ESP8266 - Motion Sensor - Relay
This tutorial instructs you how to use ESP8266 and motion sensor to control relay. In detail:
- ESP8266 activates the relay when motion is detected
- ESP8266 deactivates the relay when motion is not present
By connecting a relay to a light bulb, LED strip, motor or actuator, we can use ESP8266 and a motion sensor to control the light bulb, LED strip, motor or actuator.
This can be applied in an automation process that triggers actions upon detecting human presence.
Hardware Preparation
Or you can buy the following sensor kit:
1 | × | DIYables Sensor Kit 30 types, 69 units |
Overview of Relay and Motion Sensor
If you are unfamiliar with relay and motion sensor (pinout, functioning, programming ...), the following tutorials can help:
Wiring Diagram
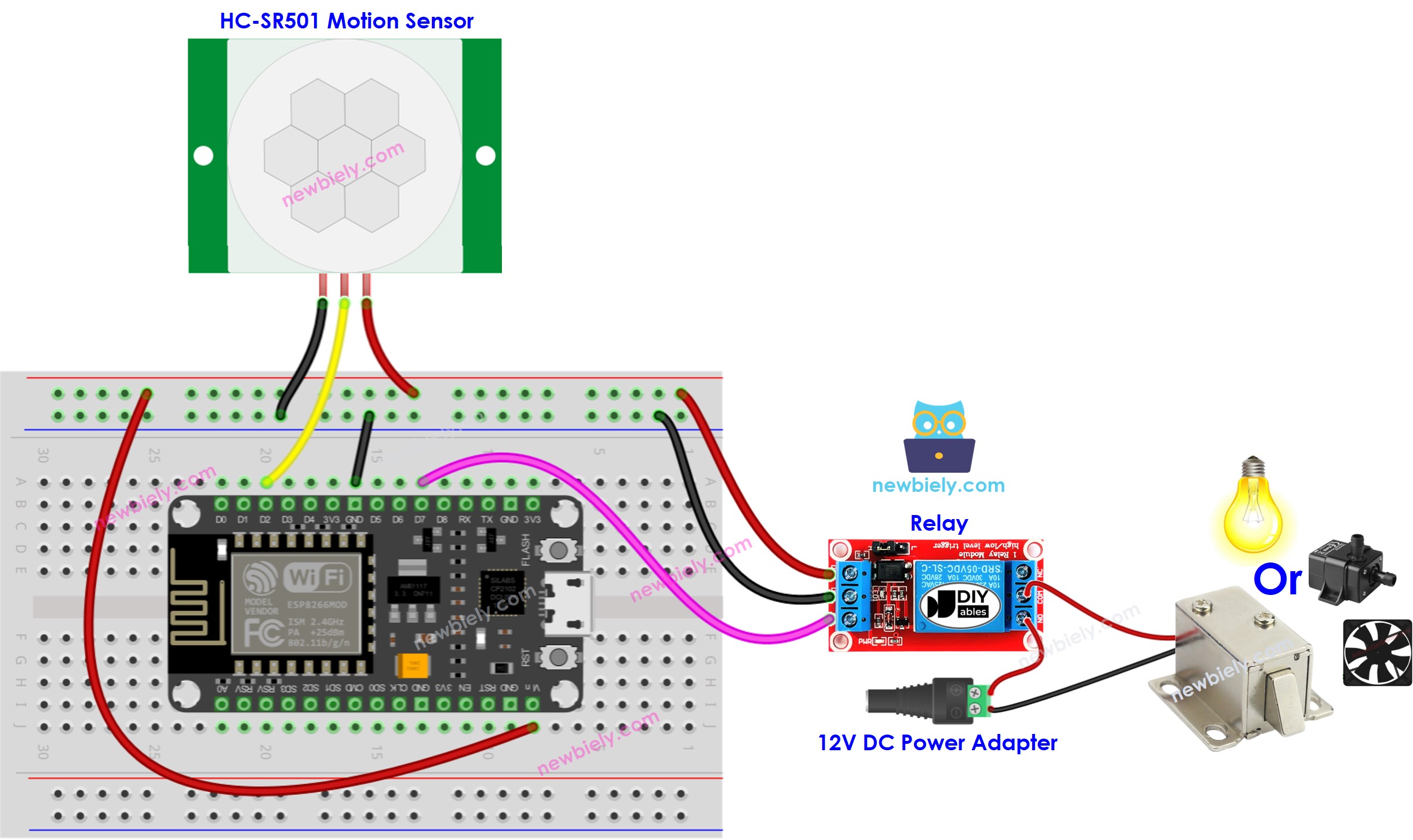
This image is created using Fritzing. Click to enlarge image
See more in ESP8266's pinout and how to supply power to the ESP8266 and other components.
Initial Setting
Time Delay Adjuster | Screw it in anti-clockwise direction fully. |
Detection Range Adjuster | Screw it in clockwise direction fully. |
Repeat Trigger Selector | Put jumper as shown on the image. |
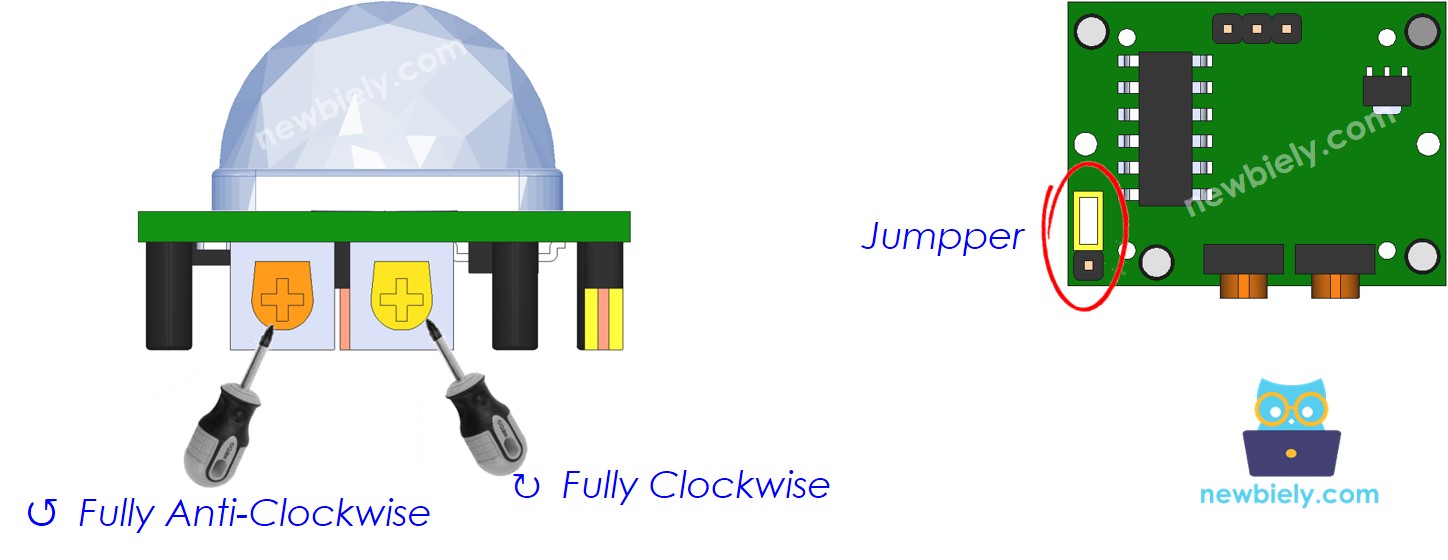
ESP8266 Code
Detailed Instructions
To get started with ESP8266 on Arduino IDE, follow these steps:
- Check out the how to setup environment for ESP8266 on Arduino IDE tutorial if this is your first time using ESP8266.
- Wire the components as shown in the diagram.
- Connect the ESP8266 board to your computer using a USB cable.
- Open Arduino IDE on your computer.
- Choose the correct ESP8266 board, such as (e.g. NodeMCU 1.0 (ESP-12E Module)), and its respective COM port.
- Connect your ESP8266 to your computer using a USB cable.
- Launch the Arduino IDE, select the correct board and port.
- Copy the code and open it in the Arduino IDE.
- Click the Upload button in the Arduino IDE to send the code to the ESP8266.
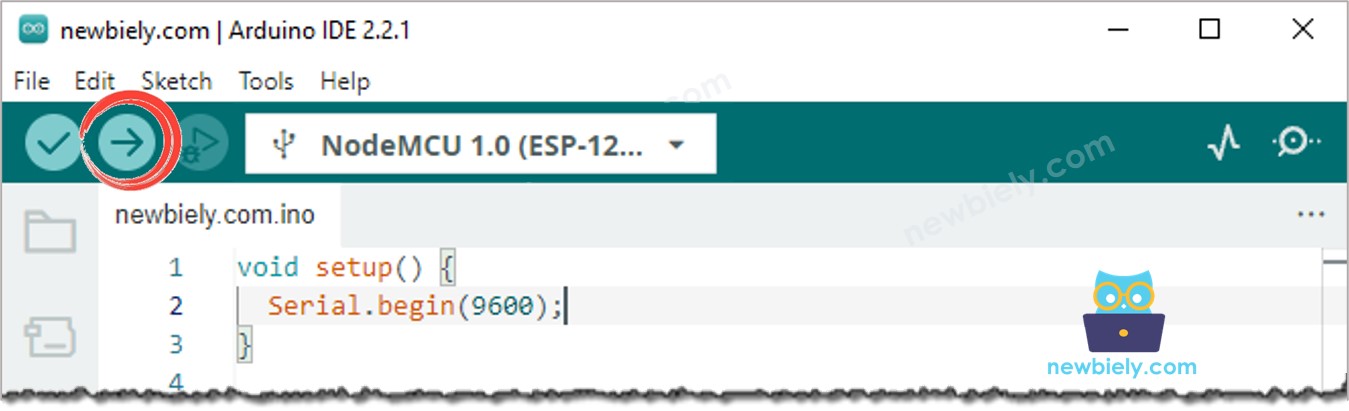
Code Explanation
Check out the line-by-line explanation contained in the comments of the source code!