ESP8266 - Switch
The toggle switch, also known as the ON/OFF switch, has two states: ON (closed) and OFF (open). When pressed, the switch will toggle between the two states and maintain its state even when released.
This tutorial instructs you how to use ESP8266 with the ON/OFF switch. In detail, we will learn:
- How to connect the ESP8266 to the ON/OFF switch.
- How to program the ESP8266 to read the state of the ON/OFF switch.
- How to program the ESP8266 to check if the ON/OFF switch is changed or not.
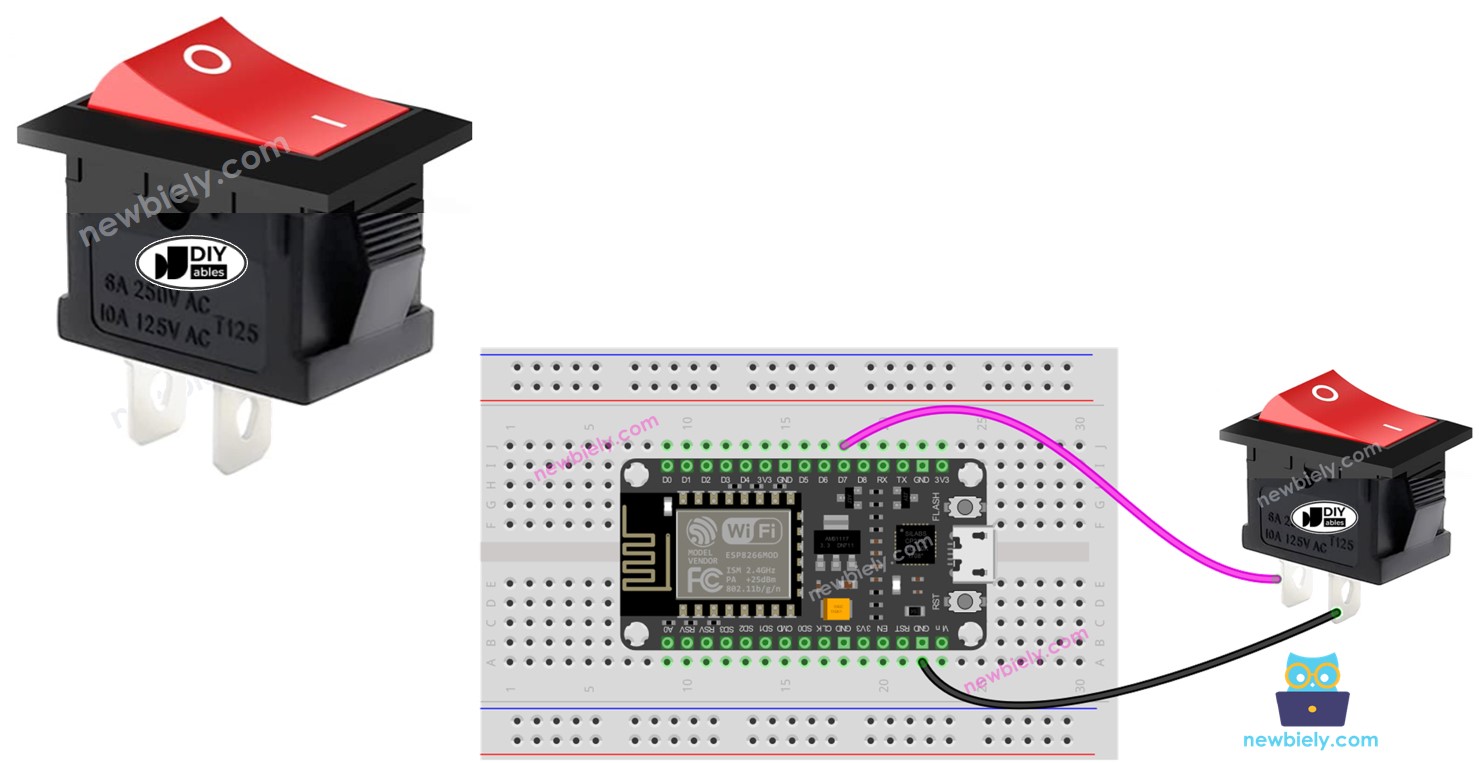
Do not mix up the following:
Hardware Preparation
Or you can buy the following kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables .
Overview of ON/OFF Switch
A switch that can be toggled between two states, ON and OFF, is known as an ON/OFF Switch. When pressed, it will change its state, either from ON to OFF or OFF to ON. The switch will remain in this state until it is pressed again.
The Switch Pinout
There are two varieties of the ON/OFF Switch: two-pin and three-pin.
In this tutorial, we will be utilizing a two-pin switch. With this kind, there is no requirement to differentiate between the two pins.
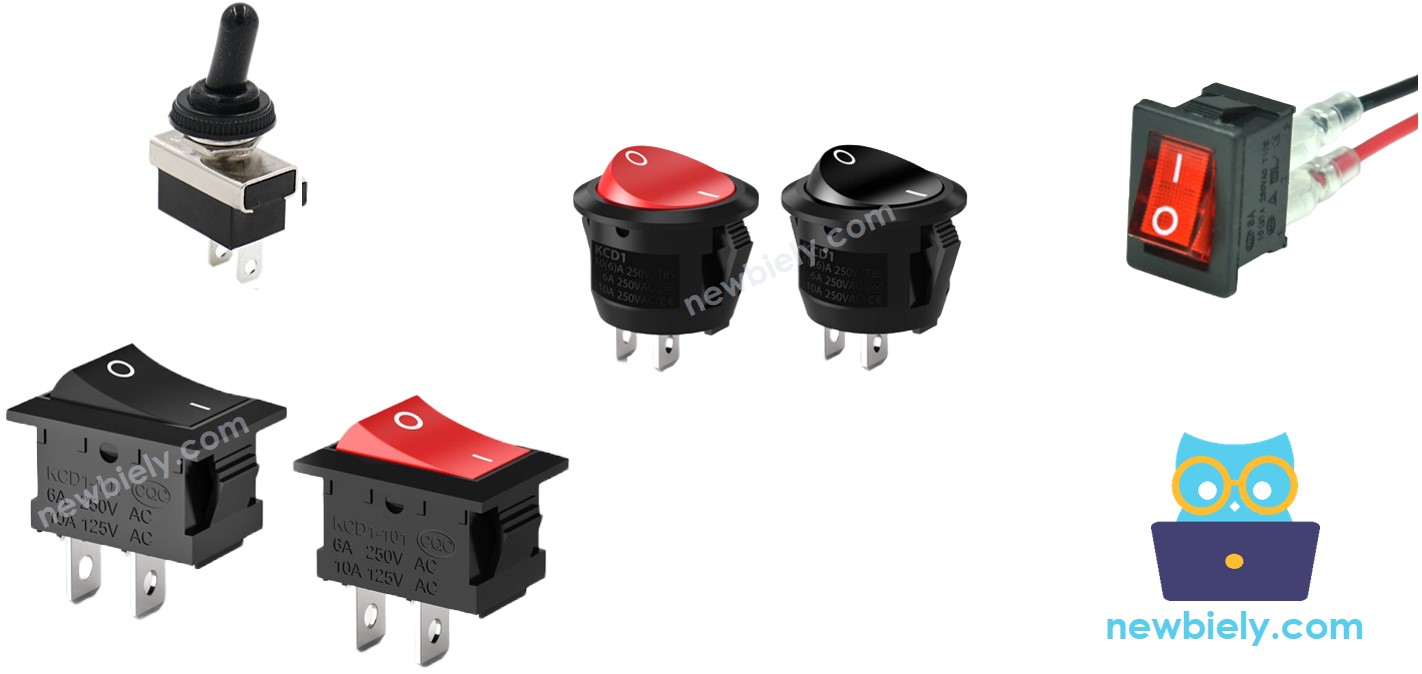
How It Works
There are two methods of using an ON/OFF switch. The following is a wiring table for the switch and the reading state on ESP8266 in both cases:
pin 1 | pin 2 | ESP8266 Input Pin's State | |
---|---|---|---|
1 | GND | ESP8266 Input Pin (with pull-up) | HIGH ⇒ OFF, LOW ⇒ ON |
2 | VCC | ESP8266 Input Pin (with pull-down) | HIGH ⇒ ON, LOW ⇒ OFF |
We must select one of the two options presented. The remainder of the tutorial will utilize the first option.
Wiring Diagram
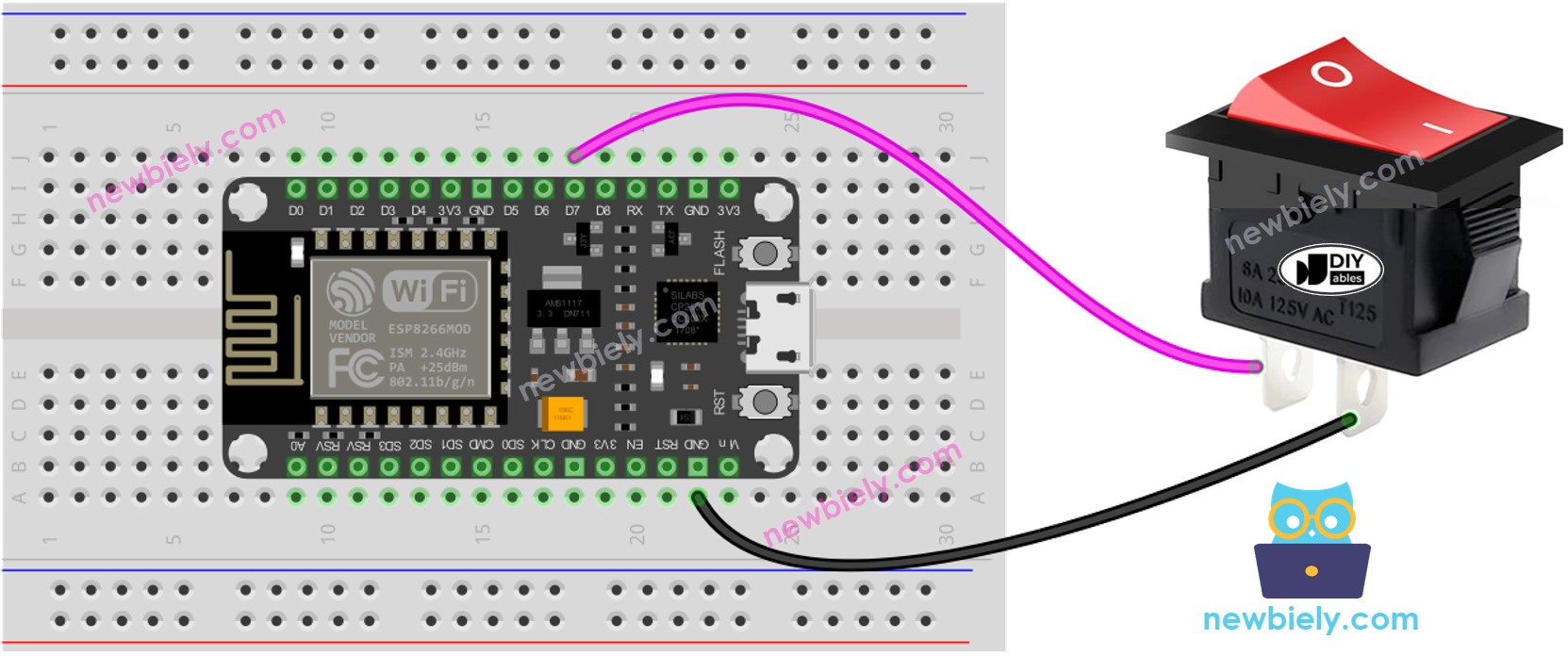
This image is created using Fritzing. Click to enlarge image
See more in ESP8266's pinout and how to supply power to the ESP8266 and other components.
For a secure and reliable wiring connection, we suggest using a Soldering Iron to solder the wires and pins of the ON/OFF switch. To ensure safety, we recommend using Heat Shrink Tube to cover the connection.
ESP8266 Code - ON/OFF Switch
As with a button, an ON/OFF switch also requires debouncing (for more information, please see Why needs debounce for the button, ON/OFF switch?). This can make coding complex. Fortunately, the ezButton library provides this debouncing function, as well as an internal pull-up register, making programming simpler.
※ NOTE THAT:
There are two common applications:
- The first: If the switch is set to ON, perform one action. If the switch is set to OFF, take a different action in response.
- The second: If the switch is flipped from ON to OFF (or OFF to ON), do something.
Detailed Instructions
To get started with ESP8266 on Arduino IDE, follow these steps:
- Check out the how to setup environment for ESP8266 on Arduino IDE tutorial if this is your first time using ESP8266.
- Wire the components as shown in the diagram.
- Connect the ESP8266 board to your computer using a USB cable.
- Open Arduino IDE on your computer.
- Choose the correct ESP8266 board, such as (e.g. NodeMCU 1.0 (ESP-12E Module)), and its respective COM port.
- Do the wiring as indicated in the wiring diagram.
- Connect your ESP8266 to a PC using a USB cable.
- Open the Arduino IDE.
- Install the ezButton library. Refer to the instructions on How To.
- Choose the correct board and port.
- Click the Upload button on the Arduino IDE to upload the code to the ESP8266.
- Turn the switch to the ON position.
- Check out the result on the Serial Monitor.
- Then turn the switch to the OFF position.
- Check out the result on the Serial Monitor.