ESP8266 - LED - Blink Without Delay
Let us imagine that ESP8266 has two jobs to accomplish: blinking an LED and monitoring the state of a button which can be pressed at any time. If we use the delay() function (as explained in a past tutorial), ESP8266 may miss out on some of the button presses. In other words, ESP8266 would not be able to fully execute the second task.
This tutorial instructs you how to make ESP8266 blink an LED and monitor the state of a button without missing any of its presses.
We will go through three examples and compare the distinctions between them:
- ESP8266 blinking an LED with the delay() function
- ESP8266 blinking an LED with the millis() function
- ESP8266 blinking an LED with the ezLED library
This method is not just limited to blinking LED and checking the button's state. It generally allows ESP8266 to perform multiple tasks simultaneously without blocking each other.
Hardware Preparation
Or you can buy the following kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables .
Wiring Diagram
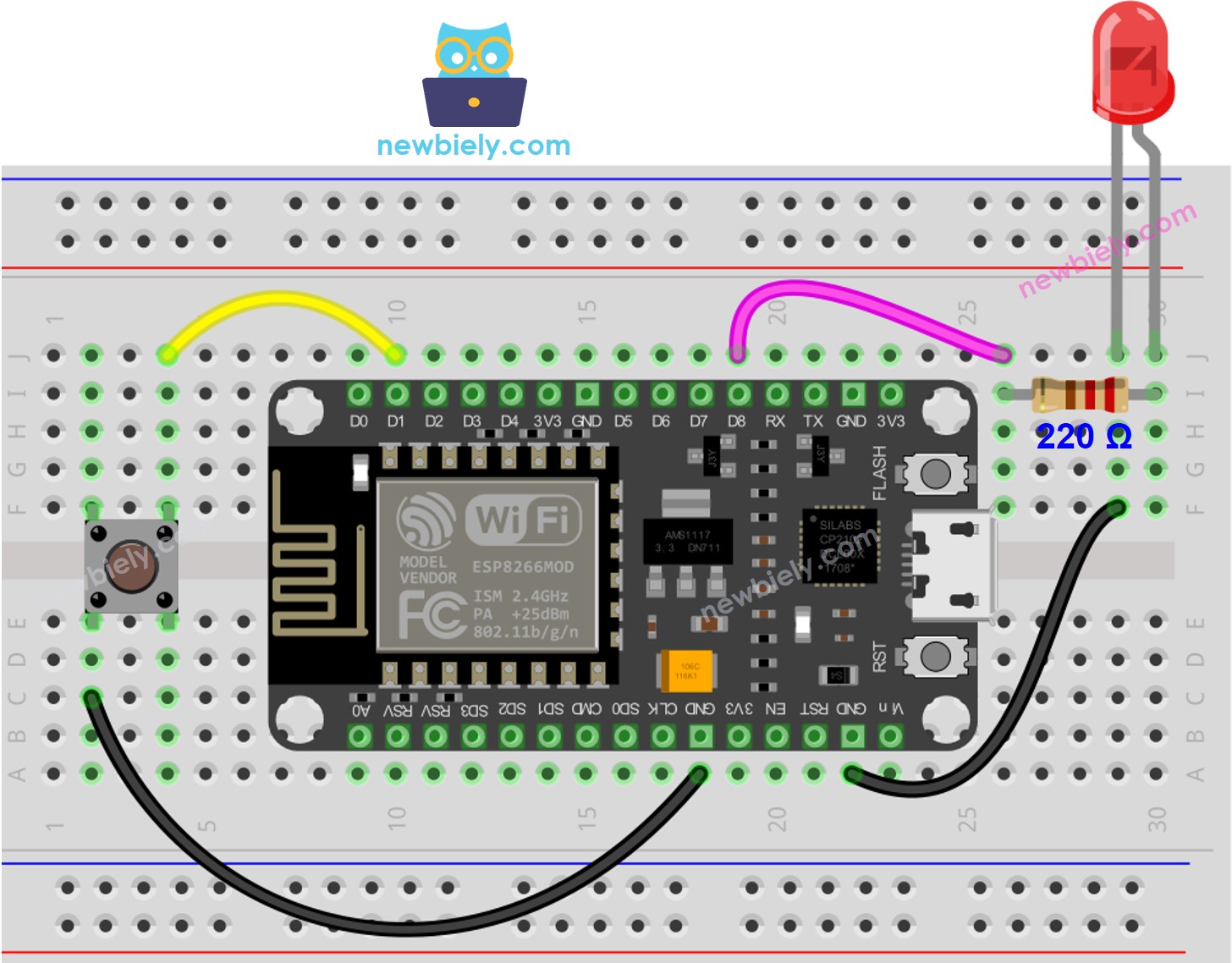
This image is created using Fritzing. Click to enlarge image
See more in ESP8266's pinout and how to supply power to the ESP8266 and other components.
ESP8266 Code - With Delay
Detailed Instructions
To get started with ESP8266 on Arduino IDE, follow these steps:
- Check out the how to setup environment for ESP8266 on Arduino IDE tutorial if this is your first time using ESP8266.
- Wire the components as shown in the diagram.
- Connect the ESP8266 board to your computer using a USB cable.
- Open Arduino IDE on your computer.
- Choose the correct ESP8266 board, such as (e.g. NodeMCU 1.0 (ESP-12E Module)), and its respective COM port.
- Plug the USB cable into the ESP8266 and PC.
- Launch the Arduino IDE, select the correct board and port.
- Copy the code and open it in the Arduino IDE.
- Click the Upload button in the Arduino IDE to compile and upload the code to the ESP8266.
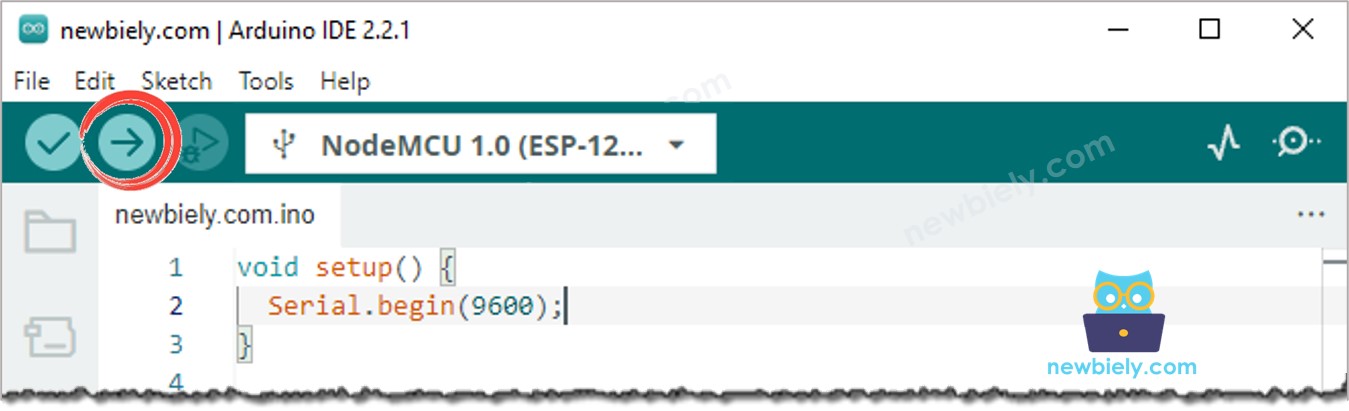
- Open the Serial Monitor.
- Press the button four times.
- Check out the LED; it will alternate between being on and off every second.
- Check the output in the Serial Monitor.
- On Serial Monitor, some pressing times were not registered. This is because, while in delay, ESP8266 is unable to perform any tasks. Consequently, it is not able to detect the pressing event.
ESP8266 Code - Without Delay
Detailed Instructions
- Wire the components as shown in the diagram.
- Connect the ESP8266 board to your computer using a USB cable.
- Open Arduino IDE on your computer.
- Choose the correct ESP8266 board, such as (e.g. NodeMCU 1.0 (ESP-12E Module)), and its respective COM port.
- Execute the code and press the button 4 times.
- Check out the LED, which will alternate between ON and OFF at regular intervals of one second.
- Check the output in the Serial Monitor.
- All occurrences of urgent matters were identified.
Code Explanation
Check out the line-by-line explanation contained in the comments of the source code!
Adding More Tasks
The ESP8266 code below does
- Makes two LEDs blink with different intervals.
- Checks the state of the button.
Video Tutorial
Extendability
This method allows ESP8266 to perform multiple tasks simultaneously, without one task blocking another. For instance, sending a request to the Internet and waiting for the response, while also blinking LED indicators and monitoring the cancel button.