ESP8266 - Rain Sensor
The rain sensor detects and measures rain/snow levels, offering both digital (LOW/HIGH) and analog outputs. This tutorial demonstrates using an ESP8266 to connect and utilize the rain sensor, covering:
- Connecting the rain sensor to the ESP8266.
- Programming the ESP8266 to detect rain through the digital signal.
- Programming the ESP8266 to measure rain levels using the analog signal.
Afterward, you can customize the code to trigger a motor or warning when rain/snow is detected.
Hardware Preparation
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables .
Overview of Rain Sensor
The rain sensor can detect rain presence or measure water level. It offers two options through a digital output pin and an analog output pin.
The sensor comprises two components: the sensing pad and the electronic module
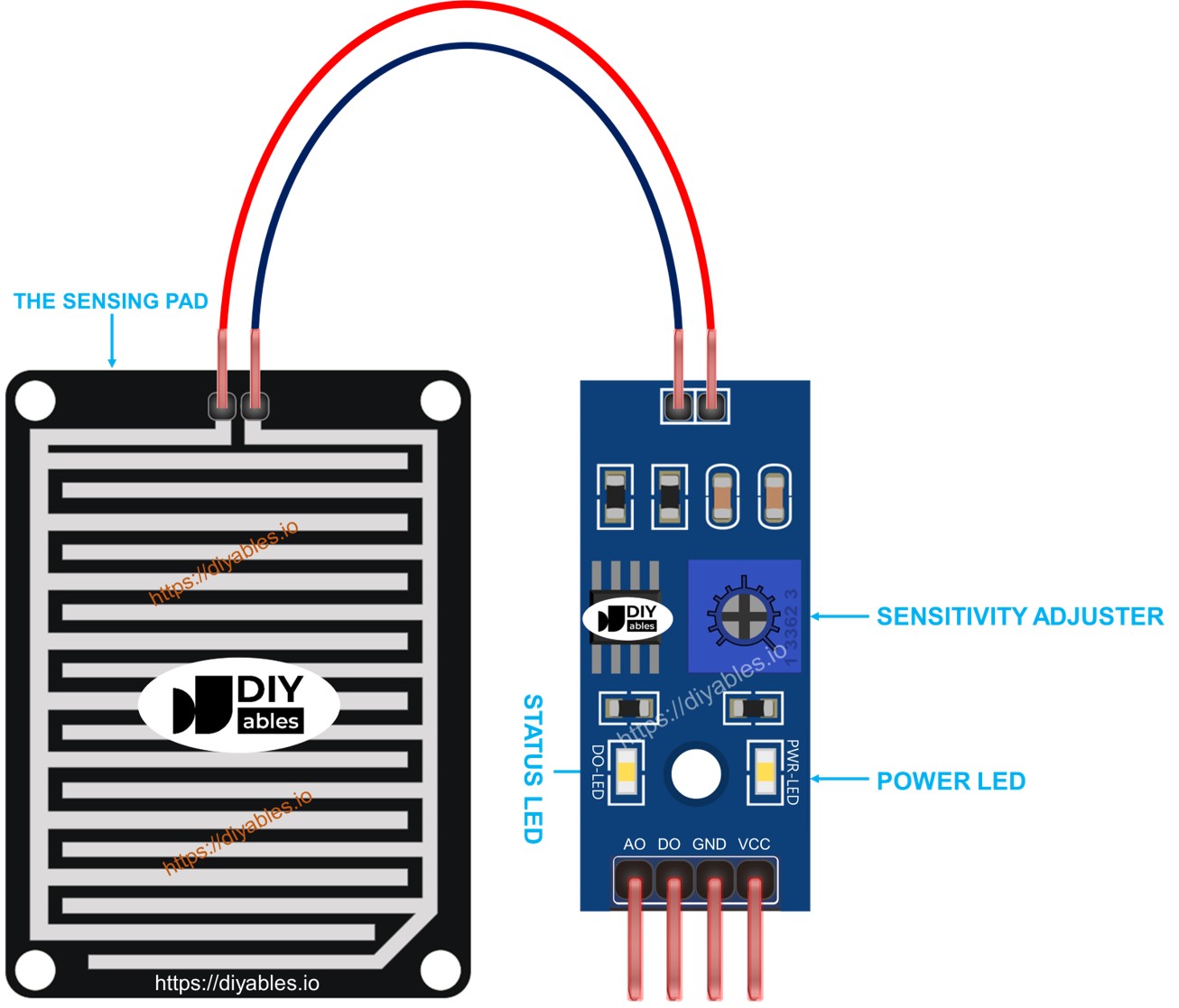
The sensing pad
The sensing pad, positioned outdoors to encounter rain/snow (e.g., on the roof), features exposed copper traces categorized into two groups: power traces and sense traces. These traces remain unconnected unless water or snow bridges them. Both power and sense traces are identical, allowing flexibility in designating one as the power trace and the other as the sense trace.
The electronic module
The electronic module of the rain sensor converts signals from the sensing pad into analog or digital values readable by the ESP8266 NodeMCU. It includes four pins:
- VCC pin: Connects to VCC (3.3V to 5V).
- GND pin: Connects to GND (0V).
- DO pin: Digital output pin, indicating HIGH when rain is not detected and LOW when detected. The rain detection threshold is adjustable with a built-in potentiometer.
- AO pin: Analog output pin, with the value decreasing with increased water on the sensing pad and increasing with decreased water.
Furthermore, it features two LED indicators:
- PWR-LED indicator for power.
- DO-LED indicator for the rain state on the DO pin, illuminating when rain is present.
How It Works
FRegarding the DO pin:
- The module is equipped with a built-in potentiometer for setting the sensitivity threshold.
- When the intensity exceeds the threshold, rain is detected, and the sensor's output pin goes LOW, triggering the illumination of the DO-LED.
- Conversely, when the intensity falls below the threshold, rain is not detected, the output pin goes HIGH, and the DO-LED turns off.
Concerning the AO pin:
- The AO pin's value decreases with more water in the sensing pad.
- Conversely, the AO pin's value increases with less water in the sensing pad.
It's important to note that the potentiometer does not influence the value on the AO pin.
Wiring Diagram
As previously mentioned, connecting the VCC pin of the sensor directly to the 3.3V or 5V pins on the ESP8266 can shorten the sensor's lifespan due to electrochemical corrosion. The recommended approach is to connect the VCC pin of the rain sensor to an output pin on the ESP8266. By programming that pin, the rain sensor can be powered only during readings, minimizing the impact of electrochemical corrosion.
Since the rain sensor module has two outputs, you can choose to use one or both of them, depending on what you need.
- The wiring diagram between ESP8266 and the rain sensor when using DO only.
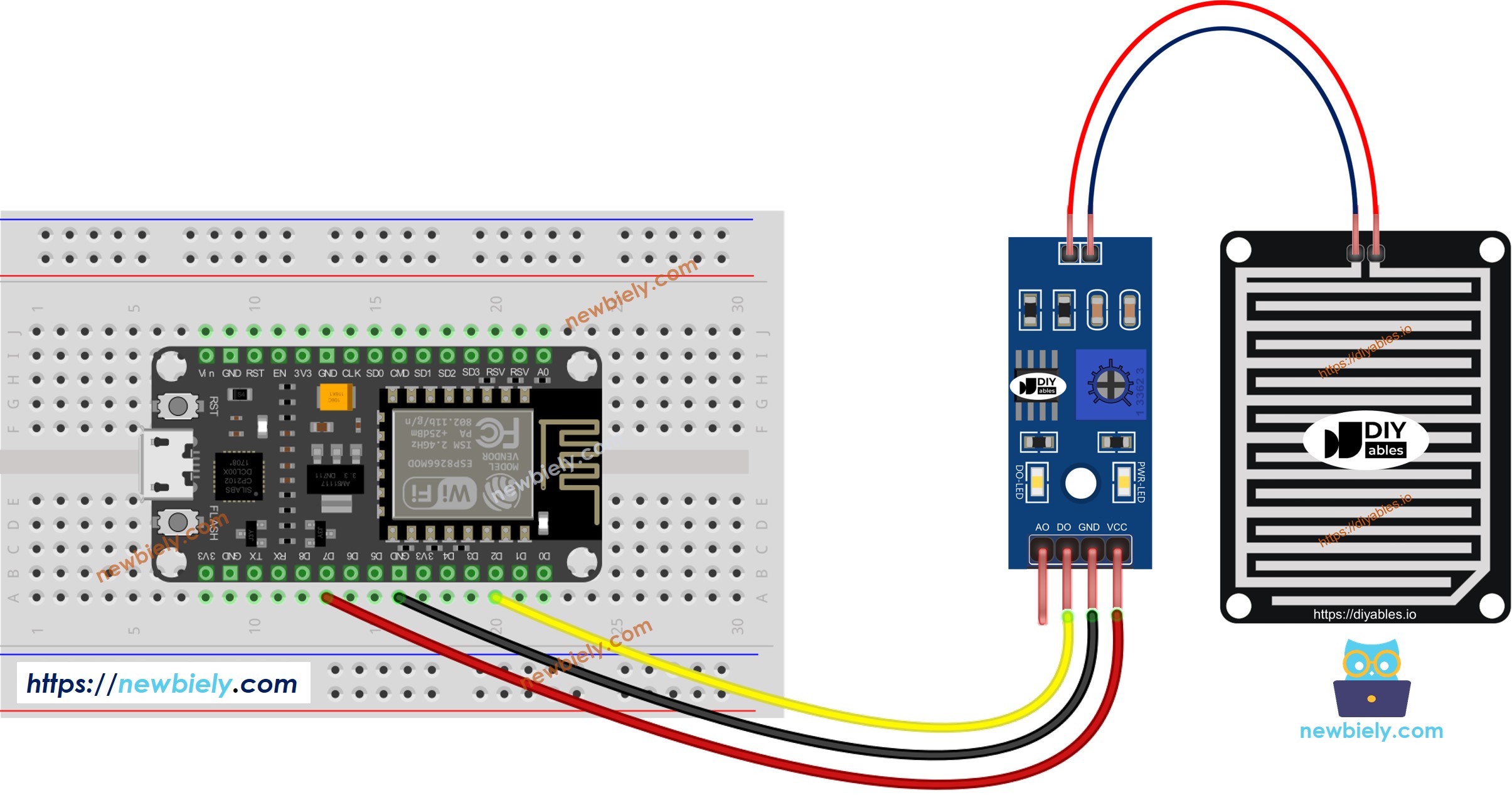
This image is created using Fritzing. Click to enlarge image
- The wiring diagram between ESP8266 and the rain sensor when using AO only.
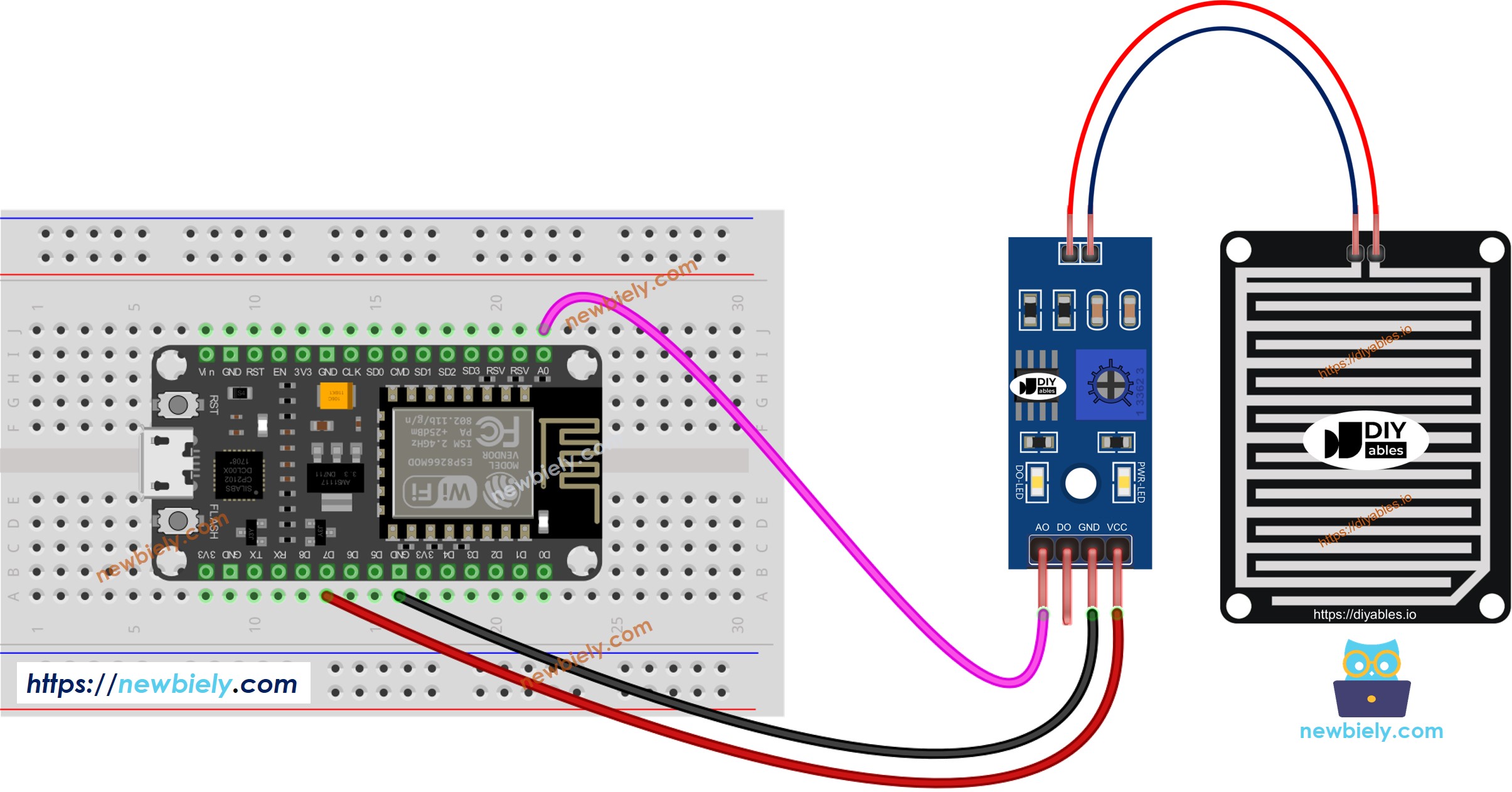
This image is created using Fritzing. Click to enlarge image
- The wiring diagram between ESP8266 and the rain sensor when using both AO an DO.
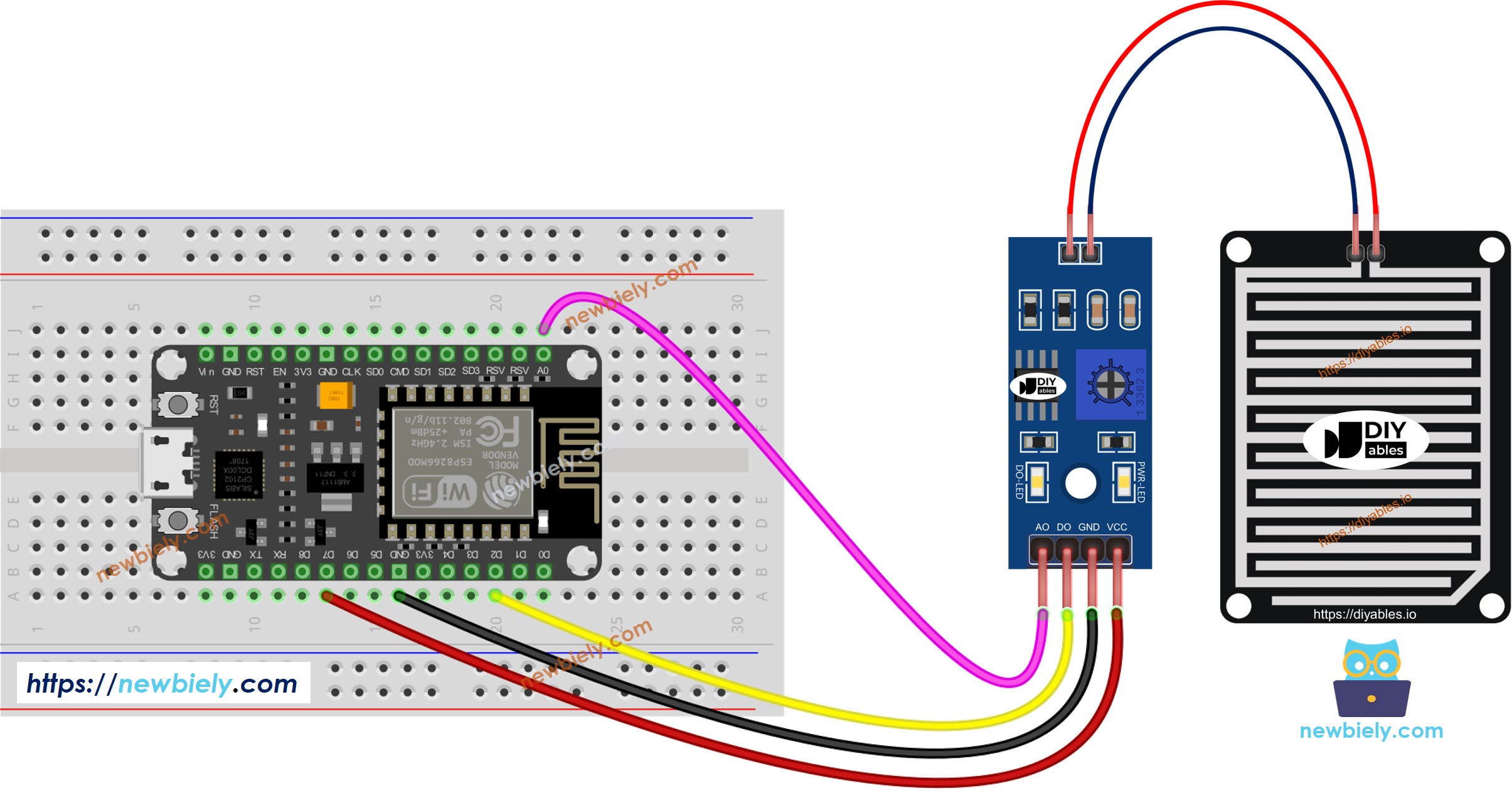
This image is created using Fritzing. Click to enlarge image
See more in ESP8266's pinout and how to supply power to the ESP8266 and other components.
ESP8266 Code - Read value from DO pin
Detailed Instructions
To get started with ESP8266 on Arduino IDE, follow these steps:
- Check out the how to setup environment for ESP8266 on Arduino IDE tutorial if this is your first time using ESP8266.
- Wire the components as shown in the diagram.
- Connect the ESP8266 board to your computer using a USB cable.
- Open Arduino IDE on your computer.
- Choose the correct ESP8266 board, such as (e.g. NodeMCU 1.0 (ESP-12E Module)), and its respective COM port.
- Copy the above code and open with Arduino IDE
- Click Upload button on Arduino IDE to upload code to ESP8266
- Drop some water to the rain sensor
- Check out the result on the Serial Monitor.
Please keep in mind that if you notice the LED status remaining on constantly or off even when the sensor faces to a rain, you can adjust the potentiometer to fine-tune the sensitivity of the sensor.
ESP8266 Code - Read value from AO pin
Detailed Instructions
- Wire the components as shown in the diagram.
- Connect the ESP8266 board to your computer using a USB cable.
- Open Arduino IDE on your computer.
- Choose the correct ESP8266 board, such as (e.g. NodeMCU 1.0 (ESP-12E Module)), and its respective COM port.
- Copy the above code and open with Arduino IDE
- Click Upload button on Arduino IDE to upload code to ESP8266
- Drop some water to the rain sensor
- Check out the result on the Serial Monitor.
※ NOTE THAT:
This tutorial uses the analogRead() function to get data from an ADC (Analog-to-Digital Converter) that's connected to a sensor or another part. The ESP8266's ADC works well for projects where you don't need very precise readings. But remember, the ESP8266's ADC isn't very accurate for detailed measurements. If your project needs to be very precise, you might want to use a separate ADC like the ADS1115 with the ESP8266, or use Arduino like the Arduino Uno R4 WiFi, which has a more reliable ADC.