ESP8266 - Touch Sensor
This tutorial instructs you how to use the capacitive touch sensor with ESP8266. In detail, we will learn:
- How the touch sensor works
- How to connect the touch sensor to ESP8266
- How to program ESP8266 to read the state from the touch sensor
- How to program ESP8266 to detect the touching/untouching event.
Hardware Preparation
1 | × | ESP8266 NodeMCU | |
1 | × | USB Cable Type-C | |
1 | × | Touch Sensor | |
1 | × | Jumper Wires | |
1 | × | Breadboard | |
1 | × | (Recommended) Screw Terminal Expansion Board for ESP8266 | |
1 | × | (Recommended) Power Splitter For ESP8266 Type-C |
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables .
Overview of Touch Sensor
A capacitive touch sensor, also known as a touch button or touch switch, is commonly used to control devices, such as a touchable lamp. It has the same purpose as a button, but is used in place of a button on many modern devices due to its sleek appearance.
The Touch Sensor Pinout
The touch sensor has three pins:
- GND pin: This should be connected to the ground voltage (0V).
- VCC pin: This should be connected to the VCC voltage (5V or 3.3V).
- SIGNAL pin: This is an output pin. It will be LOW when not touched and HIGH when touched. This pin should be connected to an ESP8266 input pin.
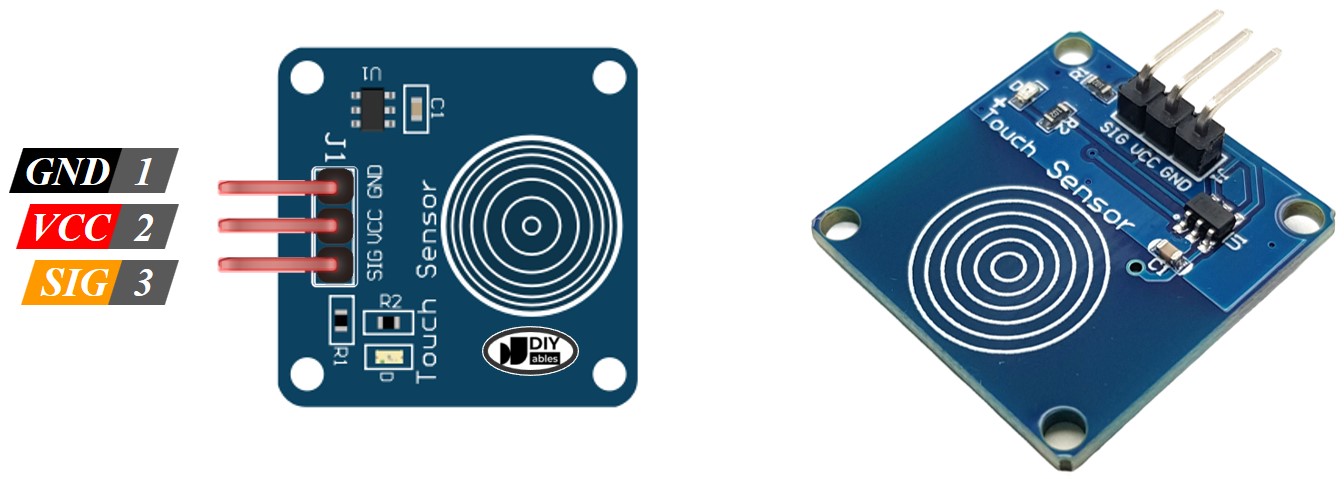
How It Works
- When the sensor is not being interacted with, the SIGNAL pin of the sensor is at a LOW level.
- However, when the sensor is touched, the SIGNAL pin of the sensor is at a HIGH level.
ESP8266 - Touch Sensor
The SIGNAL pin of the touch sensor is connected to an input pin of an ESP8266. We can determine if the touch sensor has been touched by examining the state of the ESP8266's pin.
Wiring Diagram
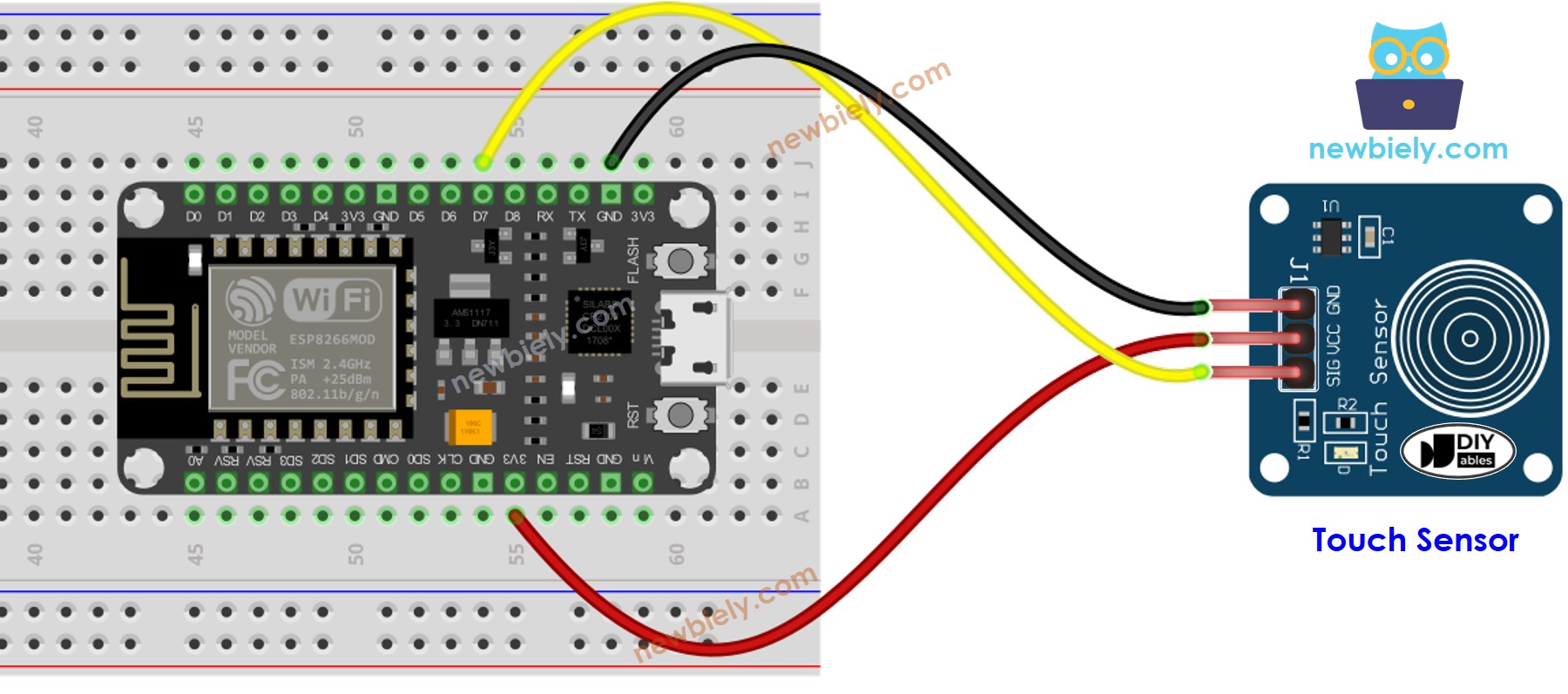
This image is created using Fritzing. Click to enlarge image
See more in ESP8266's pinout and how to supply power to the ESP8266 and other components.
How To Program For Touch Sensor
- Set the ESP8266 pin to the digital input mode using the pinMode() function. For example:
- Read the state of the ESP8266 pin through the digitalRead() function.
※ NOTE THAT:
There are two commonly used scenarios:
- The first: If the input state is HIGH, carry out one action. If the input state is LOW, execute the opposite action.
- The second: If the input state changes from LOW to HIGH (or HIGH to LOW), perform an action.
We select one of them based on the purpose of the application. For example, if we want to use a touch sensor to control an LED:
- If the goal is to have the LED be ON when the sensor is touched and OFF when the sensor is NOT touched, we SHOULD use the first scenario.
- If the goal is to have the LED toggle between ON and OFF each time we touch the sensor, we SHOULD use the second scenario.
Touch Sensor - ESP8266 Code
The tutorial provides two sample code:
- ESP8266 reads the value from the touch sensor and prints it on the Serial Monitor.
- ESP8266 detects whether the sensor is touched or released.
ESP8266 reads the value from the touch sensor and print to the Serial Monitor
Detailed Instructions
To get started with ESP8266 on Arduino IDE, follow these steps:
- Check out the how to setup environment for ESP8266 on Arduino IDE tutorial if this is your first time using ESP8266.
- Wire the components as shown in the diagram.
- Connect the ESP8266 board to your computer using a USB cable.
- Open Arduino IDE on your computer.
- Choose the correct ESP8266 board, such as (e.g. NodeMCU 1.0 (ESP-12E Module)), and its respective COM port.
- Copy the code and open it in the Arduino IDE.
- Click the Upload button in the IDE to transfer the code to the ESP8266.
- Place your finger on the sensor and then remove it.
- Check the output in the Serial Monitor.
ESP8266 detects the sensor is touched or released
Detailed Instructions
- Copy the code and open it with the Arduino IDE.
- Click the Upload button on the Arduino IDE to compile and upload the code to the ESP8266.
- Keep your finger touching the sensor.
- Check out the result on the Serial Monitor.
- Remove your finger from the sensor.
- Check the Serial Monitor for the result.