ESP8266 - Force Sensor
This tutorial instructs you how to use ESP8266 with the force sensor. In detail, we will learn:
- How the force sensor work
- How to connect the force sensor to ESP8266
- How to program ESP8266 to read the value from the force sensor
As a typical application, You can put your things on the force sensor, and then if the ESP8266 detects a change in force, it means someone has grabbed your belongings.
Hardware Preparation
Or you can buy the following kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables .
Overview of Force Sensor
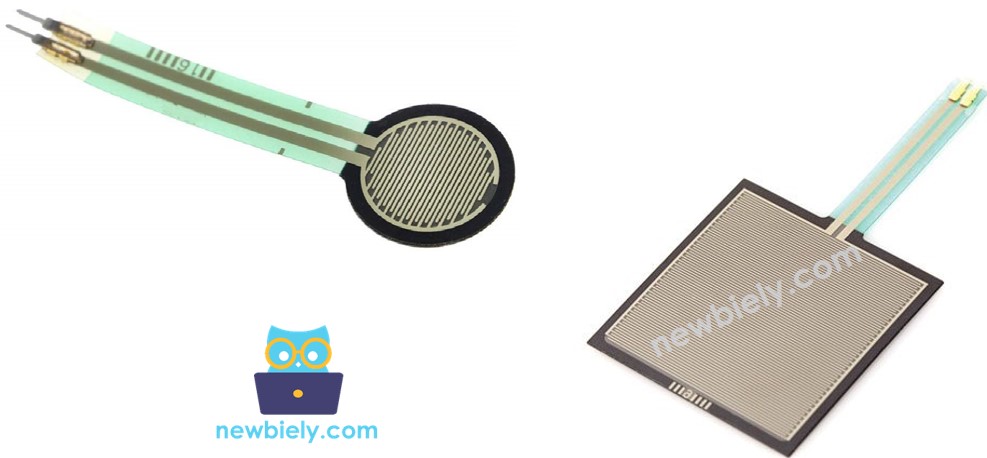
The force sensor is referred to as a force sensing resistor, force sensitive resistor, or simply FSR. It is essentially a resistor that alters its resistive value in response to the amount of pressure applied. The force sensor has the following characteristics:
- It is inexpensive and simple to use.
- It is effective at detecting physical pressure and squeezing.
- It is not suitable for determining the weight it is bearing.
The force sensor is used in a variety of portable electronics, such as electronic drums, mobile phones, and handheld gaming devices.
The Force Sensor Pinout
A force sensor has two pins, and due to it being a type of resistor, there is no need to differentiate between them. Both pins are symmetrical.
How It Works
The force sensor is essentially a resistor that alters its resistance depending on the amount of pressure applied. The greater the pressure, the lower the resistance between the two pins will become.
Wiring Diagram
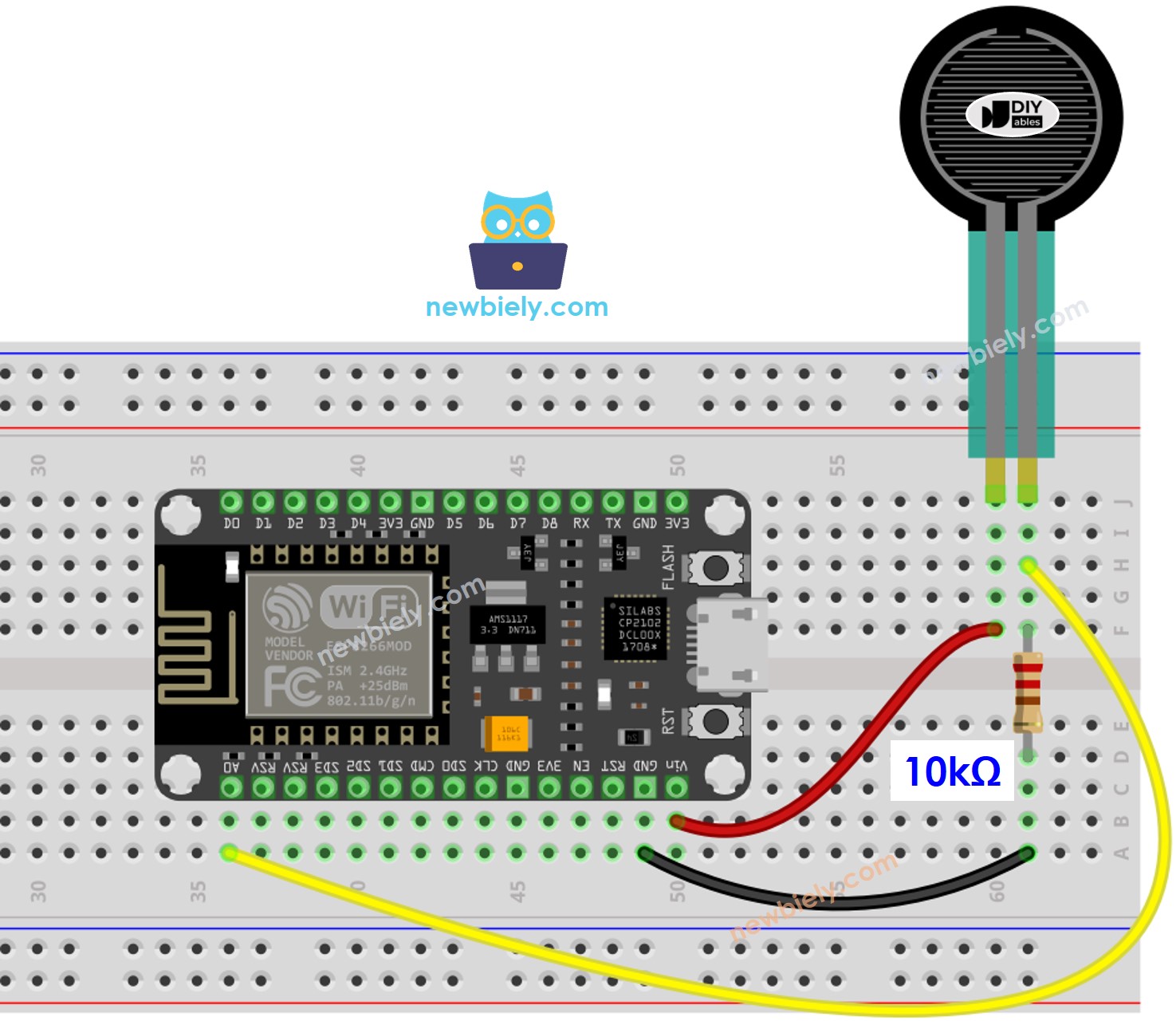
This image is created using Fritzing. Click to enlarge image
See more in ESP8266's pinout and how to supply power to the ESP8266 and other components.
How To Program For Force Sensor
We can connect the force sensor to an analog input pin of ESP8266, and then use analogRead() function to read the analog value from the pin. This allows us to determine how much the force sensor has been pressed.
ESP8266 Code
Detailed Instructions
To get started with ESP8266 on Arduino IDE, follow these steps:
- Check out the how to setup environment for ESP8266 on Arduino IDE tutorial if this is your first time using ESP8266.
- Wire the components as shown in the diagram.
- Connect the ESP8266 board to your computer using a USB cable.
- Open Arduino IDE on your computer.
- Choose the correct ESP8266 board, such as (e.g. NodeMCU 1.0 (ESP-12E Module)), and its respective COM port.
- Copy the code and open it with the Arduino IDE.
- Click the Upload button in the IDE to transfer the code to the ESP8266.
- Press the force sensor.
- Check the output on the Serial Monitor.
※ NOTE THAT:
This tutorial uses the analogRead() function to get data from an ADC (Analog-to-Digital Converter) that's connected to a sensor or another part. The ESP8266's ADC works well for projects where you don't need very precise readings. But remember, the ESP8266's ADC isn't very accurate for detailed measurements. If your project needs to be very precise, you might want to use a separate ADC like the ADS1115 with the ESP8266, or use Arduino like the Arduino Uno R4 WiFi, which has a more reliable ADC.