ESP8266 - Motion Sensor MP3 Player
This tutorial instructs you on how to use ESP8266, an HC-SR501 motion sensor, and an MP3 player to trigger the playback of a recorded audio file upon detecting motion. This project is versatile and can be tailored for applications like automatically delivering recorded audio instructions or warnings whenever a human presence is detected.
Hardware Preparation
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables.
Overview of MP3 Player and Motion Sensor
If you do not know about MP3 player and motion sensor (pinout, how it works, how to program ...), learn about them in the following tutorials:
Wiring Diagram
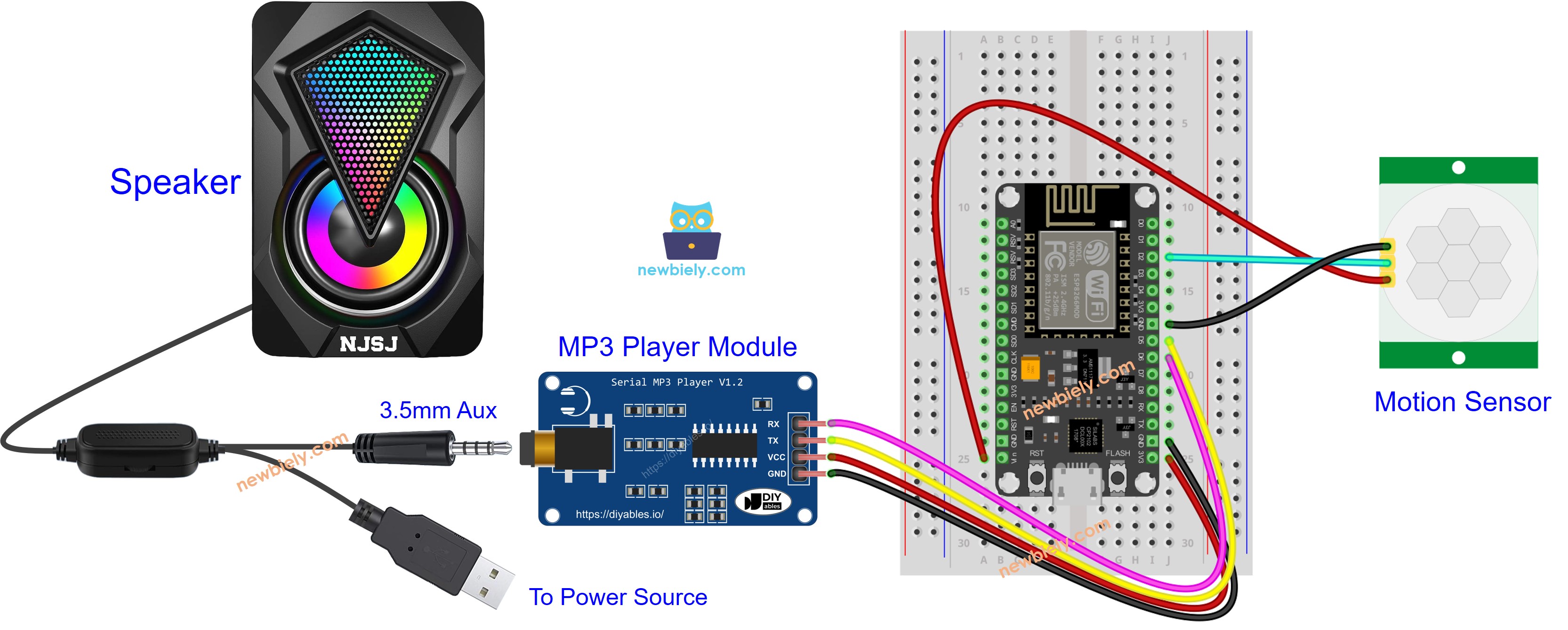
This image is created using Fritzing. Click to enlarge image
See more in ESP8266's pinout and how to supply power to the ESP8266 and other components.
Preparation
- Pre-store the recorded mp3 file that we want to play to the micro SD Card.
- Insert the micro SD Card to the MP3 player module
- Connect the MP3 player module to ESP8266
- Connect the speaker to the MP3 player module to a
- Connect the speaker to a power source.
- Connect the motion sensor to the ESP8266.
- Do setting for motion sensor as below table
Time Delay Adjuster | Screw it in anti-clockwise direction fully. |
Detection Range Adjuster | Screw it in clockwise direction fully. |
Repeat Trigger Selector | Put jumper as shown on the image. |
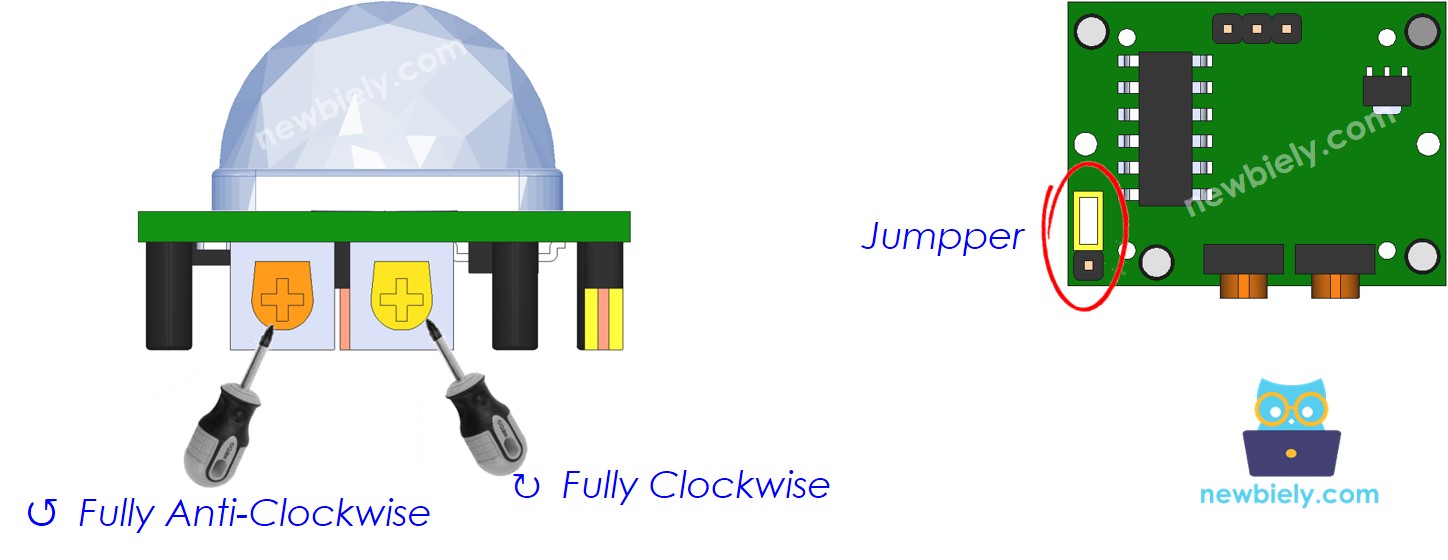
ESP8266 Code - Motion Sensor Controls MP3 Player
Detailed Instructions
To get started with ESP8266 on Arduino IDE, follow these steps:
- Check out the how to setup environment for ESP8266 on Arduino IDE tutorial if this is your first time using ESP8266.
- Wire the components as shown in the diagram.
- Connect the ESP8266 board to your computer using a USB cable.
- Open Arduino IDE on your computer.
- Choose the correct ESP8266 board, such as (e.g. NodeMCU 1.0 (ESP-12E Module)), and its respective COM port.
- Connect ESP8266 to PC via USB cable
- Open Arduino IDE, select the right board and port
- Copy the above code and open with Arduino IDE
- Click Upload button on Arduino IDE to upload code to ESP8266
- Move your hand in front of sensor
- Check out the audio from MP3 player