ESP8266 - DC Motor
This tutorial instructs you how to use ESP8266 to control DC Motor. In detail, we will learn:
- How DC motor works
- How to use ESP8266 and L298N driver to control a DC motor
- How to program ESP8266 to control the speed and direction of a DC motor
- How to program ESP8266 to control two DC motors simultaneously
Hardware Preparation
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables.
Overview of DC Motor
DC Motor Pinout
A DC Motor has two wires, the positive one usually being red and the negative one black.
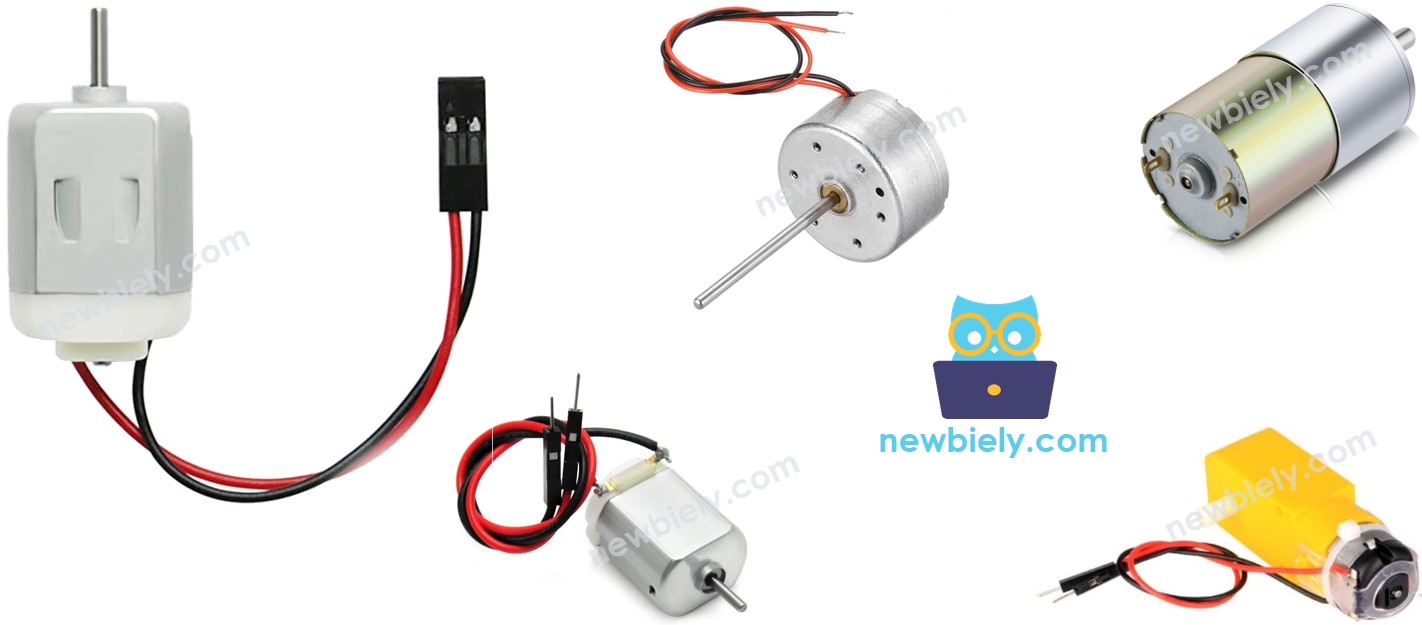
How It Works
When purchasing a DC motor, it is essential to identify the voltage at which it operates. For instance, let us consider a 12V DC motor.
When you power the 12V DC motor by a 12V power source:
- Connect 12V and GND to the positive wire and negative wire, respectively: the DC motor rotates at maximum speed in the clockwise direction
- Connect 12V and GND to the negative wire and positive wire, respectively: the DC motor rotates at maximum speed in the anti-clockwise direction
As previously mentioned, by swapping the power pole between two wires of the DC motor, the direction of rotation is reversed. This is a method used to control the direction of the DC motor, not manually but through programming.
If the power supplied to DC motors is less than 12V, the motor will still rotate but not at its highest speed. This implies that by adjusting the voltage of the power source, we can alter the speed of the DC motor. Nevertheless, this approach is not commonly used due to the complexity of controlling the voltage of the power source. Therefore, the voltage of the power source is kept constant and the speed of the DC motor is regulated through a PWM signal. The greater the duty cycle of the PWM, the faster the DC motor rotates.
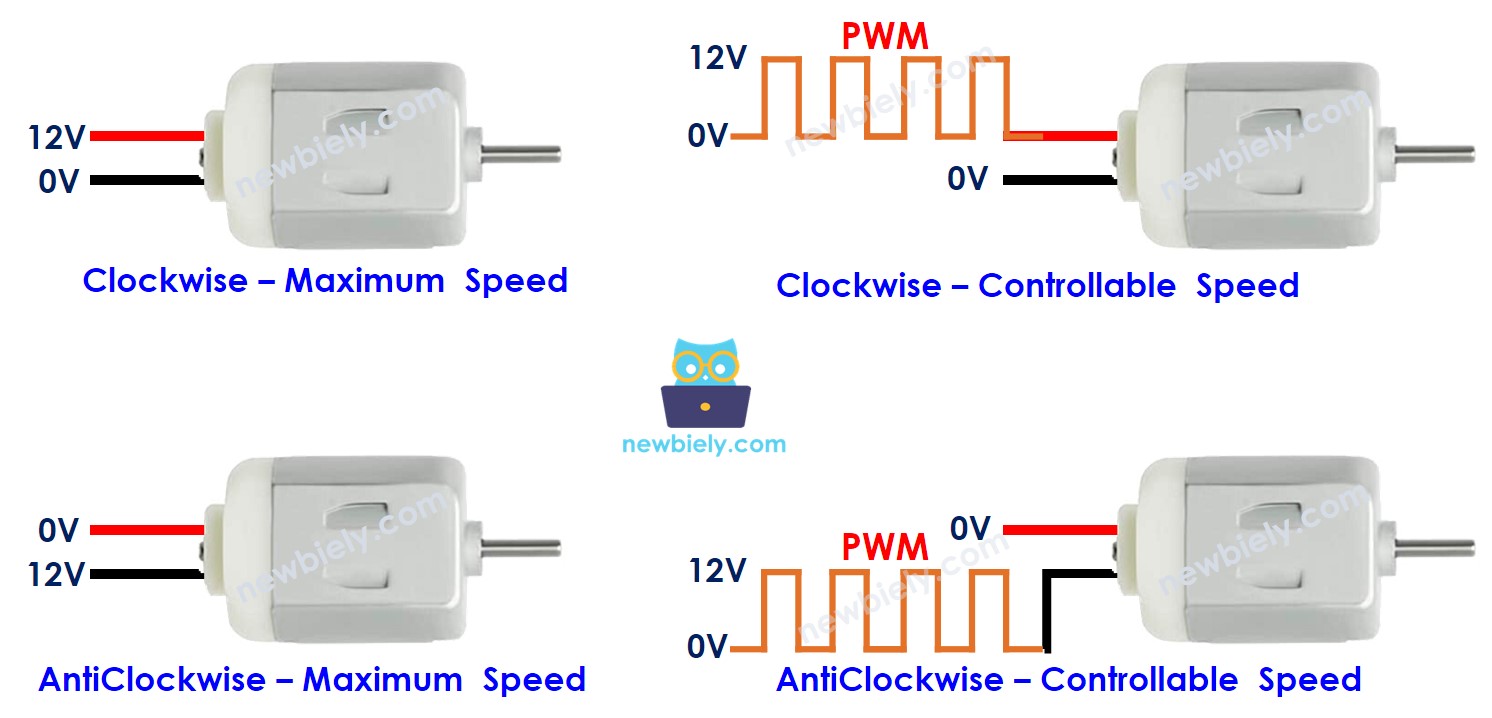
How to control DC motor using ESP8266
Controlling a DC motor involves two components: speed and direction. ESP8266 can generate a PWM signal, but it has low voltage and current, so it cannot be used to control the DC motor. To bridge the gap between ESP8266 and the DC motor, a hardware driver is needed. This driver performs two tasks:
- Amplifying the PWM signal from ESP8266 in terms of current and voltage for speed control
- Receiving the control signal from ESP8266 to switch the polarity of the power supply for direction control.
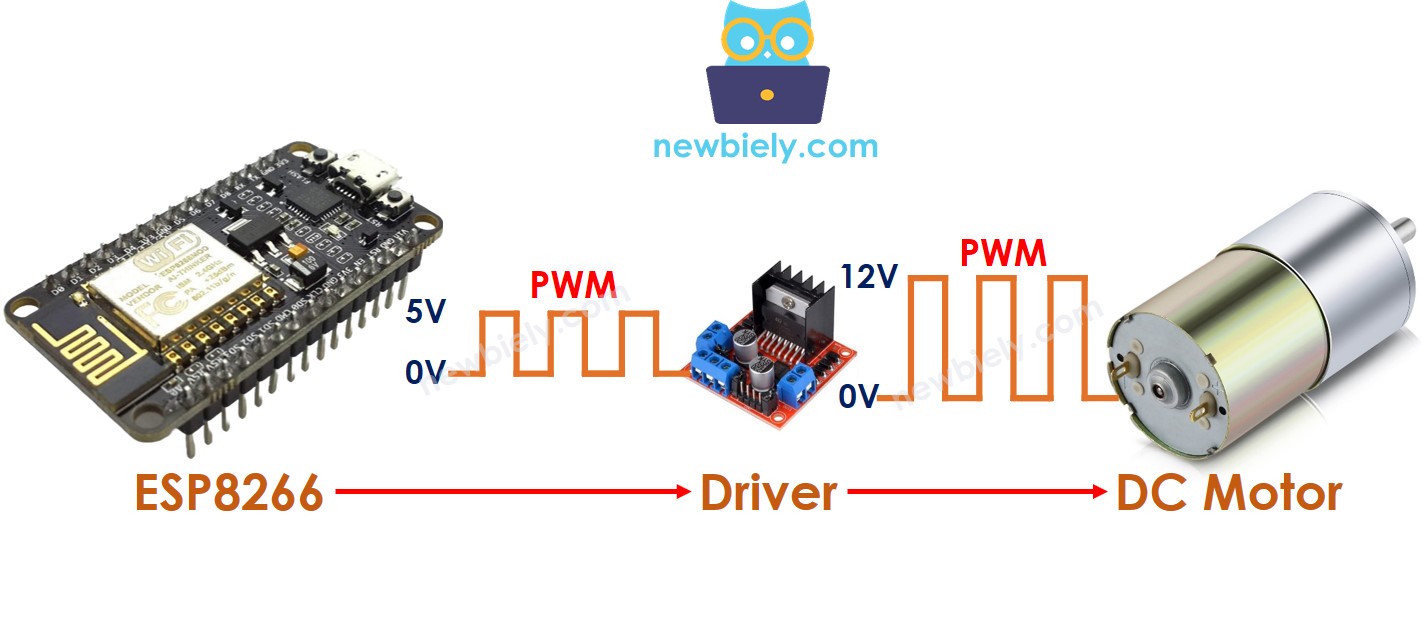
※ NOTE THAT:
- This tutorial can be used for any DC motor. 12V DC motor is just one example.
- When you are controlling a 5V DC motor, even though the ESP8266 pin outputs 5V (which is the same as the DC motor voltage), you still need to have a driver in between the ESP8266 and the DC motor because the ESP8266 pin does not provide enough current for the DC motor.
There are numerous types of chips, and modules such as L293D and L298N can be used as DC motor drivers. In this tutorial, we will be utilizing the L298N driver.
Overview of L298N Driver
The L298N Driver can be used to manage DC motors and stepper motors. This tutorial instructs you how to use it to control a DC motor.
L298N Driver Pinout
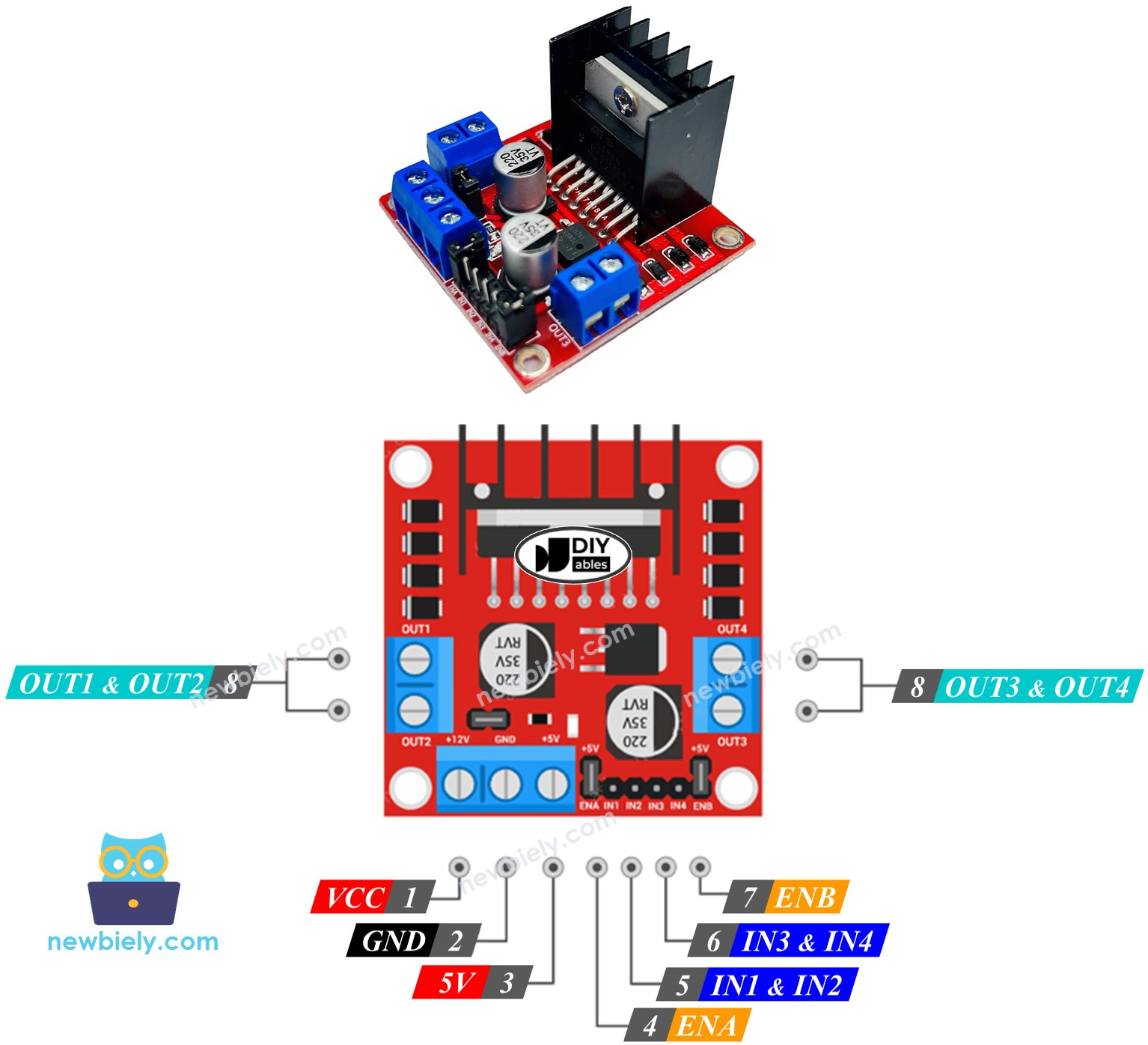
The L298N Driver is capable of controlling two DC motors independently simultaneously, referred to as motor A and motor B. This Driver has 13 pins in total.
The common pins for both motors:
- VCC pin: This pin supplies power for the motor, with a voltage range of 5 to 35V.
- GND pin: This is a common ground pin, which needs to be connected to 0V (GND).
- 5V pin: This pin supplies power for the L298N module, and can be supplied by 5V from an ESP8266.
Motor A pins (Channel A):
- ENA pins: This pin can be used to control the speed of Motor A. By removing the jumper and connecting it to a PWM input, we can adjust the speed of Motor A.
- IN1 & IN2 pins: These pins are used to control the direction of Motor A's rotation. When one of these pins is set to HIGH and the other is set to LOW, Motor A will spin. If both pins are either HIGH or LOW, Motor A will not move.
- OUT1 & OUT2 pins: These pins are connected to Motor A.
Motor B pins (Channel B):
- These pins are used to provide power to Motor B.
- ENB pins: This pin can be connected to a PWM input to control the speed of Motor B. Removing the jumper will enable this.
- IN3 & IN4 pins: These pins are used to determine the spinning direction of Motor B. When one of them is HIGH and the other is LOW, Motor B will spin. If both the inputs are either HIGH or LOW, the Motor B will stop.
- OUT3 & OUT4 pins: These pins are connected to Motor B and provide power to it.
The L298N driver has two input powers:
- One for the DC motor, with a voltage range of 5 to 35V, connected to the VCC and GND pins.
- One for the internal operation of the L298N module, with a voltage range of 5 to 7V, connected to the 5V and GND pins.
Remove all the jumpers from the L298N driver for the sake of simplicity. This is necessary as the jumpers are used for advanced uses or other purposes.
We can control two DC motors independently simultaneously by using an ESP8266 and an L298N Driver. To regulate each motor, we only require three pins from the ESP8266.
※ NOTE THAT:
The remainder of this tutorial will focus on controlling a DC motor using channel A. Similar steps can be taken to control the other DC motor.
How To Control the Speed of DC Motor via L298N Driver
It is easy to regulate the speed of a DC motor by producing a PWM signal to the ENA pin of L298N. This can be done by:
- Connecting an ESP8266 pin to the ENA of L298N
- Generating a PWM signal to the ENA pin by using analogWrite() function. The L298N Driver will amplify the PWM signal to the DC motor.
The speed can range from 0 to 255. When the speed is 0, the motor will cease to move. At a speed of 255, the motor will spin at its maximum velocity.
How To Control the Direction of DC Motor via L298N Driver
The rotation of a motor can be adjusted by providing a logic HIGH/LOW to IN1 and IN2 pins. The table below shows how to control the direction in both channels.
IN1 pin | IN2 pin | Direction |
---|---|---|
LOW | LOW | Motor A stops |
HIGH | HIGH | Motor A stops |
HIGH | LOW | Motor A spins Clockwise |
LOW | HIGH | Motor A spins Anti-Clockwise |
Accordingly:
- ESP8266 code for rotating in a clockwise direction.
- ESP8266 code for rotating in a anti-clockwise direction.
※ NOTE THAT:
The rotation of the DC motor can be reversed by connecting the OUT1 and OUT2 pins in an opposite manner.
How To Stop DC Motor Spinning
There are two methods for stopping a DC motor:
- Reduce the speed to 0
- Sets both IN1 and IN2 pins to the same value, either LOW or HIGH.
- Or
How to control a DC motor using L298N driver.
Wiring Diagram
It is essential to take out all three jumpers from the L298N module prior to connecting the wires.
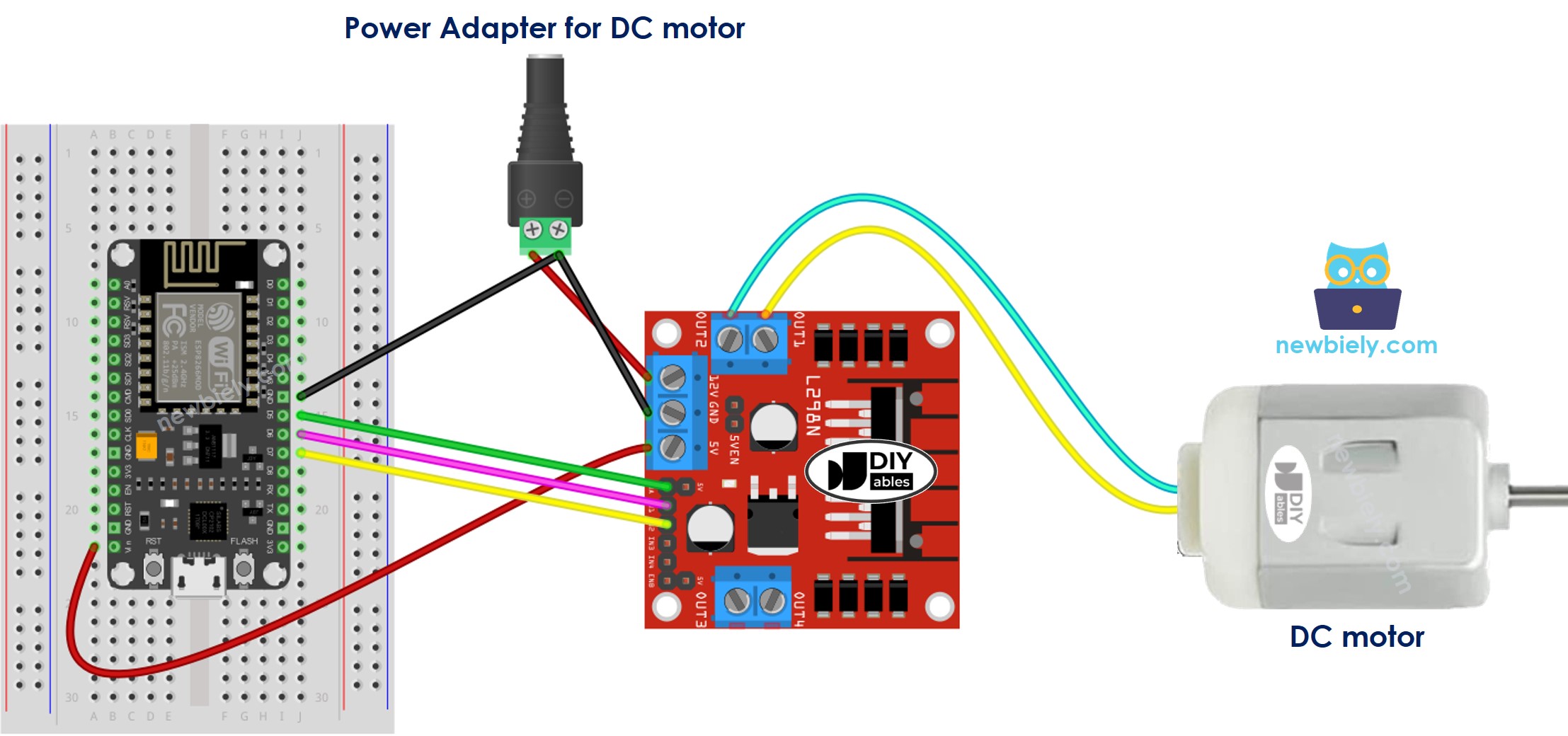
This image is created using Fritzing. Click to enlarge image
See more in ESP8266's pinout and how to supply power to the ESP8266 and other components.
ESP8266 Code
The following code does:
- Increases the speed of a DC motor
- Alters the direction
- Decreases the speed of a DC motor
- Halts the motor
Detailed Instructions
To get started with ESP8266 on Arduino IDE, follow these steps:
- Check out the how to setup environment for ESP8266 on Arduino IDE tutorial if this is your first time using ESP8266.
- Wire the components as shown in the diagram.
- Connect the ESP8266 board to your computer using a USB cable.
- Open Arduino IDE on your computer.
- Choose the correct ESP8266 board, such as (e.g. NodeMCU 1.0 (ESP-12E Module)), and its respective COM port.
- Take out all three jumpers from the L298N module.
- Copy the code and open it in the Arduino IDE.
- Click the Upload button on the Arduino IDE to compile and upload the code to the ESP8266.
- You should observe:
- The DC motor will speed up and then rotate at its maximum speed for 1 second.
- The direction of the DC motor will be changed.
- The DC motor will spin at its maximum speed in the opposite direction for 1 second.
- The DC motor will slow down.
- The DC motor will stop for 1 second.
- This process will repeat itself.
※ NOTE THAT:
This tutorial instructs you how to control the speed of a DC motor in relation to its maximum speed. To control the absolute speed (in revolutions per second), we will need to use a PID controller and an encoder. Controlling the absolute speed of the DC motor will be discussed in a separate tutorial.
How to Control two DC Motors using L298N Driver
Coming soon!