Arduino Nano - DRV8825 Stepper Motor Driver
In this guide, we will explore the DRV8825 Stepper Motor Driver and learn how to operate it with Arduino Nano to manage the stepper motor. We will cover:
- The DRV8825 stepper motor controller module
- Operating the DRV8825 stepper motor controller module
- Connecting the DRV8825 stepper motor controller to Arduino Nano and stepper motor
- Programming Arduino Nano to manage stepper motor with the DRV8825 module
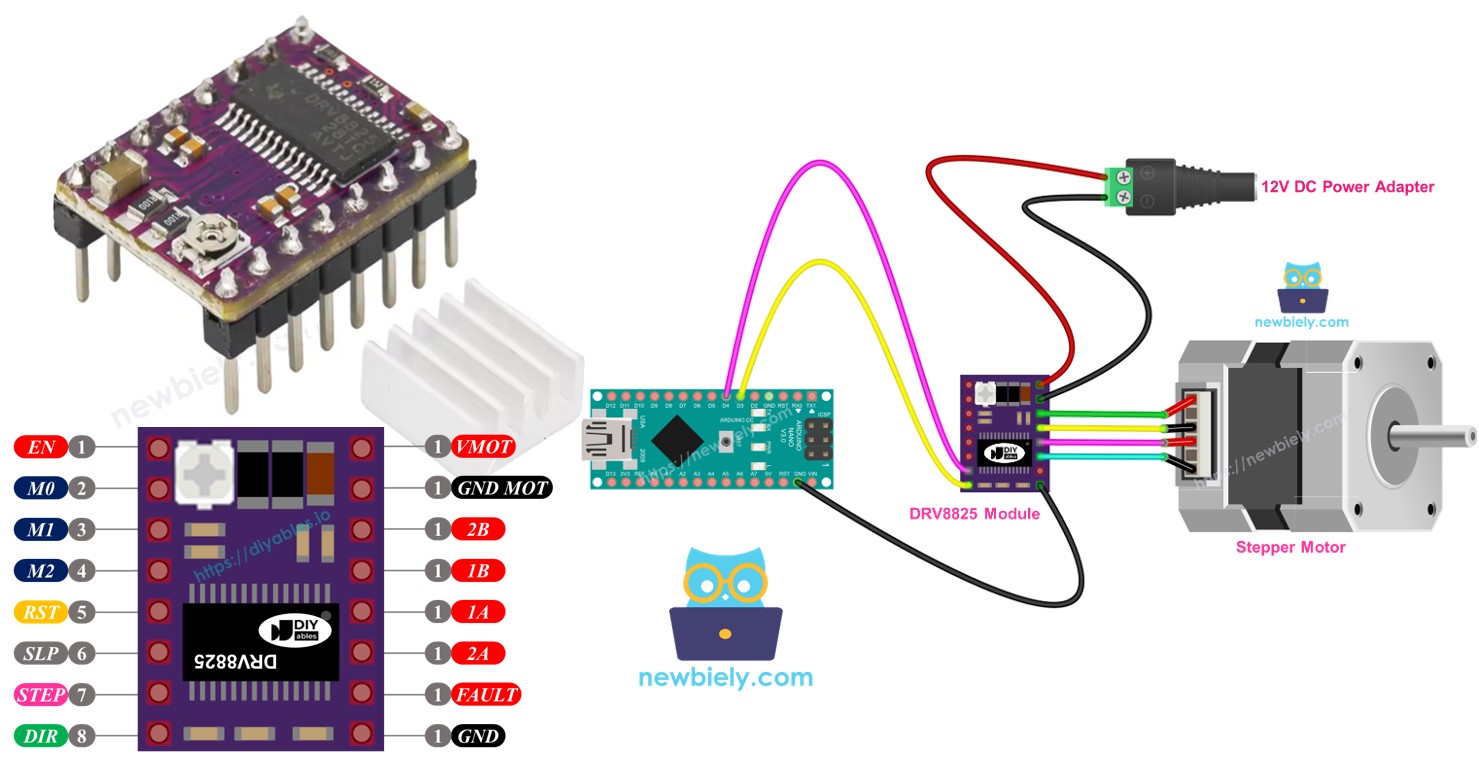
Hardware Preparation
Or you can buy the following kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables .
Overview of DRV8825 Stepper Motor Driver
The DRV8825 is a popular module for managing bipolar stepper motors often used in CNC machines, 3D printers, and robots. It offers an adjustable current control, temperature safety features, and multiple microstepping options like full-step, 1/2, 1/4, 1/8, 1/16, and 1/32. This module supports up to 2.2A per coil with adequate cooling and works within a voltage range from 8.2V to 45V, accommodating different stepper motors.
To understand stepper motor basics such as full-step, microstepping, unipolar stepper, and bipolar stepper, check out the Arduino Nano - Stepper Motor guide.
It's amazing that managing the speed and direction of a simple stepper motor like the NEMA 17 only needs two Arduino Nano pins.
DRV8825 Stepper Motor Driver Pinout
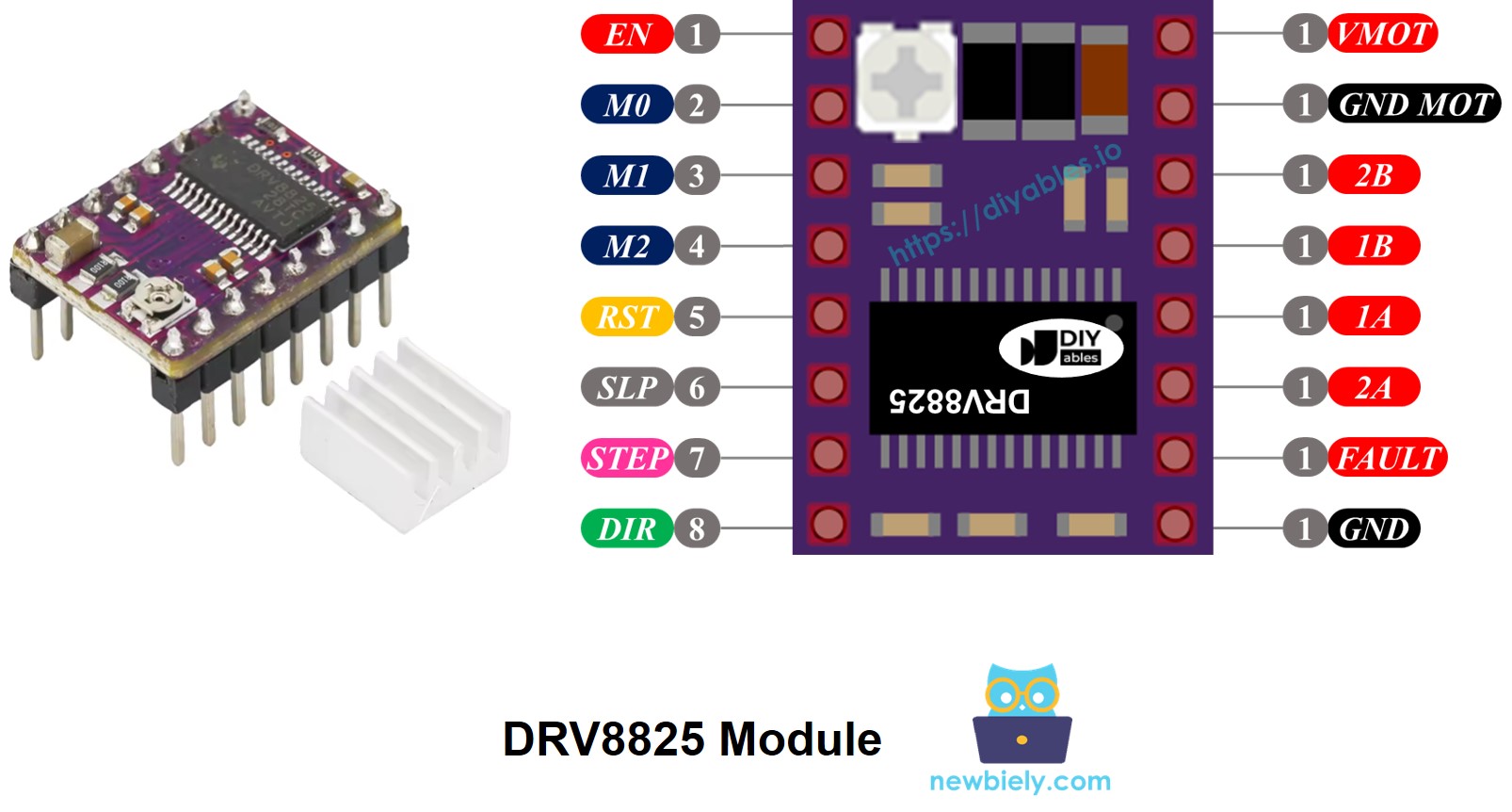
The DRV8825 Stepper motor driver has 16 pins. Here is a common layout for the DRV8825 stepper motor driver board. Keep in mind that some versions of the board might name the pins in a slightly different way, but their functions are the same.
Pin Name | Description |
---|---|
VMOT | Motor power supply (8.2 V to 45 V). This powers the stepper motor. |
GND (for Motor) | Ground reference for the motor power supply. Connect this pin to the GND of the motor power supply |
2B, 2A | Outputs to Coil B of the stepper motor. |
1A, 1B | Outputs to Coil A of the stepper motor. |
FAULT | Fault Detection Pin. This is an output pin that drives LOW whenever the H-bridge FETs are disabled as the result of over-current protection or thermal shutdown. |
GND (for Logic) | Ground reference for the logic signals. Connect this pin to the GND of Arduino Nano |
ENABLE | Active-Low pin to enable/disable the motor outputs. LOW = Enabled, HIGH = Disabled. |
M1, M2, M3 | Microstepping resolution selector pins (see table below). |
RESET | Active-Low reset pin - pulling this pin LOW resets the driver. |
SLEEP | Active-Low sleep pin - pulling this pin LOW puts the driver into low-power sleep mode. |
STEP | Step input - a rising edge on this pin advances the motor by one step (or one microstep, depending on microstepping setting). |
DIR | Direction input - sets the rotation direction of the stepper motor. |
Also, there is a tiny built-in knob that you can turn to adjust the current limit to help stop the stepper motor and driver from getting too hot.
In short, these 16 pins are grouped into the following types based on their function:
- Pins linked to the stepper motor: 1A, 1B, 2A, 2B.
- Pins connected to Arduino Nano for controlling the driver: ENABLE, M1, M2, M3, RESET, SLEEP.
- Pins connected to Arduino Nano for controlling motor direction and speed: DIR, STEP.
- Pin for sending feedback to Arduino Nano: FAULT.
- Pins connected to the motor's power source: VMOT, GND (motor power ground).
- Pin connected to the Arduino Nano's ground: GND (logic ground).
The DRV8825 module doesn't need a power supply from the Arduino Nano for its logic because it gets power from the motor's power supply through its built-in 3.3V voltage regulator. Still, it's crucial to connect the Arduino Nano's ground to the GND (logic) pin on the DRV8825 module to make sure it works correctly and shares a common ground.
Microstep Configuration
The DRV8825 driver allows for microstepping by breaking each step into smaller parts. This is done by sending varying levels of current to the motor coils.
For instance, NEMA 17 motor with a 1.8-degree step angle (200 steps per rotation):
- Full-step mode: 200 steps per rotation
- Half-step mode: 400 steps per rotation
- Quarter-step mode: 800 steps per rotation
- Eighth-step mode: 1600 steps per rotation
- Sixteenth-step mode: 3200 steps per rotation
- Thirty-second-step mode: 6400 steps per rotation
As you boost the microstepping setting, the motor operates more smoothly and accurately, but it needs more steps for each full turn. If you keep the same rate of step pulses (pulses every second), each full turn will take more time, making the motor slower.
But if your microcontroller can send pulses fast enough to match the higher step count, you can keep or even boost speed. The real limit depends on how quickly both the driver and your microcontroller can handle these pulses without missing steps.
DRV8825 Microstep Selection Pins
The DRV8825 includes three inputs for selecting microstep resolution: M0, M1, and M2 pins. By setting these pins to certain logic levels, you can select from six different microstepping resolutions:
M0 Pin | M1 Pi | M2 Pi | Microstep Resolution |
---|---|---|---|
Low | Low | Low | Full step |
High | Low | Low | Half step |
Low | High | Low | 1/4 step |
High | High | Low | 1/8 step |
Low | Low | High | 1/16 step |
High | Low | High | 1/32 step |
Low | High | High | 1/32 step |
High | High | High | 1/32 step |
These tiny selection pins come with built-in resistors that pull them down, keeping them in a LOW state normally. If not connected, the motor will work in full-step mode.
How it Works
To operate a stepper motor with the DRV8825 module, you require at least two Arduino Nano pins: one for the DIR pin and another for the STEP pin. The DRV8825 decodes these signals from the Arduino Nano to accurately move the stepper motor.
- STEP Pin: Every pulse on the STEP pin moves the motor by one small step or a full step, depending on your setup.
- DIR Pin: Sets which way the motor turns.
The driver uses these signals and its settings to send control commands to the motor via the 1A, 1B, 2A, and 2B pins.
You can also set up extra pins on the DRV8825 module (ENABLE, M1, M2, M3, RESET, SLEEP) in one of three methods:
- Disconnect them so the driver works with basic settings.
- Connect them directly to GND or VCC for a steady mode.
- Link them to Arduino Nano pins to manage these functions actively in your programming.
Wiring Diagram between Arduino Nano, DRV8825 module and Stepper Motor
The diagram below shows the basic connections required between the Arduino Nano, DRV8825 module, and the stepper motor. With this configuration, the DRV8825 driver works in its standard mode (full-step).
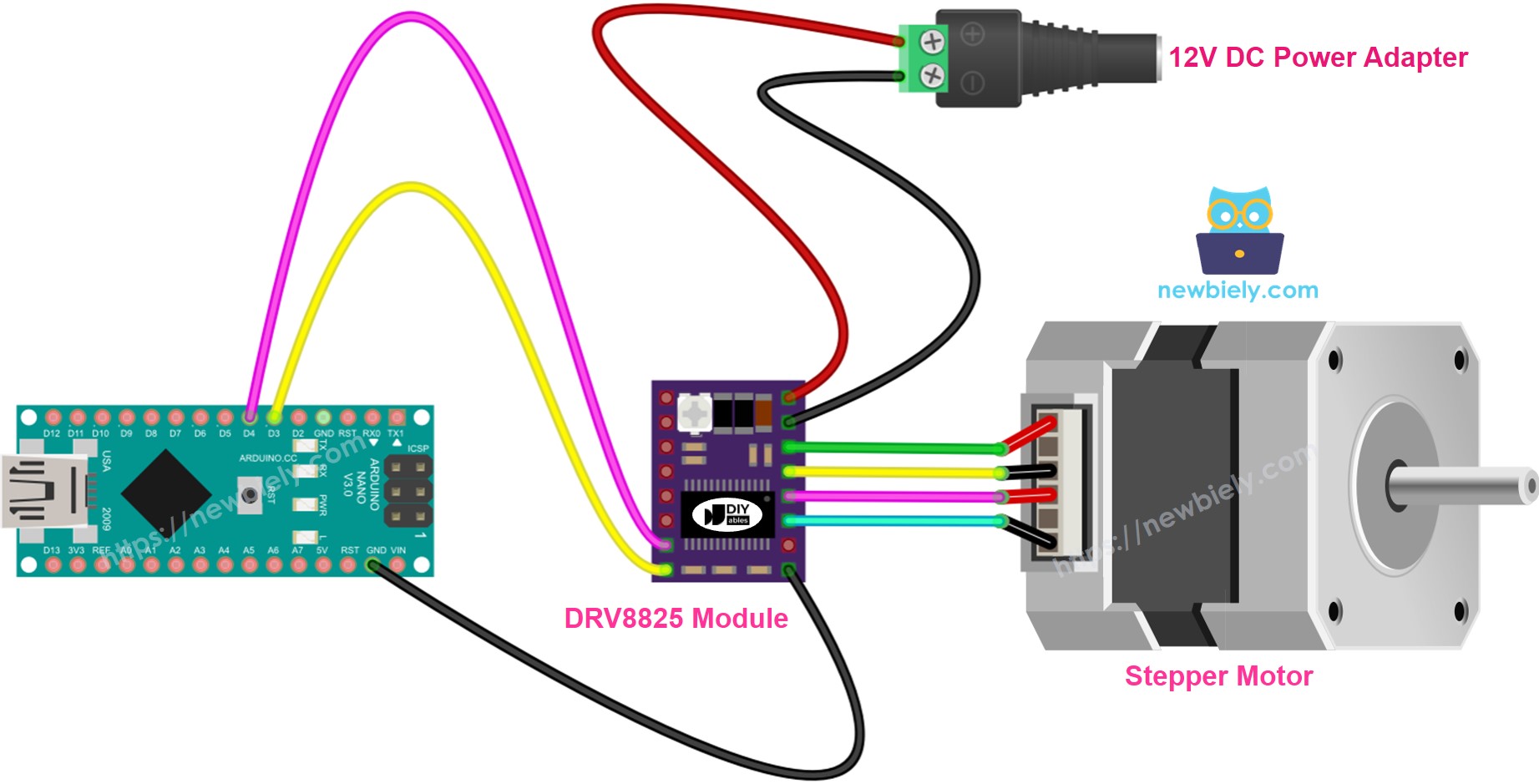
This image is created using Fritzing. Click to enlarge image
See The best way to supply power to the Arduino Nano and other components.
In depth:
- VMOT: Attach to the motor's power source (e.g., 12V).
- GND (for Motor): Link to the motor power supply's ground.
- 1A, 1B, 2A, 2B: Connect these to the coils of the stepper motor.
- STEP: Attach to the Arduino Nano digital pin D4.
- DIR: Link to the Arduino Nano digital pin D3.
- GND (for Logic): Connect to the Arduino Nano's GND pin.
- Other pins: Do not connect.
Arduino Nano Code
Detailed Instructions
- Copy the code and start the Arduino IDE.
- Go to the Libraries section in the left menu of the Arduino IDE.
- Look for "AccelStepper", and locate the AccelStepper library by Mike McCauley.
- Press the Install button to add the AccelStepper library.
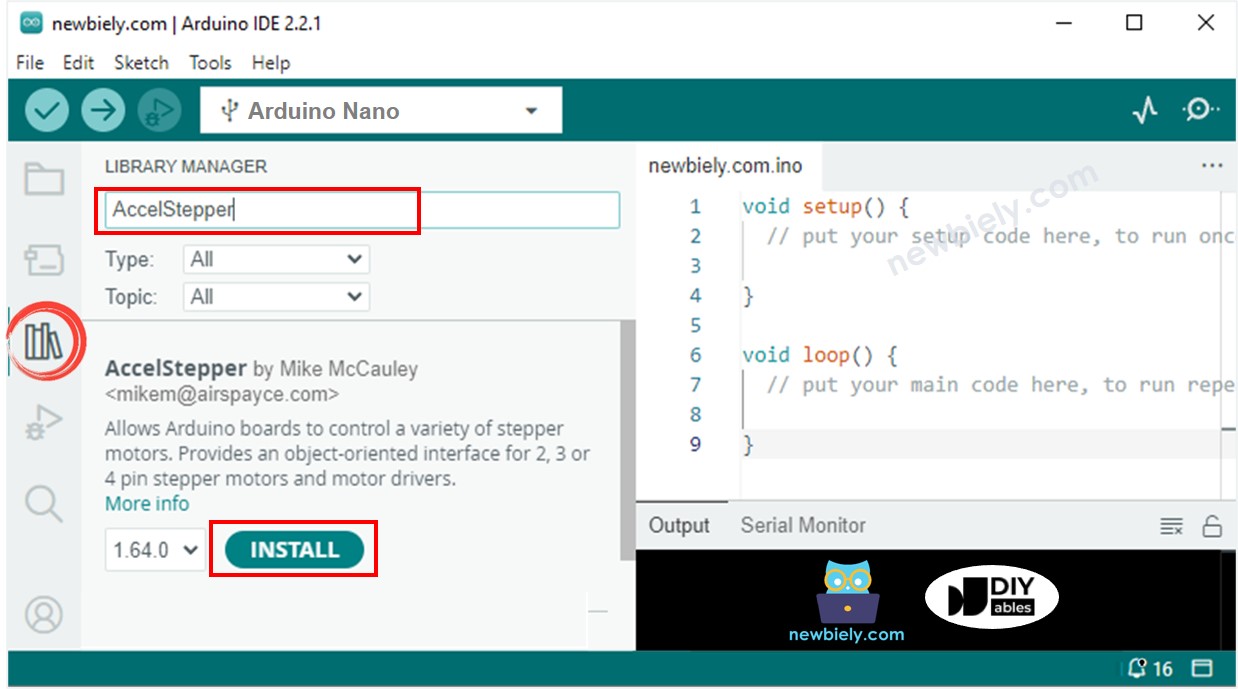
- Copy the code and open it in Arduino IDE
- Click the Upload button in Arduino IDE to upload the code to Arduino Nano
- You will see the motor move back and forth
When you use the motor in full-step mode, its movement might not be very smooth, which is common. For smoother movement, turn on microstepping by setting the M1, M2, and M3 pins.