ESP8266 - Potentiometer Relay
This tutorial instructs you how to use ESP8266 and potentiometer to control relay. In detail:
- ESP8266 checks if the potentiometer's analog value is higher or lower than a threshold and act accordingly - turning the relay on or off
- ESP8266 checks if the potentiometer's output voltage is above or below a threshold and act accordingly - turning the relay on or off
By attaching a relay to a light bulb, LED strip, motor, or actuator.. We can then use the ESP8266 and potentiometer to control the light bulb, LED strip, motor, or actuator...
Hardware Preparation
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables .
Overview of Relay and Potentiometer
If you are unfamiliar with relay and potentiometer (including pinout, functionality, and programming), the following tutorials can help:
Wiring Diagram
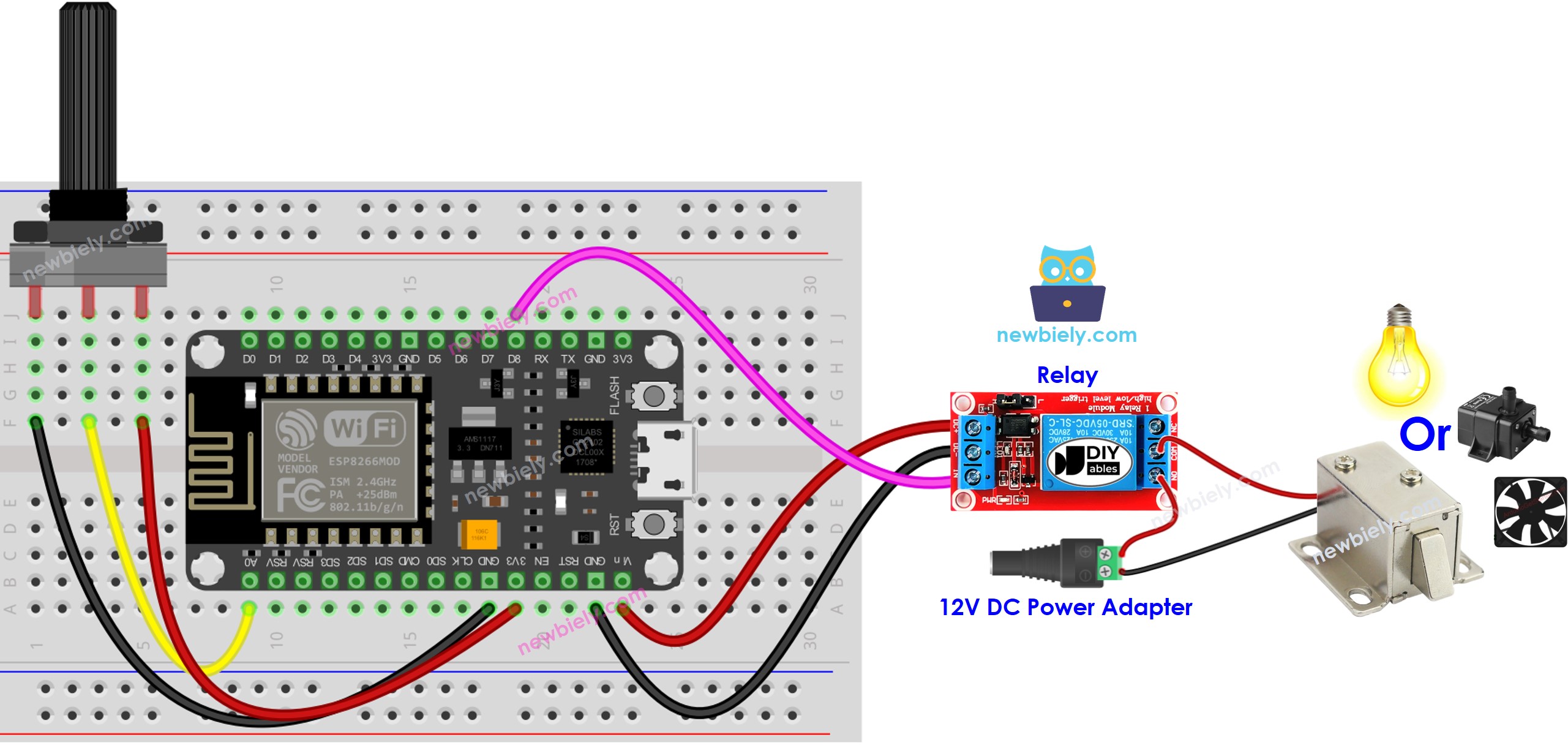
This image is created using Fritzing. Click to enlarge image
See more in ESP8266's pinout and how to supply power to the ESP8266 and other components.
ESP8266 Code - Analog Threshold
Detailed Instructions
To get started with ESP8266 on Arduino IDE, follow these steps:
- Check out the how to setup environment for ESP8266 on Arduino IDE tutorial if this is your first time using ESP8266.
- Wire the components as shown in the diagram.
- Connect the ESP8266 board to your computer using a USB cable.
- Open Arduino IDE on your computer.
- Choose the correct ESP8266 board, such as (e.g. NodeMCU 1.0 (ESP-12E Module)), and its respective COM port.
- Connect your ESP8266 to the computer using a USB cable.
- Launch the Arduino IDE, select the correct board and port.
- Copy the code above and open it in the Arduino IDE.
- Click the Upload button in the Arduino IDE to compile and upload the code to the ESP8266.
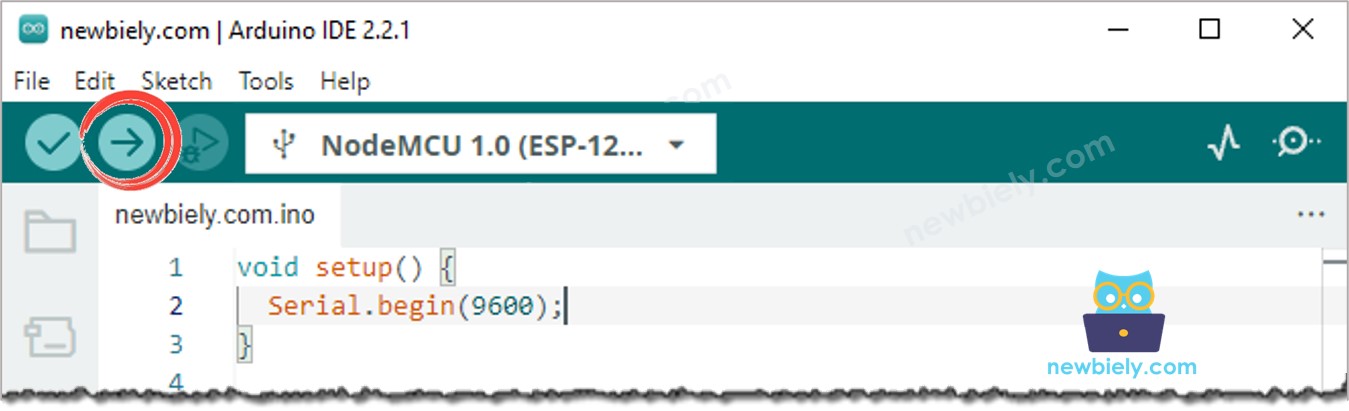
- Turn the potentiometer
- Check out the alteration in the relay's condition
Code Explanation
Check out the line-by-line explanation contained in the comments of the source code!
ESP8266 Code - Voltage Threshold
The analog value of a potentiometer is changed to a voltage value. This voltage is then compared to a voltage threshold, which causes the relay to be triggered.