ESP8266 - Buzzer
This tutorial instructs you how to program ESP8266 to control a 12V active buzzer to produce a loud sound. If you want to control 5V active/passive buzzer, please check out this ESP8266 Piezo Buzzer tutorial
Hardware Preparation
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables .
Overview of 12V Active Buzzer
The 12V Active Buzzer can produce a loud sound, which is suitable for the alarming system.
Pinout
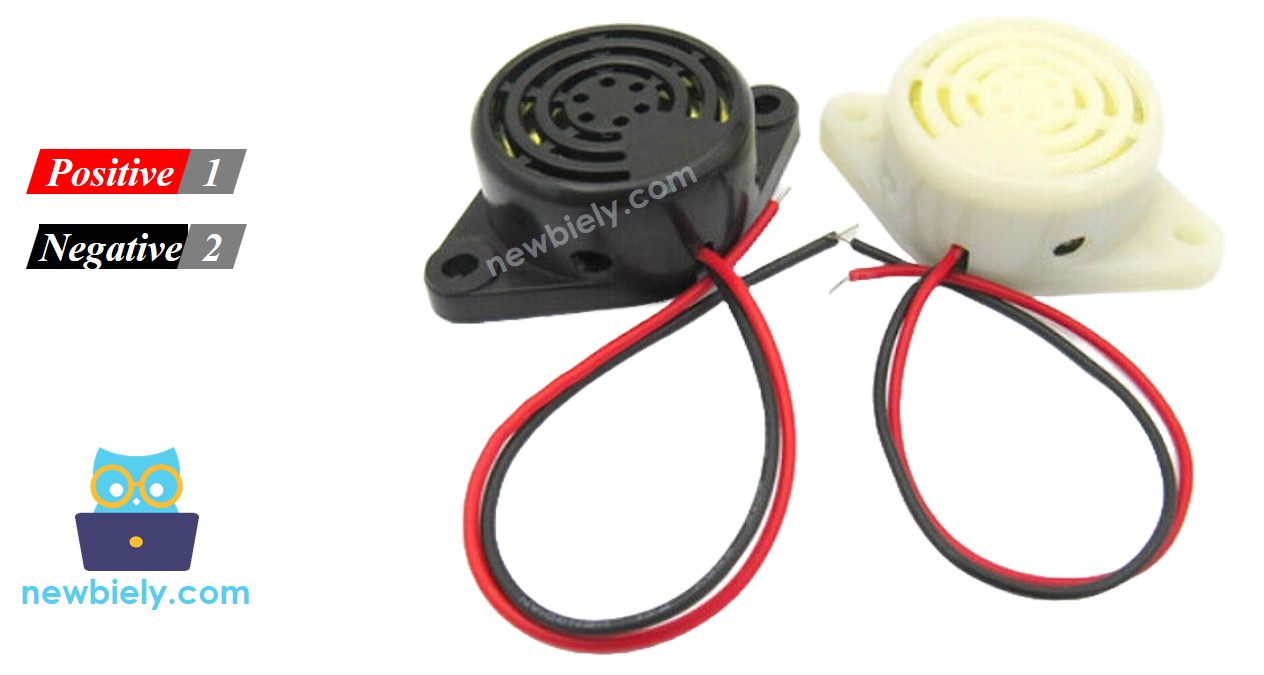
12V Active Buzzer usually has two pins:
- Negative (-) pin (black): needs to be connected to GND of DC power supply
- Positive (+) pin (red): needs to be connected to 12V of DC power supply
How to Control 12V Active Buzzer
If 12V active buzzer is powered by 12V power supply, it make sound. To control a 12V active buzzer, we need to use a relay in between ESP8266 and 12V active buzzer. ESP8266 can control the 12V active buzzer via the relay. If you do not know about relay (pinout, how it works, how to program ...), learn about relay in the ESP8266 - Relay tutorial
Wiring Diagram
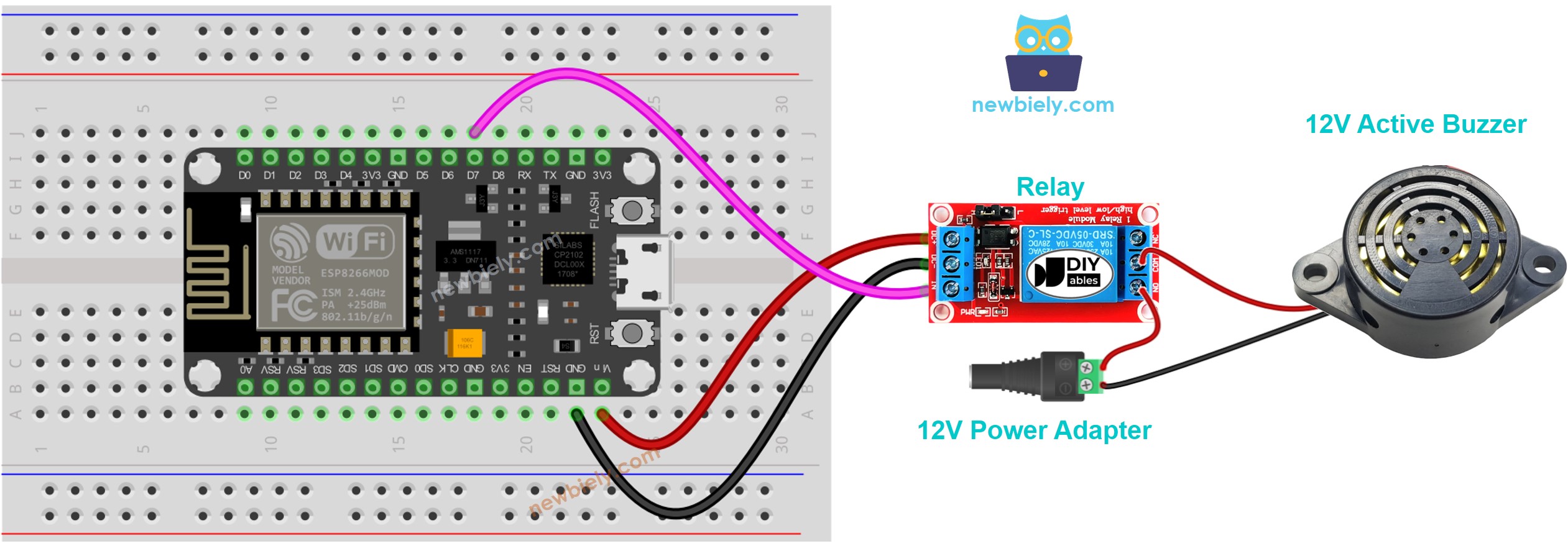
This image is created using Fritzing. Click to enlarge image
See more in ESP8266's pinout and how to supply power to the ESP8266 and other components.
ESP8266 Code
The below code repeatedly turns the 12V active buzzer ON in two seconds and OFF in five seconds,
Detailed Instructions
To get started with ESP8266 on Arduino IDE, follow these steps:
- Check out the how to setup environment for ESP8266 on Arduino IDE tutorial if this is your first time using ESP8266.
- Wire the components as shown in the diagram.
- Connect the ESP8266 board to your computer using a USB cable.
- Open Arduino IDE on your computer.
- Choose the correct ESP8266 board, such as (e.g. NodeMCU 1.0 (ESP-12E Module)), and its respective COM port.
- Connect ESP8266 to PC via USB cable
- Open Arduino IDE, select the right board and port
- Copy the above code and open with Arduino IDE
- Click Upload button on Arduino IDE to upload code to ESP8266
- See the 12V active buzzer's state
Code Explanation
Read the line-by-line explanation in comment lines of code!