ESP8266 - RS232
In this tutorial, we'll delve into using RS232 communication with ESP8266, covering:
- Connecting ESP8266 to the TTL to RS232 module for improved communication.
- Programming ESP8266 to read data from the TTL to RS232 module effectively.
- Instructing ESP8266 to send data to the TTL to RS232 module.
The tutorial includes guidance for both Hardware Serial and SoftwareSerial.
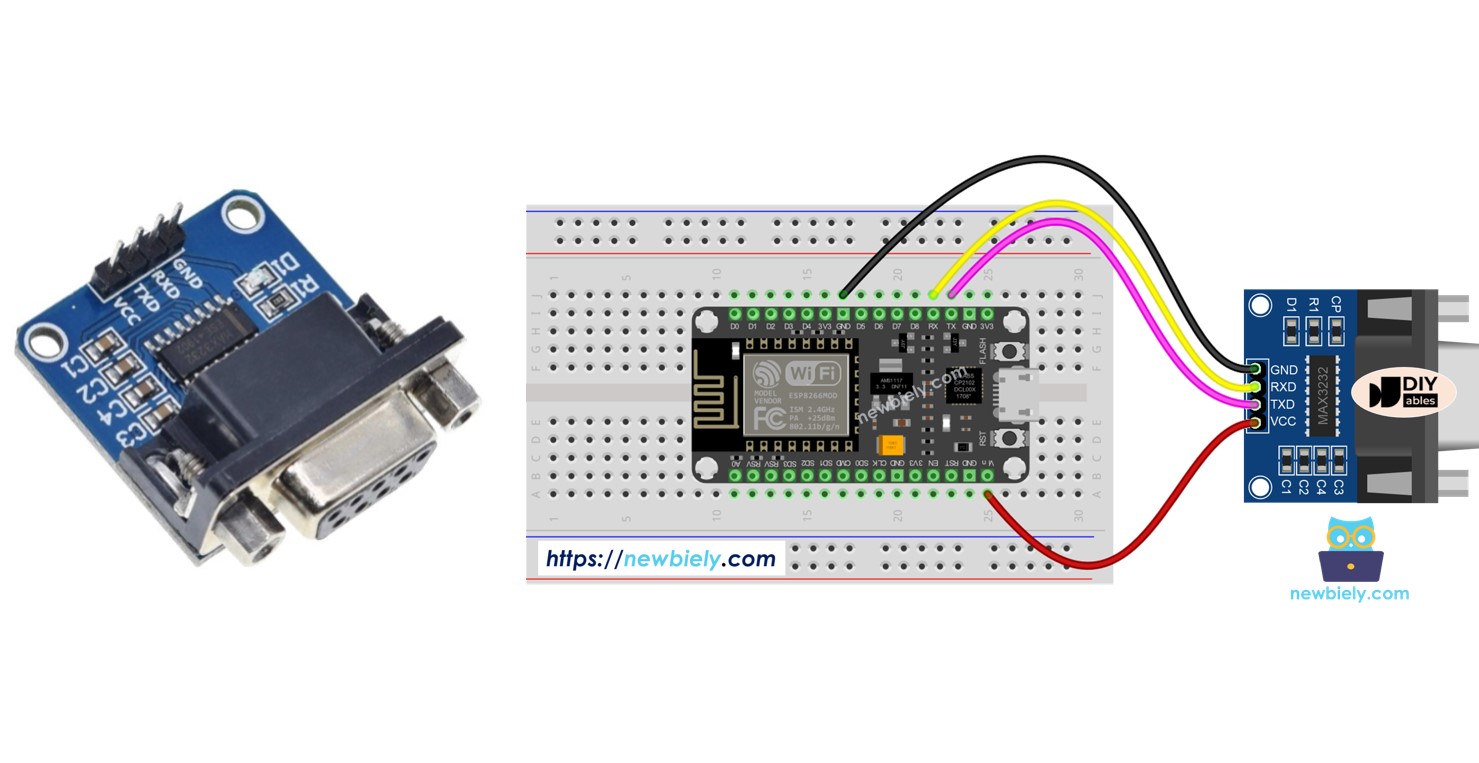
Hardware Preparation
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables .
Overview of TTL to RS232 Module
When you're working with serial stuff on ESP8266 and you're throwing around Serial.print(), Serial.read(), and Serial.write(), what's happening is your ESP8266 is sending data out through its TX pin and catching data coming in on its RX pin. Now, here's the deal - those signals on TX and RX are at TTL level, which is cool and all, but they don't travel too far. So, if you're planning on exchanging data over long distances, you've got to upgrade that signal.
Enter the TTL to RS232 module. This nifty gadget turns your TTL signal into RS232 and back again. It's like magic for making your communication reach far and wide.
Pinout
The RS232 to TTL module has two interfaces:
- The TTL interface (connnected to ESP8266) includes 4 pins
- VCC pin: power pin, needs to be connected to VCC (5V)
- GND pin: power pin, needs to be connected to GND (0V)
- RXD pin: data pin, needs to be connected a RX pin of ESP8266
- TXD pin: data pin, needs to be connected a TX pin of ESP8266
- The RS232 interface: DB9 female D-Sub connector, connect this to the serial device
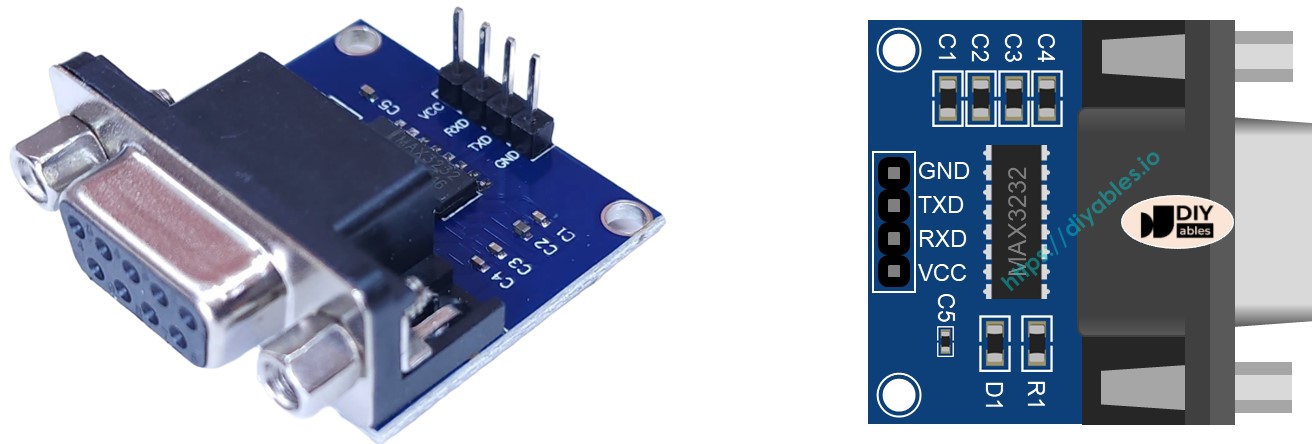
Wiring Diagram
- Wiring diagram if using hardware serial
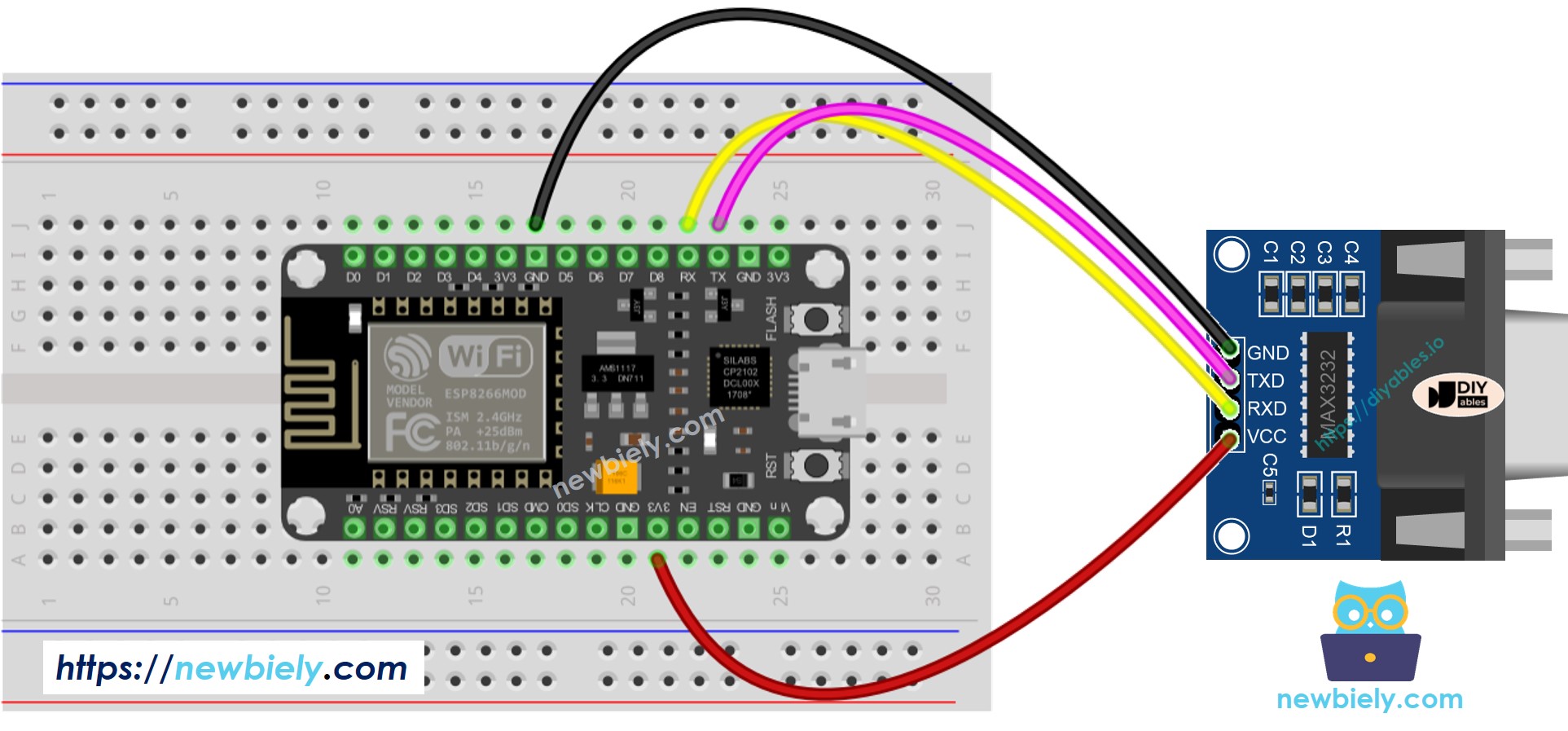
This image is created using Fritzing. Click to enlarge image
- Wiring diagram if using software serial
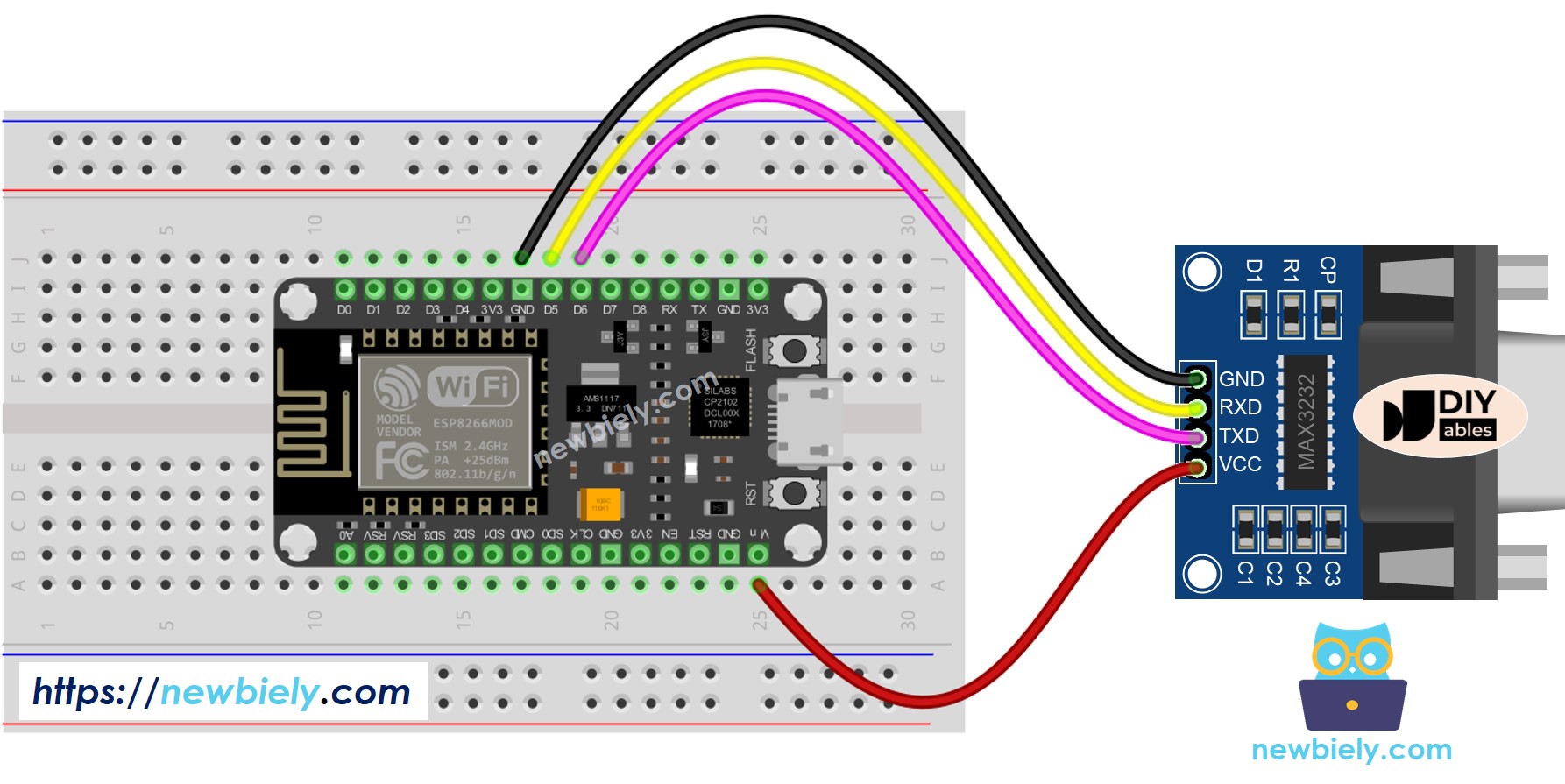
This image is created using Fritzing. Click to enlarge image
See more in ESP8266's pinout and how to supply power to the ESP8266 and other components.
How To Program ESP8266 to use the RS232 module
- Initializes the Serial interface:
- If you use SoftwareSerial, you need to include the library and declare a SoftwareSerial object:
- To read data come from RS232, you can use the following functions:
- To write data to RS232, you can use the following functions:
- And more functions to use with RS232 in Serial reference
ESP8266 Code for Hardware Serial
ESP8266 Code for Software Serial
Testing
You can do a test by sending data from your PC to ESP8266 via RS232 and vice versa. To do it, follow the below steps:
- Connect ESP8266 to your PC via RS232-to-USB cable as below:
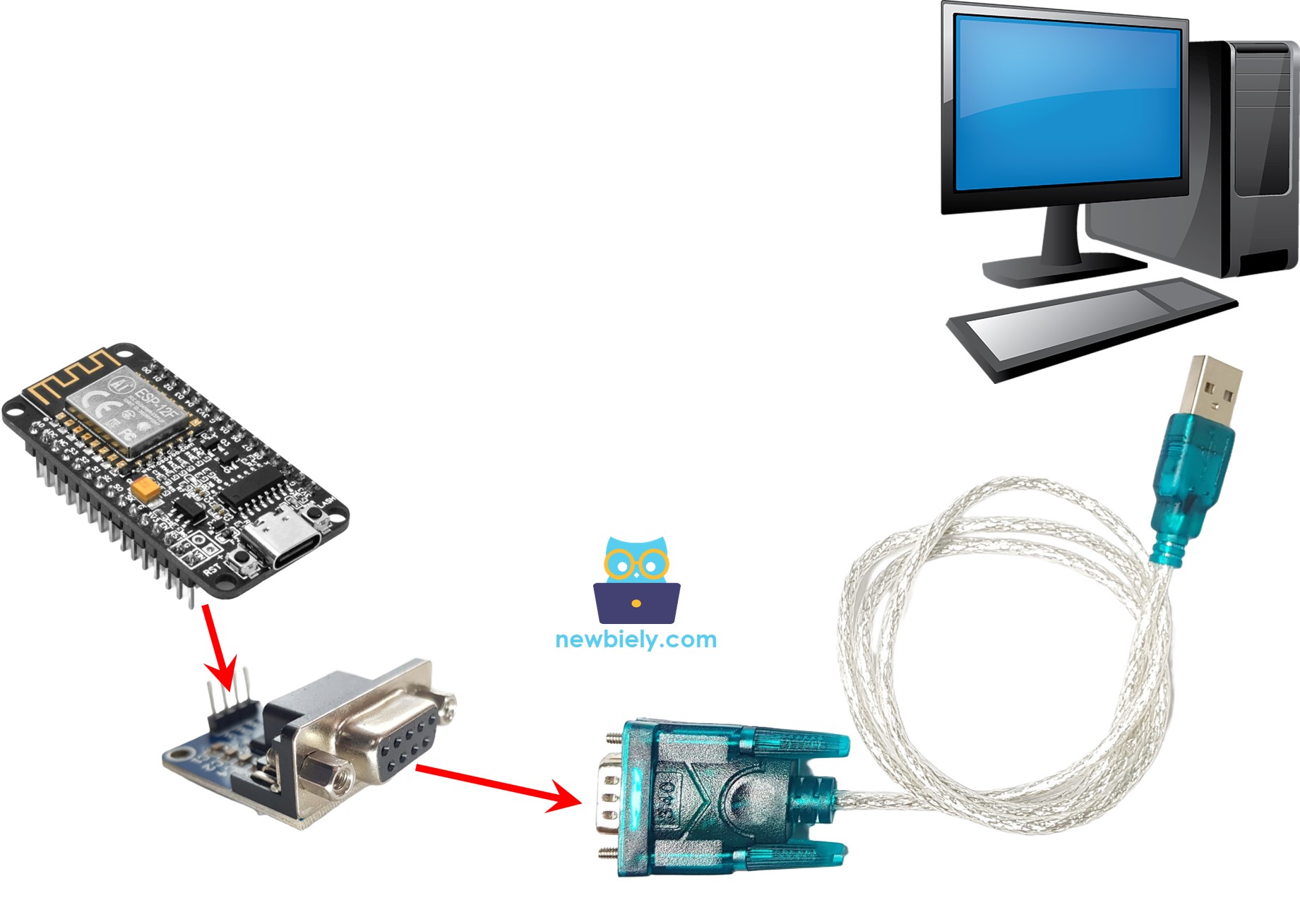
- Open the Serial Terminal Program and configure the Serial parameters (COM port, baurate...)
- Type some data from the Serial Termial to send it to ESP8266.
- If successful, you will see the echo data on the Serial Terminal.