ESP8266 - LDR Module
The LDR light sensor module can sense and measure the amount of light around it. It has two outputs: one that gives a digital signal (either LOW or HIGH) and another that gives an analog signal.
This tutorial instructs you how to use an ESP8266 and an LDR light sensor module to detect and measure the light. We will cover the following:
- How to connect the LDR light sensor module to an ESP8266.
- How to program the ESP8266 to detect light by reading the digital signal from the LDR light sensor module.
- How to program the ESP8266 to measure the light level by reading the analog signal from the LDR light sensor module.
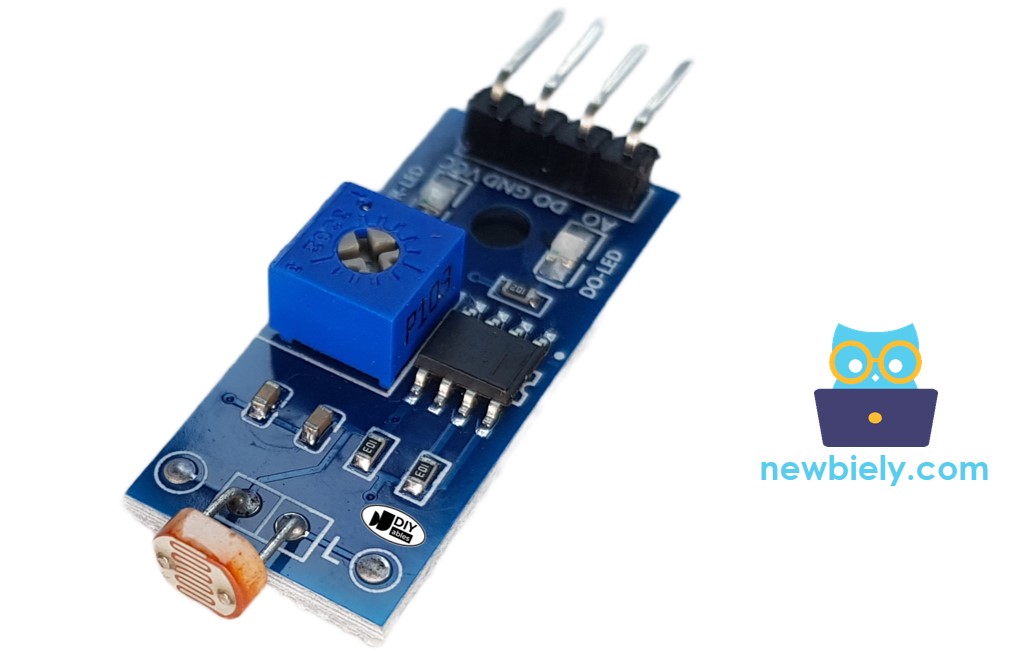
Later on, you can change the code so that when the LDR light sensor detects light, it can turn on an LED or a light bulb using a relay.
If you prefer a light sensor in its raw form, I suggest exploring the tutorial on the ESP8266 - Light Sensor.
Hardware Preparation
Or you can buy the following kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables .
Overview of LDR Light Sensor Module
You can use the LDR light sensor module to detect if there is light or measure how bright it is in the area. It has a digital output pin and an analog output pin for you to choose from.
Pinout
The LDR light sensor module has four pins:
- VCC pin: Connect it to a power source with a voltage between 3.3V and 5V.
- GND pin: Connect it to the ground, which has 0V.
- DO pin: This is a digital output pin. It gives a HIGH signal when it's dark and a LOW signal when it's light. You can adjust the threshold between darkness and lightness using a potentiometer that is built into the module.
- AO pin: This is an analog output pin. The output value decreases as the light gets brighter and increases as the light gets darker.
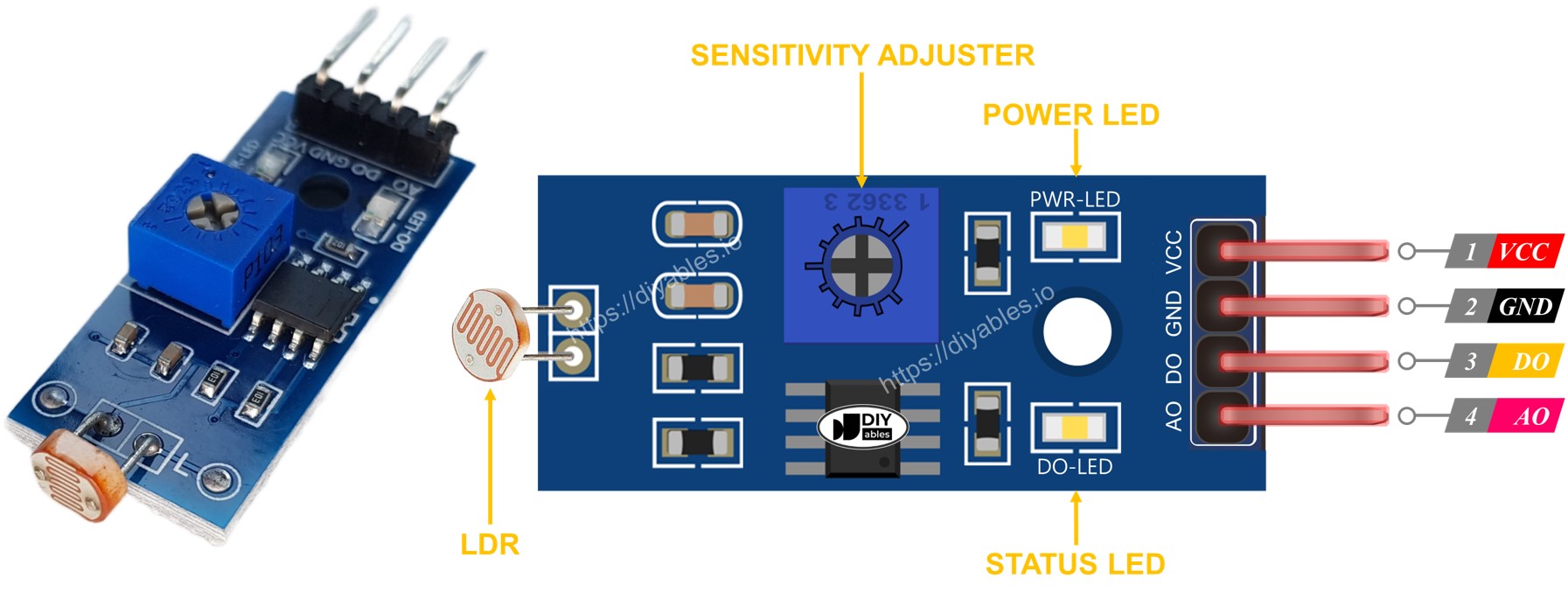
Furthermore, the LDR light sensor module includes two LED indicators:
- One PWR-LED indicator shows the power status.
- One DO-LED indicator shows the state of light on the DO pin: it turns on when there is light and turns off when it is dark.
How It Works
Regarding the DO pin:
- The LDR light sensor module has a built-in potentiometer that allows you to adjust the sensitivity or threshold for light detection.
- When the light intensity in the surrounding environment is higher than the set threshold (meaning it's light), the output pin of the sensor becomes LOW, and the DO-LED turns on.
- When the light intensity in the surrounding environment is lower than the set threshold (meaning it's dark), the output pin of the sensor becomes HIGH, and the DO-LED turns off.
Regarding the AO pin:
- The value read from the AO pin decreases as the light intensity in the surrounding environment increases (meaning it's brighter).
- The value read from the AO pin increases as the light intensity in the surrounding environment decreases (meaning it's darker).
Note that the potentiometer does not affect the value on the AO pin.
Wiring Diagram
Since the light sensor module has two outputs, you can choose to use one or both of them, depending on what you need.
- The wiring diagram between ESP8266 and the LDR light sensor module when using DO only.
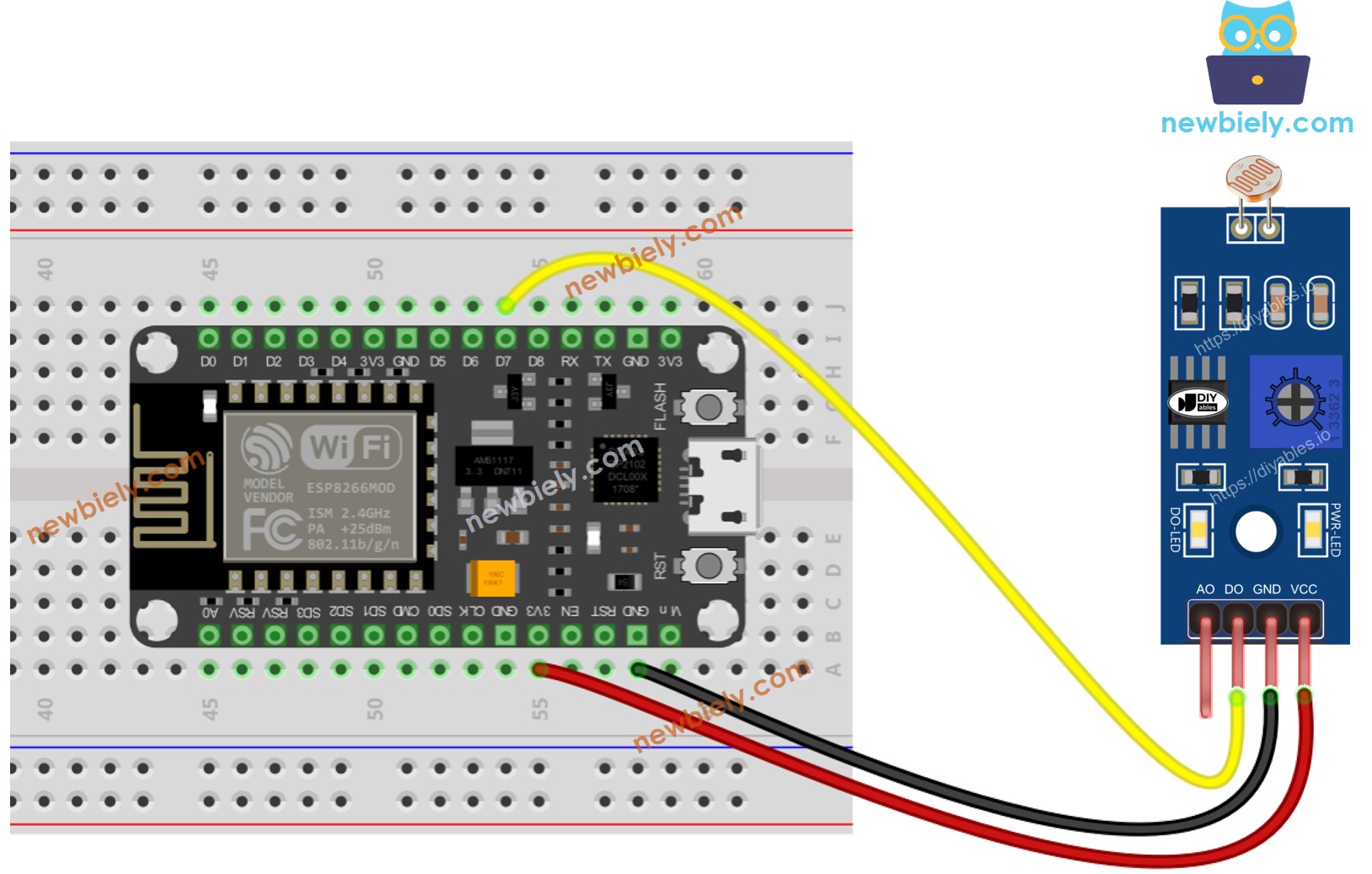
This image is created using Fritzing. Click to enlarge image
- The wiring diagram between ESP8266 and the LDR light sensor module when using AO only.
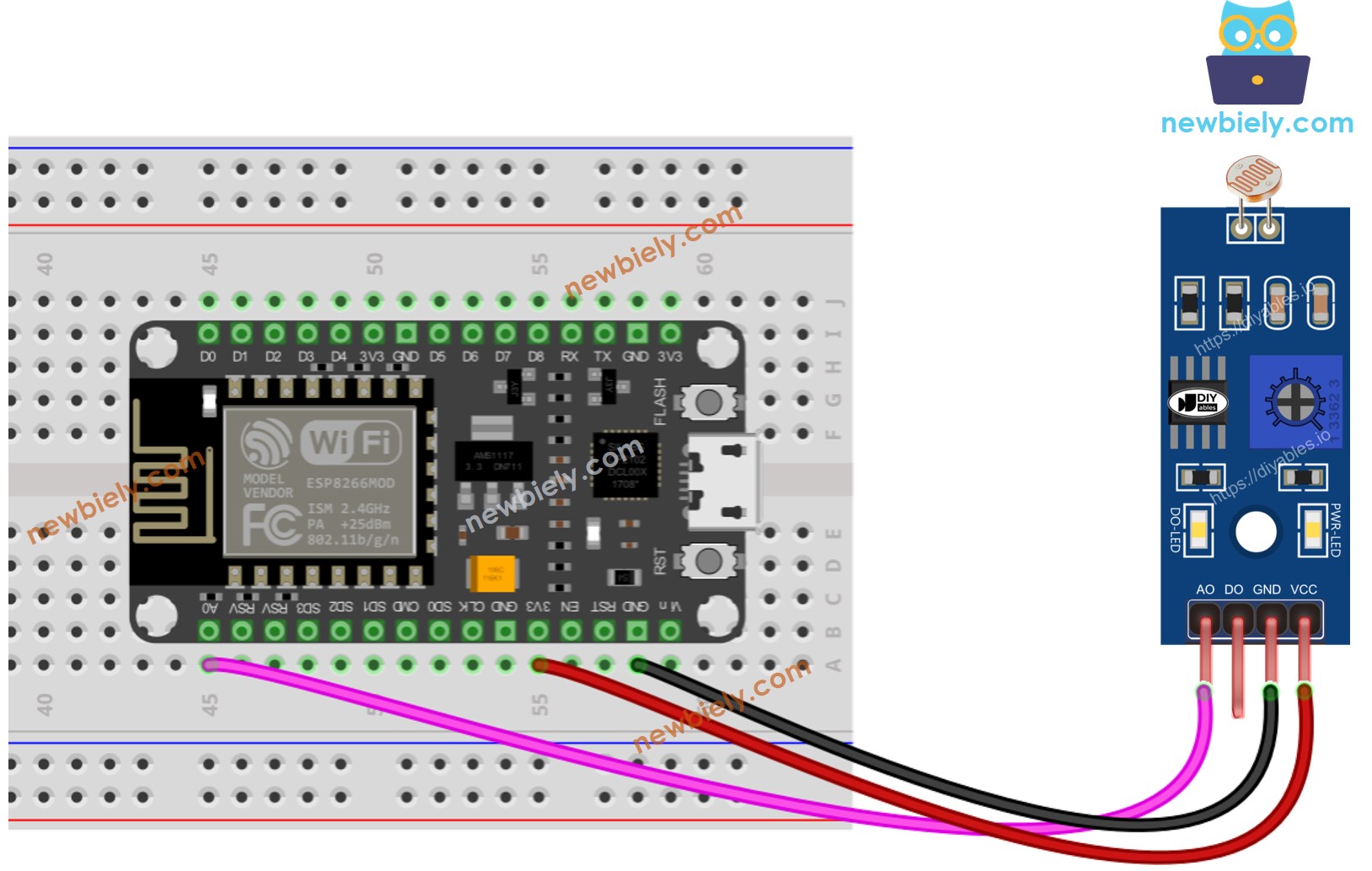
This image is created using Fritzing. Click to enlarge image
- The wiring diagram between ESP8266 and the LDR light sensor module when using both AO an DO.
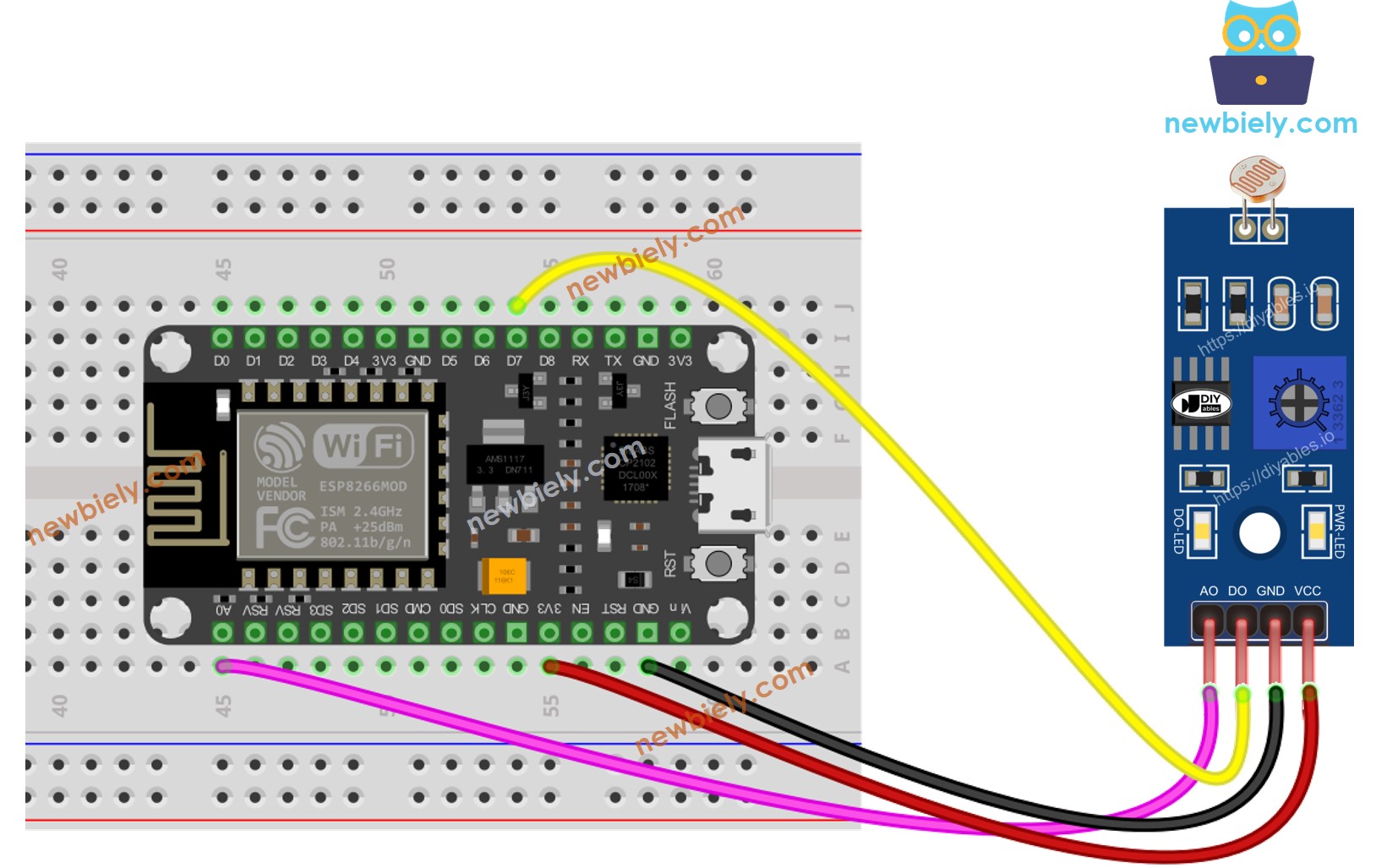
This image is created using Fritzing. Click to enlarge image
See more in ESP8266's pinout and how to supply power to the ESP8266 and other components.
ESP8266 Code - Read value from DO pin
Detailed Instructions
To get started with ESP8266 on Arduino IDE, follow these steps:
- Check out the how to setup environment for ESP8266 on Arduino IDE tutorial if this is your first time using ESP8266.
- Wire the components as shown in the diagram.
- Connect the ESP8266 board to your computer using a USB cable.
- Open Arduino IDE on your computer.
- Choose the correct ESP8266 board, such as (e.g. NodeMCU 1.0 (ESP-12E Module)), and its respective COM port.
- Copy the above code and open with Arduino IDE
- Click Upload button on Arduino IDE to upload code to ESP8266
- Cover and uncover the LDR light sensor module by your hand or something
- Check out the result on the Serial Monitor.
If you observe that the LED status remains constantly on or off, regardless of the light conditions, you have the option to adjust the potentiometer. This adjustment will allow you to fine-tune the light sensitivity of the sensor.
Additionally, you have the flexibility to modify the code to activate an LED or a light when light is detected. You can even control a servo motor to enable rotation. For detailed instructions and further information, please refer to the tutorials provided at the end of this guide.
ESP8266 Code - Read value from AO pin
Detailed Instructions
- Copy the above code and open with Arduino IDE
- Click Upload button on Arduino IDE to upload code to ESP8266
- Cover and uncover the LDR light sensor module by your hand or something
- Check out the result on the Serial Monitor.
※ NOTE THAT:
This tutorial uses the analogRead() function to get data from an ADC (Analog-to-Digital Converter) that's connected to a sensor or another part. The ESP8266's ADC works well for projects where you don't need very precise readings. But remember, the ESP8266's ADC isn't very accurate for detailed measurements. If your project needs to be very precise, you might want to use a separate ADC like the ADS1115 with the ESP8266, or use Arduino like the Arduino Uno R4 WiFi, which has a more reliable ADC.