ESP8266 - RFID
This tutorial instructs you how to use RFID/NFC with ESP8266. The RFID/NFC system consists of two components: a reader and a tag. Two of the most popular RFID/NFC readers are the RC522 and PN532. This tutorial will uses the RC522 RFID/NFC reader, which is cheap and easy to use.
The RC522 RFID/NFC reader can:
- Obtains the UID of an RFID/NFC tag
- Changes the UID of an RFID/NFC tag (only if the tag supports this)
- Stores data onto an RFID/NFC tag
- Retrieves data from an RFID/NFC tag
This tutorial focuses on:
- How to connect RC522 module to ESP8266
- How to program ESP8266 to communicate with RC522 module to read UID of RFID tag.
Hardware Preparation
1 | × | ESP8266 NodeMCU | |
1 | × | Micro USB Cable | |
1 | × | RFID/NFC RC522 Kit (reader + tags) | |
1 | × | RFID Key Fob | |
1 | × | Jumper Wires | |
1 | × | (Optional) 5V Power Adapter for ESP8266 | |
1 | × | (Optional) ESP8266 Screw Terminal Adapter |
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Overview of RFID-RC522 Module
RFID-RC522 Module Pinout
The RFID-RC522 has eight pins, some of which are common and the others are shared among three communication modes: SPI, I2C, and UART. Only one communication mode can be used at a time. The pins are:
- GND: must be connected to GND (0V)
- VCC: must be connected to VCC (3.3)
- RST: is a pin for resetting and power-down. When this pin goes low, hard power-down is enabled. On the rising edge, the module is reset.
- IRQ: is an interrupt pin that can alert the microcontroller when an RFID tag is in its vicinity.
- MISO/SCL/TX: acts as MISO when SPI interface is enabled, acts as SCL when I2C interface is enabled and acts as TX when UART interface is enabled.
- MOSI: acts as MOSI when SPI interface is enabled.
- SCK: acts as SCK when SPI interface is enabled.
- SS/SDA/RX: acts as SS when SPI interface is enabled, acts as SDA when I2C interface is enabled and acts as RX when UART interface is enabled.
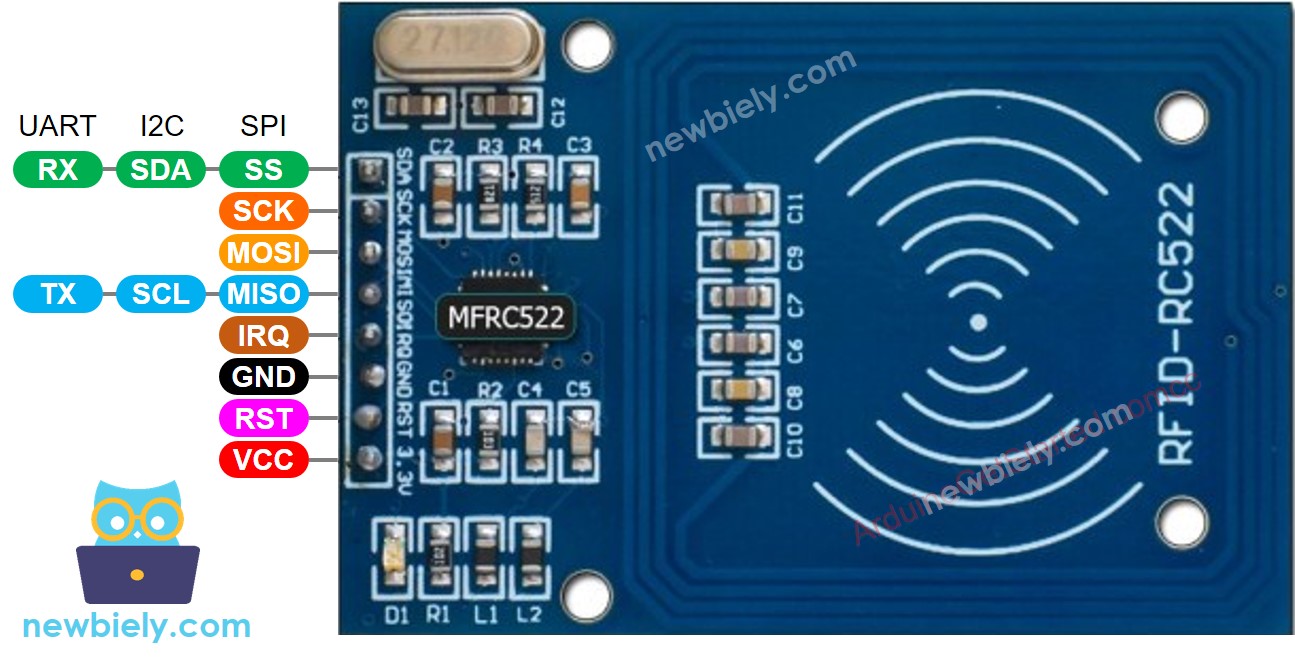
※ NOTE THAT:
- The arrangement of pins may differ depending on the manufacturer. ALWAYS follow the labels printed on the module. The illustration above is the pinout of the module from DIYables manufacturer.
- Do not connect the VCC pin to the 5V pin. This could damage your module.
- The MFRC522 library only supports SPI mode, so this guide will only use SPI communication.
How RFID/NFC Works
RFID/NFC is composed of two parts: reader and tag:
- The reader is made up of a radio frequency module and an antenna that produces a high frequency electromagnetic field.
- The tag is usually a passive device, not requiring a power source. It has a microchip to store and process data, as well as an antenna to receive and transmit signals. The tag is used to keep information such as a UID (Unique ID) and other data.
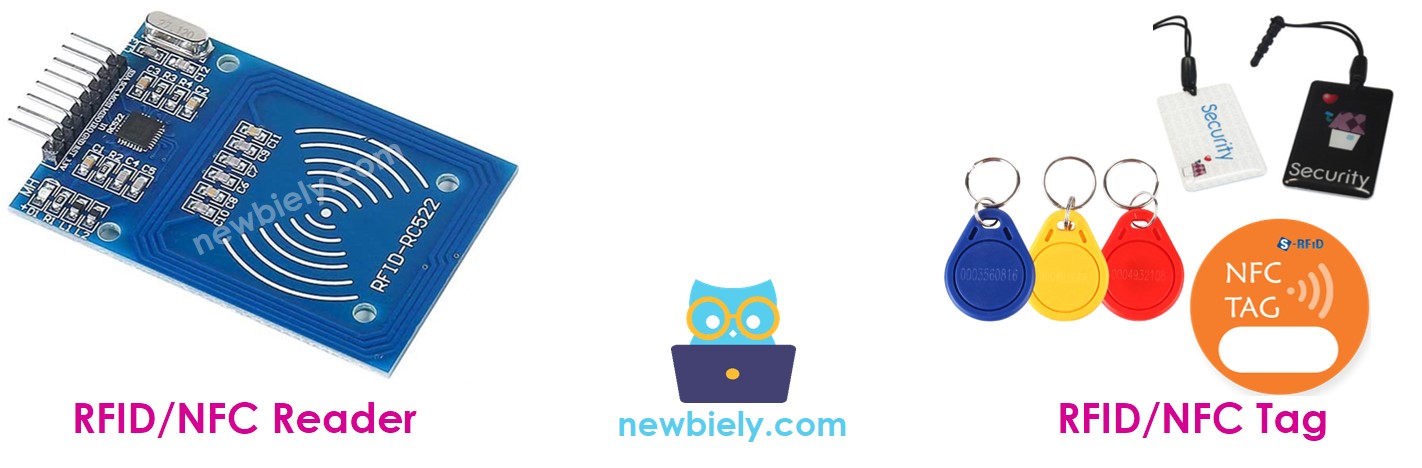
The tag must be near the reader in order to access the information it contains. The process of reading is as follows:
- The reader produces an electromagnetic field, which causes electrons to flow through the tag's antenna and power the chip.
- The chip inside the tag then responds by sending the requested information back to the reader in the form of a radio signal.
- The reader detects the signal and converts it into data.
- ESP8266 reads the data from the reader.
Wiring Diagram between RFID-RC522 Module and ESP8266
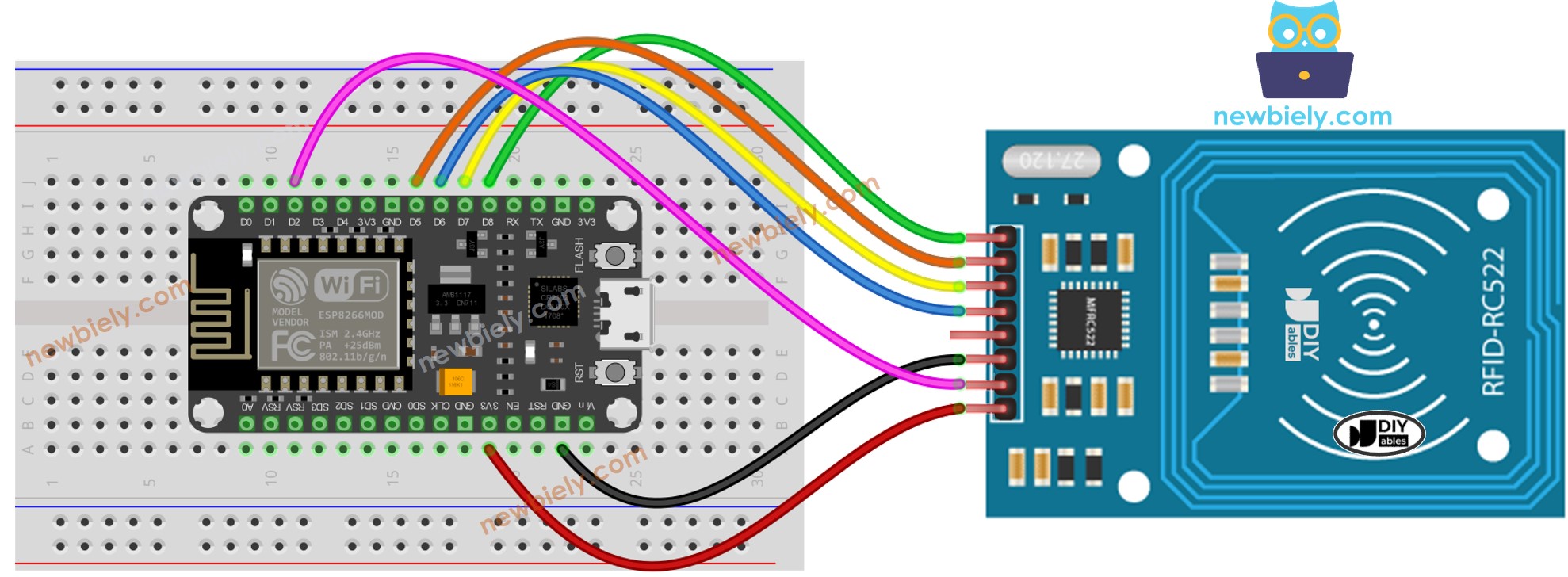
This image is created using Fritzing. Click to enlarge image
See more in ESP8266's pinout and how to supply power to the ESP8266 and other components.
If you use male-to-female jumper wires, you can directly connect ESP8266 to the RFID-RC522 module. However, if you use male-to-male jumper wires, you must connect ESP8266 to the RFID-RC522 module through a breadboard.
Wiring table of RFID/NFC RC522 Module and ESP8266
RFID/NFC RC522 | ESP8266 |
---|---|
SS | → D8 (SPI CS/SS) |
SCK | → D5 (SPI CLK) |
MOSI | → D7 (SPI MOSI) |
MISO | → D6 (SPI MISO) |
IRQ | not connected |
GND | → GND |
RST | → D2 |
VCC | → 3.3V |
ESP8266 RFID/NFC Code
Detailed Instructions
To get started with ESP8266 on Arduino IDE, follow these steps:
- Check out the how to setup environment for ESP8266 on Arduino IDE tutorial if this is your first time using ESP8266.
- Wire the components as shown in the diagram.
- Connect the ESP8266 board to your computer using a USB cable.
- Open Arduino IDE on your computer.
- Choose the correct ESP8266 board, such as (e.g. NodeMCU 1.0 (ESP-12E Module)), and its respective COM port.
- Click to the Libraries icon on the left bar of the Arduino IDE.
- Search for “MFRC522” and locate the library provided by GithubCommunity.
- Press the Install button to install the MFRC522 library.
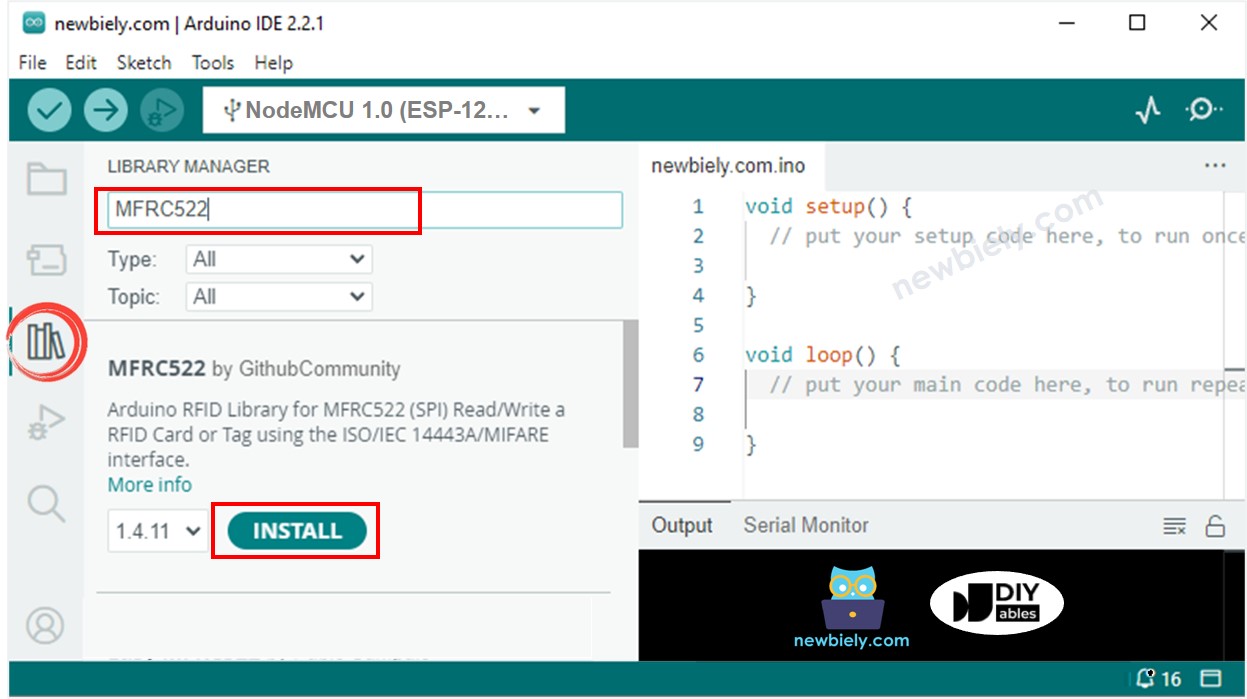
- Copy the code and open it with the Arduino IDE.
- Click the Upload button on the Arduino IDE to compile and upload the code to the ESP8266.
- Open the Serial Monitor.
- Tap some RFID/NFC tags to the RFID-RC522 module.
- Check the UID on the Serial Monitor.