ESP8266 - Button - Long Press Short Press
This tutorial instructs you how to use ESP8266 to delect the button's long press and short press. In detail, we will walk through the following examples:
- The ESP8266 detects the short press on the button.
- The ESP8266 detects the long press on the button.
- The ESP8266 detects both long press and short press on the button.
- The ESP8266 debounces for long press and short press.
In the concluding section, we explore how to implement debouncing in practical applications. To learn more about why debouncing is necessary for buttons, please refer to this article. Without debouncing, incorrect detection of a button press may occur.
Hardware Preparation
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables .
Wiring Diagram
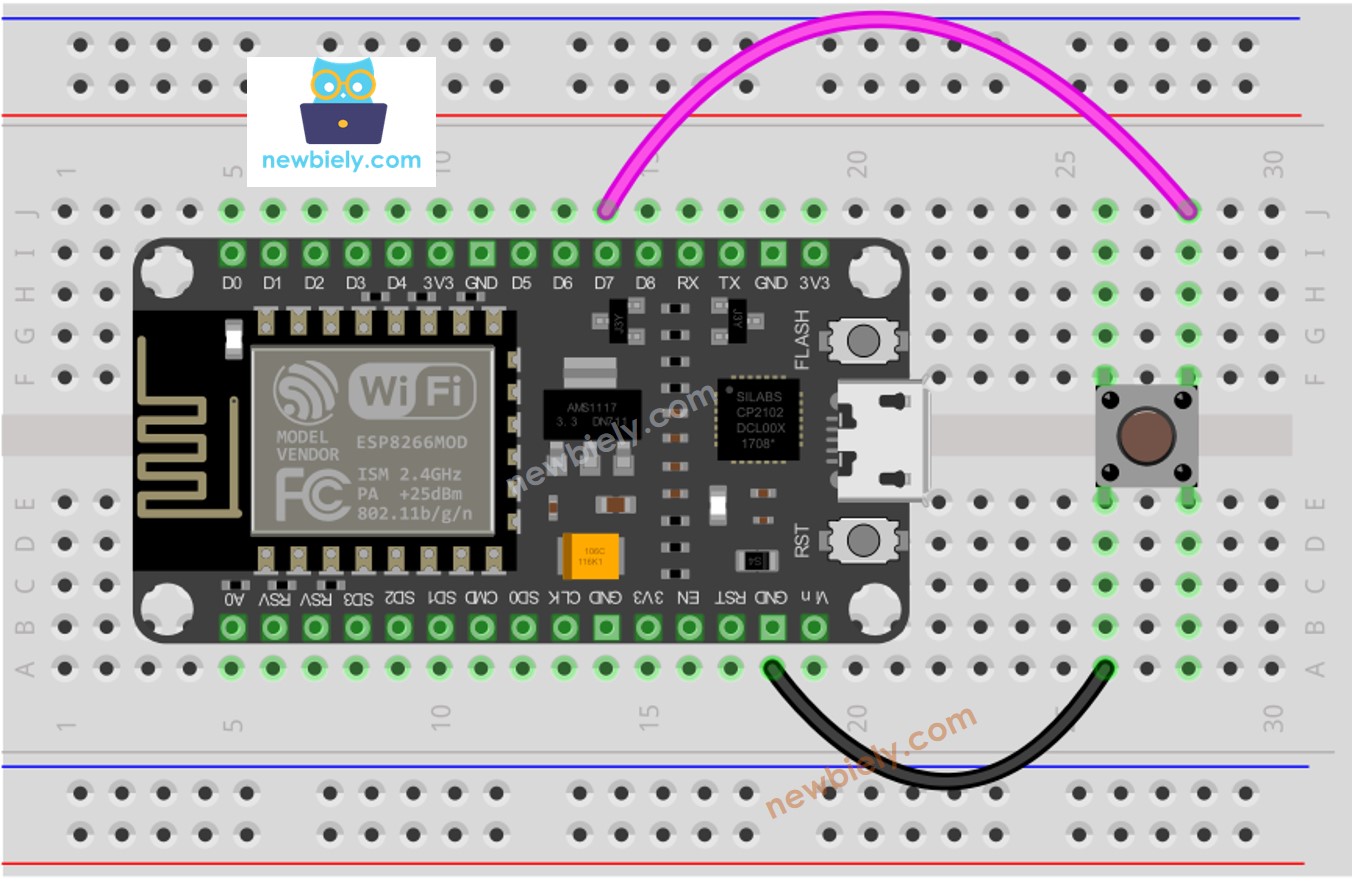
This image is created using Fritzing. Click to enlarge image
See more in ESP8266's pinout and how to supply power to the ESP8266 and other components.
In this tutorial, we will be utilizing the internal pull-up resistor. This means that the button is in a HIGH state when not pressed and a LOW state when pressed.
How To Detect Short Press
We calculate the amount of time between the pressed and released events. If this duration is less than a predetermined time, we detect a short press event.
Determine the duration of a maximum short press.
- Determine when the button has been pressed and record the time of the press.
- Detect when the button is released and record the time of its release.
- Force
- Determine the length of time and strength of pressure to be applied.
- Compare the length of the press to the predetermined time for a short press.
- Identify if it is a short press based on the comparison.
ESP8266 Code for detecting the short press
Detailed Instructions
To get started with ESP8266 on Arduino IDE, follow these steps:
- Check out the how to setup environment for ESP8266 on Arduino IDE tutorial if this is your first time using ESP8266.
- Wire the components as shown in the diagram.
- Connect the ESP8266 board to your computer using a USB cable.
- Open Arduino IDE on your computer.
- Choose the correct ESP8266 board, such as (e.g. NodeMCU 1.0 (ESP-12E Module)), and its respective COM port.
- Upload the code to the ESP8266 using the Arduino IDE.
- Press the button for a short time multiple times.
- Check the output on the Serial Monitor.
※ NOTE THAT:
The Serial Monitor may display multiple short press detections for a single press. This is the expected behavior of the button and is referred to as the “chattering phenomenon”. We will address this issue in the last part of this tutorial.
How To Detect Long Press
There are two scenarios in which long press can be detected:
- When the button is released, the long-press event will be identified
- While the button is being pressed, the long-press event will be recognized, even before it is let go.
- For the initial instance, we calculate the time interval between the pressed and released events. 2. If the duration is more than the predetermined time, then the long-press event is identified.
In the second use case, once the button is pressed, the pressing time is measured continuously and the long-press event is checked until the button is released. As the button is being held down, if the duration is greater than a predetermined amount of time, then the long-press event is detected.
ESP8266 Code for detecting long press when released
Detailed Instructions
To get started with ESP8266 on Arduino IDE, follow these steps:
- Check out the how to setup environment for ESP8266 on Arduino IDE tutorial if this is your first time using ESP8266.
- Wire the components as shown in the diagram.
- Connect the ESP8266 board to your computer using a USB cable.
- Open Arduino IDE on your computer.
- Choose the correct ESP8266 board, such as (e.g. NodeMCU 1.0 (ESP-12E Module)), and its respective COM port.
- Upload the code to ESP8266 using the Arduino IDE.
- Wait for one second and then press and release the button.
- Check out the result on the Serial Monitor.
The event of long-pressing is only detected when the button is let go.
ESP8266 Code for detecting long press during pressing
Detailed Instructions
To get started with ESP8266 on Arduino IDE, follow these steps:
- Check out the how to setup environment for ESP8266 on Arduino IDE tutorial if this is your first time using ESP8266.
- Wire the components as shown in the diagram.
- Connect the ESP8266 board to your computer using a USB cable.
- Open Arduino IDE on your computer.
- Choose the correct ESP8266 board, such as (e.g. NodeMCU 1.0 (ESP-12E Module)), and its respective COM port.
- Upload the code to the ESP8266 using the Arduino IDE.
- Wait a few seconds and then press and release the button.
- Check the Serial Monitor to view the results.
The event of pressing and holding down the button will only be detected when the button has not been released.
How To Detect Both Long Press and Short Press
Short Press and Long Press after released
Detailed Instructions
To get started with ESP8266 on Arduino IDE, follow these steps:
- Check out the how to setup environment for ESP8266 on Arduino IDE tutorial if this is your first time using ESP8266.
- Wire the components as shown in the diagram.
- Connect the ESP8266 board to your computer using a USB cable.
- Open Arduino IDE on your computer.
- Choose the correct ESP8266 board, such as (e.g. NodeMCU 1.0 (ESP-12E Module)), and its respective COM port.
- Upload the code to ESP8266 using the Arduino IDE.
- Press the button for a long and short duration.
- Check out the result on the Serial Monitor.
※ NOTE THAT:
The Serial Monitor may display multiple short press detections when a long press is made. This is the expected behavior of the button and is referred to as the "chattering phenomenon". The issue will be addressed in the final part of this tutorial.
Short Press and Long Press During pressing
Detailed Instructions
To get started with ESP8266 on Arduino IDE, follow these steps:
- Check out the how to setup environment for ESP8266 on Arduino IDE tutorial if this is your first time using ESP8266.
- Wire the components as shown in the diagram.
- Connect the ESP8266 board to your computer using a USB cable.
- Open Arduino IDE on your computer.
- Choose the correct ESP8266 board, such as (e.g. NodeMCU 1.0 (ESP-12E Module)), and its respective COM port.
- Upload the code to your ESP8266 using the Arduino IDE.
- Press the button for a short and long duration.
- Check out the result on the Serial Monitor.
※ NOTE THAT:
The Serial Monitor might display multiple short presses being detected when a long press is made. This is the expected behavior of the button and is referred to as the "chattering phenomenon". A solution to this issue will be provided in the final section of this tutorial.
Long Press and Short Press with Debouncing
It is essential to implement debouncing on the button in various applications.
Debouncing can be tricky, particularly when multiple buttons are involved. To make it simpler for novices, we have created a library called ezButton.
We will use this library in the codes below
Short Press and Long Press with debouncing after released
Detailed Instructions
To get started with ESP8266 on Arduino IDE, follow these steps:
- Check out the how to setup environment for ESP8266 on Arduino IDE tutorial if this is your first time using ESP8266.
- Wire the components as shown in the diagram.
- Connect the ESP8266 board to your computer using a USB cable.
- Open Arduino IDE on your computer.
- Choose the correct ESP8266 board, such as (e.g. NodeMCU 1.0 (ESP-12E Module)), and its respective COM port.
- To install the ezButton library, refer to How To.
- Upload the code to ESP8266 using the Arduino IDE.
- Press and hold the button for a short or long period of time.
- Check out the result on the Serial Monitor.
Short Press and Long Press with debouncing During Pressing
Detailed Instructions
- Wire the components as shown in the diagram.
- Connect the ESP8266 board to your computer using a USB cable.
- Open Arduino IDE on your computer.
- Choose the correct ESP8266 board, such as (e.g. NodeMCU 1.0 (ESP-12E Module)), and its respective COM port.
- Get the ezButton library installed. Refer to How To for instructions.
- Utilize the Arduino IDE to upload the code to the ESP8266.
- Press and hold the button, then release it.
- Check the Serial Monitor to observe the outcome.
Video Tutorial
Why Needs Long Press and Short Press
- To minimize the quantity of buttons, one button can be used for multiple purposes. For example, a short press can be used to switch operation modes, and a long press can be used to turn off the device.
- Long presses are used to prevent accidental presses. For instance, some devices use a button for factory reset. If it is pressed accidentally, it can be hazardous. To avoid this, the device is designed so that factory reset is only activated when the button is long-pressed (e.g. for more than 5 seconds).