ESP8266 - Stepper Motor Limit Switch
This tutorial instructs you how to use ESP8266 to control a stepper motor via a limit switch and an L298N driver. Specifically, we will cover:
- How to program ESP8266 to stop the stepper motor when a limit switch is touched.
- How to program ESP8266 to change direction of the stepper motor when a limit switch is touched.
- How to program ESP8266 to change direction of the stepper motor by two limit switches installed in opposite position.
Hardware Preparation
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables .
Overview of Stepper Motor and Limit Switch
If you are unfamiliar with stepper motor and limit switch (including pinout, functioning, programming, etc.), the following tutorials can provide you with more information:
- ESP8266 - Limit Switch tutorial
- ESP8266 - Stepper Motor tutorial
Wiring Diagram
This tutorial provides the wiring diagram for two cases: One stepper motor + one limit switch, One stepper motor + two limit switches.
- Wiring Diagram between ESP8266, stepper motor and, and a limit switch.
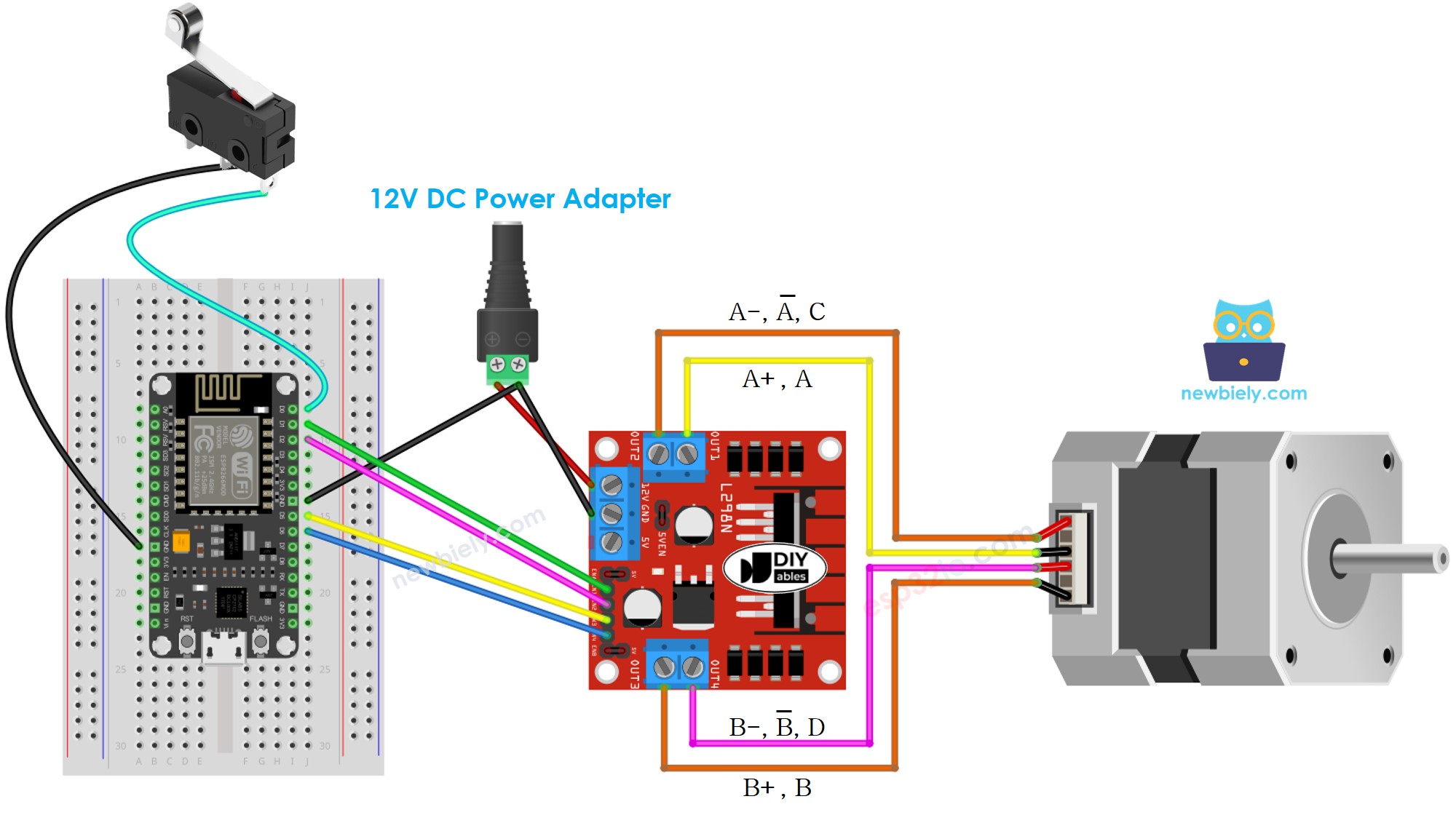
This image is created using Fritzing. Click to enlarge image
- Wiring Diagram between ESP8266, stepper motor and, and two limit switches.
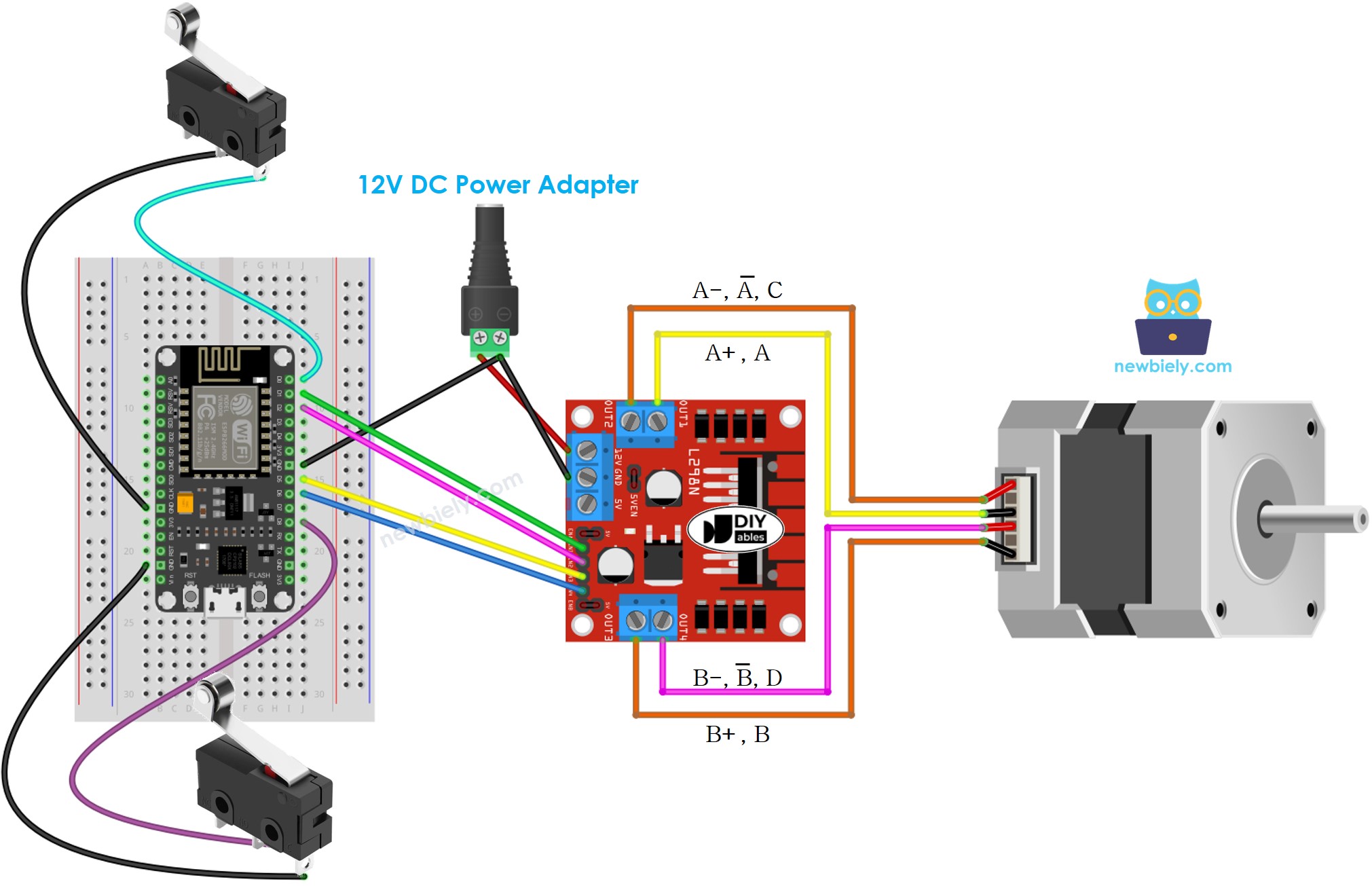
This image is created using Fritzing. Click to enlarge image
See more in ESP8266's pinout and how to supply power to the ESP8266 and other components.
※ NOTE THAT:
The wiring connection between the stepper motor and L298N may vary depending on the type of stepper motor. Therefore, it is important to take a look at this ESP8266 - Stepper Motor tutorial to understand how to make the connection.
ESP8266 Code - Stop Stepper Motor by a Limit Switch
There are various methods to stop a stepper motor:
- Invoke stepper.stop() function: This will not cause an instantaneous stop, but rather a gradual one
- Omit calling stepper.run() function: This will result in an immediate stop of the stepper motor
The code below will cause a stepper motor to rotate continuously until a limit switch is engaged, at which point it will stop immediately.
Detailed Instructions
To get started with ESP8266 on Arduino IDE, follow these steps:
- Check out the how to setup environment for ESP8266 on Arduino IDE tutorial if this is your first time using ESP8266.
- Wire the components as shown in the diagram.
- Connect the ESP8266 board to your computer using a USB cable.
- Open Arduino IDE on your computer.
- Choose the correct ESP8266 board, such as (e.g. NodeMCU 1.0 (ESP-12E Module)), and its respective COM port.
- Connect a USB cable to the ESP8266 and PC.
- Open the Arduino IDE, select the appropriate board and port.
- Click to the Libraries icon on the left bar of the Arduino IDE.
- Search for “ezButton”, then locate the button library provided by ArduinoGetStarted.com.
- Press the Install button to install ezButton library.
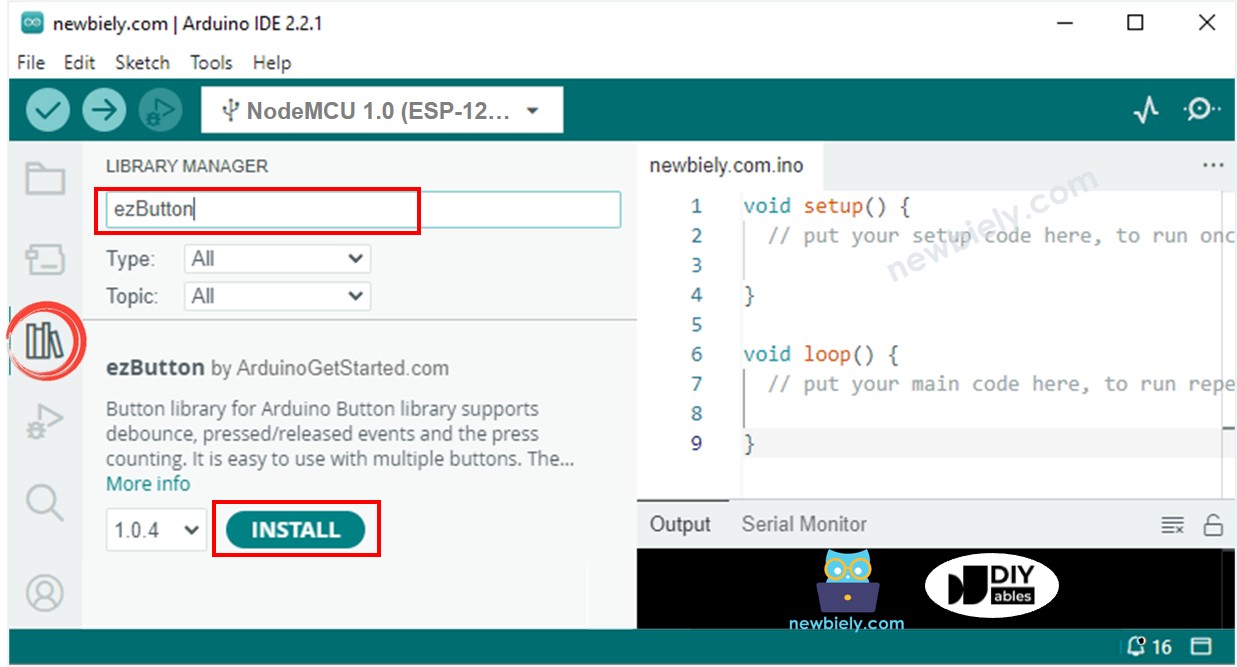
- Look up “AccelStepper” and locate the AccelStepper library created by Mike McCauley.
- Press the Install button to add the AccelStepper library.
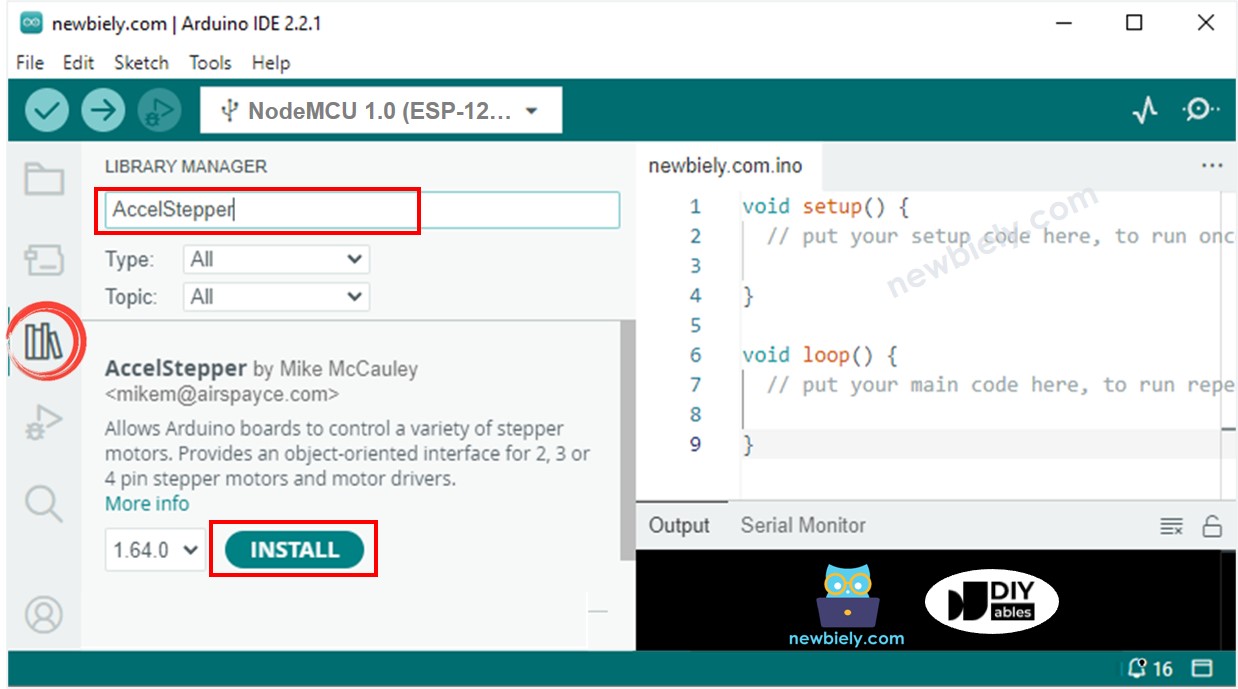
- Copy the code and open it with the Arduino IDE.
- Click the Upload button in order to transfer the code to the ESP8266.
- If the wiring is done correctly, the motor will rotate in a clockwise direction.
- Tap the limit switch and the motor will be stopped right away.
- The result that appears on the Serial Monitor should look like this.
Code Explanation
Check out the line-by-line explanation contained in the comments of the source code!
ESP8266 Code - Change Direction of Stepper Motor by a Limit Switch
The code below causes a stepper motor to rotate continuously, and it will reverse its direction when a limit switch is touched.
Detailed Instructions
To get started with ESP8266 on Arduino IDE, follow these steps:
- Check out the how to setup environment for ESP8266 on Arduino IDE tutorial if this is your first time using ESP8266.
- Wire the components as shown in the diagram.
- Connect the ESP8266 board to your computer using a USB cable.
- Open Arduino IDE on your computer.
- Choose the correct ESP8266 board, such as (e.g. NodeMCU 1.0 (ESP-12E Module)), and its respective COM port.
- Copy the code and open it with the Arduino IDE.
- Click the Upload button to send the code to the ESP8266.
- If the wiring is correct, the motor will rotate in a clockwise direction.
- When you touch the limit switch, the stepper motor's rotation will be reversed to an anti-clockwise direction.
- Touching the limit switch again will cause the stepper motor to rotate clockwise again.
- The output on the Serial Monitor should look like this.
ESP8266 Code - Change Direction of Stepper Motor by two Limit Switches
The code below causes a stepper motor to spin continuously, and it will reverse its direction when either of two limit switches is touched.
Detailed Instructions
- Wire the components as shown in the diagram.
- Connect the ESP8266 board to your computer using a USB cable.
- Open Arduino IDE on your computer.
- Choose the correct ESP8266 board, such as (e.g. NodeMCU 1.0 (ESP-12E Module)), and its respective COM port.
- Copy the code and open it with the Arduino IDE.
- Click the Upload button in the IDE to upload the code to the ESP8266.
- If the wiring is correct, the motor should spin in a clockwise direction.
- When you touch limit switch 1, the stepper motor's direction should switch to anti-clockwise.
- Touching limit switch 2 should cause the motor to reverse direction again, this time to clockwise.
- The result displayed on the Serial Monitor should look like the following.