ESP8266 - LM35 Temperature Sensor
This tutorial instructs you how to use ESP8266 to read the temperature from LM35 sensor. In detail, we will learn:
- How to connect ESP8266 to LM35 temperature sensor.
- How to program ESP8266 to get the temperature from LM35 sensor.
Hardware Preparation
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables .
Overview of LM35 Temperature Sensor
The LM35 Temperature Sensor Pinout
The LM35 temperature sensor has three pins:
- GND pin: This should be connected to the ground (0V)
- VCC pin: This should be connected to the VCC (5V)
- OUT pin: This signal pin gives an output voltage that is linearly proportional to the temperature, and should be connected to an analog pin on ESP8266.
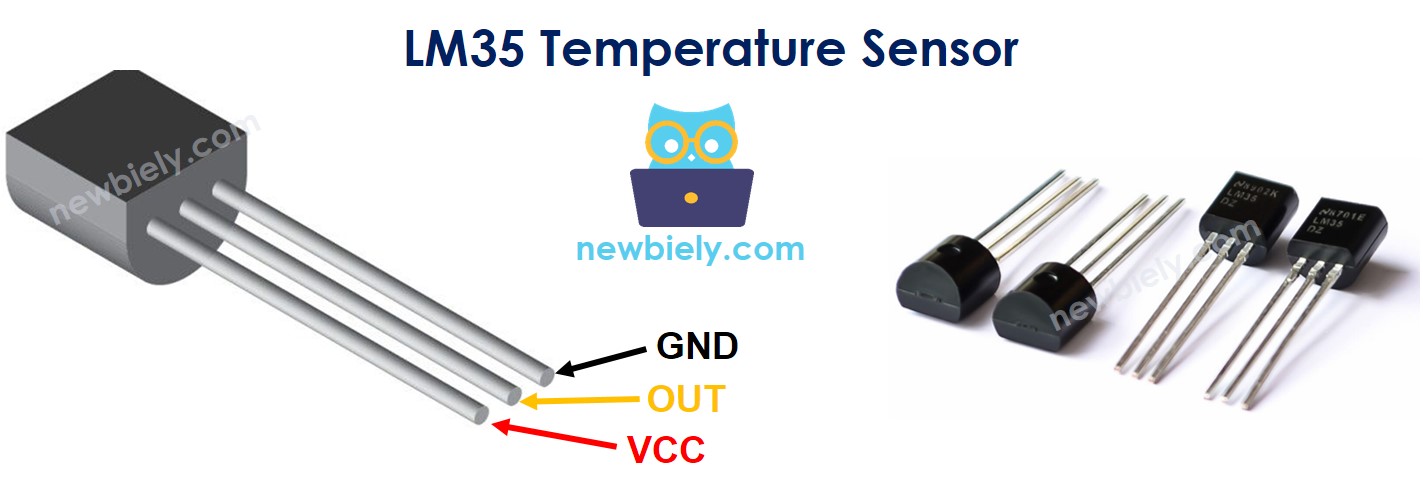
How It Works
The LM35 produces a voltage that is linearly proportional to the Centigrade temperature. The output of the LM35 has a scale factor of 10 mV/°C. This implies that the temperature can be determined by dividing the voltage (in mV) at the output pin by 10.
Wiring Diagram
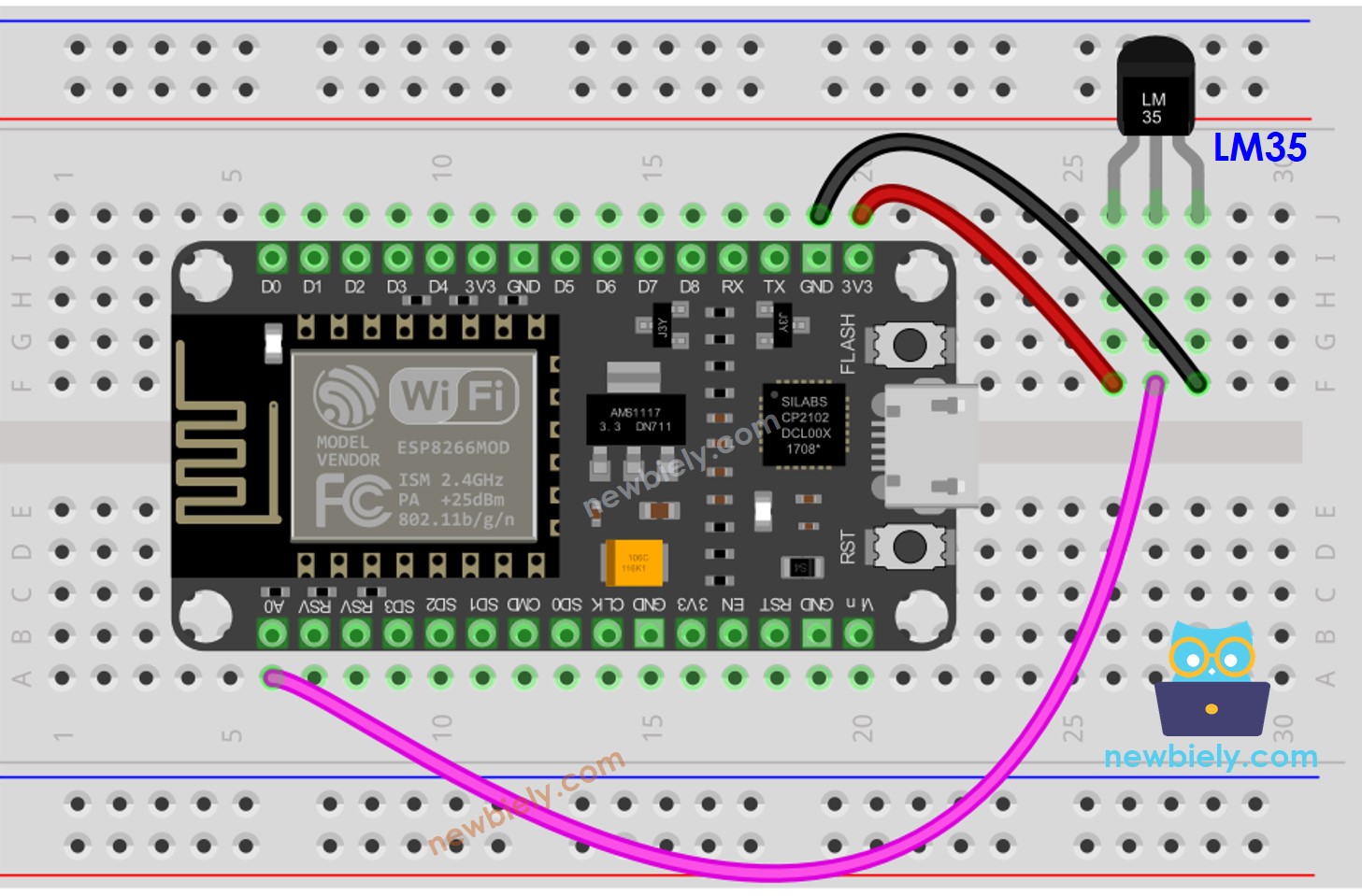
This image is created using Fritzing. Click to enlarge image
See more in ESP8266's pinout and how to supply power to the ESP8266 and other components.
How To Program For LM35 Temperature Sensor
- Retrieve the ADC value from the temperature sensor by utilizing the analogRead() function.
- Transform the ADC value into a voltage in millivolts.
- Transform the voltage into Celsius temperature.
- Optionally, convert Celsius to Fahrenheit.
ESP8266 Code
Detailed Instructions
To get started with ESP8266 on Arduino IDE, follow these steps:
- Check out the how to setup environment for ESP8266 on Arduino IDE tutorial if this is your first time using ESP8266.
- Wire the components as shown in the diagram.
- Connect the ESP8266 board to your computer using a USB cable.
- Open Arduino IDE on your computer.
- Choose the correct ESP8266 board, such as (e.g. NodeMCU 1.0 (ESP-12E Module)), and its respective COM port.
- Copy the code and open it with the Arduino IDE.
- Click the Upload button in the IDE to upload the code to the ESP8266.
- Hold the sensor in your hand.
- Check the results on the Serial Monitor.
※ NOTE THAT:
This tutorial uses the analogRead() function to get data from an ADC (Analog-to-Digital Converter) that's connected to a sensor or another part. The ESP8266's ADC works well for projects where you don't need very precise readings. But remember, the ESP8266's ADC isn't very accurate for detailed measurements. If your project needs to be very precise, you might want to use a separate ADC like the ADS1115 with the ESP8266, or use Arduino like the Arduino Uno R4 WiFi, which has a more reliable ADC.