ESP8266 - Soil Moisture Sensor
This tutorial instructs you how to use a moisture sensor with ESP8266. Specifically, we will look at:
- The differences between a resistive and capacitive moisture sensor
- How to program ESP8266 to read the value of the moisture sensor using ESP8266
- How to use ESP8266 to calibrate the moisture sensor
- How ESP8266 determines if the soil is wet or dry
Hardware Preparation
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables .
Buy Note: Many soil moisture sensors available in the market are unreliable, regardless of their version. We strongly recommend buying the sensor with TLC555I Chip from the DIYables brand using the link provided above. We tested it, and it worked reliably.
Overview of Soil Moisture Sensor Sensor
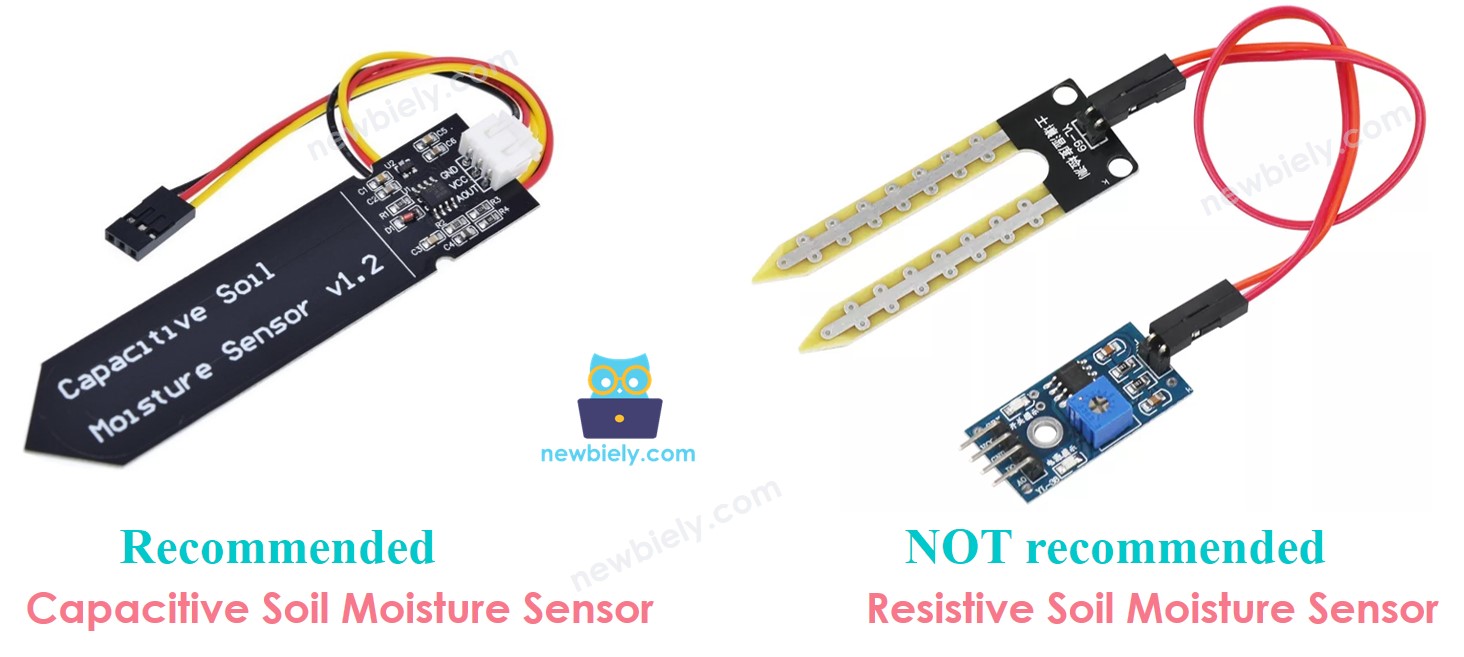
There are two types of moisture sensors: the resistive moisture sensor and the capacitive moisture sensor.
Both sensors offer soil moisture information. However, their methods of operation differ. We suggest using the capacitive moisture sensor due to the following:
- The resistive soil moisture sensor is prone to corrosion over time as electrical current passes between its probes, leading to electrochemical corrosion.
- The capacitive soil moisture sensor, on the other hand, corrodes much slower than the resistive soil moisture sensor. That is because its electrodes are not exposed and is comparatively resistant to corrosion.
This is an illustration of a resistive soil moisture sensor that has been damaged by corrosion.
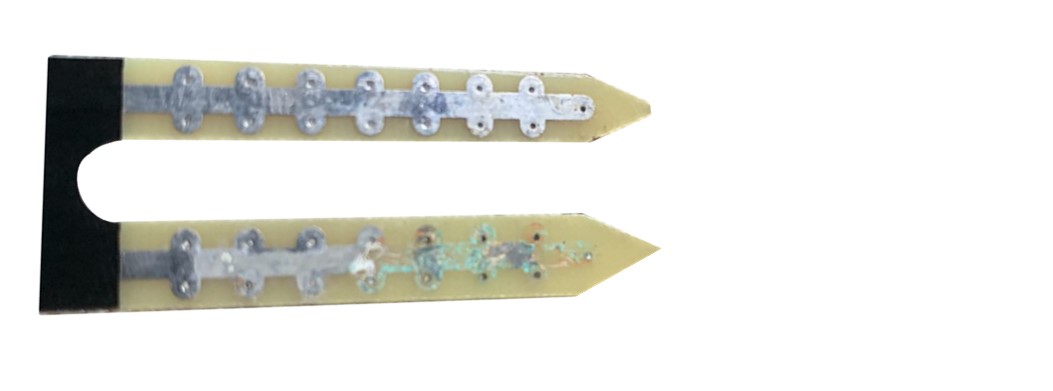
The remainder of this tutorial will be utilizing the capacitive soil moisture sensor.
Capacitive Soil Moisture Sensor Pinout
The capacitive soil moisture sensor has three pins:
- GND pin: This must be connected to GND (0V).
- VCC pin: This must be connected to VCC (5V or 3.3v).
- AOUT pin: This is the analog signal output pin which produces a voltage that is proportional to the soil moisture level. This should be connected to an analog input pin on an ESP8266.
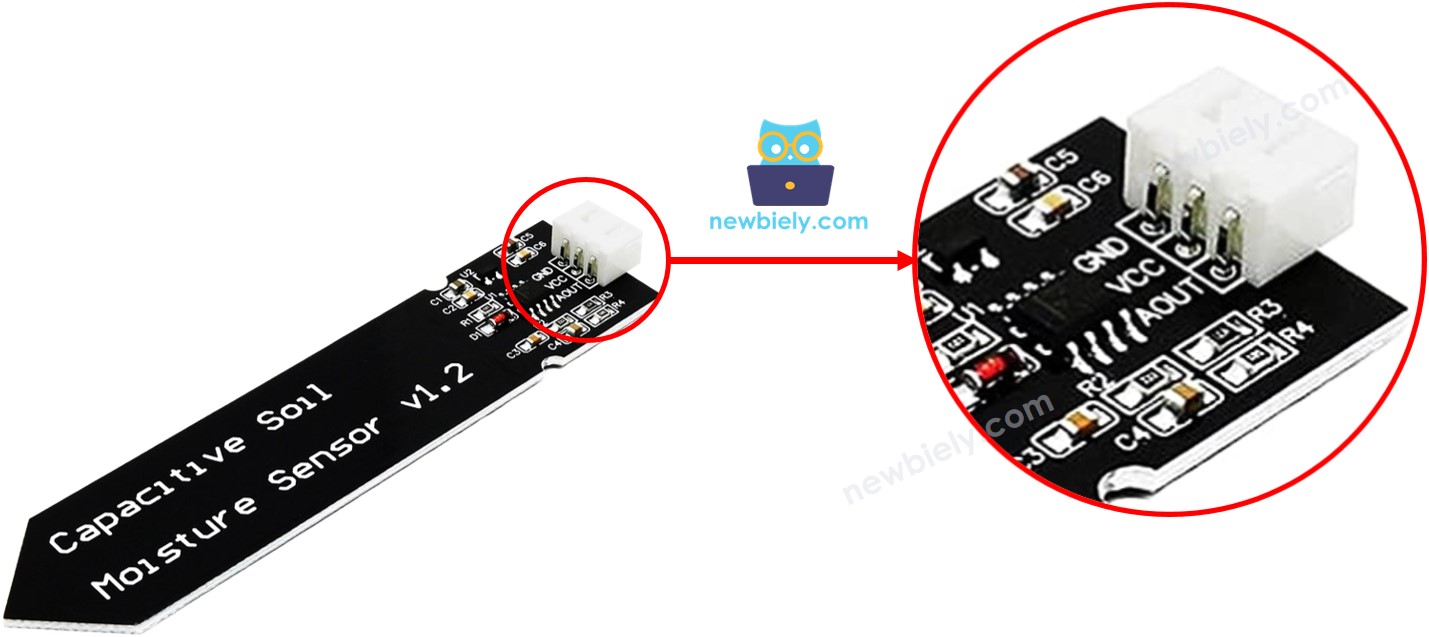
How It Works
The amount of water present in the soil has a inverse proportion to the voltage level of the AOUT pin.
Wiring Diagram
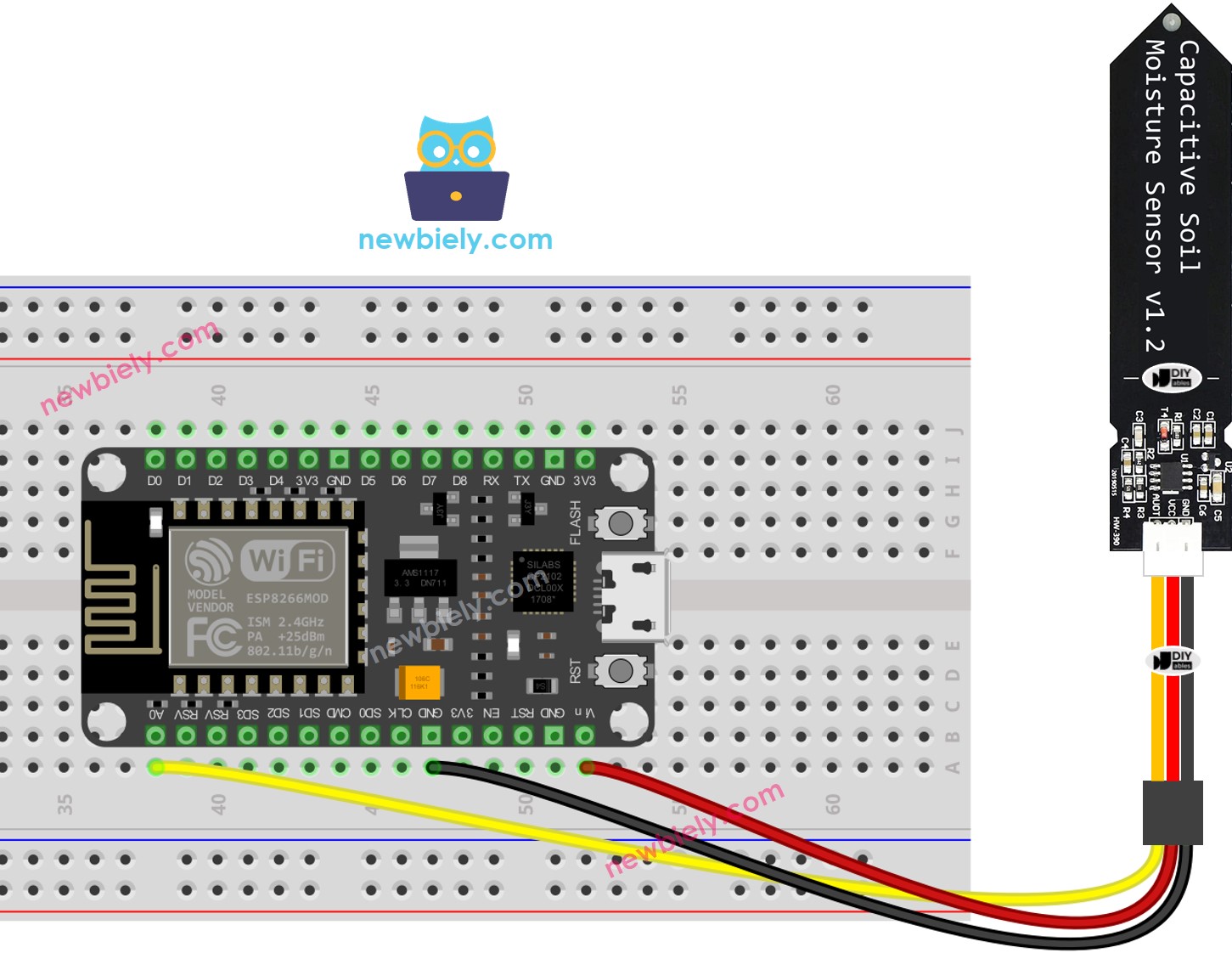
This image is created using Fritzing. Click to enlarge image
See more in ESP8266's pinout and how to supply power to the ESP8266 and other components.
ESP8266 Code for reading value from soil moisture sensor
Detailed Instructions
To get started with ESP8266 on Arduino IDE, follow these steps:
- Check out the how to setup environment for ESP8266 on Arduino IDE tutorial if this is your first time using ESP8266.
- Wire the components as shown in the diagram.
- Connect the ESP8266 board to your computer using a USB cable.
- Open Arduino IDE on your computer.
- Choose the correct ESP8266 board, such as (e.g. NodeMCU 1.0 (ESP-12E Module)), and its respective COM port.
- Copy the code and open it with Arduino IDE.
- Click the Upload button on Arduino IDE to compile and upload the code to ESP8266.
- Bury the sensor in soil, then pour water into the soil. Or slowly submerge it into a cup of salt water.
- Check out the result on Serial Monitor. It looks like the below:
※ NOTE THAT:
- Don't use pure water for testing because it doesn't conduct electricity, so it won't change the sensor readings.
- The sensor readings will never be zero. It's usual for the values to be between 500 and 600, although this might change based on factors such as how deep the sensor is placed, the type of soil or water, and the voltage of the power supply.
- Avoid burying the circuit part (found on top of the sensor) in soil or water, as it could harm the sensor.
Calibration for Capacitive Soil Moisture Sensor
The value obtained from the moisture sensor is not absolute. It is based on the soil's composition and water content. In order to accurately determine a boundary between wet and dry, we must perform calibration.
Instructions for Calibration:
- Execute the code on ESP8266
- Place the moisture sensor in the soil
- Gradually add water to the soil
- Monitor the Serial Monitor
- Note the value when the soil changes from dry to wet. This is referred to as the THRESHOLD.
ESP8266 code determines if the soil is wet or dry
Once the calibration is done, update the THRESHOLD value that you noted down in the code below. This code will be used to decide if the soil is wet or dry.
The output seen on the Serial Monitor.
※ NOTE THAT:
This tutorial uses the analogRead() function to get data from an ADC (Analog-to-Digital Converter) that's connected to a sensor or another part. The ESP8266's ADC works well for projects where you don't need very precise readings. But remember, the ESP8266's ADC isn't very accurate for detailed measurements. If your project needs to be very precise, you might want to use a separate ADC like the ADS1115 with the ESP8266, or use Arduino like the Arduino Uno R4 WiFi, which has a more reliable ADC.