ESP8266 - Feedback Actuator
In a prior tutorial, we discussed the linear actuator without feedback. This tutorial instructs you the feedback linear actuator (also known as the feedback linear actuator). This type of linear actuator provides information to identify the position of its stroke and control it. Specifically, we will be learning:
- The functioning of a feedback linear actuator
- How to determine the position of the feedback linear actuator (in millimeters)
- How to control the position of a linear actuator
Hardware Preparation
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables .
Overview of Feedback Linear Actuator
A linear actuator with feedback is one that has a signal to identify its location and manage it. A potentiometer is used to generate the voltage value in relation to the stroke's position as the feedback.
Feedback Linear Actuator Pinout
A Feedback Linear Actuator has 5 wires:
- Actuator Positive wire: This wire is used to control the linear actuator by applying high voltage (12V, 24V, 48V...).
- Actuator Negative wire: This wire is used to control the linear actuator by applying high voltage (12V, 24V, 48V...).
- 5V wire: this wire is used for the feedback potentiometer. It should be connected to 5V or 3.3V
- GND wire: this wire is used for the feedback potentiometer. It should be connected to GND
- Potentiometer wire: (also referred to as feedback wire, or output wire) this wire outputs the voltage value in accordance with the stroke's position.
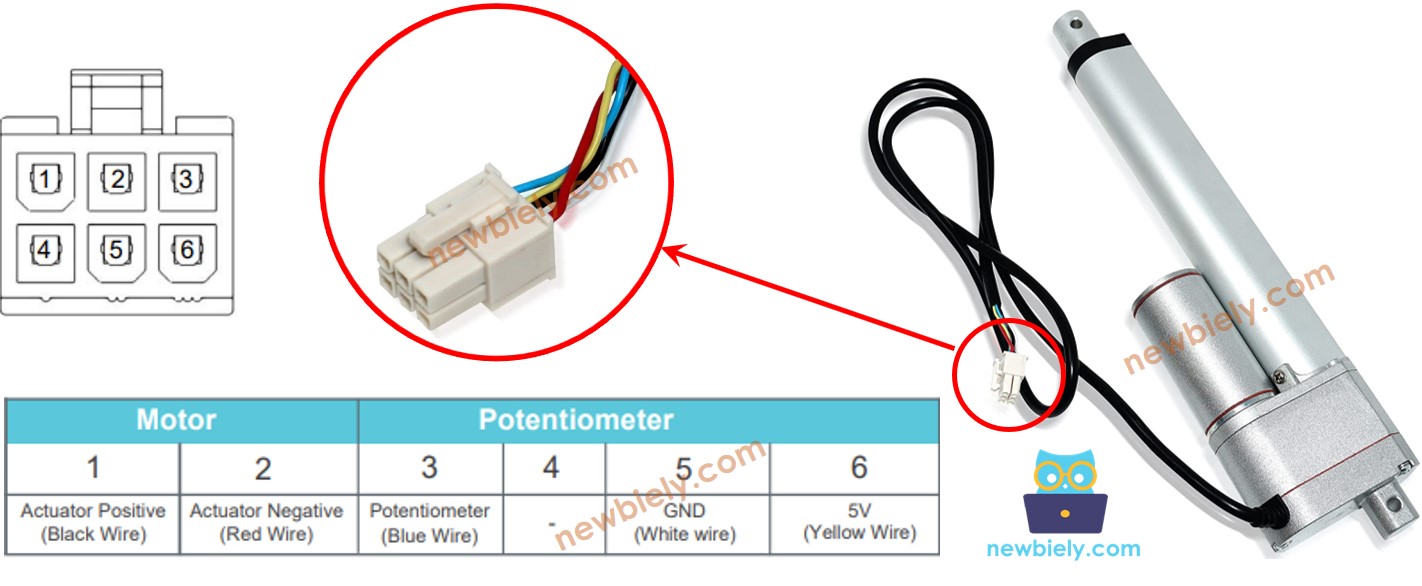
How It Works
If we supply high voltage to the positive and negative wires, the stroke of the actuator will be extended or retracted. Specifically:
- If 12V (12V, 24V, 48V...) is connected to the positive wire and GND to the negative wire, the linear actuator will extend at full speed until it reaches the limit.
- If 12V (12V, 24V, 48V...) is connected to the negative wire and GND to the positive wire, the linear actuator will retract at full speed until it reaches the limit.
- If power is cut off to the actuator (GND to both positive and negative wire), the actuator will cease extending/retracting.
※ NOTE THAT:
- The voltage required to operate the actuator is determined by its specifications. To find out the exact voltage, consult the datasheet or manual.
- Even when the power is turned off, the actuator can maintain its position while carrying a load.
The voltage in the potentiometer wire is directly related to the stroke position of the actuator. By measuring this voltage, we can ascertain the stroke's location.
Wiring Diagram
Take out all three jumpers from the L298N module prior to connecting the wires.
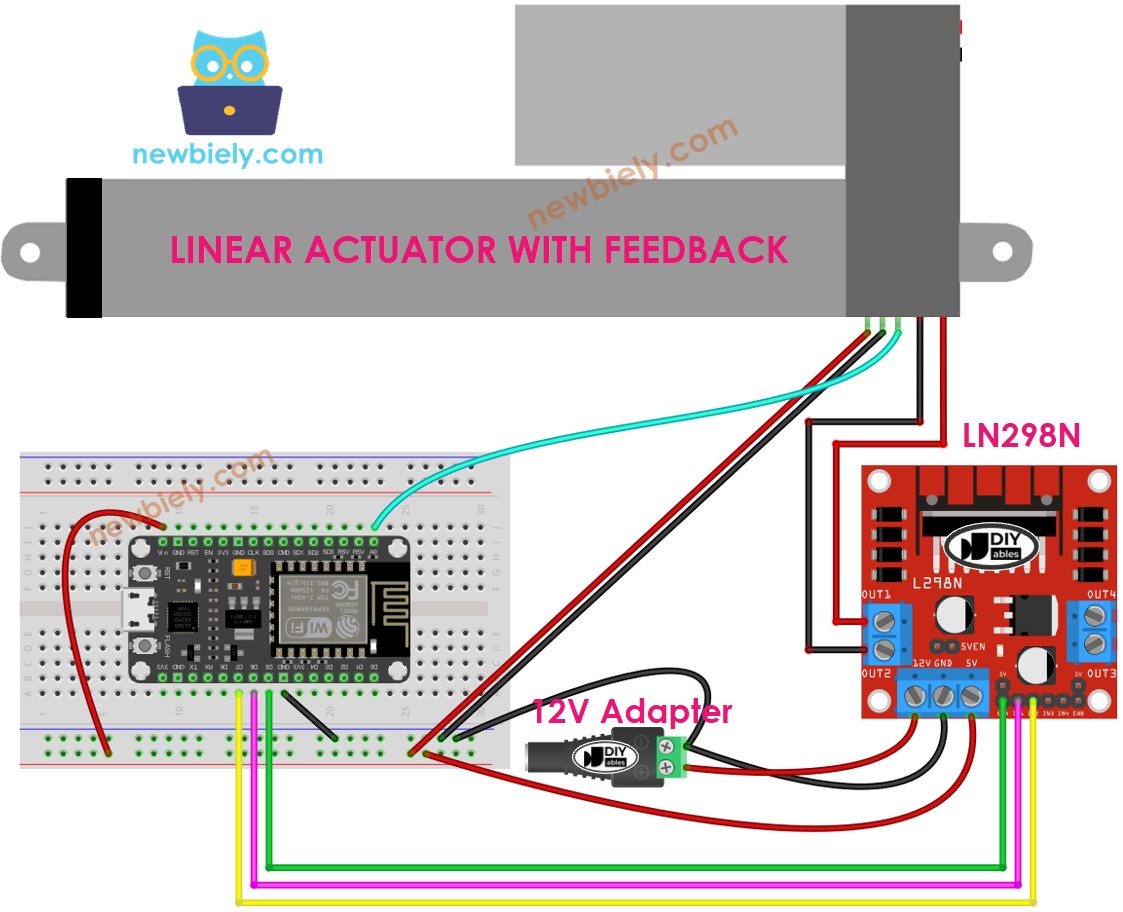
This image is created using Fritzing. Click to enlarge image
See more in ESP8266's pinout and how to supply power to the ESP8266 and other components.
How to control extend/retract a linear actuator
Check out the ESP8266 - Actuator tutorial.
How to find the position of the linear actuator
This is an illustration of how to locate the stroke on a linear actuator.
Calibration
- Determine the length of the actuator's stroke (in millimeters) by either measuring with a ruler or consulting the datasheet.
- To ascertain the output values when the linear actuator is fully extended and fully retracted, execute the code below.
- You will observe the log on Serial Monitor, as demonstrated in the example below
Note down the three values in the code below. If the minimum and maximum values are reversed, switch the IN1_PIN and IN2_PIN.
Make a note of the three values in the code below. If the minimum and maximum values are transposed, interchange the IN1_PIN and IN2_PIN.
ESP8266 code that calculate the position of the actuator
- Update the code with the three calibrated values.
- Upload the code to ESP8266.
- Check out the result on the Serial Monitor.