ESP8266 - 74HC595 4-Digit 7-Segment Display
This tutorial instructs you how to use ESP8266 to control a 74HC595 4-digit 7-segment display module. In detailed, we will learn:
- How to connect ESP8266 to a 74HC595 4-digit 7-segment display module
- How to program ESP8266 to display integer numbers on a 74HC595 4-digit 7-segment display module
- How to program ESP8266 to display float numbers on a 74HC595 4-digit 7-segment display module
- How to program ESP8266 to display numbers, characters on a 74HC595 4-digit 7-segment display module
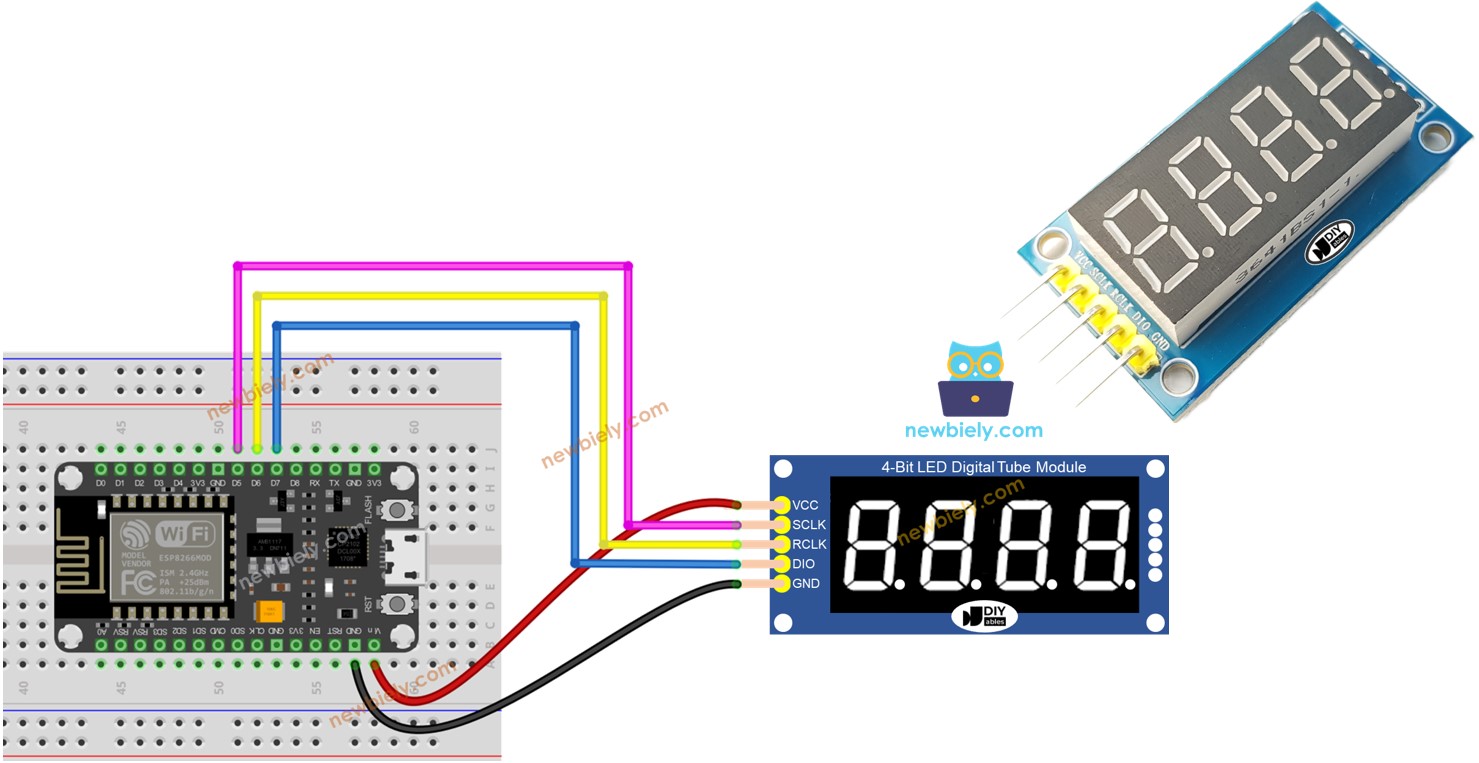
This tutorial will utilize a 4-dot 4-digit 7-segment display module capable of displaying float values. If you need to display a colon separator, please refer to the TM1637 4-digit 7-segment Display Module
Hardware Preparation
Or you can buy the following kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables .
Overview of 74HC595 4-digit 7-segment Display
An ideal module for displaying temperature or any float value is the 74HC595 4-digit 7-segment display. This module typically includes four 7-segment LEDs, four dot-shaped LEDs, and two 74HC595 drivers for each digit.
Pinout
The 74HC595 4-digit 7-segment display module includes 5 pins:
- SCLK pin: is a clock input pin. Connect to any digital pin on ESP8266.
- RCLK pin: is a clock input pin. Connect to any digital pin on ESP8266.
- DIO pin: is a Data I/O pin. Connect to any digital pin on ESP8266.
- VCC pin: supplies power to the module. Connect it to the 3.3V to 5V power supply.
- GND pin: is a ground pin.
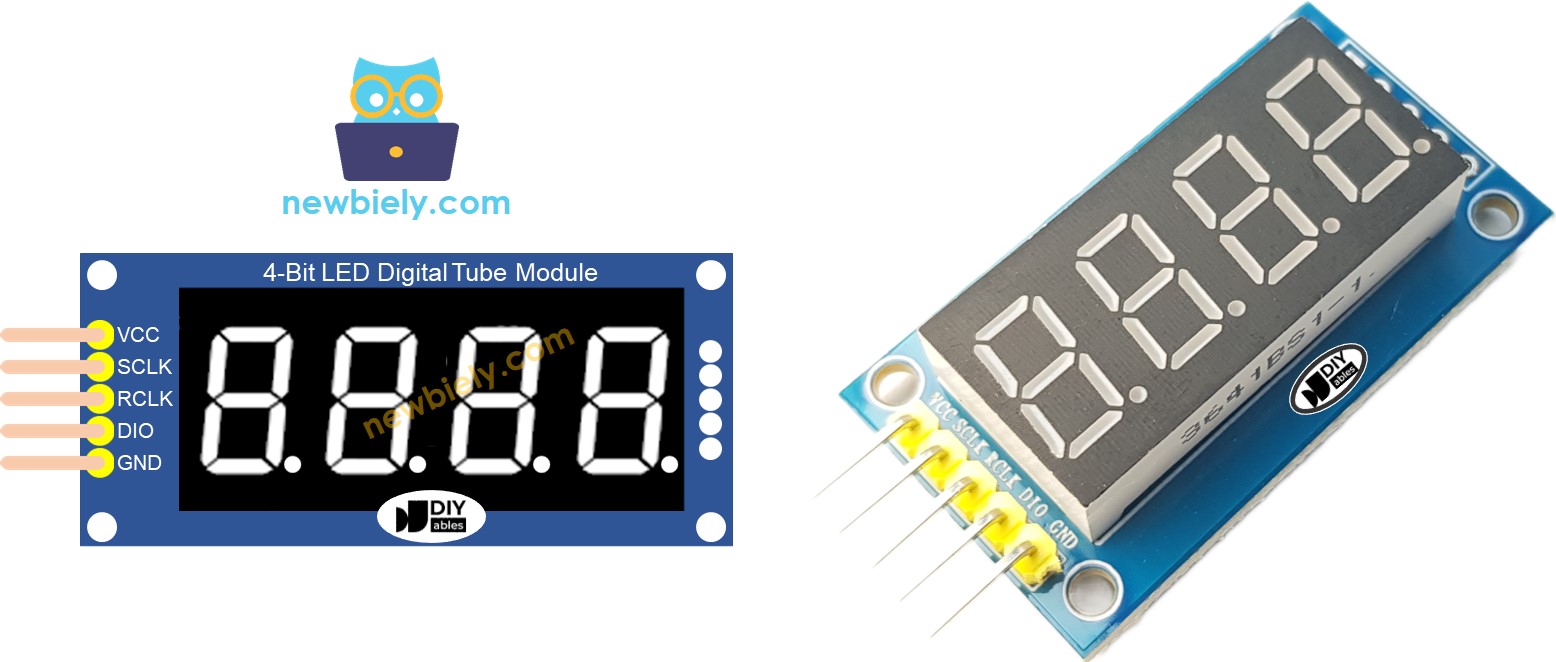
Wiring Diagram
The table below shown the wiring between ESP8266 pins and a 74HC595 4-digit 7-segment display pins:
ESP8266 | 74HC595 7-segment display |
---|---|
Vin | 5V |
D5 | SCLK |
D6 | RCLK |
D7 | DIO |
If you are using different pins, make sure to modify the pin numbers in the code accordingly.
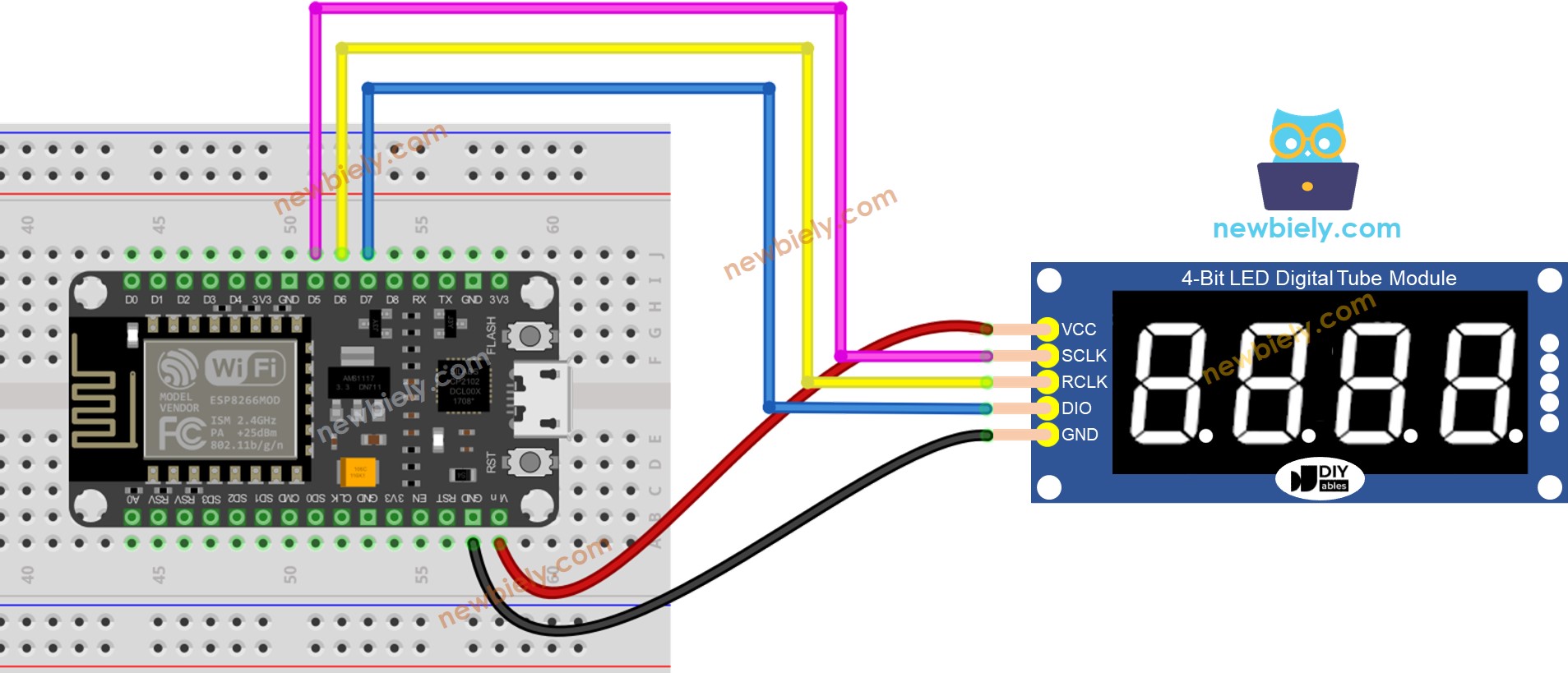
This image is created using Fritzing. Click to enlarge image
See more in ESP8266's pinout and how to supply power to the ESP8266 and other components.
Library Installation
To program easily for 74HC595 4-digit 7-segment Display, we need to install DIYables_4Digit7Segment_74HC595 library by DIYables.io. Follow the below steps to install the library:
- Click to the Libraries icon on the left bar of the Arduino IDE.
- Search “DIYables_4Digit7Segment_74HC595”, then find the DIYables_4Digit7Segment_74HC595 library by DIYables.io
- Click Install button.
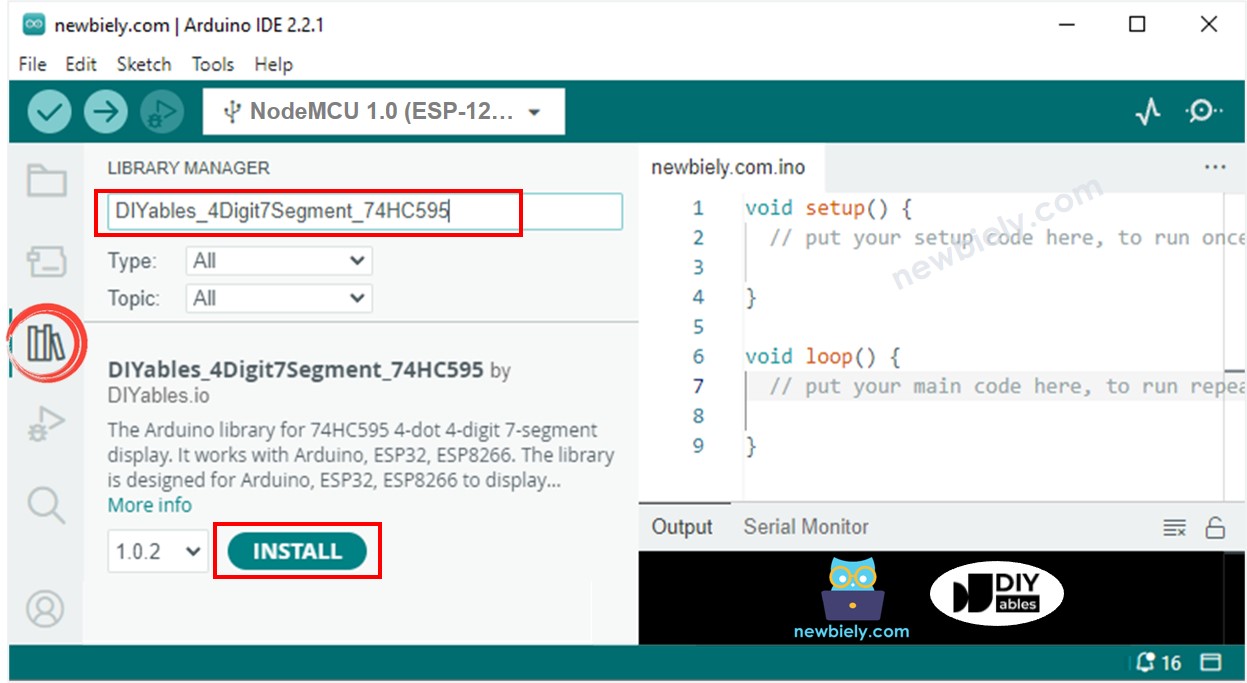
You can also see this library on Github
How To Program For 74HC595 4-digit 7-segment using ESP8266
- Include the library
- Define ESP8266's pins that connects to SCLK, RCLK and DIO of the display module. For example, pin D7, D6 and D5
- Create a display object of type DIYables_4Digit7Segment_74HC595
- Then you can display the integer numbers with the zero-padding option, supporting the negative number:
- You can display the float numbers with the decimal place, zero-padding options, supporting the negative number:
- You can also display number, decimal point, character digit-by-digit by using lower-level functions:
- Because the 74HC595 4-digit 7-segment module uses the multiplexing technique to control individual segments and LEDs, ESP8266 code MUST:
- Call display.show() function in the main loop
- Not use delay() function in the main loop
You can see more detail in the the library reference
ESP8266 Code - Display Integer
Detailed Instructions
To get started with ESP8266 on Arduino IDE, follow these steps:
- Check out the how to setup environment for ESP8266 on Arduino IDE tutorial if this is your first time using ESP8266.
- Wire the components as shown in the diagram.
- Connect the ESP8266 board to your computer using a USB cable.
- Open Arduino IDE on your computer.
- Choose the correct ESP8266 board, such as (e.g. NodeMCU 1.0 (ESP-12E Module)), and its respective COM port.
- Copy the above code and open with ESP8266 IDE
- Click Upload button on ESP8266 IDE to upload code to ESP8266
- See the states of the 7-segment display