ESP8266 - RFID - Relay
This tutorial instructs you how to use an RFID/NFC tag to trigger a relay with the help of ESP8266. You can further expand upon this tutorial by using the relay to control motors, actuators, and more.
Hardware Preparation
Or you can buy the following kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables .
Overview of RFID/NFC RC522 Module and Relay
If you are not familiar with the RFID/NFC RC522 Module and relay (including pinout, functionality, and programming), then check out the following tutorials:
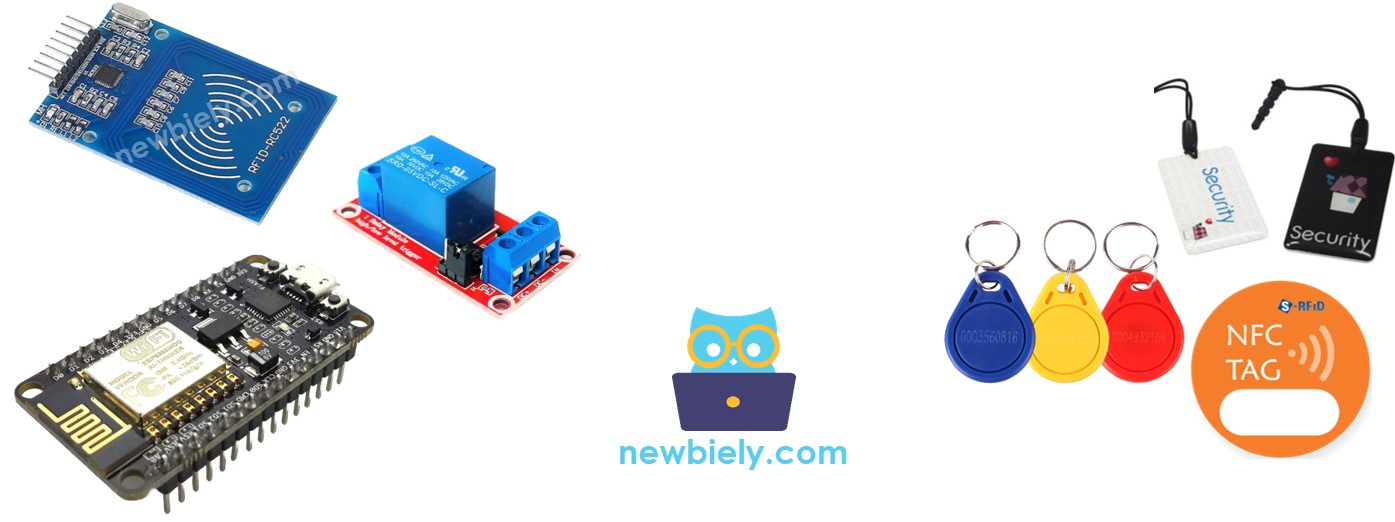
How It Works
- The RFID/NFC reader reads the UID from a tag when it is tapped.
- ESP8266 then takes this UID and compares it with the UIDs that have been preset in the code.
- If the UID matches one of these predefined UIDs, the ESP8266 will activate the relay.
Wiring Diagram
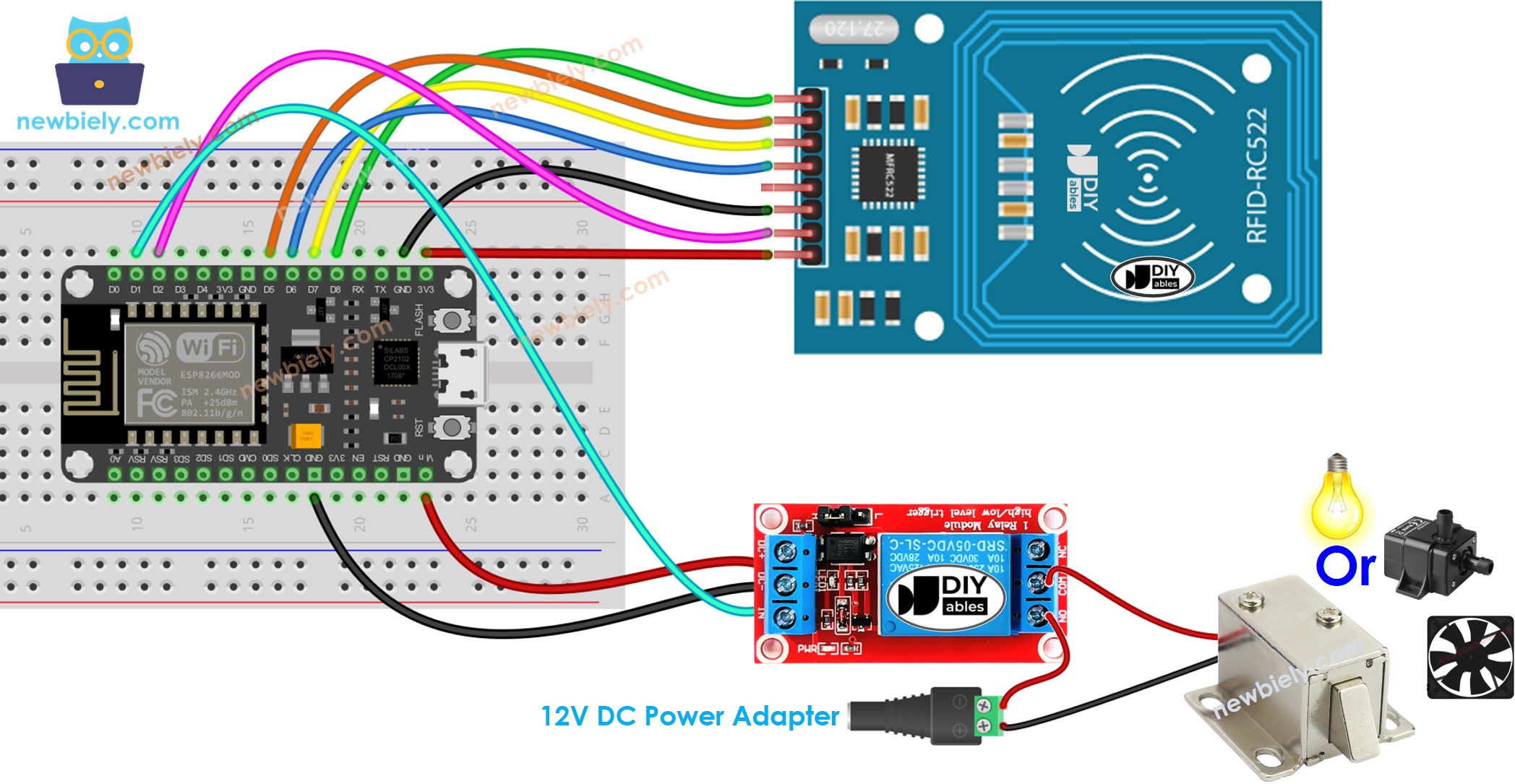
This image is created using Fritzing. Click to enlarge image
See more in ESP8266's pinout and how to supply power to the ESP8266 and other components.
※ NOTE THAT:
The sequence of pins may differ depending on the manufacturer. ALWAYS use the labels printed on the module. The image above displays the pinout of modules from DIYables producer.
ESP8266 Code - Single RFID/NFC Tag
Detailed Instructions
To get started with ESP8266 on Arduino IDE, follow these steps:
- Check out the how to setup environment for ESP8266 on Arduino IDE tutorial if this is your first time using ESP8266.
- Wire the components as shown in the diagram.
- Connect the ESP8266 board to your computer using a USB cable.
- Open Arduino IDE on your computer.
- Choose the correct ESP8266 board, such as (e.g. NodeMCU 1.0 (ESP-12E Module)), and its respective COM port.
In order to discover the UID of an RFID/NFC tag, the first step is to copy the code and open it with Arduino IDE. After that, click the Upload button on the Arduino IDE to upload the code to the ESP8266. Then, open the Serial Monitor and tap the RFID/NFC tag on the RFID-RC522 module. Finally, the UID will be displayed on the Serial Monitor.
After obtaining the UID:
- Replace the value of byte authorizedUID[4] = {0xFF, 0xFF, 0xFF, 0xFF}; with the UID in line 18 of the code. For example, byte authorizedUID[4] = {0x3A, 0xC9, 0x6A, 0xCB};
- Upload the code to ESP8266 again
- Tap an RFID/NFC tag on the RFID-RC522 module
- Check out the output on the Serial Monitor
- Tap a different RFID/NFC tag on the RFID-RC522 module.
- Check the output on the Serial Monitor.
※ NOTE THAT:
- To facilitate testing, the active time is set to two seconds, however it should be increased for practical use/demonstration.
- Installation of the MFRC522 library is required. Please refer to ESP8266 - RFID/NFC RC522 tutorial for more information.