ESP8266 - Button - Debounce
When a button is pressed or released, or a switch is flipped, newbies often assume that its state changes from LOW to HIGH or HIGH to LOW. In reality, this is not the case. Due to mechanical and physical characteristics, the state of the button (or switch) may fluctuate between LOW and HIGH multiple times per single user's action. This is known as chattering. Chattering can cause a single press to be read as multiple presses, leading to malfunctioning in certain applications.
The method to prevent this problem is referred as debouncing or debounce. This tutorial instructs you how to do it when using the button with ESP8266. We will learn though the below steps:
ESP8266 code without debouncing a button.
ESP8266 code with debouncing a button.
ESP8266 code with debouncing a button by using the ezButton library.
ESP8266 code with debouncing for multiple buttons.
Or you can buy the following kits:
Disclosure: Some of the links provided in this section are Amazon affiliate links. We may receive a commission for any purchases made through these links at no additional cost to you.
Additionally, some of these links are for products from our own brand,
DIYables .
If you are not familiar with buttons (including pinouts, functioning, and programming), the following tutorials can help you:
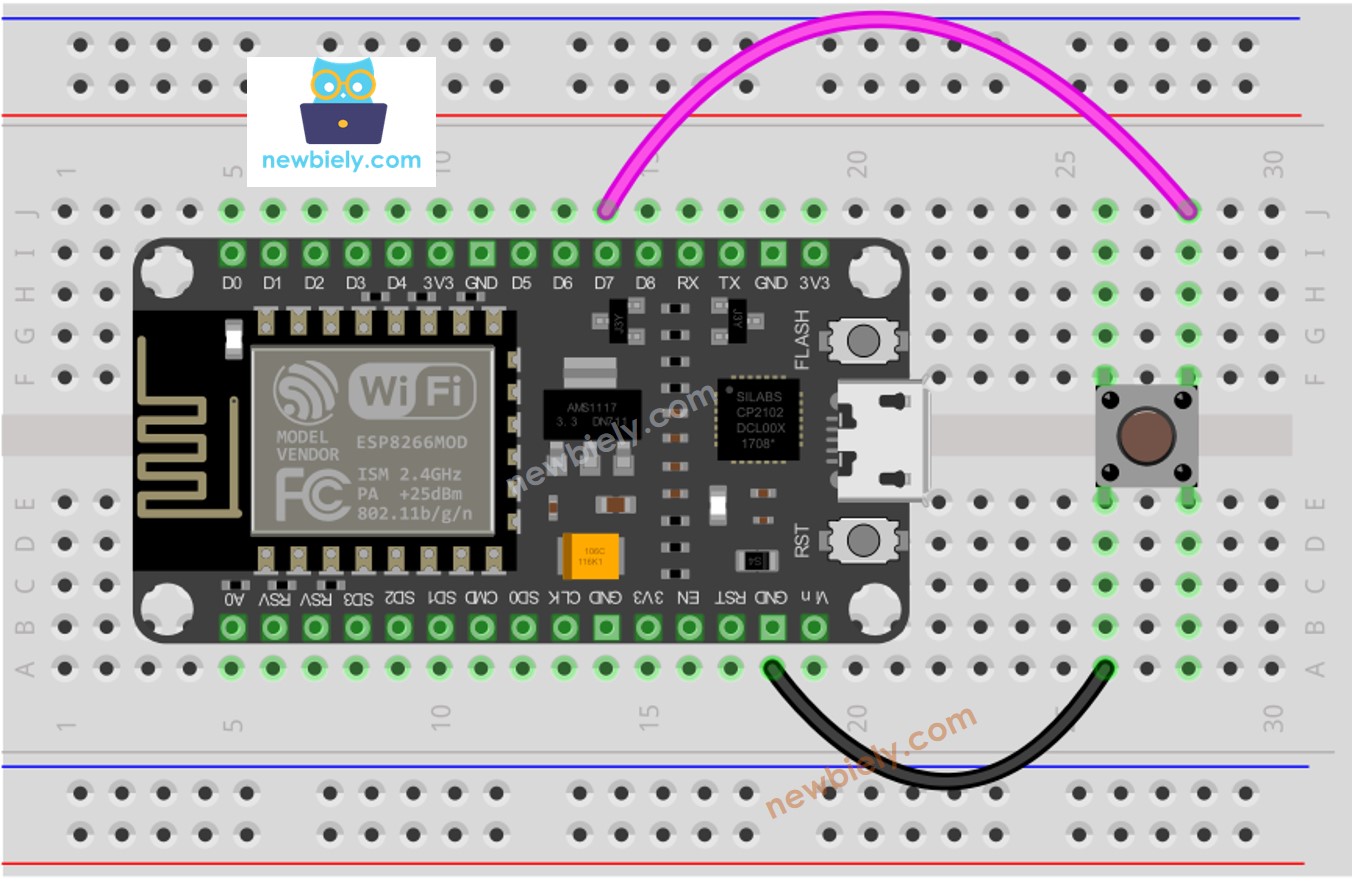
This image is created using Fritzing. Click to enlarge image
Let us take a look at the ESP8266 code for both WITH and WITHOUT debounce and compare their respective behaviors.
Before exploring debouncing, take a look at the code without it and observe its behavior.
To get started with ESP8266 on Arduino IDE, follow these steps:
Wire the components as shown in the diagram.
Connect the ESP8266 board to your computer using a USB cable.
Open Arduino IDE on your computer.
Choose the correct ESP8266 board, such as (e.g. NodeMCU 1.0 (ESP-12E Module)), and its respective COM port.
Connect your ESP8266 to your computer using a USB cable.
Launch the Arduino IDE, select the appropriate board and port.
Copy the code below and open it in the Arduino IDE.
#define BUTTON_PIN D7
int prev_button_state = LOW;
int button_state;
void setup() {
Serial.begin(9600);
pinMode(BUTTON_PIN, INPUT_PULLUP);
}
void loop() {
button_state = digitalRead(BUTTON_PIN);
if (prev_button_state == HIGH && button_state == LOW)
Serial.println("The button is pressed");
else if (prev_button_state == LOW && button_state == HIGH)
Serial.println("The button is released");
prev_button_state = button_state;
}
The button is pressed
The button is pressed
The button is pressed
The button is released
The button is released
You may see that, sometime, you only pressed and released the button one time. Nevertheless, ESP8266 perceives it as multiple presses and releases. This is the chattering phenomenon mentioned at the beginning of the tutorial. Let's see how to fix it in the next part.
The below code applies the method called debounce to prevent the chattering phenomenon.
#define BUTTON_PIN D7
#define DEBOUNCE_TIME 50
int lastSteadyState = LOW;
int lastFlickerableState = LOW;
int button_state;
unsigned long lastDebounceTime = 0;
void setup() {
Serial.begin(9600);
pinMode(BUTTON_PIN, INPUT_PULLUP);
}
void loop() {
button_state = digitalRead(BUTTON_PIN);
if (button_state != lastFlickerableState) {
lastDebounceTime = millis();
lastFlickerableState = button_state;
}
if ((millis() - lastDebounceTime) > DEBOUNCE_TIME) {
if(lastSteadyState == HIGH && button_state == LOW)
Serial.println("The button is pressed");
else if(lastSteadyState == LOW && button_state == HIGH)
Serial.println("The button is released");
lastSteadyState = button_state;
}
}
Wire the components as shown in the diagram.
Connect the ESP8266 board to your computer using a USB cable.
Open Arduino IDE on your computer.
Choose the correct ESP8266 board, such as (e.g. NodeMCU 1.0 (ESP-12E Module)), and its respective COM port.
Copy the code above and open it with the Arduino IDE.
Click the Upload button on the Arduino IDE to compile and upload the code to the ESP8266.
Open the Serial Monitor.
Hold down the button for a few seconds, then let go.
Check the Serial Monitor to view the outcome.
The button is pressed
The button is released
As you can observe, you only pressed and released the button once. ESP8266 is able to detect it as a single press and release, thus eliminating any unnecessary chatter.
We have developed a library, ezButton, to make it simpler for those just starting out, especially when they are using multiple buttons. You can find out more about the ezButton library here.
#include <ezButton.h>
ezButton button(D7);
void setup() {
Serial.begin(9600);
button.setDebounceTime(50);
}
void loop() {
button.loop();
if(button.isPressed())
Serial.println("The button is pressed");
if(button.isReleased())
Serial.println("The button is released");
}
#include <ezButton.h>
ezButton button1(D5);
ezButton button2(D6);
ezButton button3(D7);
void setup() {
Serial.begin(9600);
button1.setDebounceTime(50);
button2.setDebounceTime(50);
button3.setDebounceTime(50);
}
void loop() {
button1.loop();
button2.loop();
button3.loop();
if(button1.isPressed())
Serial.println("The button 1 is pressed");
if(button1.isReleased())
Serial.println("The button 1 is released");
if(button2.isPressed())
Serial.println("The button 2 is pressed");
if(button2.isReleased())
Serial.println("The button 2 is released");
if(button3.isPressed())
Serial.println("The button 3 is pressed");
if(button3.isReleased())
Serial.println("The button 3 is released");
}
The schematic for the code above:. The illustration of the wiring for the code:. The representation of the connections for the code:
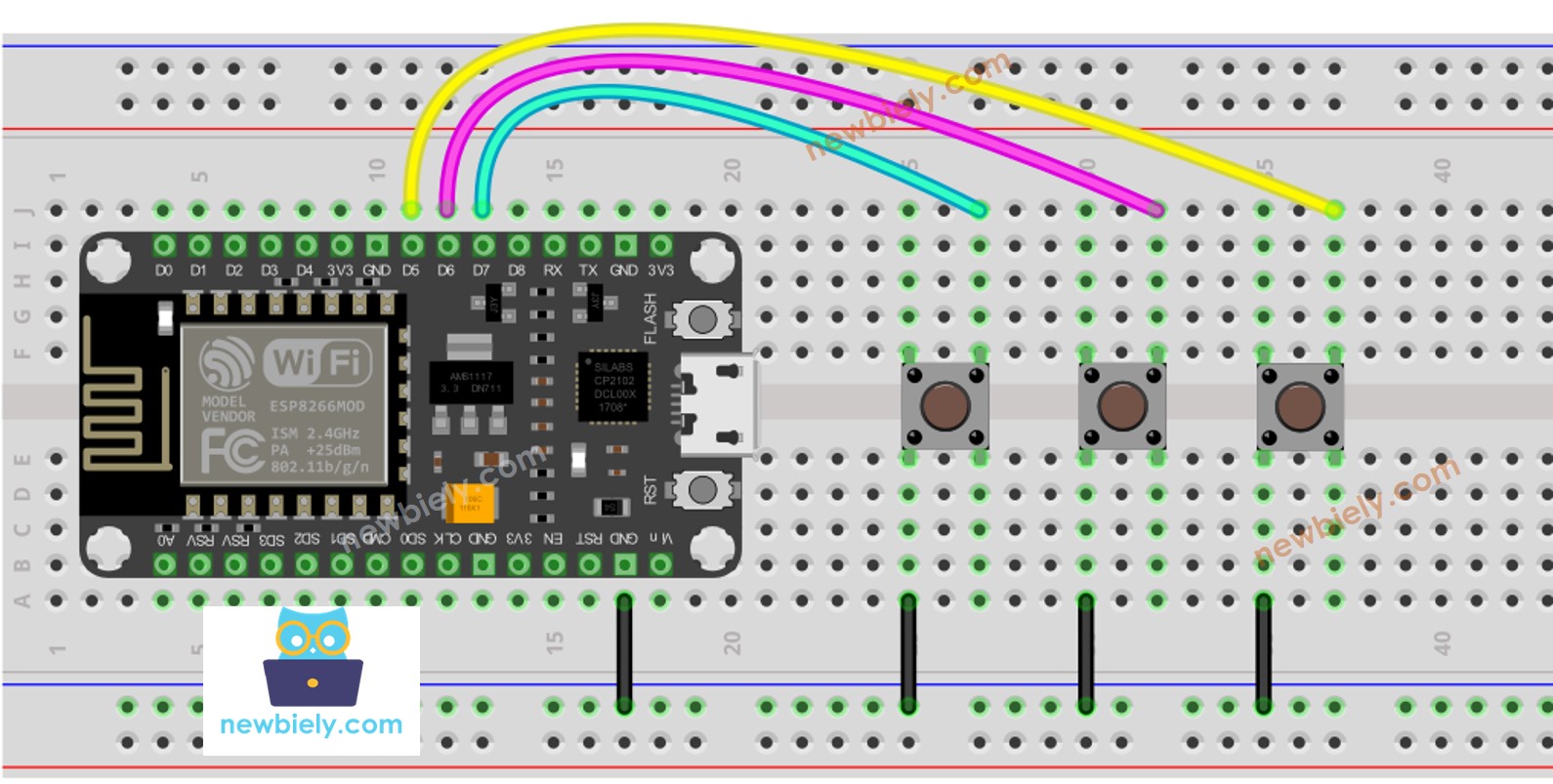
This image is created using Fritzing. Click to enlarge image
See more in ESP8266's pinout and how to supply power to the ESP8266 and other components.
The debounce technique can be used for switches, touch sensors, and more.