ESP8266 - Water/Liquid Valve
This tutorial instructs you how to use an ESP8266 and a solenoid valve to control the flow of liquids such as water, beer, and oil. This same technique can be applied to controlling gas flow.
Hardware Preparation
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables .
Overview of Water/Liquid Valve
The Water/Liquid Valve Pinout
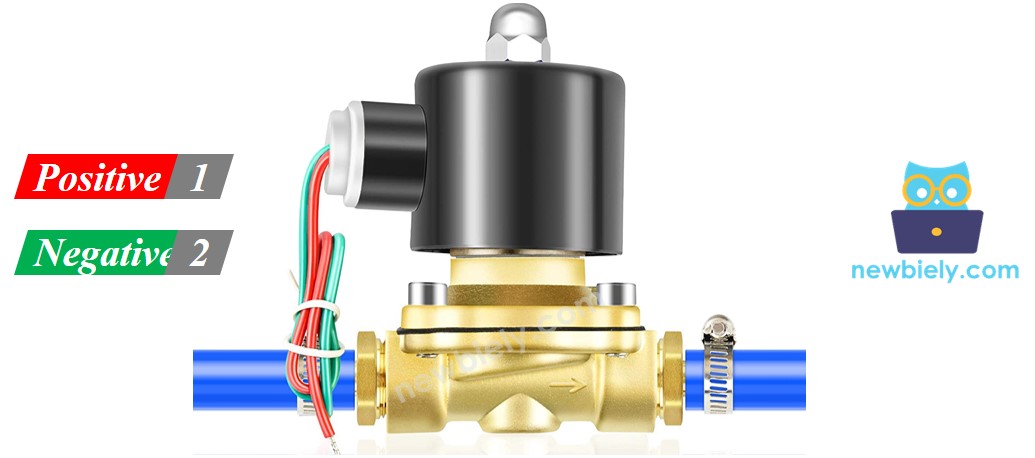
A solenoid valve typically has two terminals:
- The Positive (+) pin (red) should be connected to a 12V DC power supply
- The Negative (-) pin (black or other) should be connected to the GND of a DC power supply
How Water/Liquid Valve works
Typically, the valve is in a shut position. When 12V DC is supplied to the two terminals, the valve opens allowing water/liquid to pass through.
※ NOTE THAT:
- For certain types of valves, an internal gasket arrangement necessitates a minimum pressure to open the valve once 12V DC is applied. This pressure can be generated by the flow of liquid.
- Moreover, for some valves, the liquid is only able to flow in one direction.
How to Control Water/Liquid Solenoid Valve using ESP8266
If the valve is supplied with 12V, it will open. To control the valve, a relay must be used between ESP8266 and the valve. ESP8266 can then control the solenoid valve through the relay.
If you are unfamiliar with relays (pinout, how they work, how to program them, etc.), you can learn about them in the ESP8266 - Relay tutorial.
Wiring Diagram
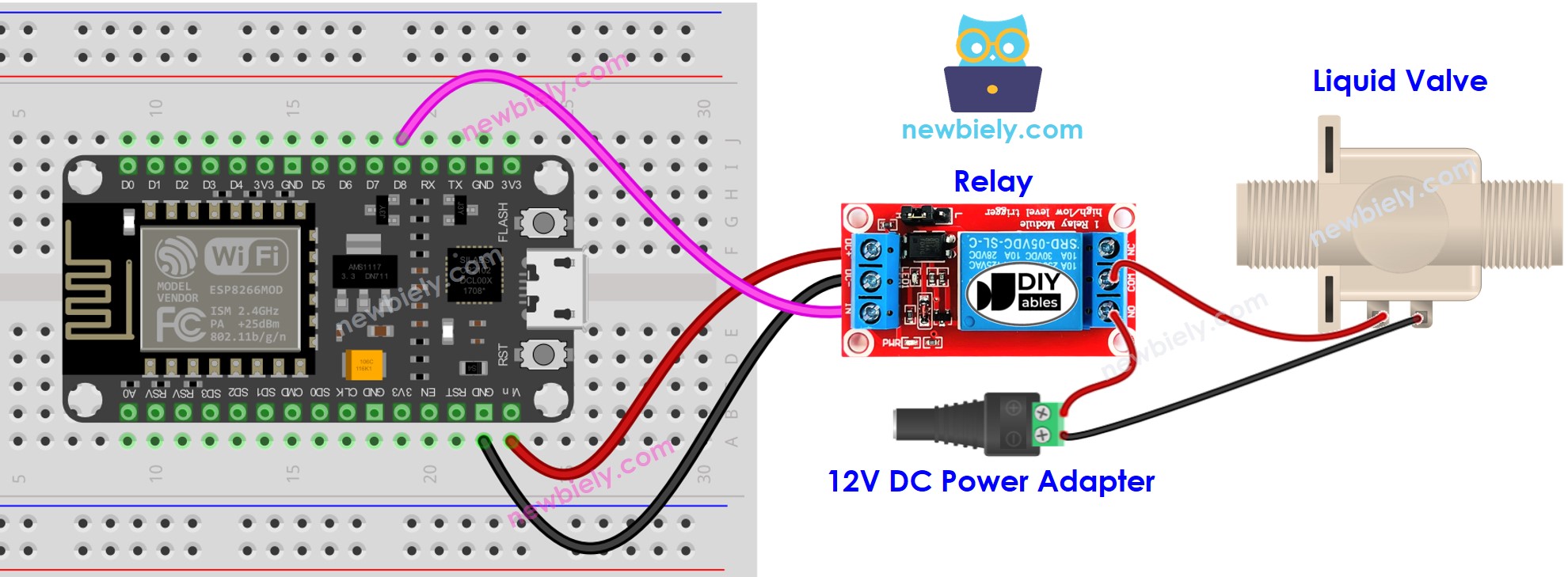
This image is created using Fritzing. Click to enlarge image
See more in ESP8266's pinout and how to supply power to the ESP8266 and other components.
ESP8266 Code for Controlling Liquid Valve
The below code turns the water valve ON for five seconds and then OFF for five seconds
Detailed Instructions
To get started with ESP8266 on Arduino IDE, follow these steps:
- Check out the how to setup environment for ESP8266 on Arduino IDE tutorial if this is your first time using ESP8266.
- Wire the components as shown in the diagram.
- Connect the ESP8266 board to your computer using a USB cable.
- Open Arduino IDE on your computer.
- Choose the correct ESP8266 board, such as (e.g. NodeMCU 1.0 (ESP-12E Module)), and its respective COM port.
- Sensor output on Serial Monitor
- Connect your ESP8266 to your computer using a USB cable.
- Launch the Arduino IDE, select the correct board and port.
- Copy the code above and open it in the Arduino IDE.
- Click the Upload button in the Arduino IDE to send the code to the ESP8266.
- Check the output of the water flow sensor on the Serial Monitor.
Code Explanation
Check out the line-by-line explanation contained in the comments of the source code!