ESP8266 - LM35 Temperature Sensor OLED
This tutorial instructs you how to use ESP8266 to obtain the temperature from an LM35 sensor and show it on an OLED.
Hardware Preparation
Or you can buy the following kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Disclosure: Some of the links provided in this section are Amazon affiliate links. We may receive a commission for any purchases made through these links at no additional cost to you.
Additionally, some of these links are for products from our own brand, DIYables .
Additionally, some of these links are for products from our own brand, DIYables .
Overview of OLED and LM35 Temperature Sensor
If you are unfamiliar with OLED and LM35 Temperature Sensor (including pinout, functionality, and programming), the following tutorials can help:
- ESP8266 - OLED tutorial
- ESP8266 - LM35 Temperature Sensor tutorial
Wiring Diagram
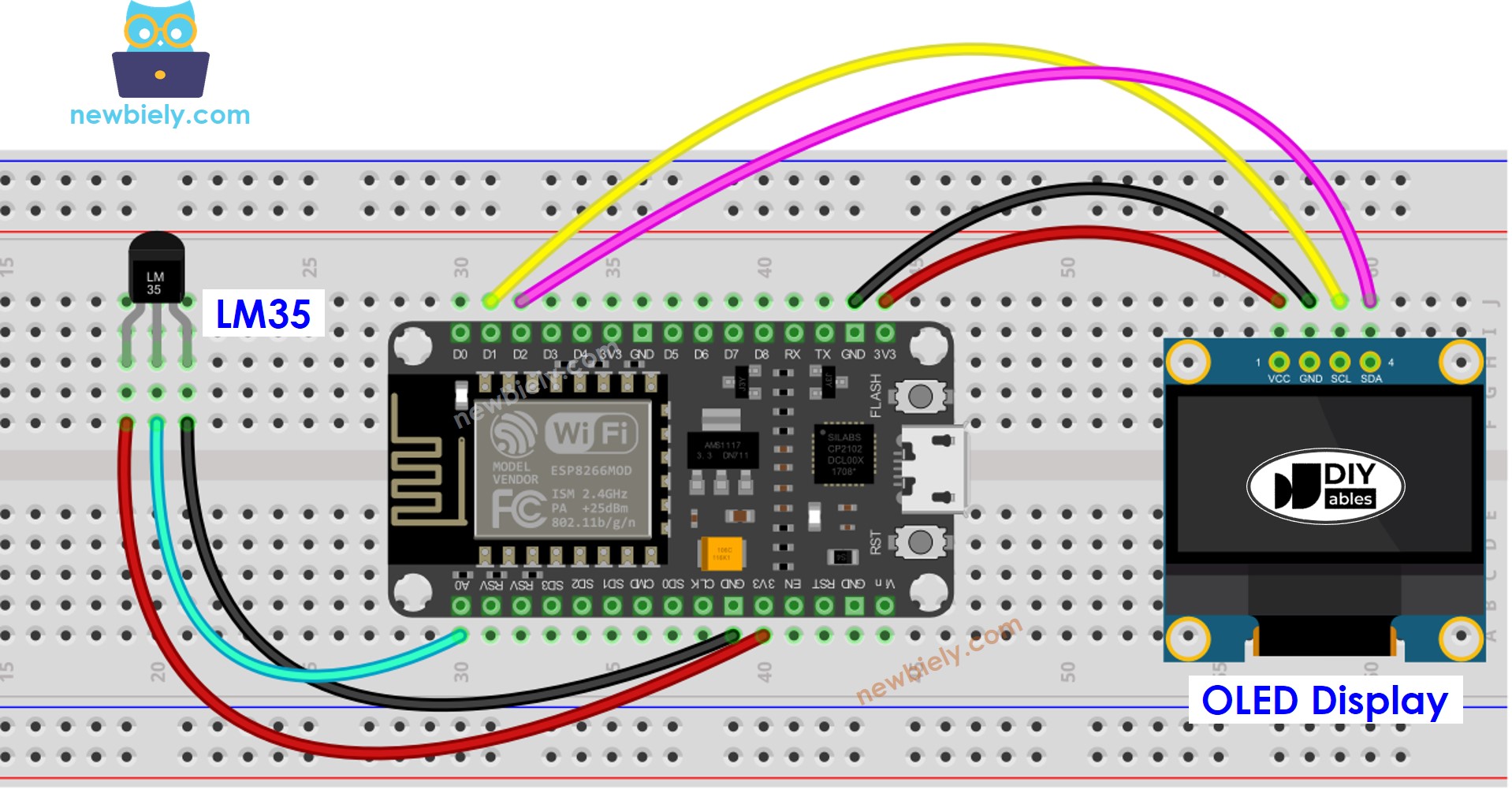
This image is created using Fritzing. Click to enlarge image
See more in ESP8266's pinout and how to supply power to the ESP8266 and other components.
ESP8266 Code - LM35 Temperature Sensor - OLED
/*
* This ESP8266 NodeMCU code was developed by newbiely.com
*
* This ESP8266 NodeMCU code is made available for public use without any restriction
*
* For comprehensive instructions and wiring diagrams, please visit:
* https://newbiely.com/tutorials/esp8266/esp8266-lm35-temperature-sensor-oled
*/
#include <Wire.h>
#include <Adafruit_GFX.h>
#include <Adafruit_SSD1306.h>
#define OLED_WIDTH 128 // OLED display width, in pixels
#define OLED_HEIGHT 64 // OLED display height, in pixels
#define ADC_VREF_mV 5000.0 // in millivolt
#define ADC_RESOLUTION 1024.0
#define PIN_LM35 A0 // pin connected to LM35 temperature sensor
Adafruit_SSD1306 oled(OLED_WIDTH, OLED_HEIGHT, &Wire, -1); // create SSD1306 display object connected to I2C
String temperature_str;
void setup() {
Serial.begin(9600);
// initialize OLED display with address 0x3C for 128x64
if (!oled.begin(SSD1306_SWITCHCAPVCC, 0x3C)) {
Serial.println(F("SSD1306 allocation failed"));
while (true);
}
delay(2000); // wait for initializing
oled.clearDisplay(); // clear display
oled.setTextSize(2); // text size
oled.setTextColor(WHITE); // text color
oled.setCursor(0, 10); // position to display
temperature_str.reserve(10); // to avoid fragmenting memory when using String
}
void loop() {
// get the ADC value from the LM35 temperature sensor
int adcVal = analogRead(PIN_LM35);
// convert the ADC value to voltage in millivolt
float milliVolt = adcVal * (ADC_VREF_mV / ADC_RESOLUTION);
// convert the voltage to the temperature in Celsius
float temperature_C = milliVolt / 10;
temperature_str = String(temperature_C, 2); // two decimal places
temperature_str += char(247) + String("C");
Serial.println(temperature_str); // print the temperature in Celsius to Serial Monitor
oled_display_center(temperature_str); // display temperature on OLED
}
void oled_display_center(String text) {
int16_t x1;
int16_t y1;
uint16_t width;
uint16_t height;
oled.getTextBounds(text, 0, 0, &x1, &y1, &width, &height);
// center the display both horizontally and vertically
oled.clearDisplay(); // clear display
oled.setCursor((OLED_WIDTH - width) / 2, (OLED_HEIGHT - height) / 2);
oled.println(text); // text to display
oled.display();
}
Detailed Instructions
To get started with ESP8266 on Arduino IDE, follow these steps:
- Check out the how to setup environment for ESP8266 on Arduino IDE tutorial if this is your first time using ESP8266.
- Wire the components as shown in the diagram.
- Connect the ESP8266 board to your computer using a USB cable.
- Open Arduino IDE on your computer.
- Choose the correct ESP8266 board, such as (e.g. NodeMCU 1.0 (ESP-12E Module)), and its respective COM port.
- Click to the Libraries icon on the left bar of the Arduino IDE.
- Search for “SSD1306” and locate the SSD1306 library from Adafruit.
- Click the Install button to add the library.
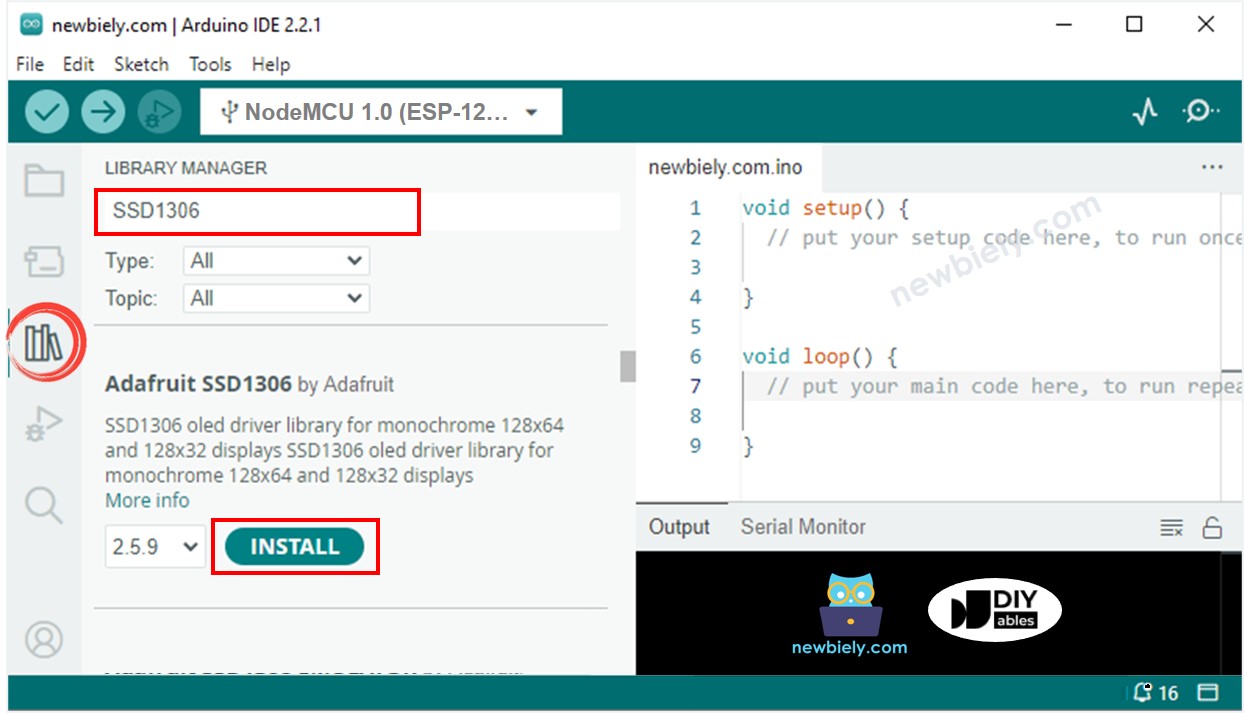
- You will be prompted to install some additional library dependencies.
- To install all of them, click the Install All button.
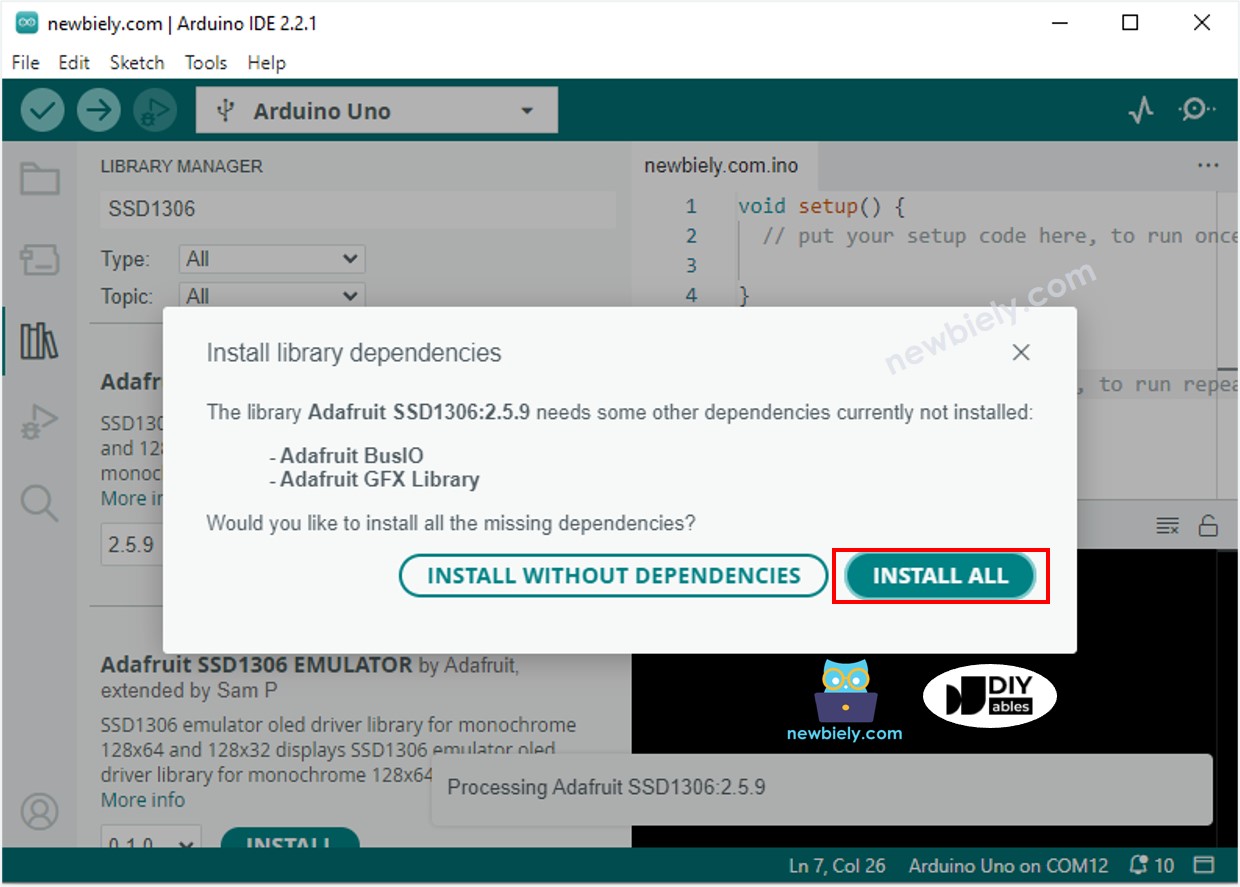
- Copy the code above and open it in the Arduino IDE.
- Click the Upload button in the Arduino IDE to send the code to the ESP8266.
- Place the sensor in hot and cold water or hold it in your hand.
- Check out the results on the OLED display and the Serial Monitor.
※ NOTE THAT:
The code in question will center the text both horizontally and vertically on an OLED display.