ESP8266 - DHT11
This tutorial instructs you how to use ESP8266 to read the temperature and humidity from DHT11 sensor. In detail, we will learn:
- How to connect ESP8266 to the DHT11 sensor
- How to program ESP8266 to read temperature and humidity values from a DHT11
Hardware Preparation
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables .
Overview of DHT11 Temperature and Humidity Sensor
The Temperature and Humidity Sensor Pinout
The DHT11 on the market comes with two forms: sensor and module.
The DHT11 sensors has four pins:
- GND pin: must be connected to GND (0V)
- VCC pin: must be connected to VCC (5V)
- DATA pin: used for communication between the sensor and ESP8266
- NC pin: Not connected, this pin can be disregarded
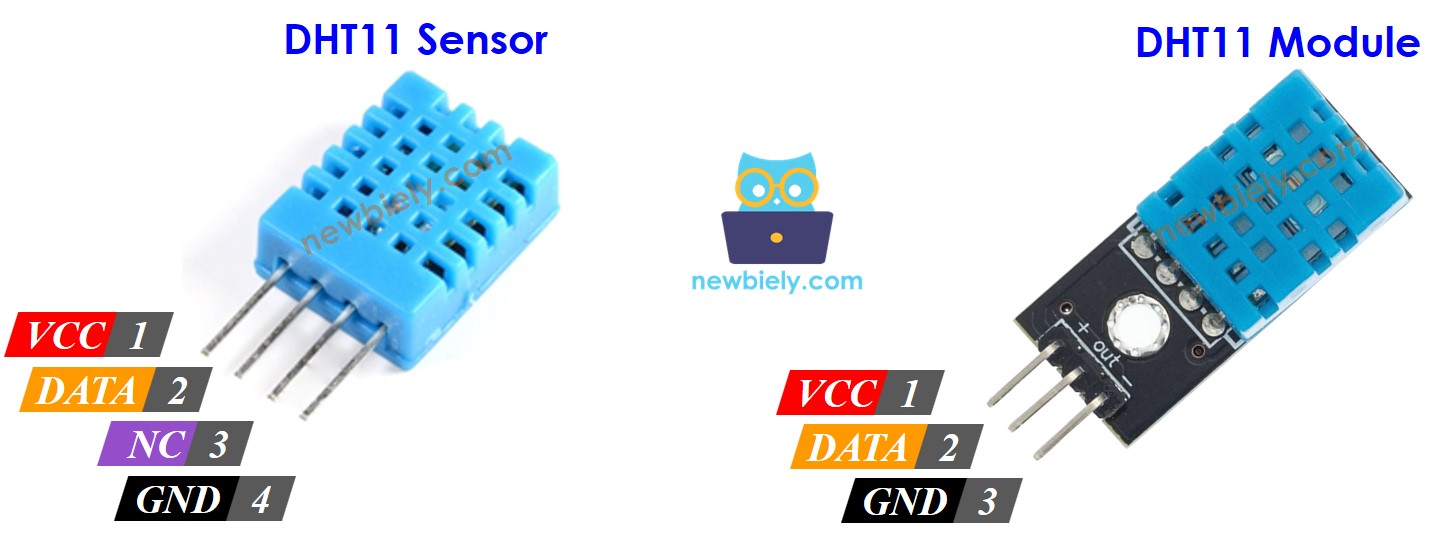
The DHT11 module has three pins:
- GND pin (-): must be connected to GND (0V)
- VCC pin (+): must be connected to VCC (5V)
- OUT pin: used for communication between the sensor and ESP8266
※ NOTE THAT:
The arrangement of the pins on a module may be different depending on the manufacturer. It is IMPERATIVE to use the labels printed on the module for reference. Be sure to take a close look!
Wiring Diagram
ESP8266 - DHT11 Sensor Wiring
A resistor with a value between 5K and 10K Ohms is necessary to maintain the data line at a high level, thus enabling communication between the sensor and the ESP8266.
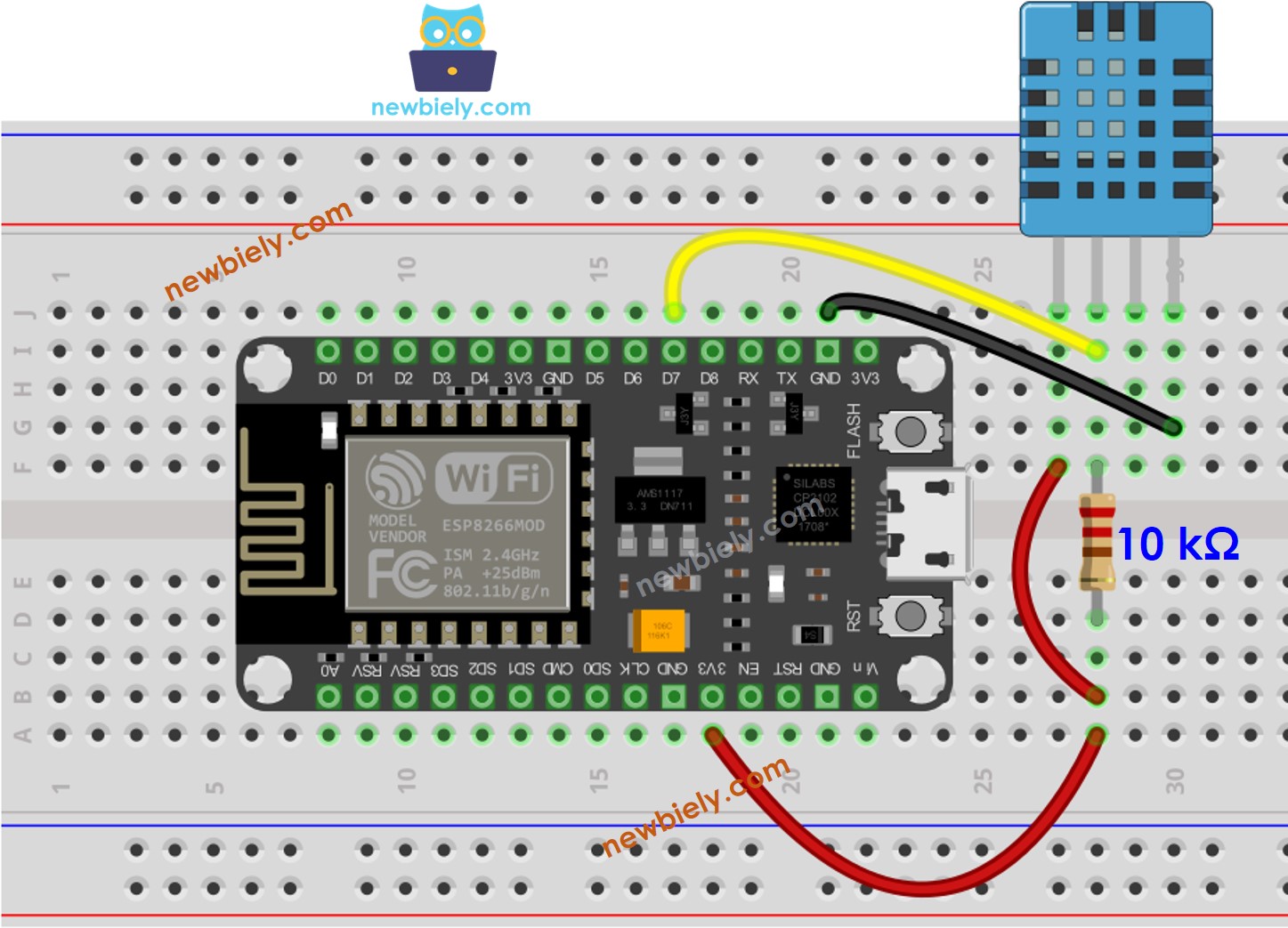
This image is created using Fritzing. Click to enlarge image
ESP8266 - DHT11 Module Wiring
Most DHT11 sensor modules have an integrated resistor, thus eliminating the need for additional wiring or soldering.
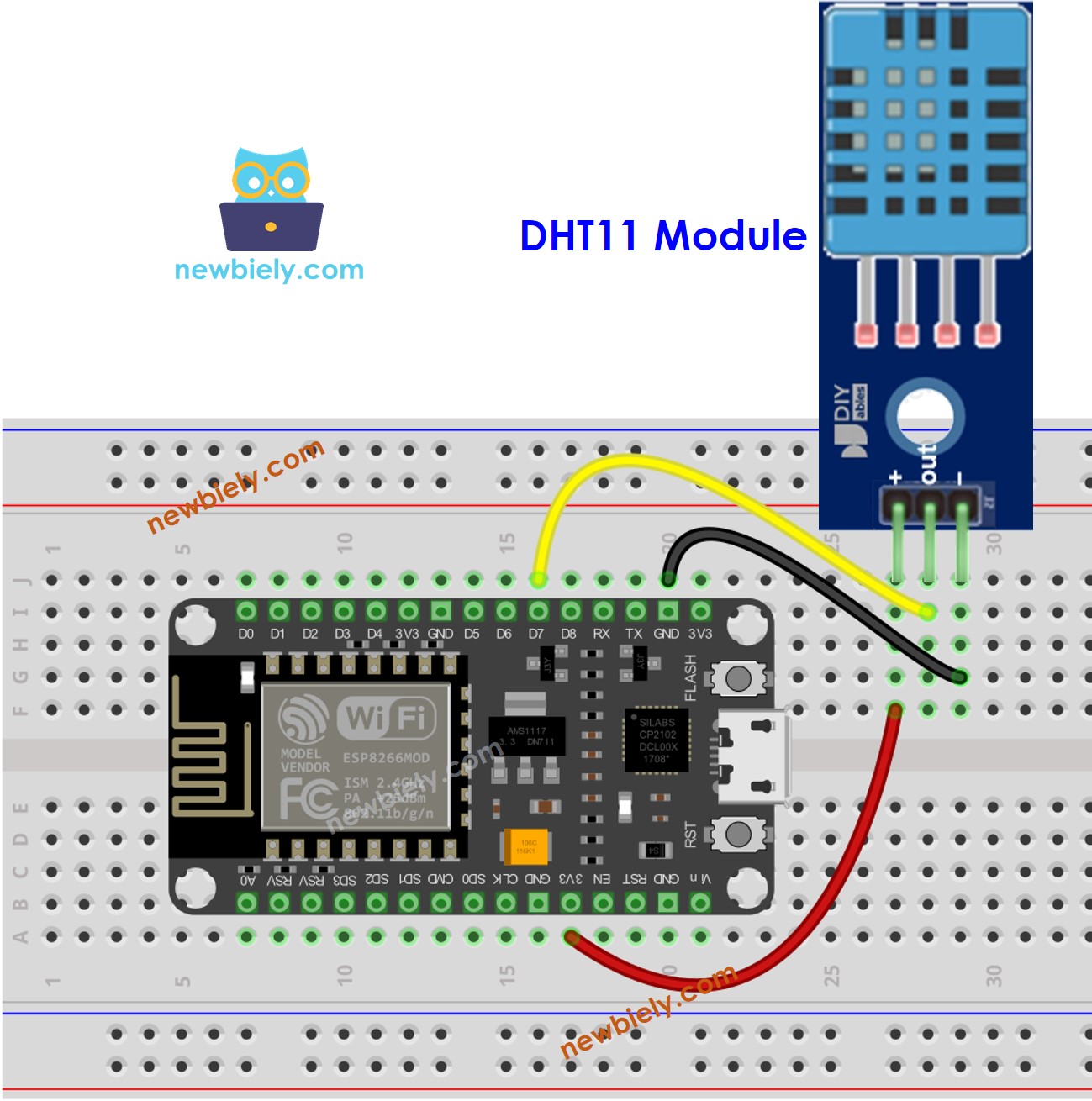
This image is created using Fritzing. Click to enlarge image
See more in ESP8266's pinout and how to supply power to the ESP8266 and other components.
How To Program For DHT11 Temperature Sensor
- To begin, the library must be included:
- Specify the ESP8266 pin that is connected to the DHT11 sensor.
- Specify the type of sensor: DHT11
- Create a DHT object.
- Begin the setup of the sensor:
- Read the humidity value.
- Read the temperature in Celsius.
- Read the temperature in Fahrenheit.
ESP8266 Code for DHT11
Detailed Instructions
To get started with ESP8266 on Arduino IDE, follow these steps:
- Check out the how to setup environment for ESP8266 on Arduino IDE tutorial if this is your first time using ESP8266.
- Wire the components as shown in the diagram.
- Connect the ESP8266 board to your computer using a USB cable.
- Open Arduino IDE on your computer.
- Choose the correct ESP8266 board, such as (e.g. NodeMCU 1.0 (ESP-12E Module)), and its respective COM port.
- Connect a USB cable to the ESP8266 and the PC.
- Open the Arduino IDE, select the correct board and port.
- Click to the Libraries icon on the left bar of the Arduino IDE.
- Search for “DHT”, and then locate the DHT sensor library by Adafruit.
- Press the Install button to complete the installation.
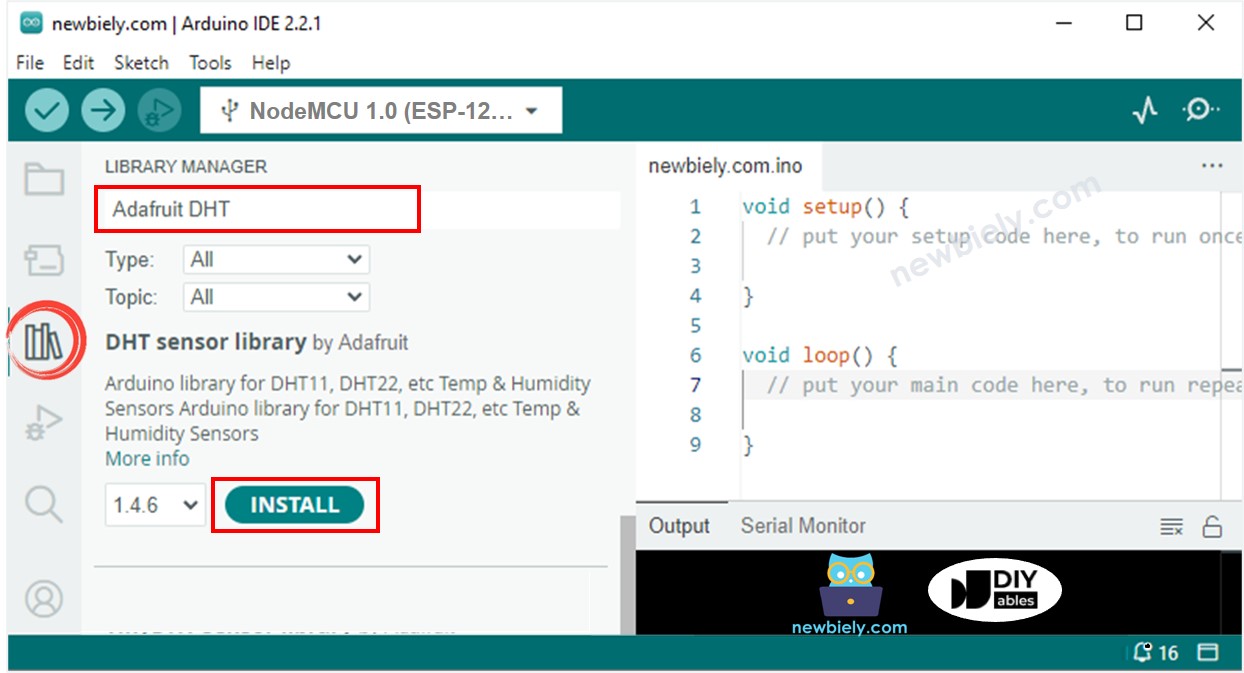
- You will be prompted to install some other library dependencies.
- To install all of these libraries, click the Install All button.
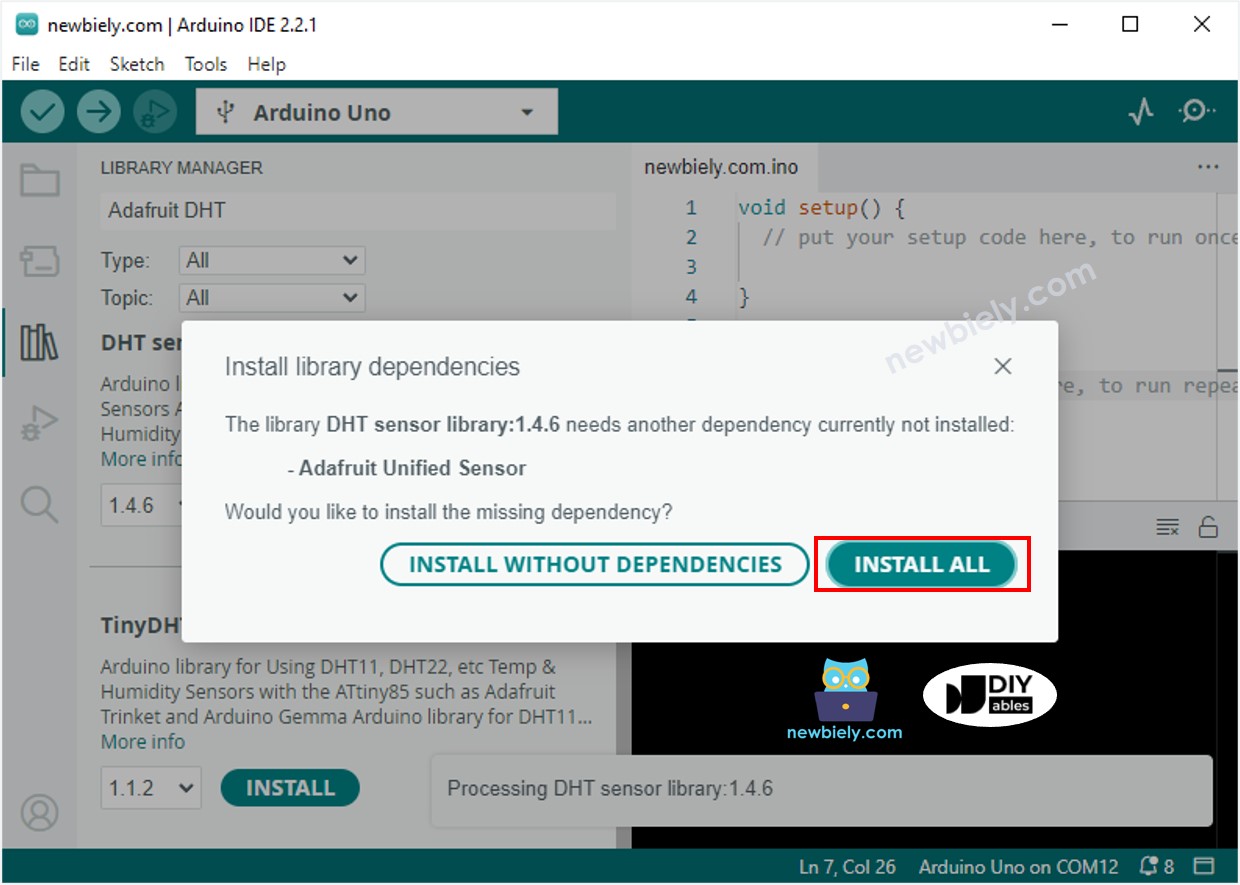
- Copy the code that corresponds to the sensor you have and open it with the Arduino IDE.
- Click the Upload button in the Arduino IDE to compile and upload the code to the ESP8266.
- Change the temperature of the environment around the sensor.
- Check out the result on the Serial Monitor.
Video Tutorial
Additional Knowledge
Let's compare DHT11 and DHT22 sensors.
The commons between DHT11 and DHT22
- Pinouts remain the same.
- The wiring to ESP8266 is unaltered.
- Programming, with the use of a library, is comparable, only requiring one line of code to be altered.
The differences between DHT11 and DHT22
DHT11 | DHT22 | |
---|---|---|
Price | ultra low cost | low cost |
Temperature Range | 0°C to 50°C | -40°C to 80°C |
Temperature Accuracy | ± 2°C | ± 0.5°C |
Humidity Range | 20% to 80% | 0% to 100% |
Humidity Accuracy | 5% | ± 2 to 5% |
Reading Rate | 1Hz (once every second) | 0.5Hz (once every 2 seconds) |
Body size | 15.5mm x 12mm x 5.5mm | 15.1mm x 25mm x 7.7mm |
Evidently, the DHT22 is more precise than the DHT11, has a wider range of measurements, yet costs more.