ESP8266 - WebSocket
In this tutorial, we will learn what WebSocket is, why we need to use it to smoothly control ESP8266, and how to use WebSocket with ESP8266. In a practical example, we will learn how to create a chat application between the web browser and ESP8266, allowing you to:
- Type a message in the chat window on the web browser and send it to ESP8266. You can adapt this to control the ESP8266.
- Receive real-time messages from ESP8266. You can adapt this to monitor ESP8266 in real-time.
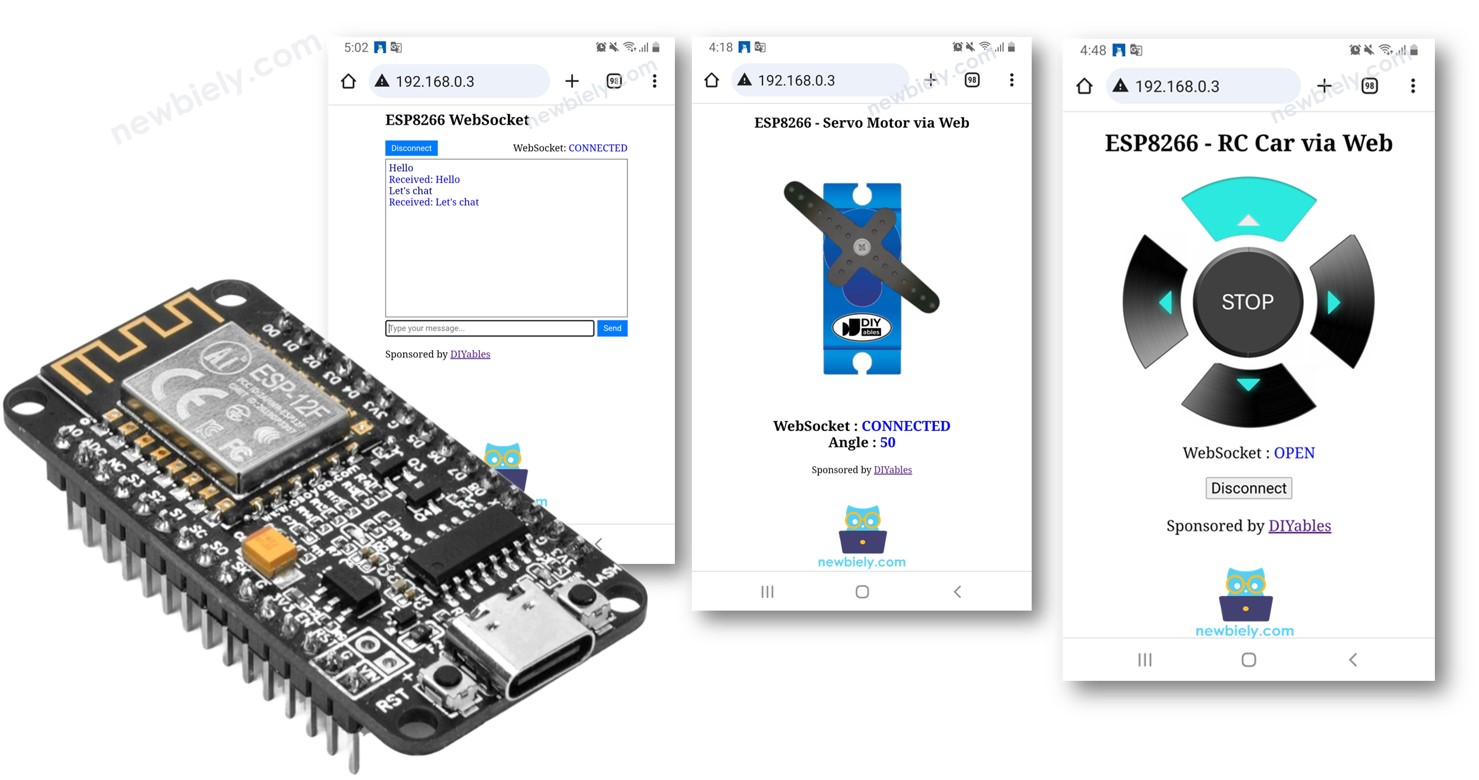
Hardware Preparation
Or you can buy the following kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables .
What is ESP8266 Websocket?
Now, you might be asking, "What's WebSocket?" It's pretty simple: WebSocket is a technology that lets a web browser talk directly to a web server in real-time.
- Without WebSocket, you have to refresh the webpage to get updates. That's not very convenient.
- With WebSocket, the webpage and the server stay connected all the time. This means they can share information instantly without having to reload the page.
You probably run into WebSocket in everyday web apps like online games, instant messaging, and stock market updates.
Why we need WebSocket to smoothly control ESP8266?
Imagine you want to control your remote-controlled car using a web interface on your phone or computer. Without WebSocket, every time you want to change the car's direction or speed, you'd need to refresh the web page. It's like having to press a "reload" button each time you want your command to reach the car.
Now, with WebSocket, it's like having a continuous and direct connection between your phone or computer and the car. You no longer need to refresh the page every time you want to steer the car or adjust its speed. It's as if the car is always listening to your commands in real-time, without any delays caused by constant page reloading.
In general, WebSocket allows you to:
- Send data from the web browser to ESP8266 without reloading the webpage.
- Send data from ESP8266 to the web browser without reloading the webpage.
This enables two-way communication in real-time manner.
Benefits of WebSocket with ESP8266:
- Real-Time Control: WebSocket enables instant communication with the ESP8266, ensuring quick responses to commands for a seamless user experience.
- Persistent Connection: Maintain a continuous link without refreshing the control page, creating an always-ready line of communication for immediate instructions.
- Efficiency: Experience prompt responses without the need for constant page reloading, enhancing overall user enjoyment and efficiency.
Web Chat with ESP8266 via WebSocket
The webpage's content (HTML, CSS, JavaScript) are stored separately on an index.h file. So, we will have two code files on Arduino IDE:
- An .ino file that is ESP8266 code, which creates a web sever and WebSocket server
- An .h file, which contains the webpage's content.
Detailed Instructions
- If this is the first time you use ESP8266, see how to setup environment for ESP8266 on Arduino IDE.
- Connect the ESP8266 board to your PC via a micro USB cable
- Open Arduino IDE on your PC.
- Select the right ESP8266 board (e.g. NodeMCU 1.0 (ESP-12E Module)) and COM port.
- Open the Library Manager by clicking on the Library Manager icon on the left navigation bar of Arduino IDE.
- Search “WebSockets”, then find the WebSockets created by Markus Sattler.
- Click Install button to install WebSockets library.
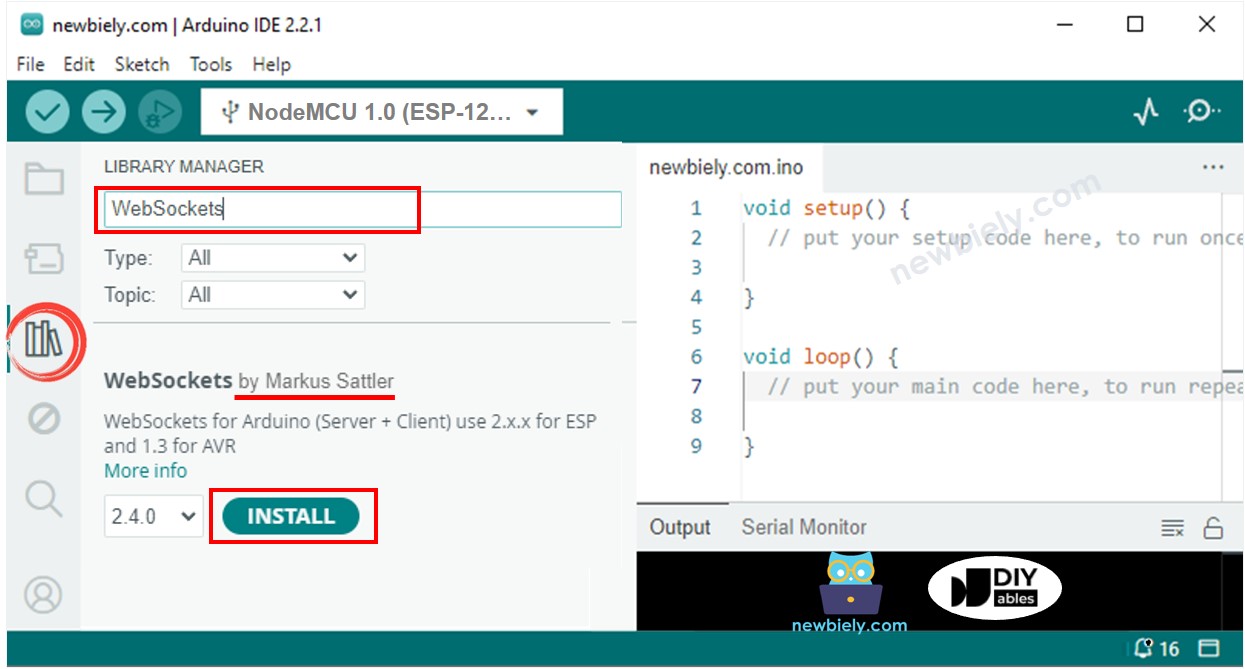
- On Arduino IDE, create new sketch, Give it a name, for example, newbiely.com.ino
- Copy the below code and open with Arduino IDE
- Create the index.h file On Arduino IDE by:
- Either click on the button just below the serial monitor icon and choose New Tab, or use Ctrl+Shift+N keys.
- Give the file's name index.h and click OK button
- Copy the below code and paste it to the index.h.
- Modify the WiFi information (SSID and password) in the code to match your own network credentials.
- Now you have the code in two files: newbiely.com.ino and index.h
- Click Upload button on Arduino IDE to upload code to ESP8266.
- Open the Serial Monitor
- Check out the result on Serial Monitor.
- Take note of the IP address displayed, and enter this address into the address bar of a web browser on your smartphone or PC.
- You will see the webpage it as below:
- Click the CONNECT button to connect the webpage to ESP8266 via WebSocket.
- Type some words and send them to ESP8266.
- You will see the response from ESP8266.
- If you modify the HTML content in the index.h and does not touch anything in newbiely.com.ino file, when you compile and upload code to ESP8266, Arduino IDE will not update the HTML content.
- To make Arduino IDE update the HTML content in this case, make a change in the newbiely.com.ino file (e.g. adding empty line, add a comment....)
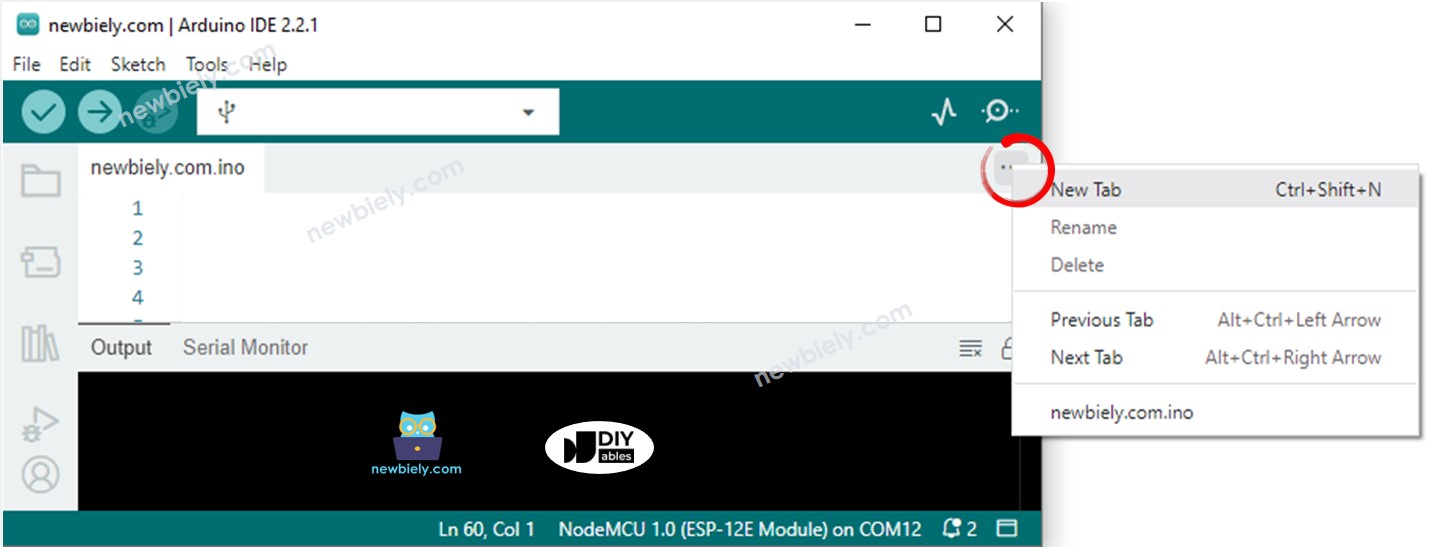
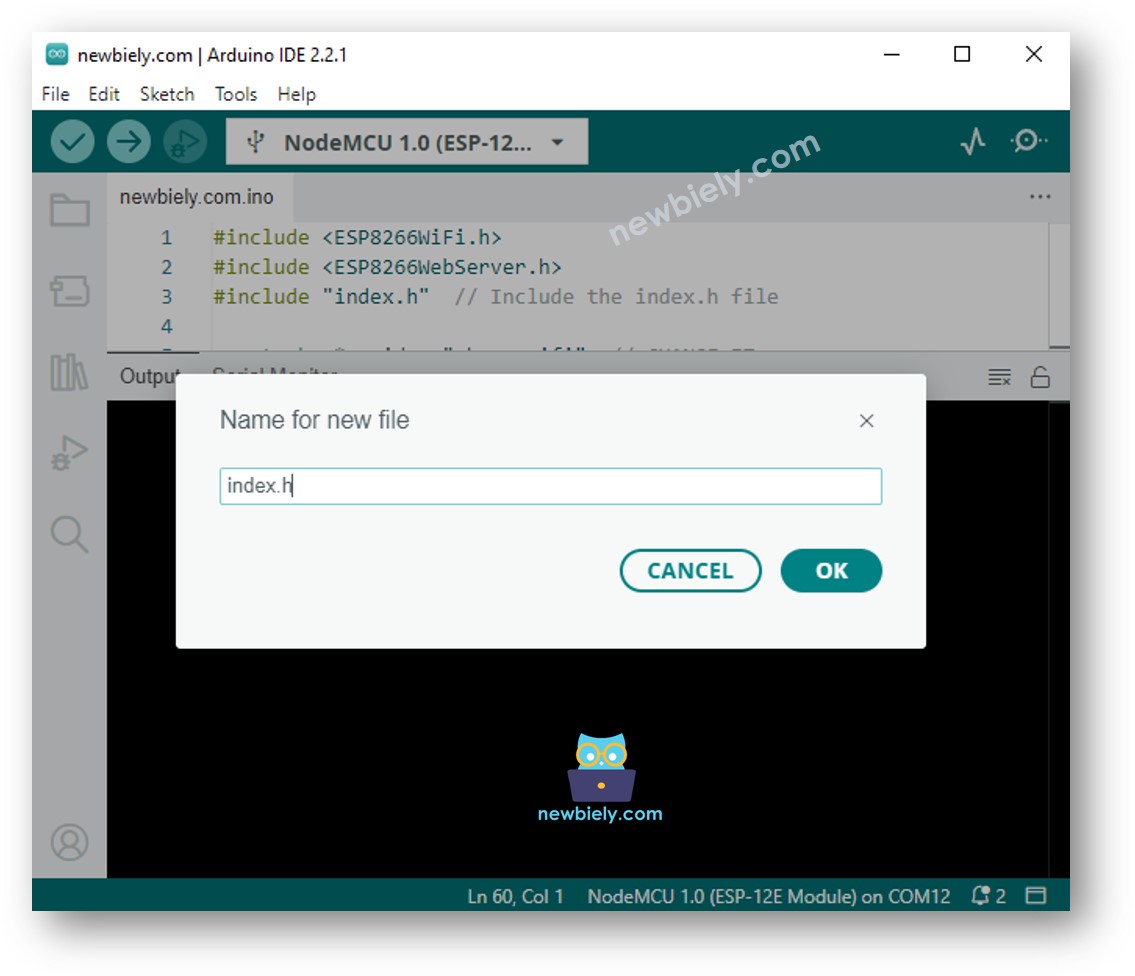
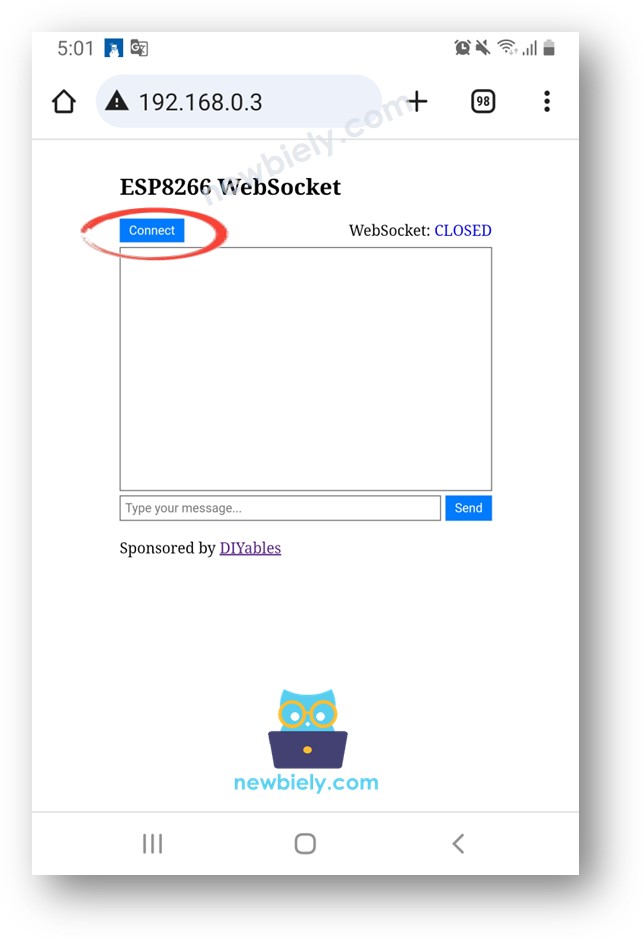
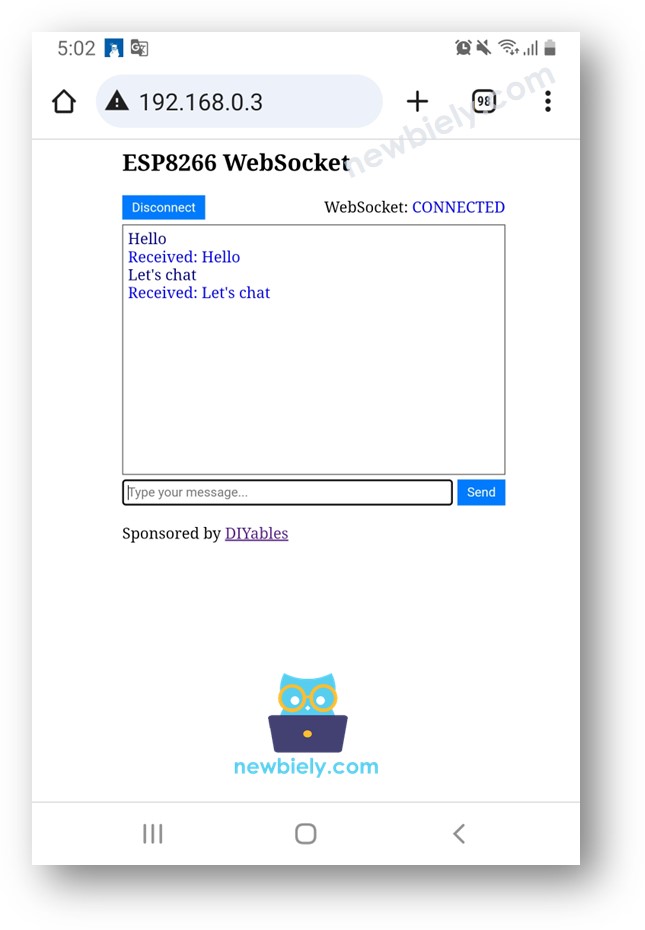
※ NOTE THAT:
Line-by-line Code Explanation
The above ESP8266 code contains line-by-line explanation. Please read the comments in the code!
How the System Operates
The ESP8266 code functions by creating both a web server and a WebSocket Server. The process unfolds as follows:
- When you input the ESP8266's IP address into a web browser, a request is made for the webpage (User Interface) from the ESP8266.
- The ESP8266's web server responds by transmitting the content of the webpage (HTML, CSS, JavaScript).
- Subsequently, your web browser displays the webpage.
- When you click the CONNECT button, the JavaScript code embedded in the webpage establishes a WebSocket connection to the WebSocket server on the ESP8266.
- With the WebSocket connection established, when you type something and click the SEND button, the JavaScript code send that text to to the ESP8266 through the WebSocket connection in the background.
- Upon receiving the angle value, the WebSocket server sends back a reply to the webpage.
You can learn other ESP8266 WebSocket examples below: