ESP8266 - Button - Servo Motor
This tutorial instructs you how to use an ESP8266 and a button to control a servo motor. In detail:
If the ESP8266 detects the button being pressed, the ESP8266 controls the servo motor to rotate to 90 degrees.
If the ESP8266 detects the button being pressed again, the ESP8266 controls the servo motor to return to 0 degrees.
The above process is repeated over and over again.
The tutorial has two sections:
Or you can buy the following kits:
Disclosure: Some of the links provided in this section are Amazon affiliate links. We may receive a commission for any purchases made through these links at no additional cost to you.
Additionally, some of these links are for products from our own brand,
DIYables .
If you are unfamiliar with servo motors and buttons (including pinouts, how they work, and how to program them), the following tutorials can help:
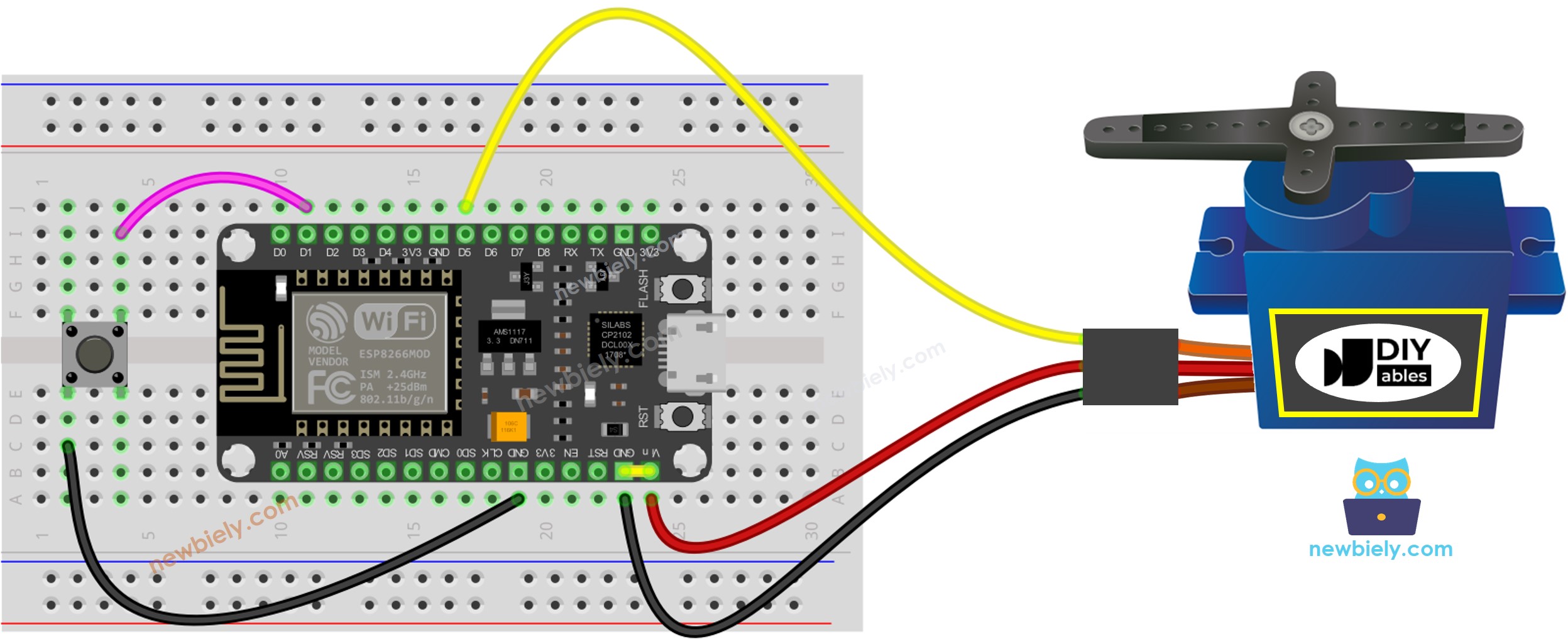
This image is created using Fritzing. Click to enlarge image
Please note that the wiring diagram shown above is only suitable for a servo motor with low torque. In case the motor vibrates instead of rotating, an external power source must be utilized to provide more power for the servo motor. The below demonstrates the wiring diagram with an external power source for servo motor.
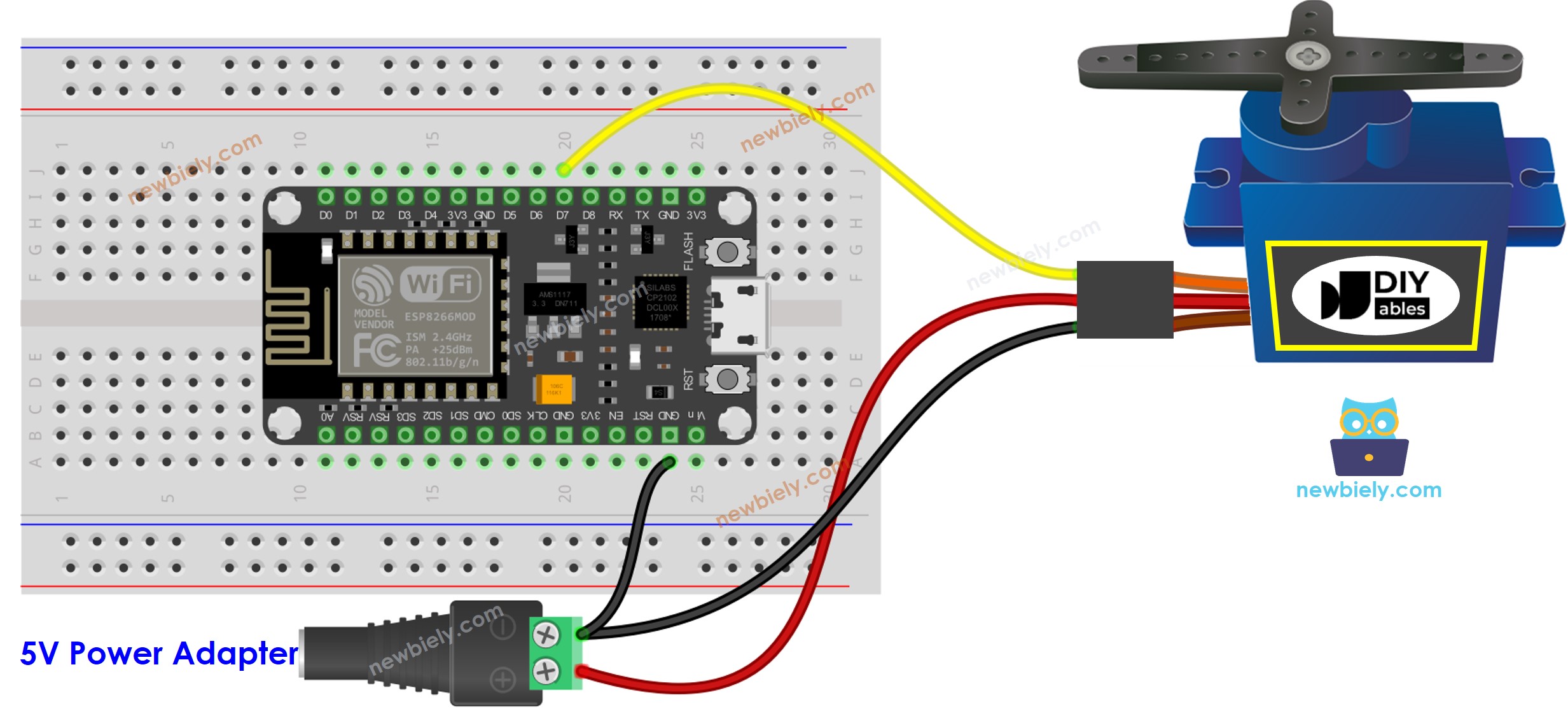
This image is created using Fritzing. Click to enlarge image
See more in ESP8266's pinout and how to supply power to the ESP8266 and other components.
Please do not forget to connect GND of the external power to GND of ESP8266.
#include <Servo.h>
#define BUTTON_PIN D1
#define SERVO_PIN D5
Servo servo;
int angle = 0;
int prev_button_state;
int button_state;
void setup() {
Serial.begin(9600);
pinMode(BUTTON_PIN, INPUT_PULLUP);
servo.attach(SERVO_PIN);
servo.write(angle);
button_state = digitalRead(BUTTON_PIN);
}
void loop() {
prev_button_state = button_state;
button_state = digitalRead(BUTTON_PIN);
if(prev_button_state == HIGH && button_state == LOW) {
Serial.println("The button is pressed");
if(angle == 0)
angle = 90;
else
if(angle == 90)
angle = 0;
servo.write(angle);
}
}
To get started with ESP8266 on Arduino IDE, follow these steps:
Wire the components as shown in the diagram.
Connect the ESP8266 board to your computer using a USB cable.
Connect the ESP8266 NodeMCU to the computer using a USB cable.
Open Arduino IDE on your computer.
Choose the correct ESP8266 board, such as (e.g. NodeMCU 1.0 (ESP-12E Module)), and its respective COM port.
Copy the above code and open it in the Arduino IDE.
Click the Upload button in the Arduino IDE to compile and upload the code to the ESP8266.
Press the button multiple times.
Check out the changes in the servo motor.
※ NOTE THAT:
In practice, the code mentioned above does not always work correctly. To ensure it functions properly, we need to debounce for the button. Debouncing the button can be difficult for those new to ESP8266. Fortunately, the ezButton library makes this process much simpler.
Why is debouncing necessary?. See ESP8266 - Button Debounce tutorial for more information.
#include <Servo.h>
#include <ezButton.h>
#define BUTTON_PIN D1
#define SERVO_PIN D5
ezButton button(BUTTON_PIN);
Servo servo;
int angle = 0;
void setup() {
Serial.begin(9600);
button.setDebounceTime(50);
servo.attach(SERVO_PIN);
servo.write(angle);
}
void loop() {
button.loop();
if (button.isPressed()) {
if (angle == 0)
angle = 90;
else if (angle == 90)
angle = 0;
Serial.print("The button is pressed => rotate servo to ");
Serial.print(angle);
Serial.println("°");
servo.write(angle);
}
}
Install the ezButton library. Refer to
How To for instructions.
Copy the above code and open it in the Arduino IDE.
Click the Upload button in the Arduino IDE to upload the code to the ESP8266.
Push the button multiple times.
Check out the changes in the servo motor.