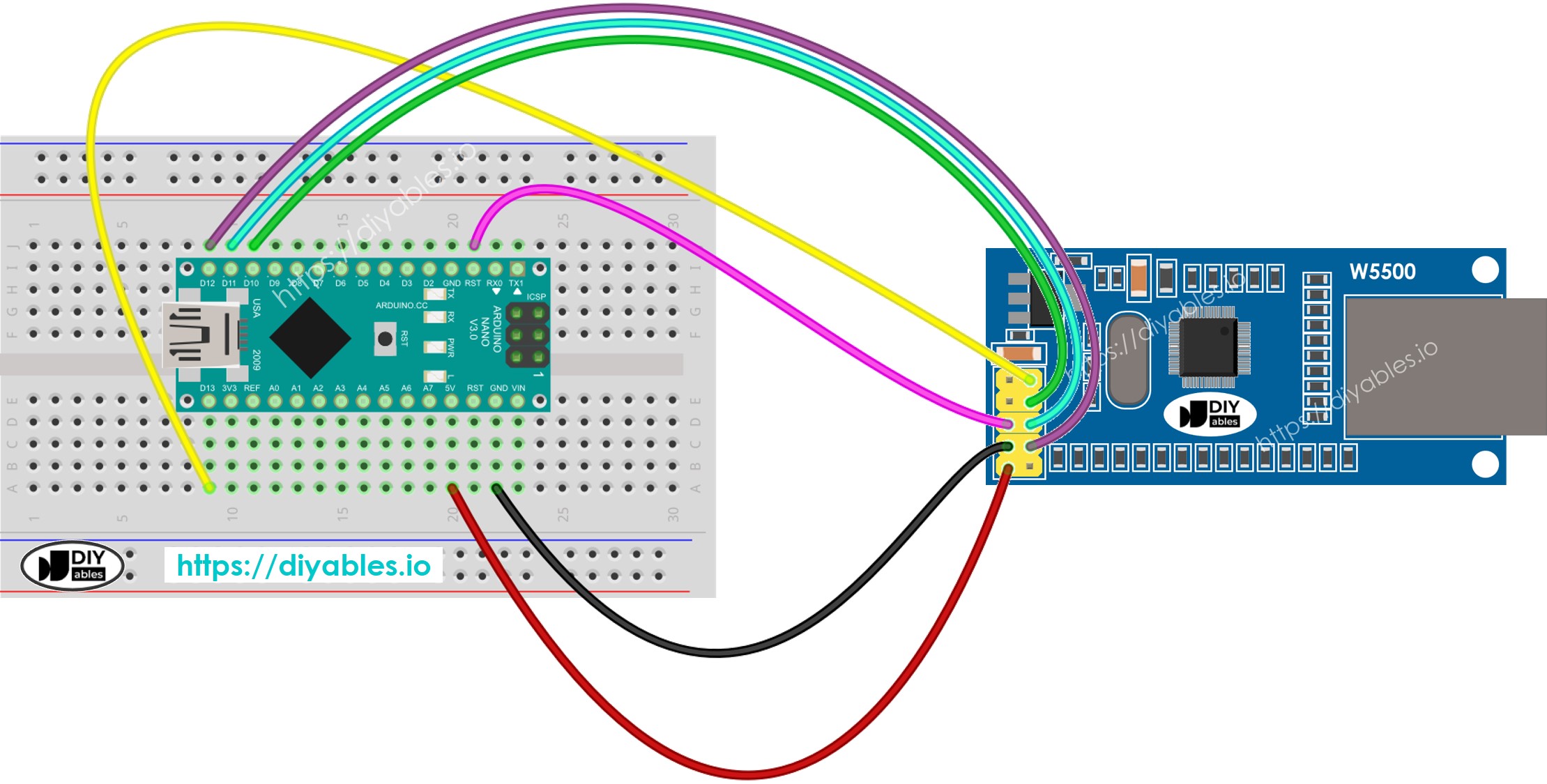
This image is created using Fritzing. Click to enlarge image
See The best way to supply power to the Arduino Nano and other components.
Arduino Nano Web Server - Hello World
This is relatively simple. The Arduino Nano code does the following tasks:
- Creating a web server that listens for HTTP requests from a web browser.
- Upon receiving a request from a web browser, the Arduino Nano responds with the following information:
- HTTP header
- HTTP body: includes "Hello World!".
- Connect the Arduino Nano to the Ethernet module according to the above wiring diagram.
- Connect an Ethernet cable to the Ethernet module.
- Connect the Arduino Nano to a PC using a USB cable.
- Launch the Arduino IDE on your PC.
- Select Arduino Nano board and corresponding COM port
- Click to the Libraries icon on the left bar of the Arduino IDE.
- Search “Ethernet”, then find the Ethernet library by Various
- Click Install button to install Ethernet library.
- Copy the above code and open with Arduino IDE
- Click Upload button on Arduino IDE to upload code to Arduino Nano
- Open the Serial Monitor
- Check out the result on Serial Monitor.
- Note the IP address displayed and enter it into the address bar of a web browser on your smartphone or PC.
- You will then see the following output on the Serial Monitor.
- Once you access the web browser using the IP address, you’ll see a simple web page displaying "Hello World!". The page will look like this:
Below is the Arduino Nano code that performs the above tasks:
Detailed Instructions
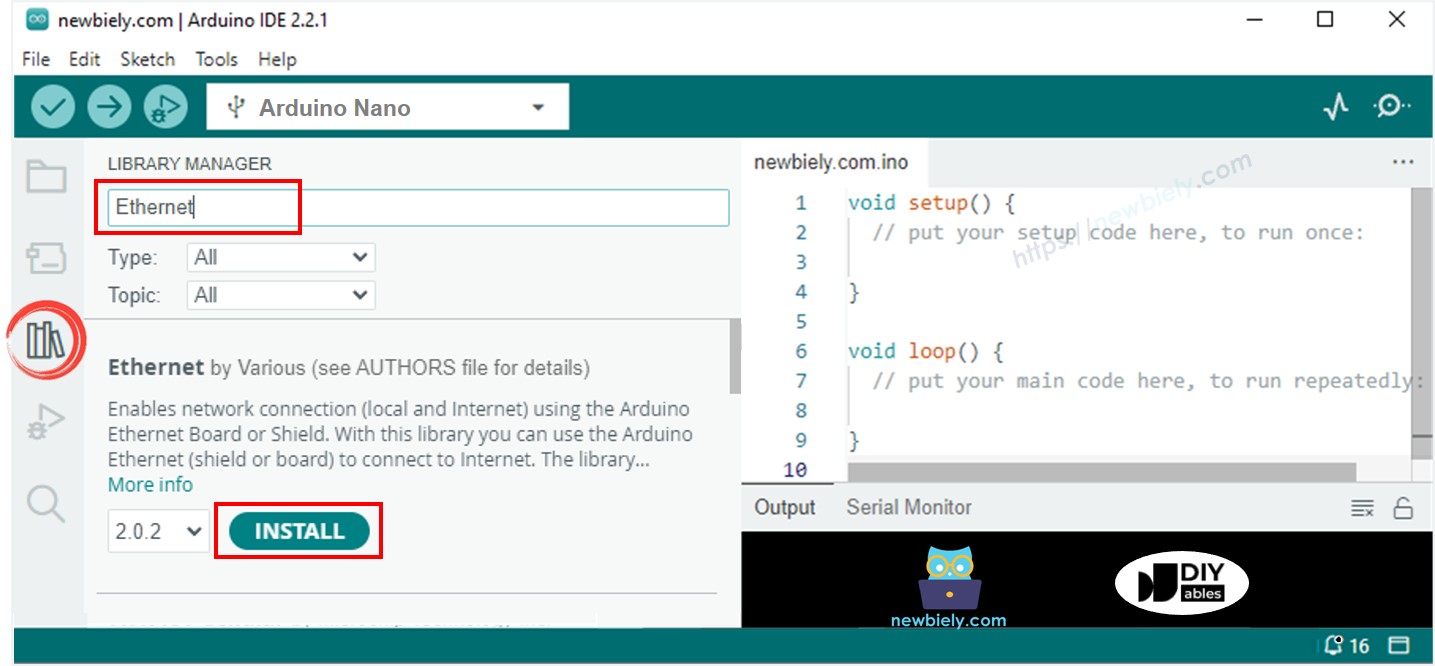
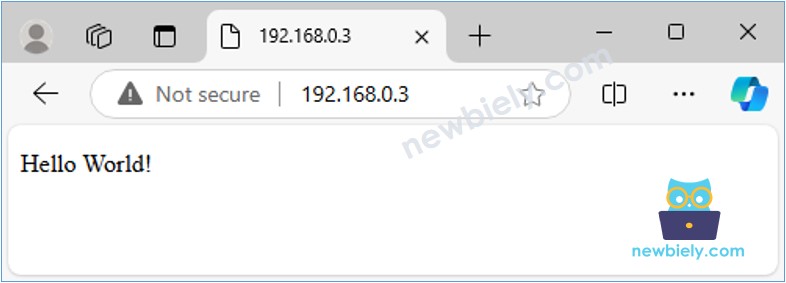
Arduino Nano Web Server - Monitoring sensor values via a webpage.
The below Arduino Nano code does the following tasks:
- Creating a web server that listens for HTTP requests from a web browser.
- Upon receiving a request from a web browser, the Arduino Nano responds with the following information:
- HTTP header
- HTTP body: This includes HTML, CSS content and the value read from the sensor.
- Copy the code above and open it in the Arduino IDE.
- Click the Upload button in the Arduino IDE to upload the code to the Arduino Nano.
- Reload the previous web page (Ctrl + F5), and you’ll see it as shown below:
Detailed Instructions
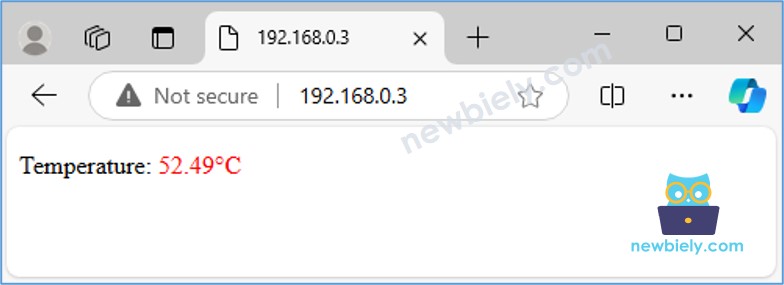
With the provided code, to update the temperature, you need to reload the page in the web browser. In the next section, Let's learn how to make the webpage automatically update the temperature value in the background without reloading.
Arduino Nano Web Server - Auto-Updating Values on the Webpage
How Auto-Updating Works
- The user enters the Arduino Nano's IP address in the web browser.
- The web browser sends an HTTP request to the Arduino Nano's home page (/).
- The Arduino responds with HTML, CSS, and JavaScript.
- The web browser displays the webpage using the received HTML and CSS.
- Every 3 seconds, the web browser runs the JavaScript code, which makes an HTTP request to the /temperature endpoint.
- The Arduino reads the sensor value and sends it back as a response.
- The web browser receives the sensor value and updates the webpage accordingly.
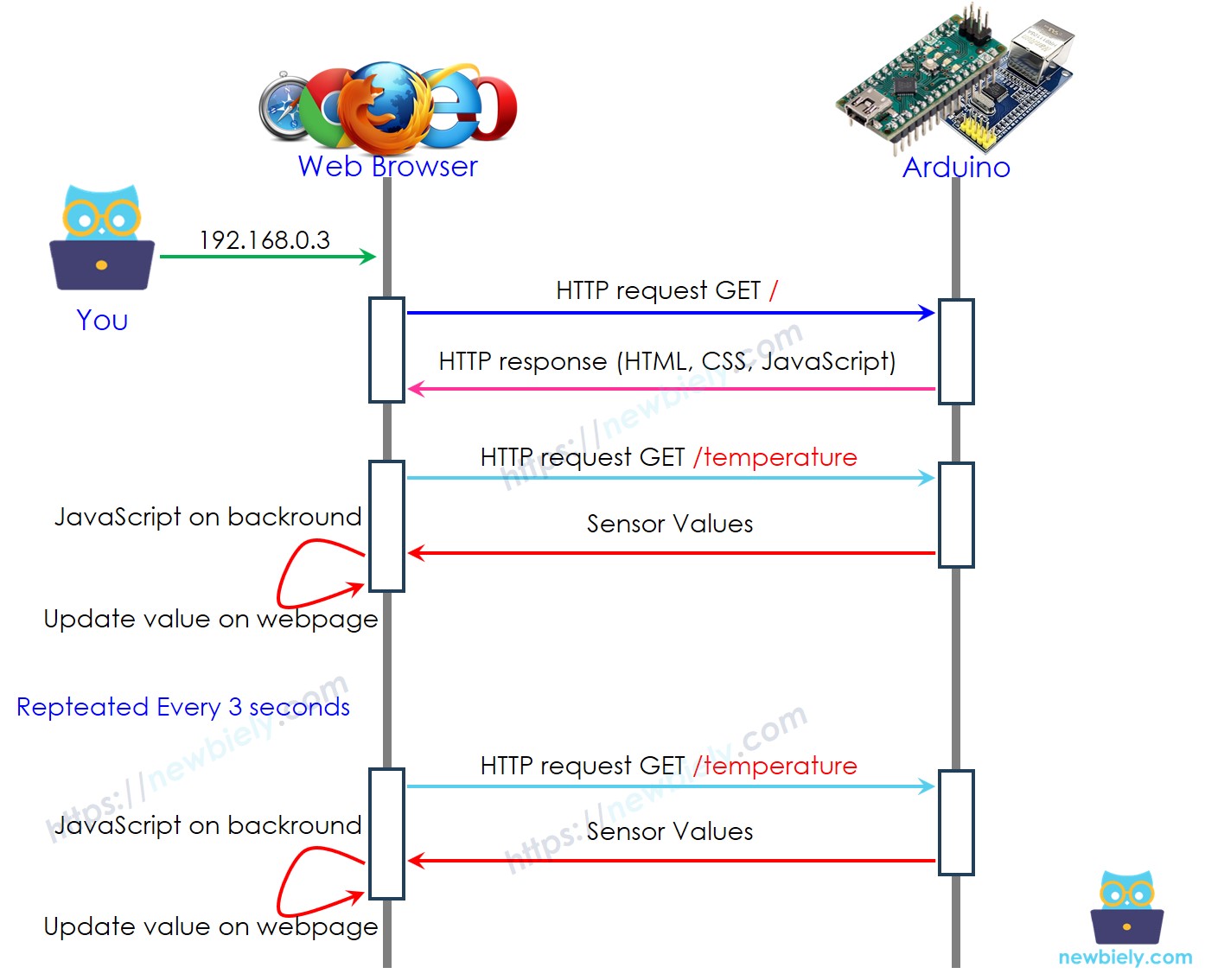
Below is the Arduino Nano code that performs the above tasks:
Detailed Instructions
- Copy the code above and open it in the Arduino IDE.
- Click the Upload button in the Arduino IDE to upload the code to the Arduino Nano.
- Reload the previous web page (Ctrl + F5), and you’ll see it as shown below:
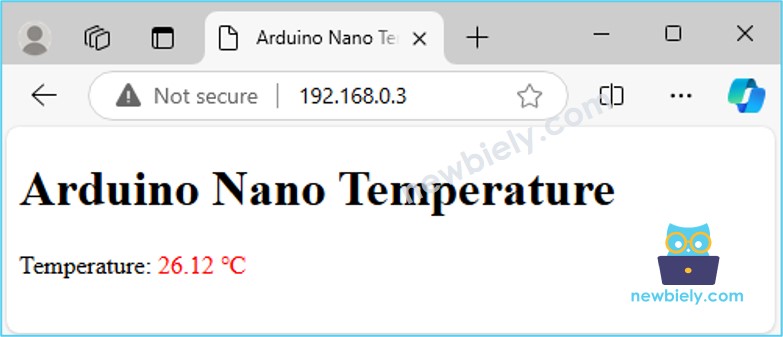
You will see that, the value is automatically updated every 3 seconds.
Separating HTML content into another file on Arduino IDE
If you want to create a simple web page with minimal content, you can embed the HTML directly in the Arduino Nano code, as previously explained.
However, for more complex and feature-rich web pages, embedding all the HTML, CSS, and JavaScript directly in the Arduino Nano code can become cumbersome. In such cases, it's better to use a different approach to manage the code:
- The Arduino Nano code will still be stored in a .ino file as usual.
- The web page content (HTML, CSS, JavaScript) will be placed in a separate .h file. This separation makes it easier to manage and update the web page without cluttering the Arduino code.
Detailed Instructions
- Open the Arduino IDE and create a new sketch. Give it a name, for example, newbiely.com.ino.
- Copy the code provided below and paste it to the created file.
- Create the index.h file On Arduino IDE by:
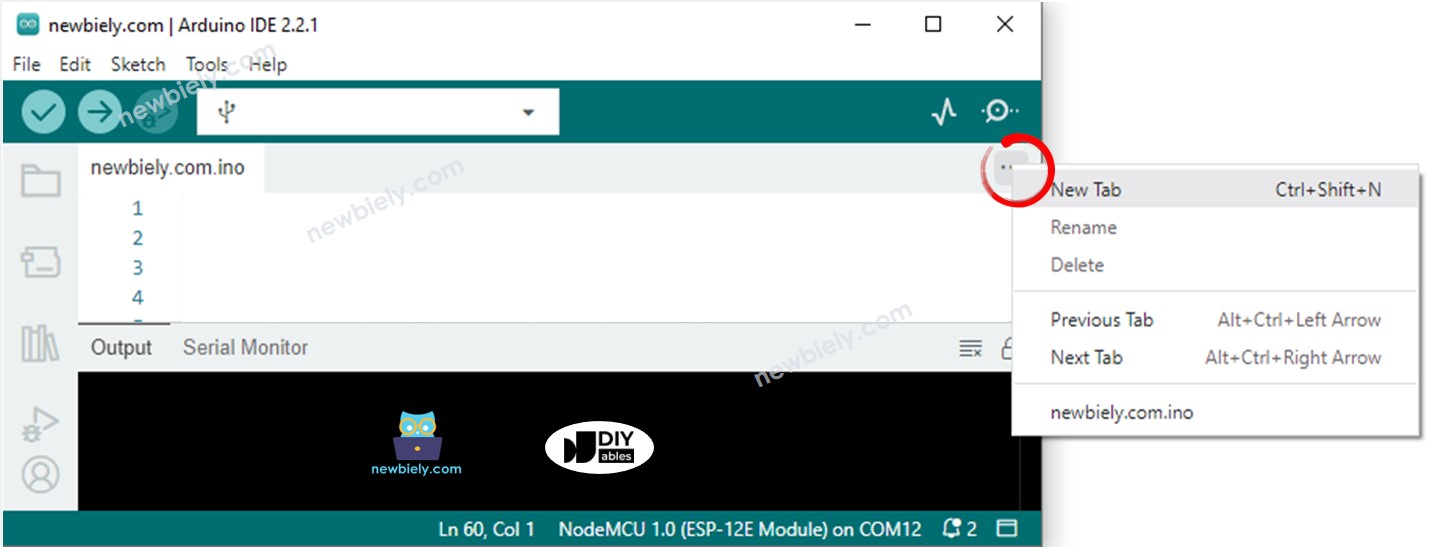
- Either click on the button just below the serial monitor icon and choose New Tab, or use Ctrl+Shift+N keys.
- Give file's name index.h and click OK button
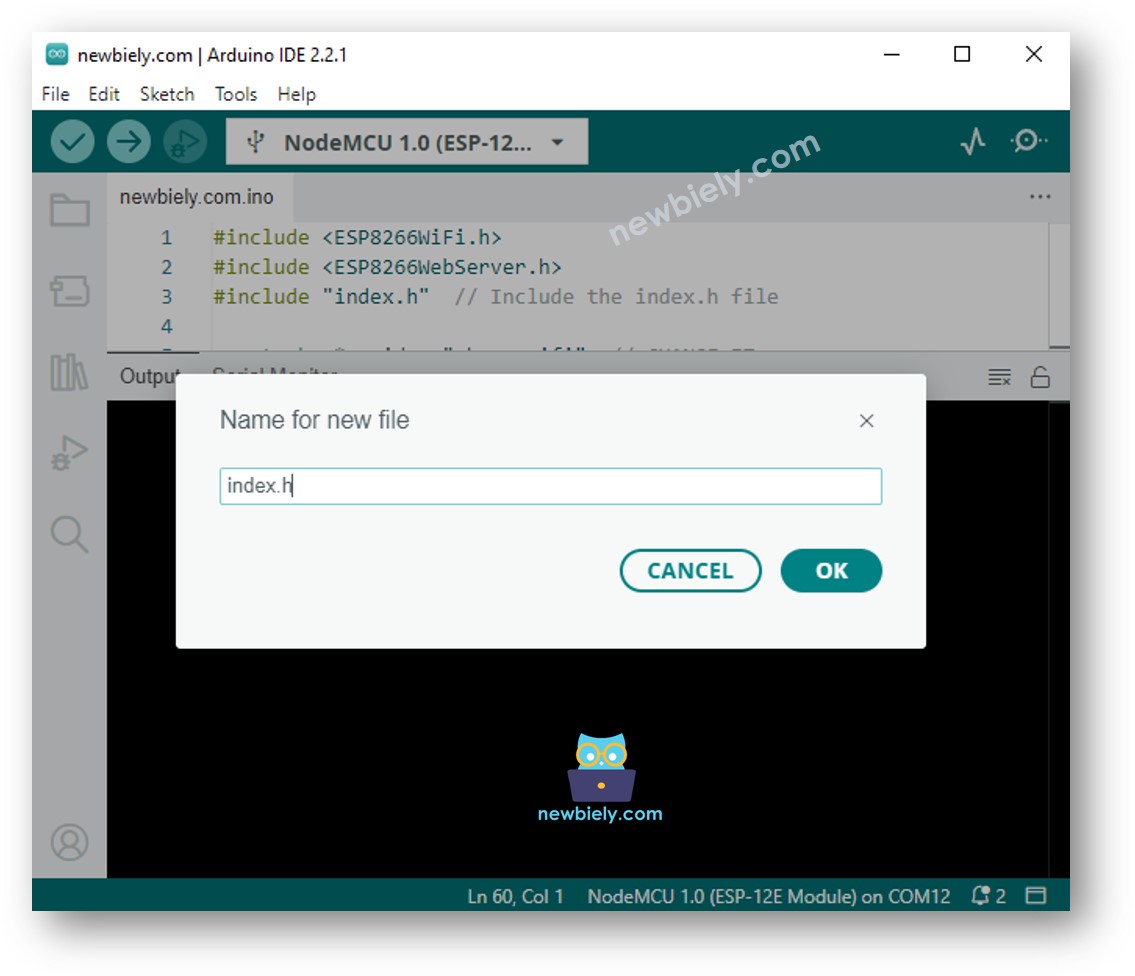
- Copy the below code and paste it to the index.h.
- Now you have the code in two files: newbiely.com.ino and index.h
- Click Upload button on Arduino IDE to upload code to Arduino Nano
- Reload the web page (using Ctrl + F5), and it will appear the same as before
※ NOTE THAT:
- If you update the HTML content in the index.h file without changing anything in the newbiely.com.ino file, the Arduino IDE won’t refresh or include the updated HTML content when you compile and upload the code to the Arduino Nano.
- To ensure the Arduino IDE updates the HTML content, you need to make a small change in the newbiely.com.ino file, such as adding a blank line or a comment. This forces the IDE to detect changes, ensuring the updated HTML content is included in the upload.
Controlling the Arduino Nano via Web
Controlling something connected to Arduino Nano is a bit more challenging than just reading a value. That's because Arduino Nano has to understand the request it receives from the web browser to know what action to take.
For a more comprehensive and detailed example, I recommend checking out the tutorials listed below: