ESP8266 - Rotary Encoder
This tutorial instructs you how to use the incremental rotary encoder with ESP8266. Here's what we'll cover:
- How the rotary encoder works
- The difference between a rotary encoder and a potentiometer (another type of knob)
- How to physically connect the rotary encoder to an ESP8266
- How to write code for the ESP8266 to read the direction and position from the rotary encoder.
Hardware Preparation
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables .
Overview of Rotary Encoder
A rotary encoder is a kind of knob that can change turning into an electric signal. It tells you how much something has twisted and where it's positioned. There are two main types:
- Incremental encoder: This one uses quick signals to measure how much something has moved.
- Absolute encoder: This type gives a special code for each position, which helps know exactly where something is, even if the power stops.
This tutorial focuses on the incremental encoder.
Rotary Encoder Module Pinout
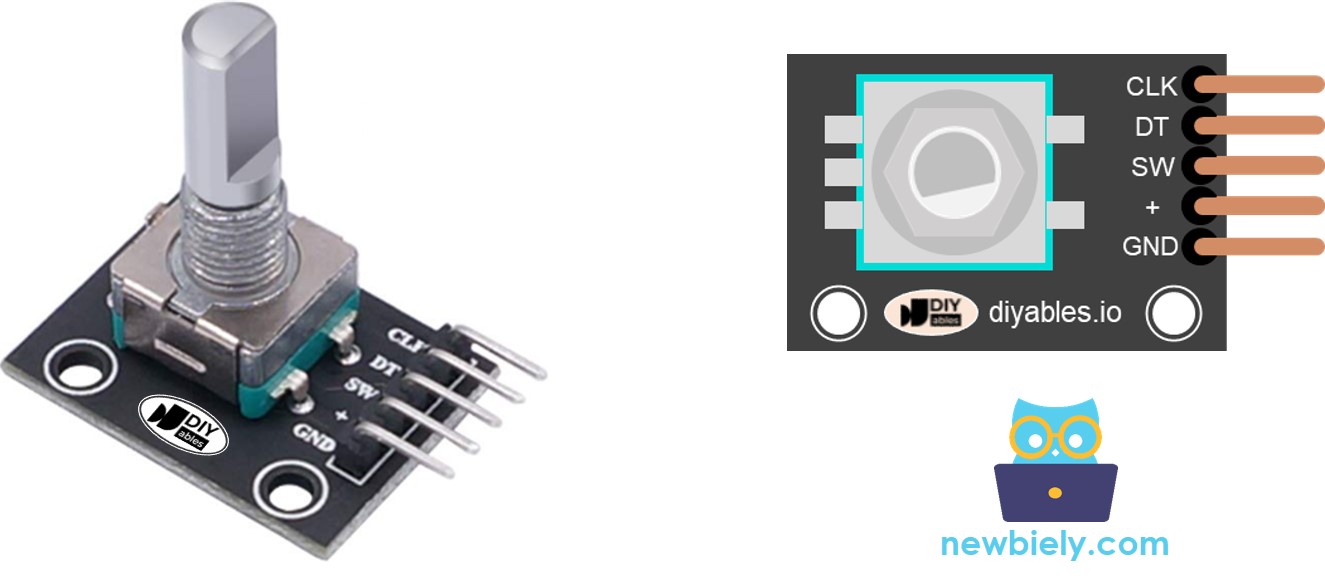
A rotary encoder module has 4 pins:
- CLK pin (Output A): is the main pulse that tells us how much rotation has occurred. Whenever you turn the knob by one detent (click) in either direction, the CLK pin outputs a signal that is a completes a full cycle (LOW → HIGH → LOW).
- DT pin (Output B): acts like the CLK pin but outputs a signal lags behind CLK signal by 90 degrees. It helps us figure out the direction of rotation (clockwise or anticlockwise).
- SW pin: is the output from the pushbutton inside the encoder. It’s normally open. If we use a pull-up resistor in this pin, the SW pin will be HIGH when the knob is not pressed, and LOW when it is pressed.
- VCC pin (+): needs to be connected to VCC (between 3.3 and 5 volts)
- GND pin: needs to be connected to GND (0V)
Rotary Encoder vs Potentiometer
You may confuse the rotary encoder with the potentiometer. but they are distinct components. Here's a comparison between them:
- Rotary encoder is like the modern version of potentiometer, but they can do more things.
- Rotary encoder can spin around in a full circle without stopping, while potentiometer can only turn about three-quarters of the circle.
- Rotary encoder outputs pulses, while potentiometer outputs the analog voltage.
- Rotary encoder is handy when you just need to figure out how much the knob has moved, not exactly where it is. Potentiometer is useful when you really need to know exactly where a knob is.
How Rotary Encoder Works
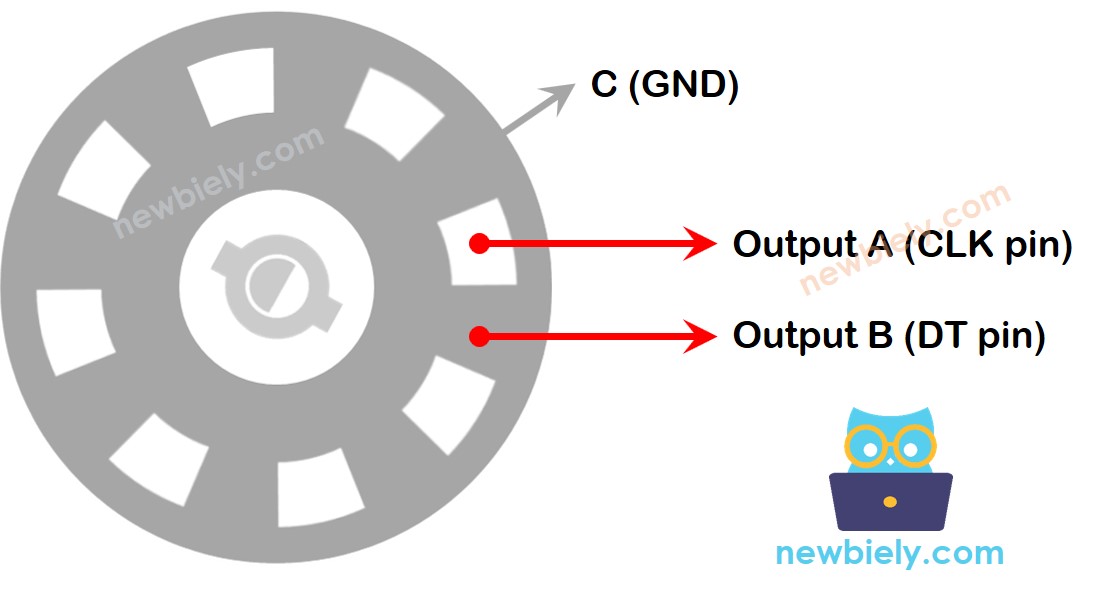
Inside the encoder, there's a disc with slots connected to a pin called C, which is like a shared ground. There are two more pins, A and B.
- When you twist the knob, pins A and B touch the shared ground pin C, but in a certain order depending on which way you turn the knob (clockwise or counter-clockwise).
- These touches create two signals. They're a bit different in timing because one pin touches the ground before the other. Two signals are 90 degrees out of sync with each other. This is called quadrature encoding.
- When you turn the knob in clockwise direction, pin A touches the ground before pin B. When you turn the knob to the counterclockwise direction, pin B touches the ground before pin A.
- By monitoring when each pin touches or leaves the ground, we can figure out which way the knob is turning. We do this by checking what happens to pin B when pin A changes.
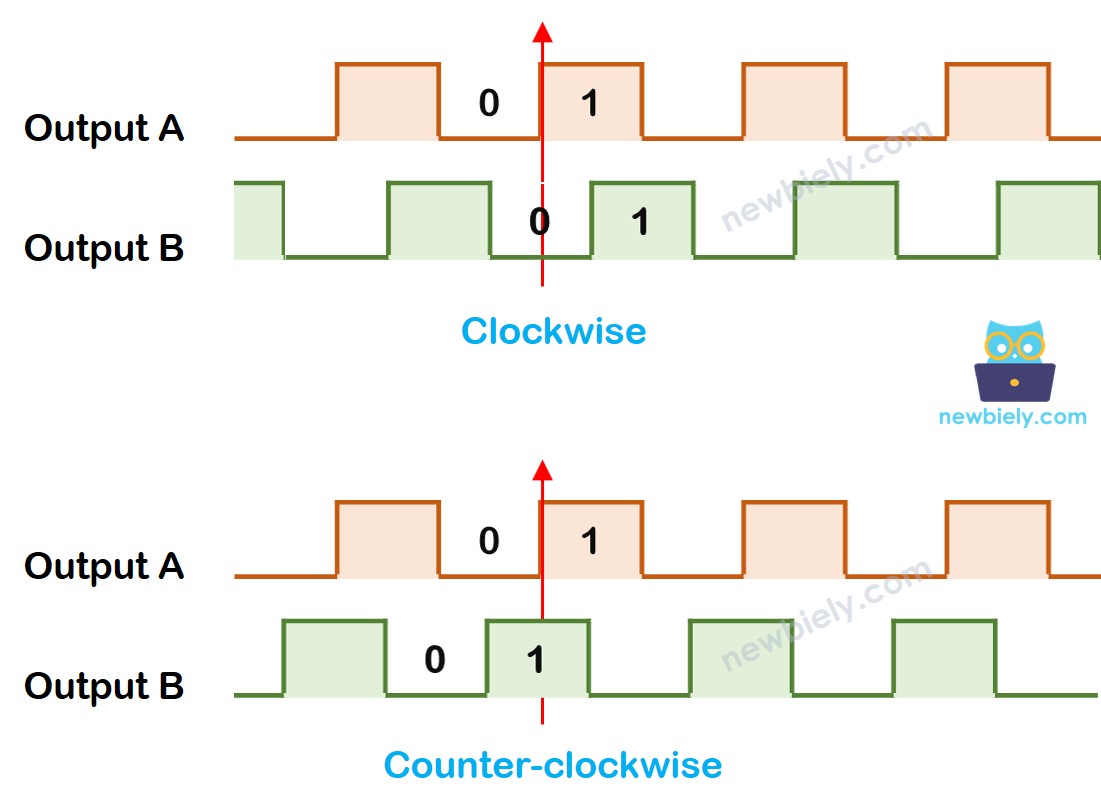
When A changes states from LOW to HIGH:
- If B is LOW, the knob is turned clockwise.
- If B is HIGH, the knob is turned counter-clockwise.
※ NOTE THAT:
Pin A and B are connected to CLK and DT pins. However, depending on the manufacturers, the order may be different. The codes provided below are tested with the rotary encoder from DIYables
How To Program For Rotary Encoder
- Check the signal from CLK pin
- If the state changes from LOW to HIGH, check the state of the DT pin.
- If the state of the DT pin is HIGH, the knob is turned in the counter-clockwise direction, increase the counter by 1
- If the state of the DT pin is LOW, the knob is turned in the clockwise direction, decrease the counter by 1
Wiring Diagram
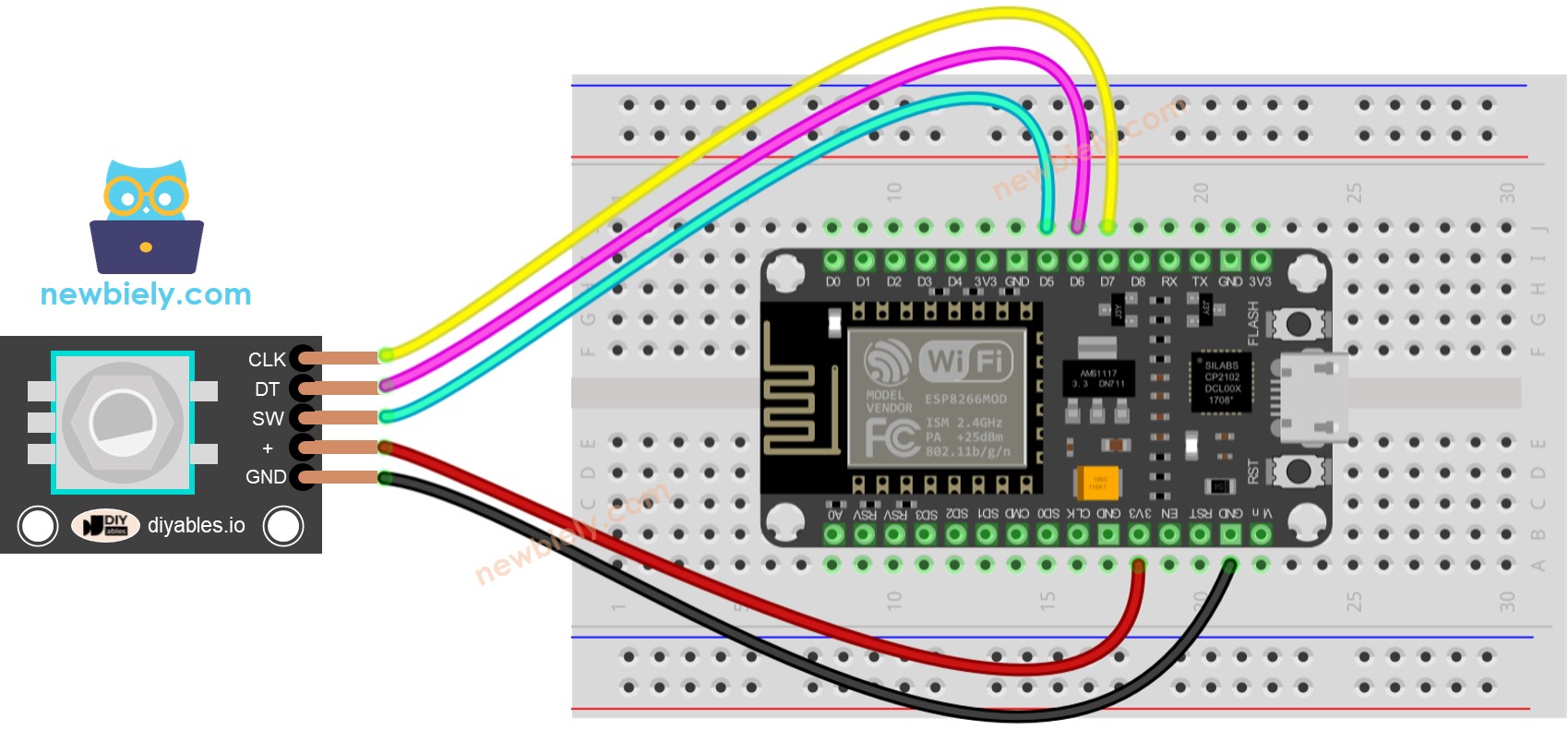
This image is created using Fritzing. Click to enlarge image
See more in ESP8266's pinout and how to supply power to the ESP8266 and other components.
ESP8266 Code – Rotary Encoder
The below ESP8266 code does:
- Detects the direction and amount of rotation of the encoder.
- If detecting the knob turned by one detent (click) in clockwise direction, increase the counter by one.
- If detecting the knob turned by one detent (click) in anticlockwise direction, decrease the counter by one.
- Detects if the button is pressed.
To simplify the code for button debouncing, the ezButton library is used.
Detailed Instructions
To get started with ESP8266 on Arduino IDE, follow these steps:
- Check out the how to setup environment for ESP8266 on Arduino IDE tutorial if this is your first time using ESP8266.
- Wire the components as shown in the diagram.
- Connect the ESP8266 board to your computer using a USB cable.
- Open Arduino IDE on your computer.
- Choose the correct ESP8266 board, such as (e.g. NodeMCU 1.0 (ESP-12E Module)), and its respective COM port.
- Install ezButton library on Arduino IDE. See How To
- Copy the above code and open with Arduino IDE
- Click Upload button on Arduino IDE to upload code to ESP8266
- Turn the knob in clockwise, then anticlockwise
- Press the knob
- Check out the result on the Serial Monitor.
Code Explanation
Check out the line-by-line comments in the code
ESP8266 Code – Rotary Encoder with Interrupt
In the previous code, repeatedly checking the pin's status using polling can waste resources and might not count correctly if other tasks take a long time. Interrupts provide a solution by avoiding continuous checking, allowing the ESP8266 to handle different tasks without missing any events.
Here’s an ESP8266 example of how to read a rotary encoder with interrupts.
Now, As you twist the knob, you'll notice information appearing on the Serial Monitor, much like what you saw in the earlier code.
※ NOTE THAT:
- You might come across tutorials on other websites that use two interrupts for a single encoder, but this is unnecessary and wasteful. Just one interrupt is sufficient.
- It's important to use the volatile keyword for global variables used in the interrupt. Neglecting this could lead to unexpected issues.
- Keep the code within the interrupt as straightforward as you can. Avoid using Serial.print() or Serial.println() inside the interrupt.
ESP8266 Rotary Encoder Application
With Rotary Encoder, we can do the following applications but not limit:
- ESP8266 - Rotary Encoder controls Position of Sevo Motor
- ESP8266 - Rotary Encoder controls Brightness of LED
- ESP8266 - Rotary Encoder controls Speed of Stepper Motor