ESP8266 - Button - Pump
This tutorial instructs you how to use ESP8266 to turn on a pump for a few seconds when a button is pressed, and then turn it off.
Hardware Preparation
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Disclosure: Some of the links provided in this section are Amazon affiliate links. We may receive a commission for any purchases made through these links at no additional cost to you.
Additionally, some of these links are for products from our own brand, DIYables.
Additionally, some of these links are for products from our own brand, DIYables.
Wiring Diagram
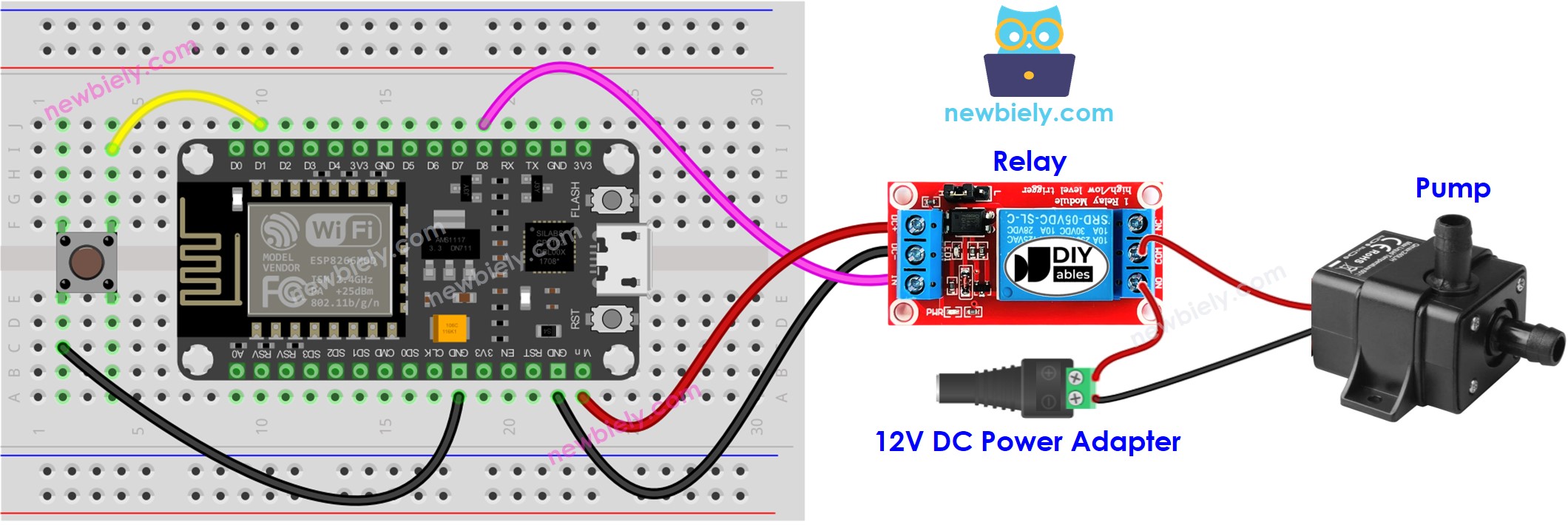
This image is created using Fritzing. Click to enlarge image
See more in ESP8266's pinout and how to supply power to the ESP8266 and other components.
ESP8266 Code
/*
* This ESP8266 NodeMCU code was developed by newbiely.com
*
* This ESP8266 NodeMCU code is made available for public use without any restriction
*
* For comprehensive instructions and wiring diagrams, please visit:
* https://newbiely.com/tutorials/esp8266/esp8266-button-pump
*/
#include <ezButton.h> // include ezButton library
#include <ezOutput.h> // include ezOutput library
ezOutput pump(A5); // create ezOutput object attached to pin A5
ezButton button(12); // create ezButton object attached to pin 12
void setup() {
Serial.begin(9600);
button.setDebounceTime(50); // set debounce time to 50 milliseconds
pump.low(); // turn pump off
}
void loop() {
pump.loop(); // MUST call the loop() function first
button.loop(); // MUST call the loop() function first
if (button.isPressed()) {
Serial.println("Pump is started");
pump.low();
pump.pulse(10000); // turn on for 10000 milliseconds ~ 10 seconds
// after 10 seconds, pump will be turned off by pump.loop() function
}
}
※ NOTE THAT:
The code above performs the following tasks:
- Debouncing the button, which is supported by the ezButton library. To learn more, please read Why do we need debouncing?
- Turning the pump on for 10 seconds when the button is pressed, and then turning it off, which is supported by the ezOutput library
- All code is non-blocking, which is supported by the ezButton and ezOutput libraries
Detailed Instructions
To get started with ESP8266 on Arduino IDE, follow these steps:
- Check out the how to setup environment for ESP8266 on Arduino IDE tutorial if this is your first time using ESP8266.
- Wire the components as shown in the diagram.
- Connect the ESP8266 board to your computer using a USB cable.
- Open Arduino IDE on your computer.
- Choose the correct ESP8266 board, such as (e.g. NodeMCU 1.0 (ESP-12E Module)), and its respective COM port.
- Connect a USB cable to the ESP8266 and the PC.
- Open the Arduino IDE and select the correct board and port.
- Click to the Libraries icon on the left bar of the Arduino IDE.
- Search for “ezButton” and locate the button library provided by ArduinoGetStarted.
- Press the Install button to install the ezButton library.
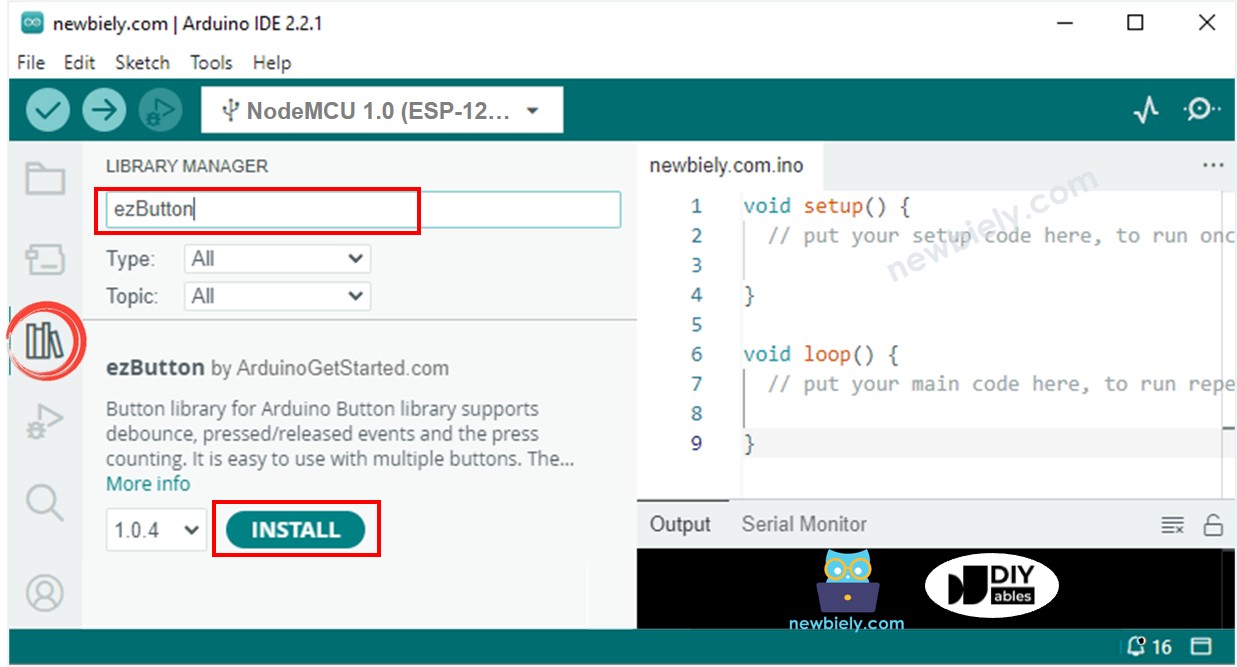
- Look for “ezOutput” and locate the output library provided by ArduinoGetStarted.
- Press the Install button to install ezOutput library.
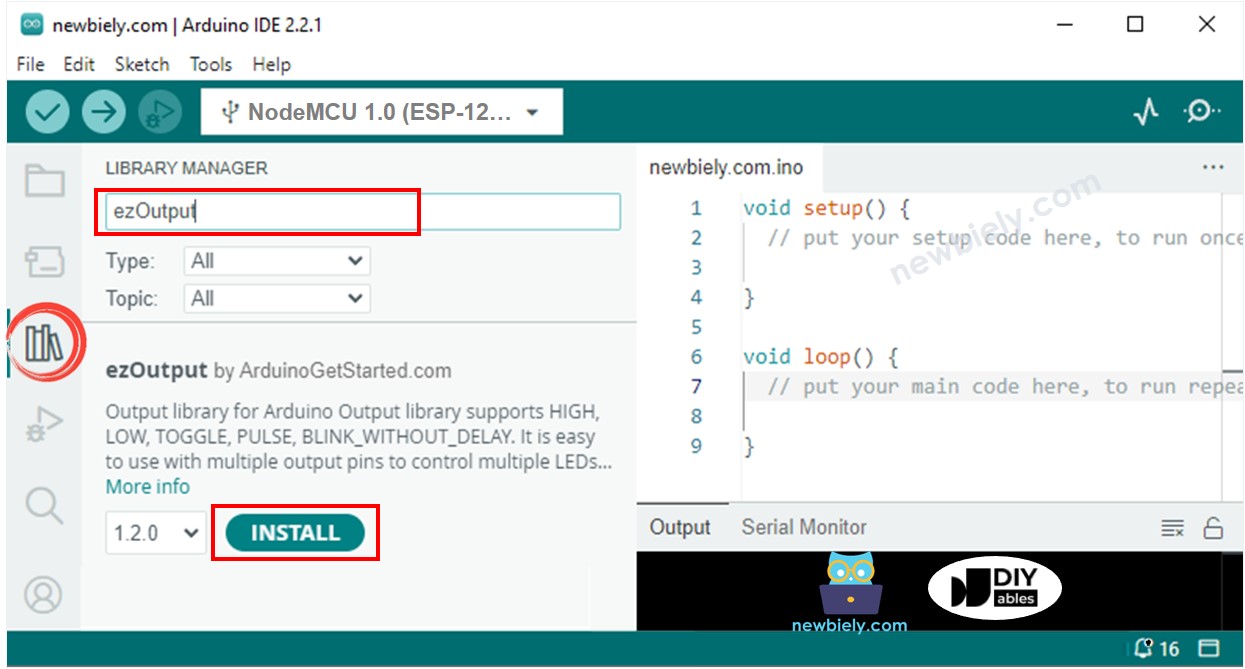
- Copy the code and open it with the Arduino IDE.
- Then, press the Upload button to send the code to the ESP8266.
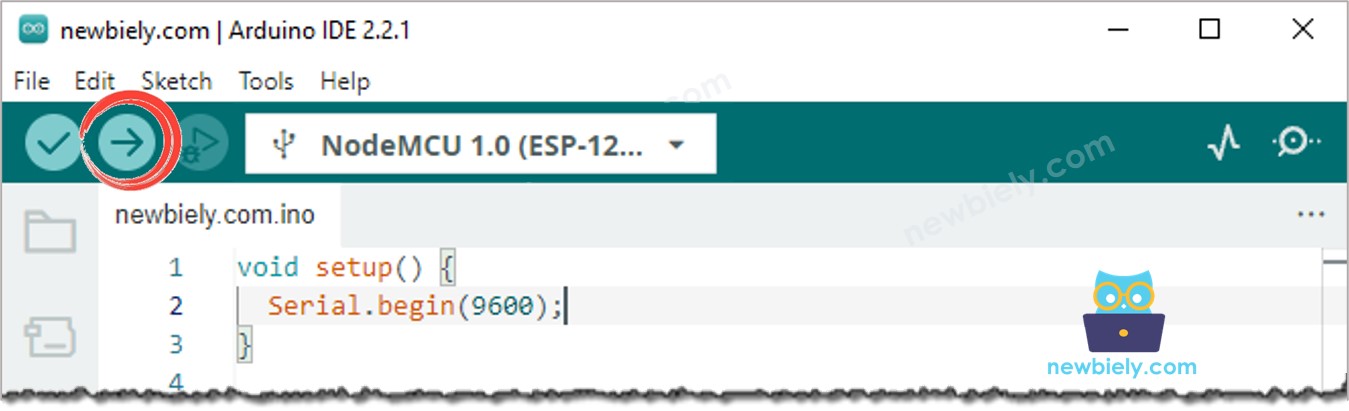
- Press the button
- Check out the pump's condition
Code Explanation
Check out the line-by-line explanation contained in the comments of the source code!