ESP8266 - Potentiometer Servo Motor
This tutorial instructs you how to use ESP8266 to control the angle of a servo motor based on the input value from a potentiometer. In detail, we will learn:
- How to connect the potentiometer and servo motor to ESP8266
- How to program ESP8266 to read the value from a potentiometer and control a servo motor accordingly.
Hardware Preparation
Or you can buy the following kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables .
Overview of Servo Motor and Potentiometer
If you are unfamiliar with servo motors and potentiometers, including pinout, functioning, and programming, the following tutorials will be helpful:
Wiring Diagram
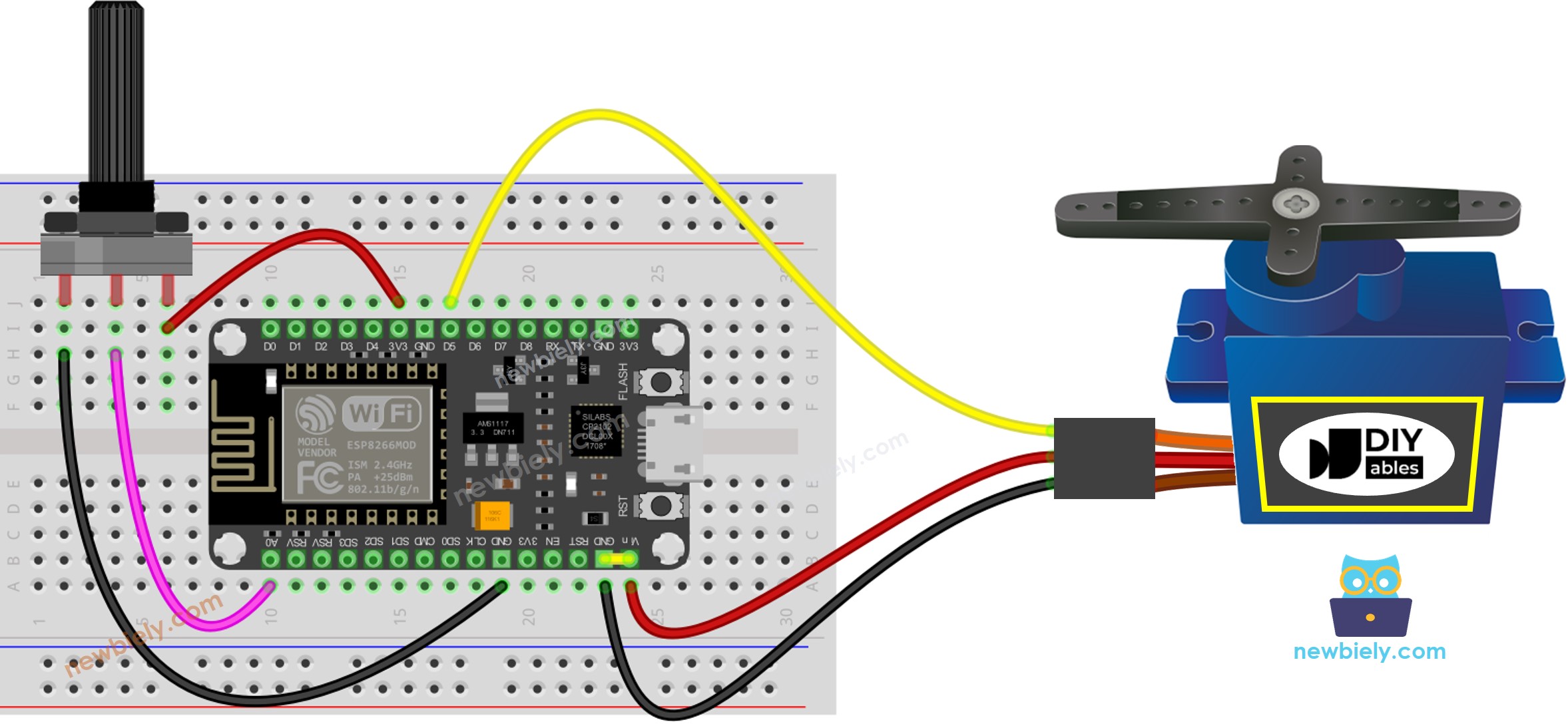
This image is created using Fritzing. Click to enlarge image
For the sake of simplicity, the above wiring diagram is utilized for testing or learning purposes, and for a small-torque servo motor. In actuality, we strongly suggest using an external power supply for the servo motor. The wiring diagram below illustrates how to connect the servo motor to an external power source.
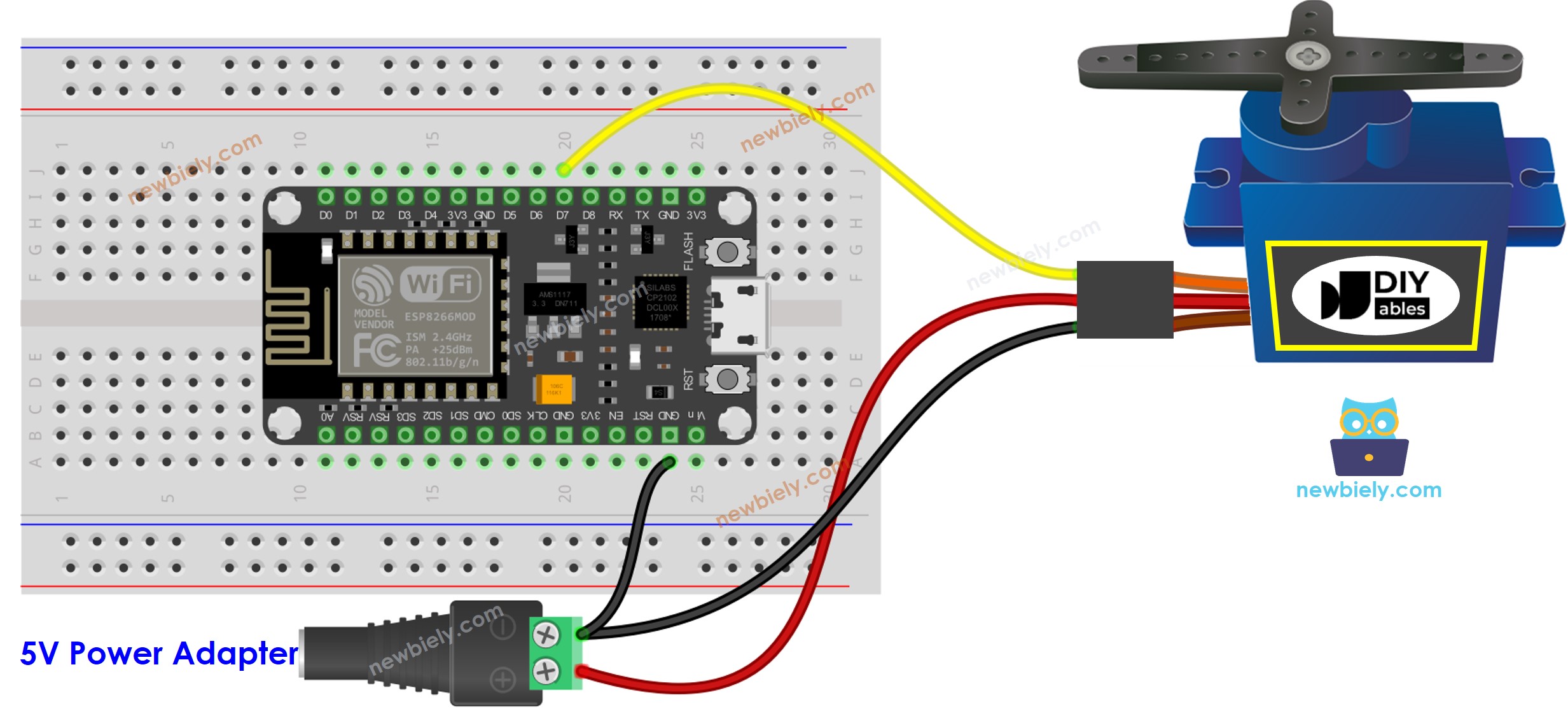
This image is created using Fritzing. Click to enlarge image
See more in ESP8266's pinout and how to supply power to the ESP8266 and other components.
How To Program
- Read the value from the potentiometer (ranging from 0 to 1023)
- Convert it it to an angle in the range of 0 to 180.
- Control the servo to the specified angle.
ESP8266 Code
Detailed Instructions
To get started with ESP8266 on Arduino IDE, follow these steps:
- Check out the how to setup environment for ESP8266 on Arduino IDE tutorial if this is your first time using ESP8266.
- Wire the components as shown in the diagram.
- Connect the ESP8266 board to your computer using a USB cable.
- Open Arduino IDE on your computer.
- Choose the correct ESP8266 board, such as (e.g. NodeMCU 1.0 (ESP-12E Module)), and its respective COM port.
- Connect your ESP8266 to your computer using a USB cable.
- Launch the Arduino IDE, select the appropriate board and port.
- Copy the code above and open it in the Arduino IDE.
- Click the Upload button in the Arduino IDE to transfer the code to the ESP8266.
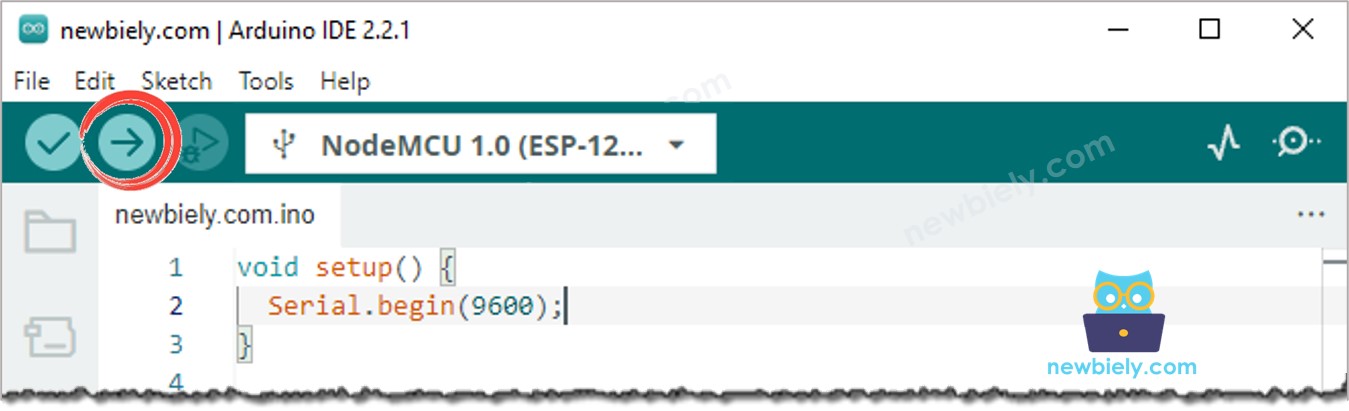
- Open the Serial Monitor
- Turn the potentiometer
- Check out the servo motor's rotation
- View the result in the Serial Monitor
Code Explanation
Check out the line-by-line explanation contained in the comments of the source code!