ESP8266 - Irrigation
This tutorial instructs you how to use ESP8266, soil moisture sensor, relay, and pump to build an automatic irrigation system for the garden with. Specifically:
- ESP8266 will be used to control the relay in order to switch on the pump when the soil moisture is dry.
- When the soil moisture is wet, ESP8266 will be used to control the relay to turn the pump off.
Hardware Preparation
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables .
Buy Note: Many soil moisture sensors available in the market are unreliable, regardless of their version. We strongly recommend buying the sensor with TLC555I Chip from the DIYables brand using the link provided above. We tested it, and it worked reliably.
Overview of soil moisture sensor and Pump
If you are not familiar with pump and soil moisture sensor (including pinout, functioning, programming, etc.), the following tutorials will be helpful:
- ESP8266 - Soil Moisture Sensor tutorial
- ESP8266 - Controls Pump tutorial
Wiring Diagram
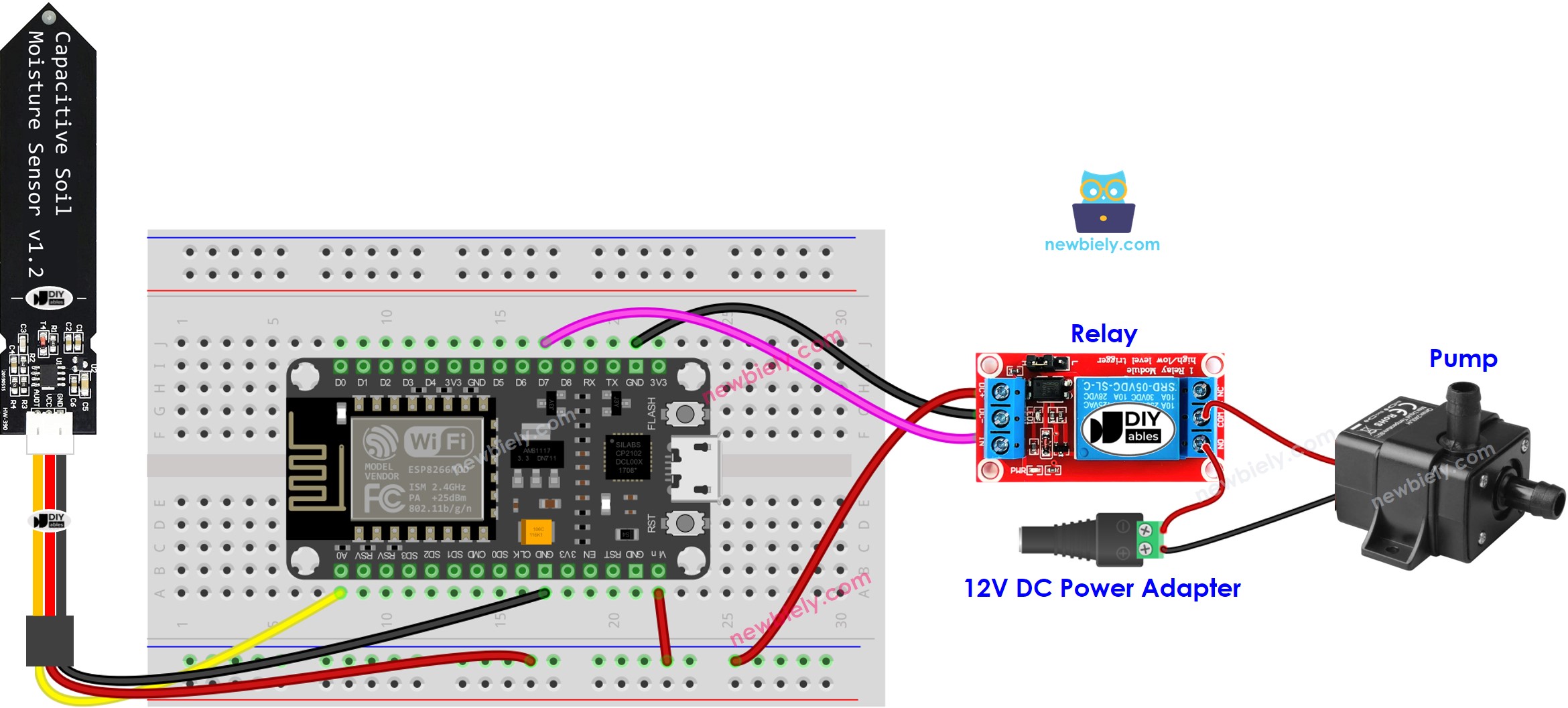
This image is created using Fritzing. Click to enlarge image
See more in ESP8266's pinout and how to supply power to the ESP8266 and other components.
ESP8266 Code
Detailed Instructions
To get started with ESP8266 on Arduino IDE, follow these steps:
- Check out the how to setup environment for ESP8266 on Arduino IDE tutorial if this is your first time using ESP8266.
- Wire the components as shown in the diagram.
- Connect the ESP8266 board to your computer using a USB cable.
- Open Arduino IDE on your computer.
- Choose the correct ESP8266 board, such as (e.g. NodeMCU 1.0 (ESP-12E Module)), and its respective COM port.
- Perform calibration to identify the wet-dry THRESHOLD, as outlined in ESP8266 - Calibrates Soil Moisture Sensor.
- Insert the calibrated THRESHOLD value into the code.
- Launch Serial Monitor on Arduino IDE.
- Upload the code to ESP8266.
- View the result on Serial Monitor.
Code Explanation
Check out the line-by-line explanation contained in the comments of the source code!