ESP8266 - DC Motor Limit Switch
In this ESP8266 tutorial, we are going to learn how to use ESP8266 to control DC motor by limit switch and L298N driver. In detail, we are going to learn:
- How to stop DC motor when a limit switch is touched
- How to change the direction of DC motor when a limit switch is touched
- How to change the direction of DC motor when two limit switches is touched
Hardware Preparation
Or you can buy the following kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables .
Overview of DC Motor and Limit Switch
If you do not know about DC motor and limit switch (pinout, how it works, how to program ...), learn about them in the following tutorials:
- ESP8266 - Limit Switch tutorial
- ESP8266 - Controls DC Motor tutorial
Wiring Diagram
This tutorial provides the ESP8266 codes for two cases: One DC motor + one limit switch, One DC motor + two limit switches.
- Wiring diagram between the DC motor and a limit switch
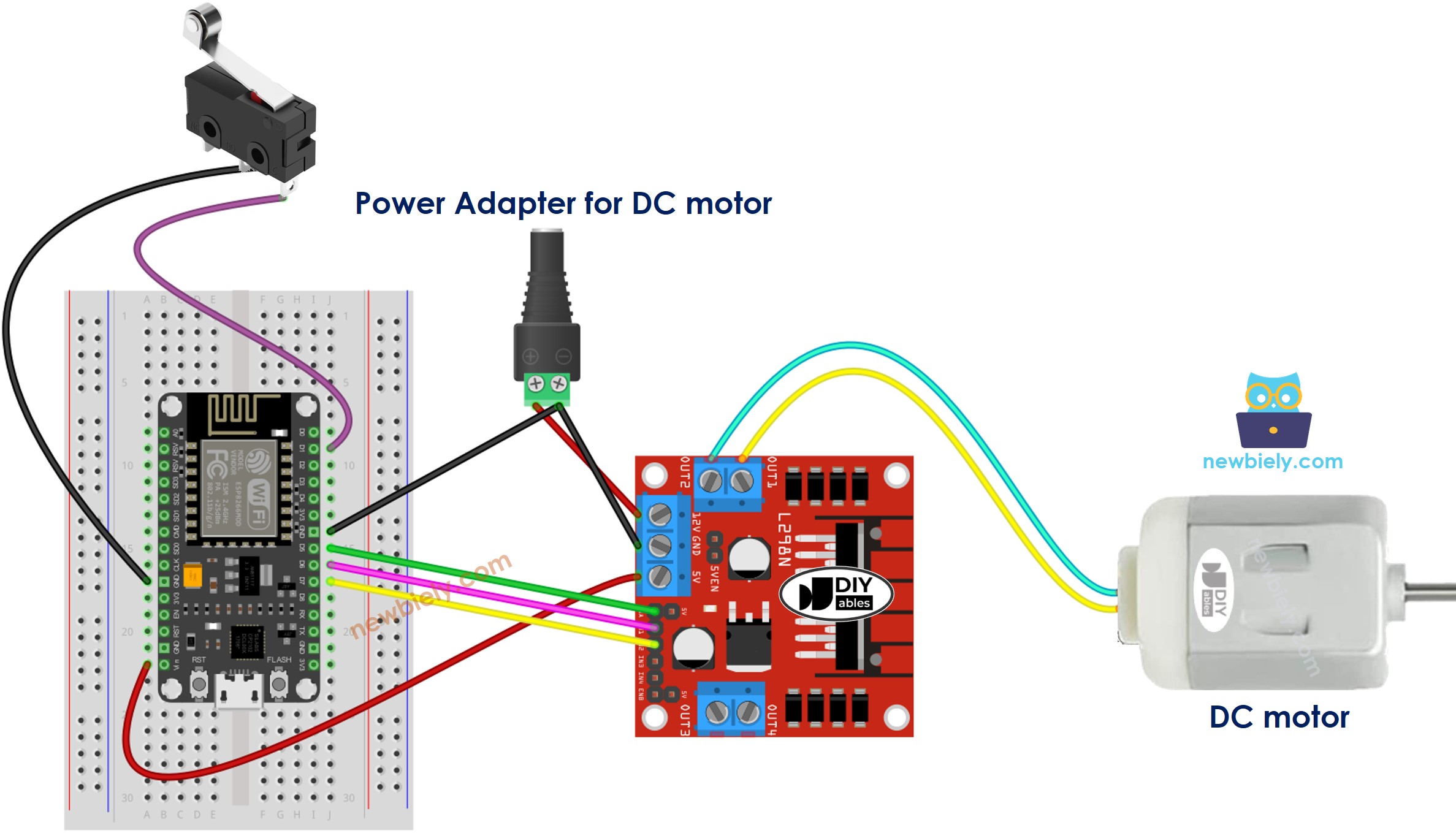
This image is created using Fritzing. Click to enlarge image
- Wiring diagram between the DC motor and two limit switches
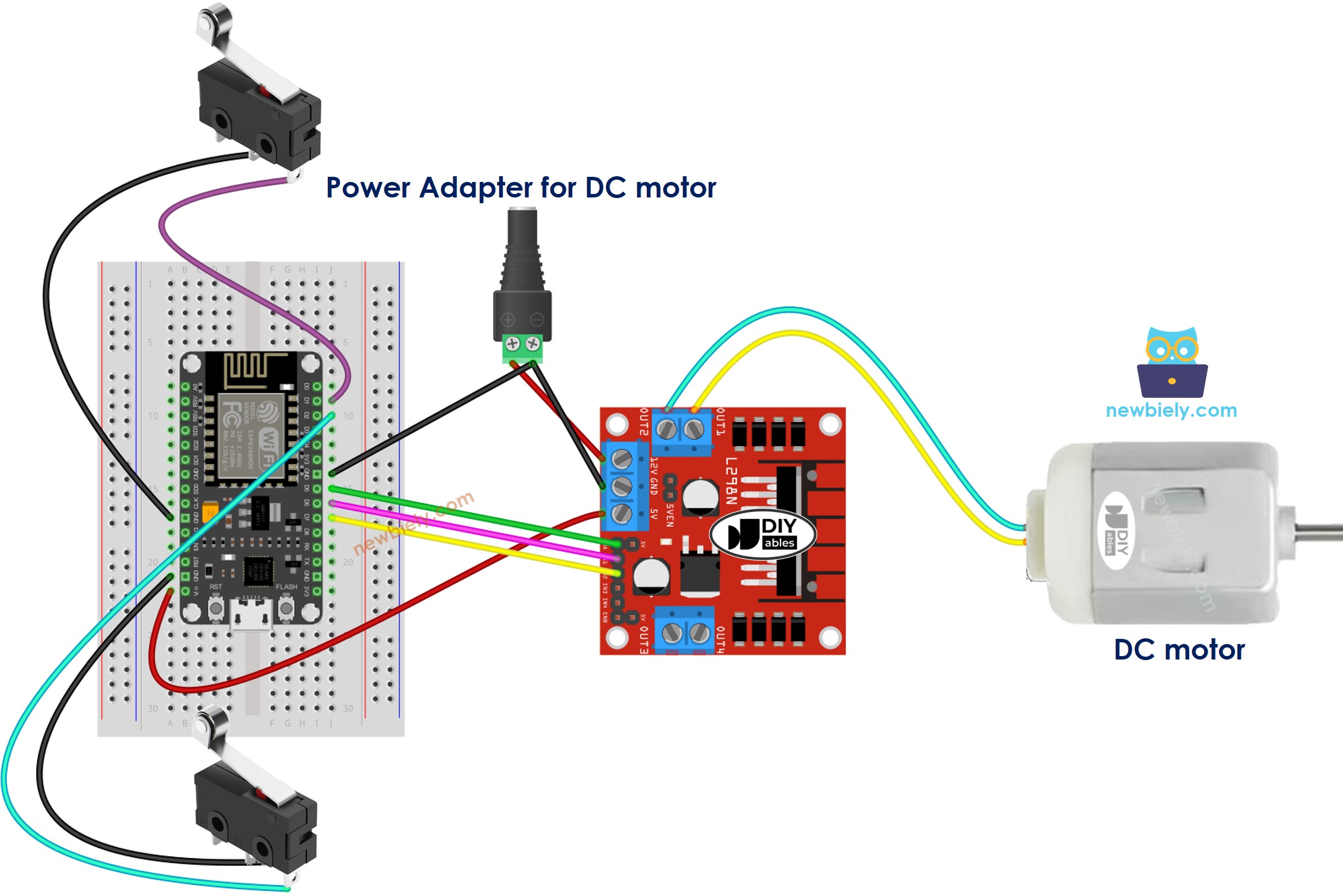
This image is created using Fritzing. Click to enlarge image
See more in ESP8266's pinout and how to supply power to the ESP8266 and other components.
ESP8266 Code - Stop DC Motor by a Limit Switch
The below code make a DC motor spin infinitely and stop immediately when a limit switch is touched
Detailed Instructions
To get started with ESP8266 on Arduino IDE, follow these steps:
- Check out the how to setup environment for ESP8266 on Arduino IDE tutorial if this is your first time using ESP8266.
- Wire the components as shown in the diagram.
- Connect the ESP8266 board to your computer using a USB cable.
- Open Arduino IDE on your computer.
- Choose the correct ESP8266 board, such as (e.g. NodeMCU 1.0 (ESP-12E Module)), and its respective COM port.
- Connect ESP8266 to PC via USB cable
- Open Arduino IDE, select the right board and port
- Click to the Libraries icon on the left bar of the Arduino IDE.
- Search “ezButton”, then find the button library by ESP8266GetStarted.com
- Click Install button to install ezButton library.
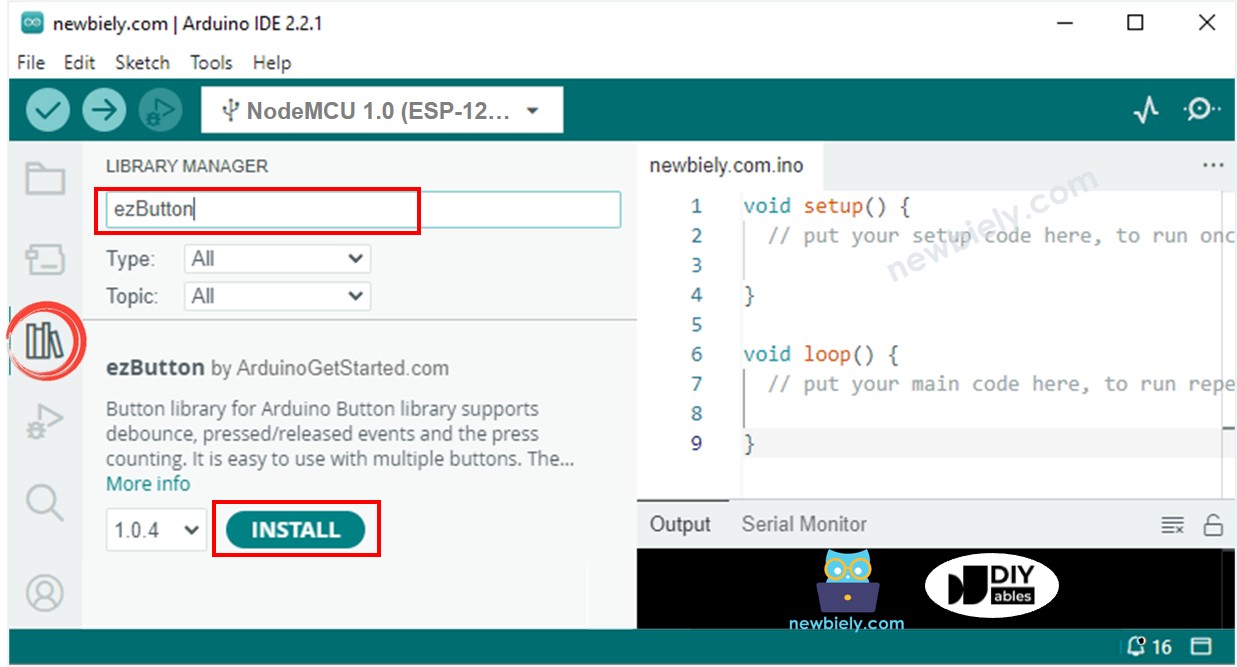
- Copy the above code and open with Arduino IDE
- Click Upload button on Arduino IDE to upload code to ESP8266
- If the wiring is correct, you will see the motor rotates clockwise direction.
- Touch the limit switch
- You will see the motor is stopped immediately
- The result on Serial Monitor looks like below
Code Explanation
Read the line-by-line explanation in comment lines of code!
ESP8266 Code - Change Direction of DC Motor by a Limit Switch
The below code make a DC motor spin infinitely and change its direction when a limit switch is touched
Detailed Instructions
- Copy the above code and open with Arduino IDE
- Click Upload button on Arduino IDE to upload code to ESP8266
- If the wiring is correct, you will see the motor spins in the clockwise direction.
- Touch the limit switch
- You will see the DC motor's direction is changed to the anti-clockwise
- Touch the limit switch again
- You will see the DC motor's direction is changed to clockwise
- The result on Serial Monitor looks like below
ESP8266 Code - Change Direction of DC Motor by two Limit Switches
The below code make a DC motor spin infinitely and change its direction when one of two limit switches is touched
Detailed Instructions
- Click Upload button on Arduino IDE to upload code to ESP8266
- If the wiring is correct, you will see the motor spins in the clockwise direction.
- Touch the limit switch 1
- You will see the DC motor's direction is changed to anti-clockwise
- Touch the limit switch 2
- You will see the DC motor's direction is changed to clockwise
- The result on Serial Monitor looks like below