ESP8266 - Bluetooth
This tutorial instructs you how to use ESP8266 to control Bluetooth HC-05 Module. In detail, we will learn:
- Utilizing Bluetooth with ESP8266
- Transmitting data from ESP8266 to a smartphone app through Bluetooth
- Receiving data on ESP8266 from the smartphone app via Bluetooth
- Controlling ESP8266 from the smartphone app via Bluetooth
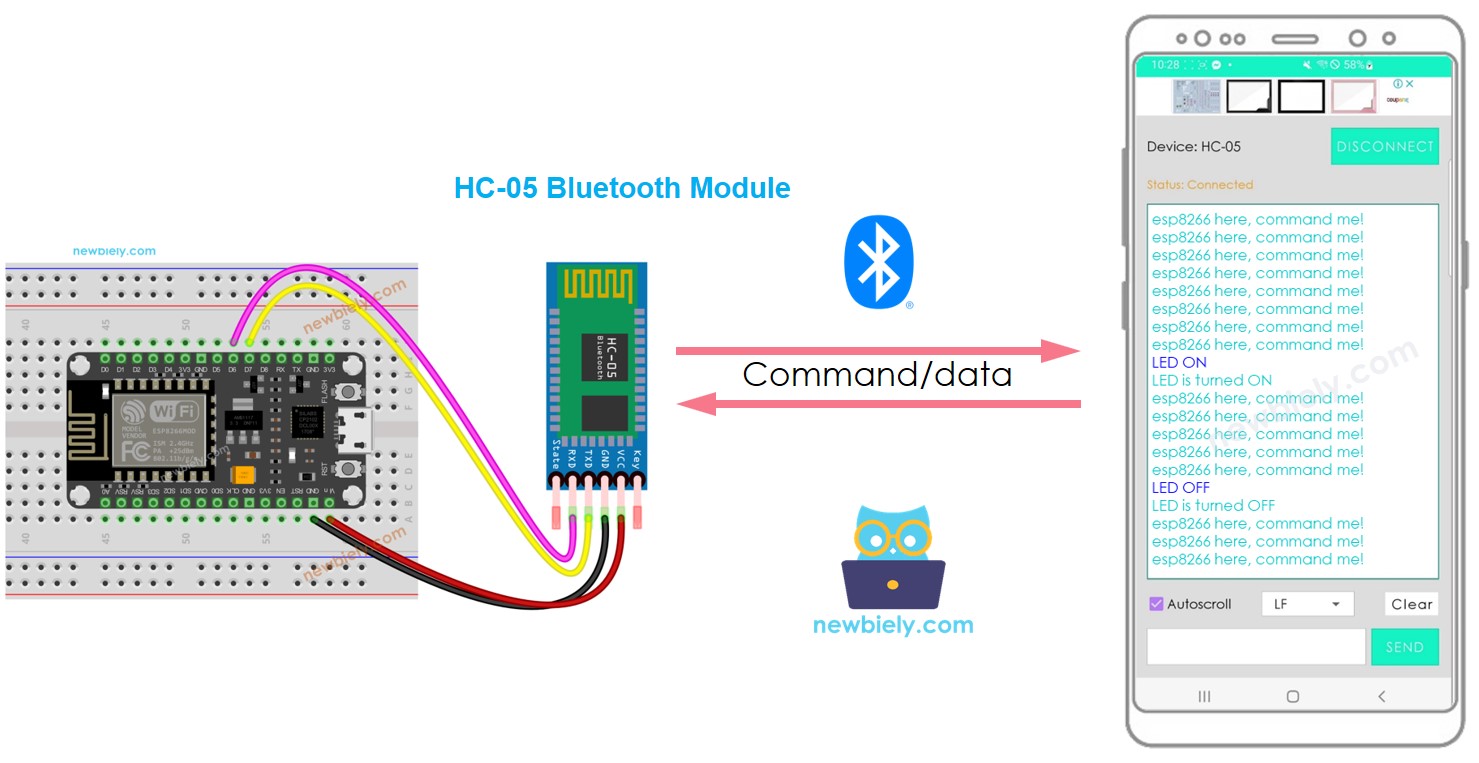
The purpose of this tutorial is to:
- Demonstrate how ESP8266 can exchange data with a smartphone app.
- Explain the use of the HC-05 Bluetooth Module.
- Show how to use the Bluetooth Serial Monitor App on Android.
- Illustrate how to control LED and servo motor from a smartphone app.
This tutorial focuses on Classic Bluetooth (Bluetooth 2.0). If you are searching for information about Bluetooth Low Energy - BLE (Bluetooth 4.0), please refer to this similar tutorial: ESP8266 - Bluetooth Low Energy
Hardware Preparation
Or you can buy the following kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables .
Overview of HC-05 Bluetooth Module
HC-05 is a Serial Bluetooth module that functions as a Serial to Bluetooth Converter. It has the capability to:
- Take in data from the Serial RX pin and transmit it to the paired device (such as a smartphone) via Bluetooth
- Receive data from Bluetooth (from the paired device) and transfer it to the Serial TX pin.
Specifically, when ESP8266 is communicating with a smartphone App (Android/iOS):
- It connects to the HC-05 Bluetooth Module through its Serial/SoftwareSerial pins.
- The HC-05 Bluetooth Module is paired with the smartphone App.
- To send data to the smartphone App, ESP8266 simply sends it to the Serial/SoftwareSerial.
- To receive data from the smartphone App, ESP8266 reads it from the Serial/SoftwareSerial.
- No special Bluetooth code is necessary on ESP8266.
The Bluetooth HC-05 Module Pinout
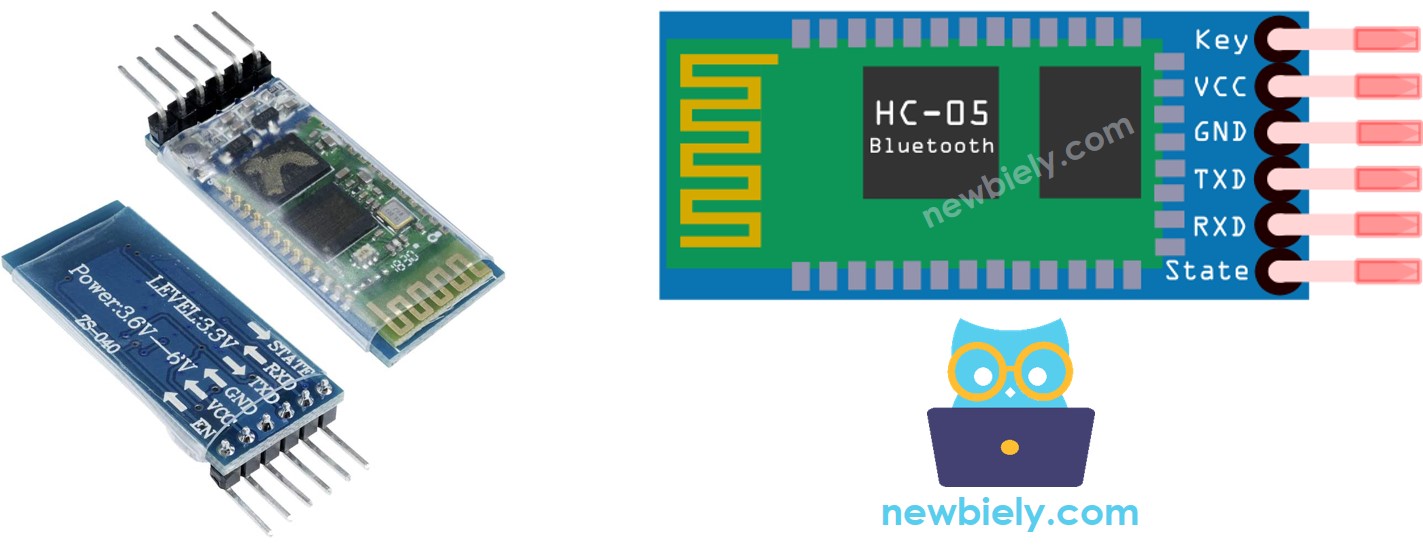
The HC-05 Bluetooth Module has 6 pins:
- Enable/Key pin: This pin is used to switch between Data Mode (set LOW) and Command mode (set HIGH). If it is not connected, it will be in Data mode by default.
- VCC pin: power pin, this should be connected to the +5V of the Supply voltage.
- GND pin: power pin, this should be connected to the GND of the power source.
- TX pin: Serial data pin, this should be connected to the RX pin of the ESP8266. The data received via Bluetooth will be sent to this pin as serial data.
- RX pin: Serial data pin, this should be connected to the TX pin of the ESP8266. The data received from this pin will be sent to the Bluetooth.
- State: The state pin is connected to the onboard LED, it can be used as feedback to check if the Bluetooth is functioning correctly.
Nevertheless, for fundamental operations, only four pins of The HC-05 Bluetooth Module are necessary to be connected to ESP8266.
The HC-05 Bluetooth Module has two integrated components:
- A LED which indicates the status of the Module:
- Blinking once every two seconds signifies that the Module has entered Command Mode
- Rapid blinking indicates that it is waiting for connection in Data Mode
- Blinking twice per second means that the connection in Data Mode was successful
- A Button which can be used to control the Key/Enable pin to select the operation mode (Data or Command Mode)
How It Works
The HC-05 Bluetooth module has two operation modes:
- Data mode which is used to exchange data with the paired device
- Command Mode which is used to configure parameters.
Luckily, the HC-05 Bluetooth module is able to operate with ESP8266 without the need for any configuration, using the default settings.
HC-05 Default Settings
Default Bluetooth Name | “HC-05” |
---|---|
Default Password | 1234 or 0000 |
Default Communication | Slave |
Default Mode | Data Mode |
Default Data Mode Baud Rate | 9600, 8, N, 1 |
Default Command Mode Baud Rate | 38400, 8, N, 1 |
Overview of Bluetooth Serial Monitor App
The Bluetooth Serial Monitor App is a mobile application with a User Interface resembling the Serial Monitor of Arduino IDE. It can be used to communicate with ESP8266 via Bluetooth without requiring any special code for the Bluetooth module in the ESP8266 code. To do this, the following steps should be followed:
- Connect ESP8266 to the HC-05 Bluetooth module
- Install the Bluetooth Serial Monitor App on your smartphone
- Open the App and pair it with the HC-05 Bluetooth module
Now, you can transmit and receive data from ESP8266 just as you would with the Serial Monitor of the Arduino IDE. You don't need to make any changes to your existing ESP8266 code or add any Bluetooth code to your new ESP8266 code.
Wiring Diagram
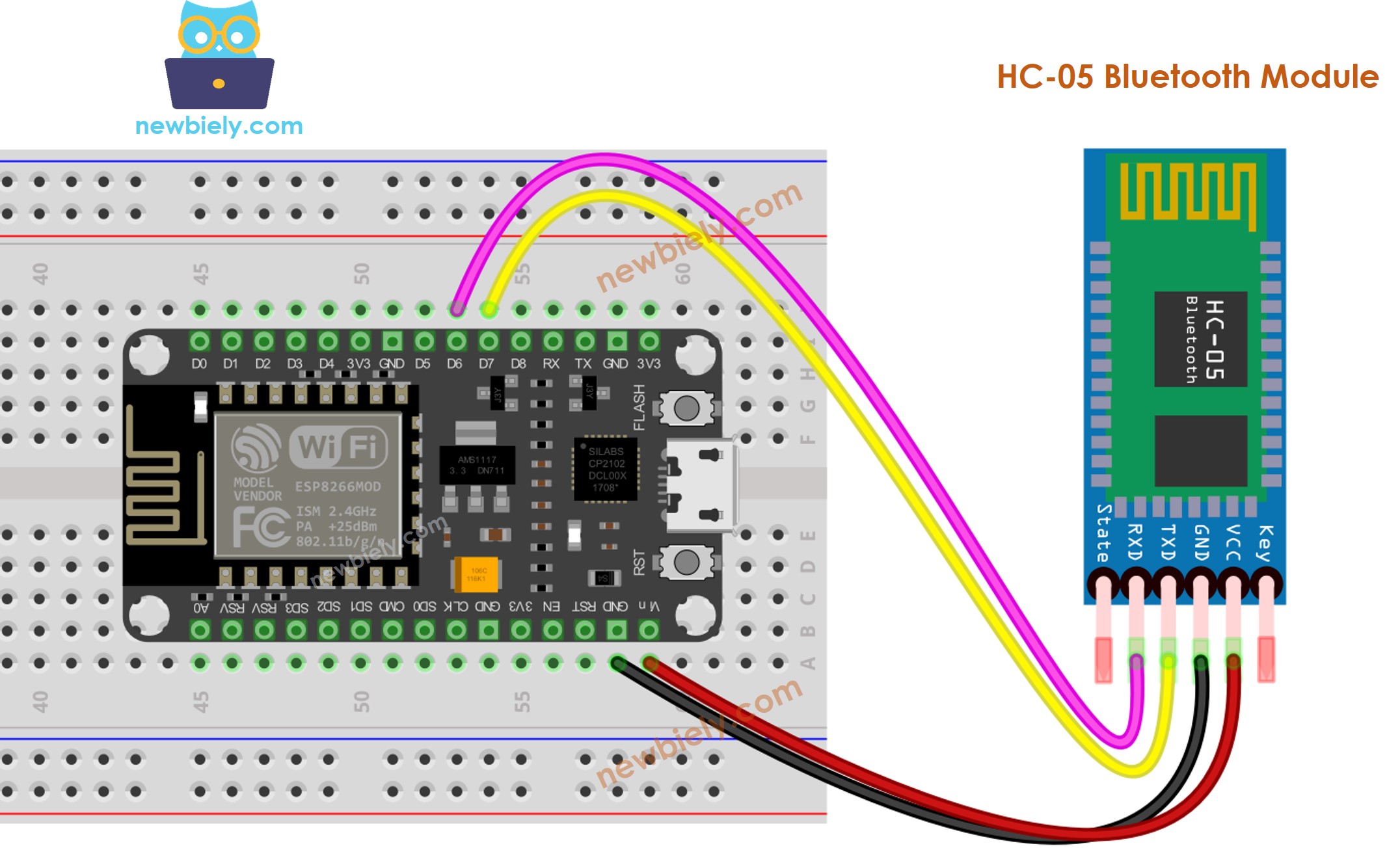
This image is created using Fritzing. Click to enlarge image
See more in ESP8266's pinout and how to supply power to the ESP8266 and other components.
Table of Wiring. Wiring Chart. Chart of Wiring
ESP8266 Pins | HC-05 Bluetooth Pins |
---|---|
Pin D7 | TX |
Pin D6 | RX |
5V | VCC |
GND | GND |
Enable/Key (NOT connected) | |
State (NOT connected) |
※ NOTE THAT:
You can use other ESP8266 pins by altering the Serial object in the ESP8266 code to a different one, such as Serial1, Serial2, or SoftwareSerial if it is available.
How To Program For Bluetooth
No programming specific to Bluetooth is necessary. We just need to utilize the Serial code.
ESP8266 sends data to Bluetooth App on Smartphone
In order to transmit data from an ESP8266 to a Bluetooth App on a Smartphone, the following code must be used on the Arduino:
In this instance, we will have the ESP8266 transmit “ESP8266 here, command me!” to the Bluetooth App on a Smartphone at one-second intervals.
Detailed Instructions
To get started with ESP8266 on Arduino IDE, follow these steps:
- Check out the how to setup environment for ESP8266 on Arduino IDE tutorial if this is your first time using ESP8266.
- Wire the components as shown in the diagram.
- Connect the ESP8266 board to your computer using a USB cable.
- Open Arduino IDE on your computer.
- Choose the correct ESP8266 board, such as (e.g. NodeMCU 1.0 (ESP-12E Module)), and its respective COM port.
- Install the Bluetooth Serial Monitor App on your smartphone. Wire the HC-05 Bluetooth module to ESP8266 as per the wiring diagram. Copy the code and open it with Arduino IDE. Click the Upload button on Arduino IDE to upload the code to ESP8266. If you fail to upload the code, disconnect the TX and RX pins from the Bluetooth module, upload the code, and then reconnect the RX/TX pin again. Open the Serial Monitor on Arduino IDE. Open the Bluetooth Serial Monitor App on your smartphone and select the Classic Bluetooth mode.
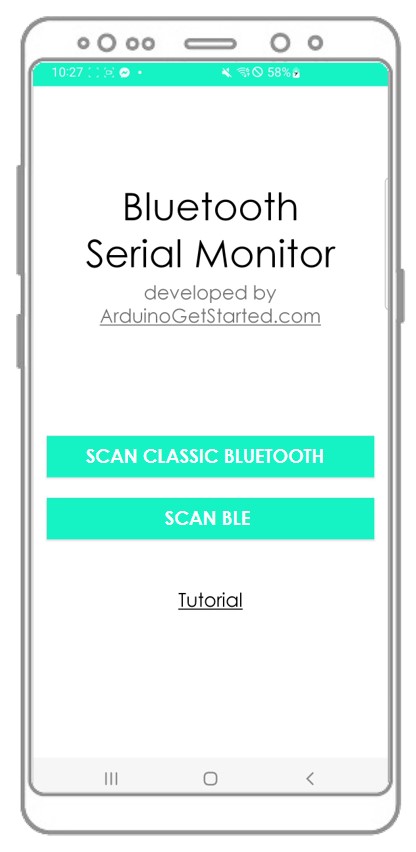
- Connect it with the HC-05 Bluetooth module.
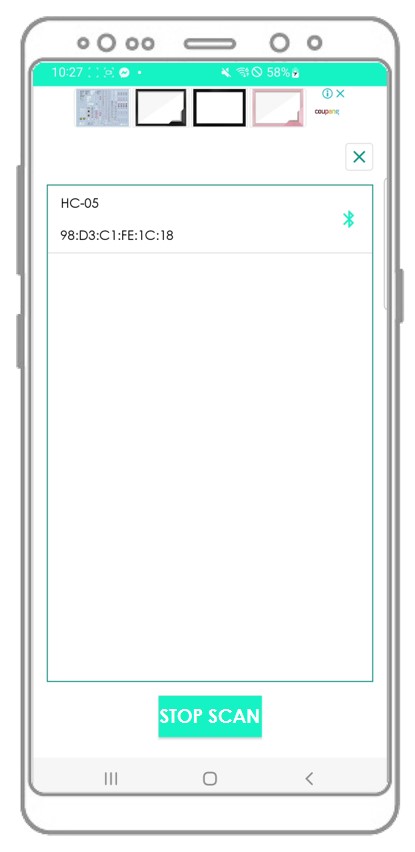
- Check out the outcome on the Android App.
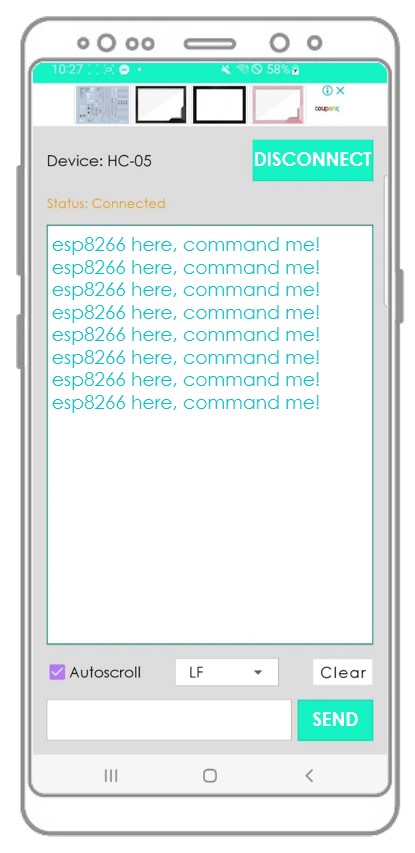
- View the outcome on the Serial Monitor of the ESP8266 Integrated Development Environment.
You will observe that the information displayed on the Serial Monitor of the Arduino IDE and the Android App are the same.
Bluetooth App Send data To ESP8266
The following code enables:
- Sending of data from a Bluetooth App to an ESP8266
- Reading of the data by the ESP8266, followed by sending of a response back to the Bluetooth App
Detailed Instructions
- Wire the components as shown in the diagram.
- Connect the ESP8266 board to your computer using a USB cable.
- Open Arduino IDE on your computer.
- Choose the correct ESP8266 board, such as (e.g. NodeMCU 1.0 (ESP-12E Module)), and its respective COM port.
- Copy the code and open it with Arduino IDE.
- Click the Upload button on Arduino IDE to compile and upload the code to ESP8266.
- Open the Serial Monitor on Arduino IDE.
- Connect the Android App to the HC-05 Bluetooth module, as done in the previous example.
- Once connected, type either "LED ON" or "LED OFF" on the Android App and press the "SEND" button.
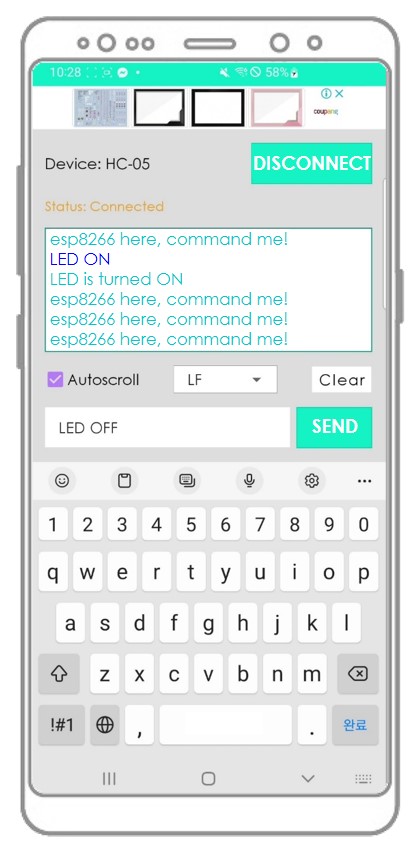
- The ESP8266 receives the data and prints the response to the Serial port. This data is then sent to the Bluetooth app, and the result can be seen on the Android App.
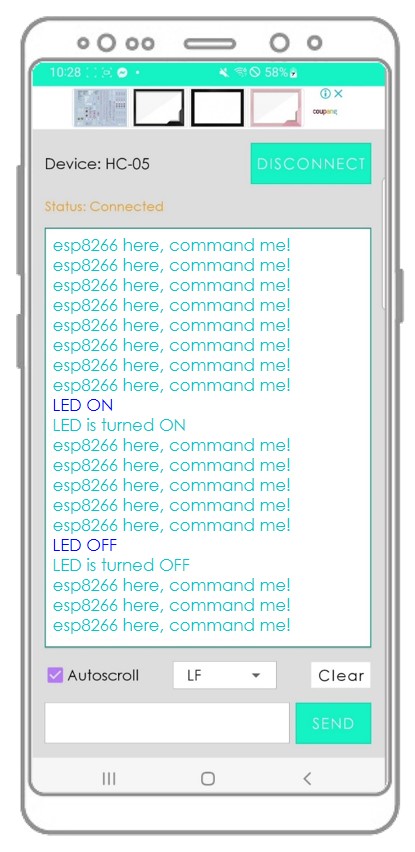
- Check out the outcome in the Serial Monitor window of the ESP8266 Integrated Development Environment.
You will observe that the information displayed on the Serial Monitor of the Arduino IDE and the Android App are the same.
ESP8266 Code - Control LED with smartphone App via Bluetooth
This ESP8266 example code will respond to two commands: “ON” and “OFF”. When received, these commands will be used to turn a built-in LED on or off via the Bluetooth Serial Monitor App.
You can find a more thorough explanation of the instructions in the ESP8266 controls LED via Bluetooth/BLE tutorial.
ESP8266 Code - Control Servo Motor with smartphone App via Bluetooth
The ESP8266 code below . * receives the angle value . * from the Bluetooth Serial Monitor App . * to control the angle of the servo motor.
You can view the instructions in greater detail in the ESP8266 controls Servo Motor via Bluetooth/BLE tutorial . For a more thorough look at the instructions, check out the ESP8266 controls Servo Motor via Bluetooth/BLE tutorial . If you need to see the instructions in greater depth, refer to the ESP8266 controls Servo Motor via Bluetooth/BLE tutorial . To get a more comprehensive view of the instructions, go to the ESP8266 controls Servo Motor via Bluetooth/BLE tutorial
If you find the Bluetooth Serial Monitor app helpful, kindly give it a 5-star rating on Play Store. Appreciate your support!