ESP8266 - Light Sensor
This tutorial instructs you how to use the light sensor with ESP8266. In detail:
- How a light sensor works
- How to connect a light sensor to an ESP8266
- How to program an ESP8266 to read the value from the light sensor
Hardware Preparation
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables .
The LDR light sensor is very affordable, but it requires a resistor for wiring, which can make the setup more complex. To simplify the wiring, you can use an LDR light sensor module as an alternative.
Overview of Light Sensor
The sensor used in this tutorial is a photoresistor, also known as a light-dependent resistor, LDR, or photocell.
It is not only used to detect light, but also to measure the intensity of the surrounding light.
The Light Sensor Pinout
A photoresistor has two pins which do not need to be distinguished, as it is a type of resistor and thus the pins are symmetric.
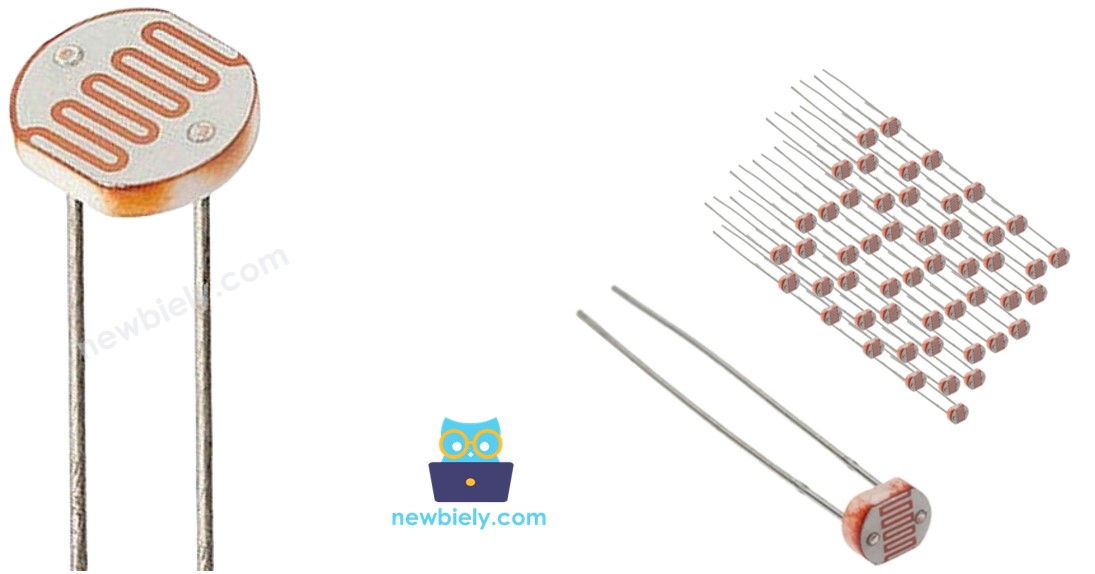
How It Works
The amount of light that the photoresistor's face is exposed to affects its resistance. By measuring this resistance, we can determine the brightness of the surrounding light.
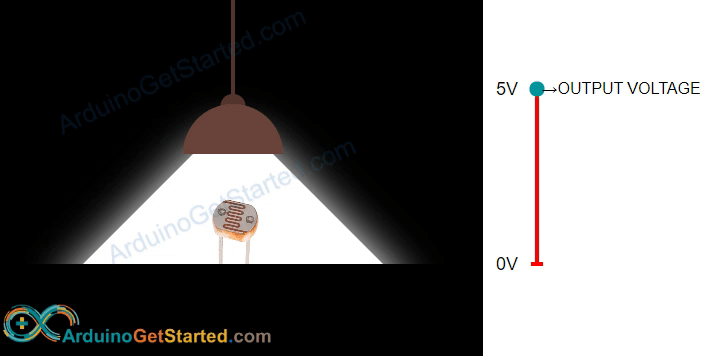
WARNING
The value from the light sensor only gives an approximate indication of the intensity of light, not a precise measure of luminous flux. Consequently, it should not be used for applications that need a high degree of accuracy.
ESP8266 - Light Sensor
ESP8266's analog pins can be used as analog inputs. These analog input pins convert the voltage (ranging from 0 volts to VCC) into integer values (ranging from 0 to 1023), referred to as ADC value or analog value.
Connect one pin of the photoresistor to an analog input pin. Using analogRead() function, read the analog value from the pin. This will allow us to determine the light levels relatively.
Wiring Diagram
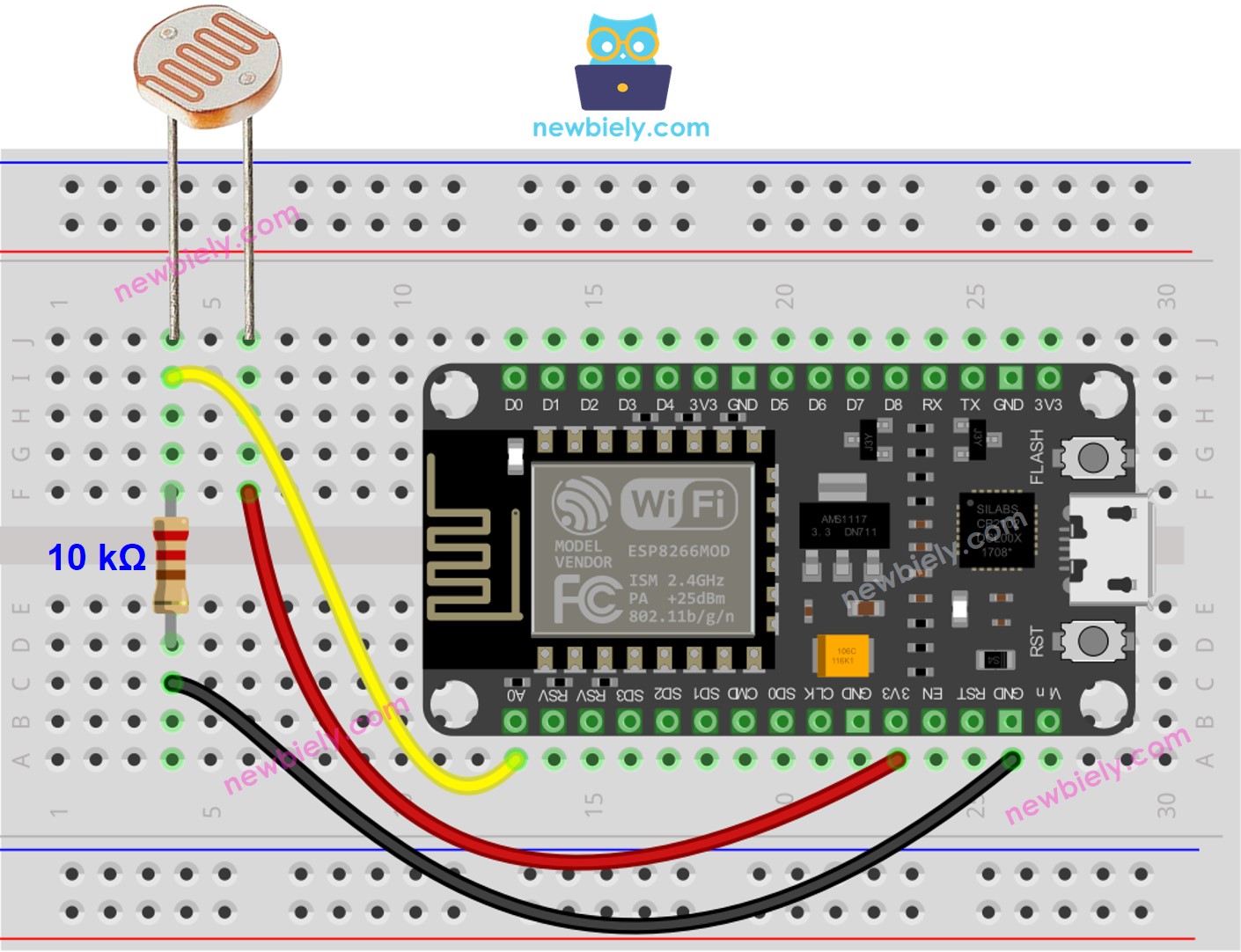
This image is created using Fritzing. Click to enlarge image
ESP8266 Code
The code below reads the value from the photocell and qualitatively determines the light level.
Detailed Instructions
To get started with ESP8266 on Arduino IDE, follow these steps:
- Check out the how to setup environment for ESP8266 on Arduino IDE tutorial if this is your first time using ESP8266.
- Wire the components as shown in the diagram.
- Connect the ESP8266 board to your computer using a USB cable.
- Open Arduino IDE on your computer.
- Choose the correct ESP8266 board, such as (e.g. NodeMCU 1.0 (ESP-12E Module)), and its respective COM port.
- Copy the code and open it with the Arduino IDE.
- Click the Upload button on the Arduino IDE to compile and upload the code to the ESP8266.
- Open the Serial Monitor.
- Shine a light on the sensor.
- Check out the result on the Serial Monitor.
Light Sensor and LED
- When the environment is dark, the code will activate the LED, otherwise it will deactivate it.
- The wiring diagram for the code above.
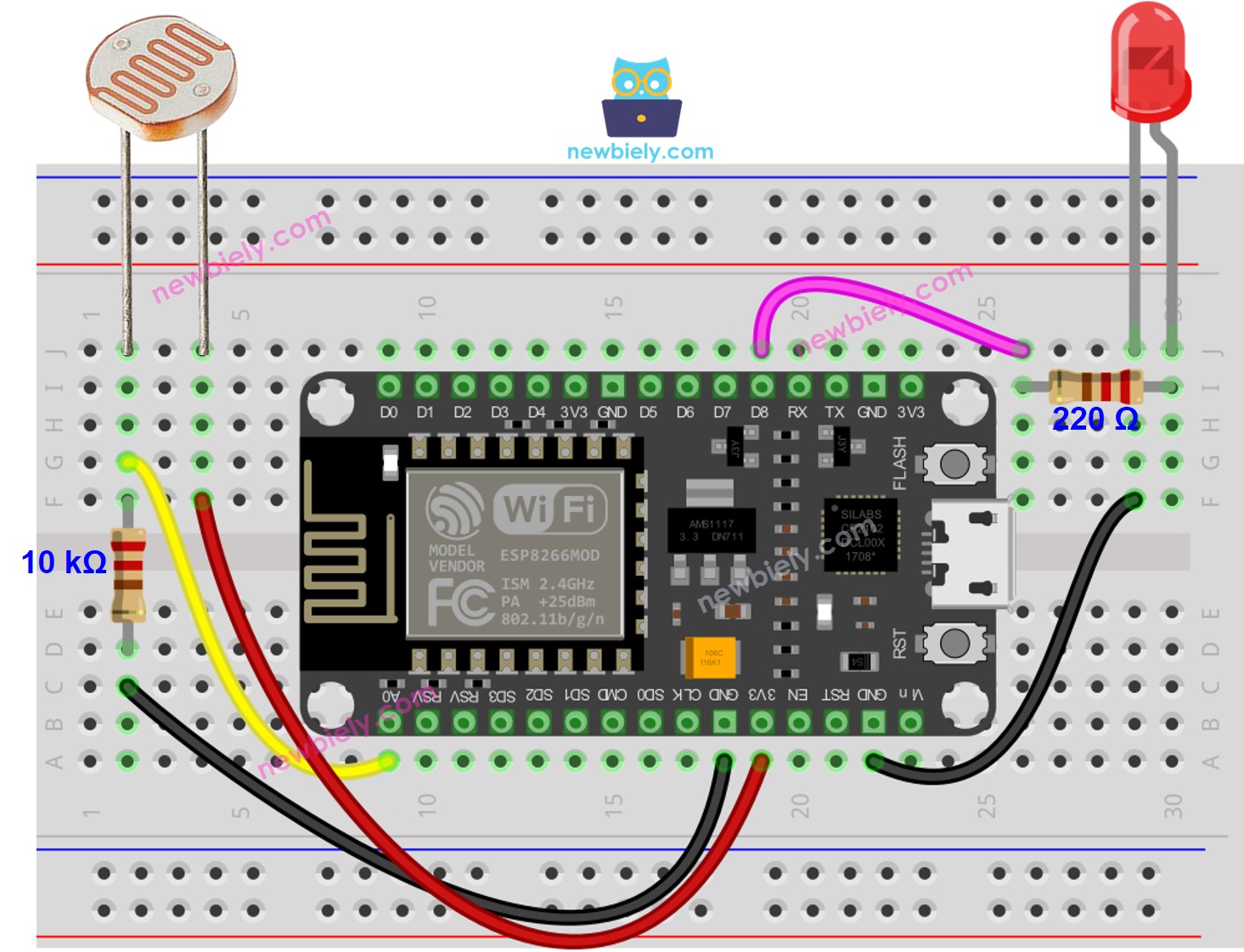
This image is created using Fritzing. Click to enlarge image
See more in ESP8266's pinout and how to supply power to the ESP8266 and other components.
※ NOTE THAT:
This tutorial uses the analogRead() function to get data from an ADC (Analog-to-Digital Converter) that's connected to a sensor or another part. The ESP8266's ADC works well for projects where you don't need very precise readings. But remember, the ESP8266's ADC isn't very accurate for detailed measurements. If your project needs to be very precise, you might want to use a separate ADC like the ADS1115 with the ESP8266, or use Arduino like the Arduino Uno R4 WiFi, which has a more reliable ADC.
Video Tutorial
Challenge Yourself
- When the light in your room is dim, activate it automatically.
- Refer to ESP8266 - Relay for instructions on how to do this.